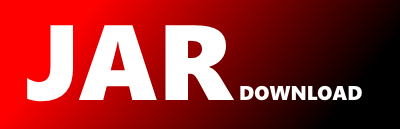
net.morimekta.tiny.server.http.TinyHttpHandler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tiny-server Show documentation
Show all versions of tiny-server Show documentation
A tiny server starter for easy and minimal microservice handling. This is based on the
assumption that the microservice does not use the admin HTTP server for anything but
kubernetes status management.
Dependencies are kept at a minimum, but should cover the basic needs of any microservice.
package net.morimekta.tiny.server.http;
import com.sun.net.httpserver.HttpExchange;
import com.sun.net.httpserver.HttpHandler;
import java.io.IOException;
import java.time.ZonedDateTime;
import java.time.format.DateTimeFormatter;
/**
* Class simplifying the HttpHandler in a similar fashion to the jakarta
* HttpServlet
class. Just implement the matching method to
* the HTTP method you need served.
*/
public abstract class TinyHttpHandler implements HttpHandler {
@Override
public void handle(HttpExchange exchange) throws IOException {
try {
exchange.getResponseHeaders().set("Date", DateTimeFormatter.RFC_1123_DATE_TIME.format(ZonedDateTime.now()));
exchange.getResponseHeaders().set("Server", "tiny-server");
switch (exchange.getRequestMethod()) {
case "GET":
doGet(exchange);
break;
case "POST":
doPost(exchange);
break;
case "HEAD":
doHead(exchange);
break;
case "OPTIONS":
doOptions(exchange);
break;
case "CONNECT":
toConnect(exchange);
break;
case "TRACE":
doTrace(exchange);
break;
case "PUT":
doPut(exchange);
break;
case "PATCH":
doPatch(exchange);
break;
case "DELETE":
doDelete(exchange);
break;
default: {
exchange.sendResponseHeaders(TinyHttpStatus.SC_INVALID_REQUEST, 0);
exchange.close();
}
}
} catch (Exception e) {
if (exchange.getResponseCode() <= 0) {
exchange.sendResponseHeaders(TinyHttpStatus.SC_INTERNAL, 0);
exchange.getResponseBody().close();
}
}
}
/**
* Handle a GET request.
*
* @param exchange The HTTP exchange.
* @throws IOException If failed to handle the request.
*/
protected void doGet(HttpExchange exchange) throws IOException {
exchange.sendResponseHeaders(TinyHttpStatus.SC_METHOD_NOT_ALLOWED, 0);
}
/**
* Handle a POST request.
*
* @param exchange The HTTP exchange.
* @throws IOException If failed to handle the request.
*/
protected void doPost(HttpExchange exchange) throws IOException {
exchange.sendResponseHeaders(TinyHttpStatus.SC_METHOD_NOT_ALLOWED, 0);
}
/**
* Handle a HEAD request.
*
* @param exchange The HTTP exchange.
* @throws IOException If failed to handle the request.
*/
protected void doHead(HttpExchange exchange) throws IOException {
exchange.sendResponseHeaders(TinyHttpStatus.SC_METHOD_NOT_ALLOWED, 0);
}
/**
* Handle an OPTIONS request.
*
* @param exchange The HTTP exchange.
* @throws IOException If failed to handle the request.
*/
protected void doOptions(HttpExchange exchange) throws IOException {
// allow OPTIONS, GET and PORT by default.
exchange.getResponseHeaders().set("Allow", "OPTIONS, GET, POST");
exchange.sendResponseHeaders(TinyHttpStatus.SC_NO_CONTENT, 0);
}
/**
* Handle a CONNECT request.
*
* @param exchange The HTTP exchange.
* @throws IOException If failed to handle the request.
*/
protected void toConnect(HttpExchange exchange) throws IOException {
exchange.sendResponseHeaders(TinyHttpStatus.SC_METHOD_NOT_ALLOWED, 0);
}
/**
* Handle a TRACE request.
*
* @param exchange The HTTP exchange.
* @throws IOException If failed to handle the request.
*/
protected void doTrace(HttpExchange exchange) throws IOException {
exchange.sendResponseHeaders(TinyHttpStatus.SC_METHOD_NOT_ALLOWED, 0);
}
/**
* Handle a PUT request.
*
* @param exchange The HTTP exchange.
* @throws IOException If failed to handle the request.
*/
protected void doPut(HttpExchange exchange) throws IOException {
exchange.sendResponseHeaders(TinyHttpStatus.SC_METHOD_NOT_ALLOWED, 0);
}
/**
* Handle a PATCH request.
*
* @param exchange The HTTP exchange.
* @throws IOException If failed to handle the request.
*/
protected void doPatch(HttpExchange exchange) throws IOException {
exchange.sendResponseHeaders(TinyHttpStatus.SC_METHOD_NOT_ALLOWED, 0);
}
/**
* Handle a DELETE request.
*
* @param exchange The HTTP exchange.
* @throws IOException If failed to handle the request.
*/
protected void doDelete(HttpExchange exchange) throws IOException {
exchange.sendResponseHeaders(TinyHttpStatus.SC_METHOD_NOT_ALLOWED, 0);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy