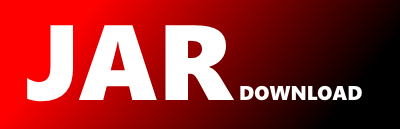
net.mossol.bot.service.impl.SimpleMatcherServiceImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of line_bot_mossol-lib Show documentation
Show all versions of line_bot_mossol-lib Show documentation
Line Bot Mossol (line_bot_mossol-lib)
package net.mossol.bot.service.impl;
import static net.mossol.bot.model.TextType.LEAVE_CHAT;
import static net.mossol.bot.model.TextType.SELECT_MENU_D;
import static net.mossol.bot.model.TextType.SELECT_MENU_J;
import static net.mossol.bot.model.TextType.SELECT_MENU_K;
import static net.mossol.bot.model.TextType.SHOW_MENU_D;
import static net.mossol.bot.model.TextType.SHOW_MENU_J;
import static net.mossol.bot.model.TextType.SHOW_MENU_K;
import static net.mossol.bot.model.TextType.TEXT;
import java.util.Map;
import javax.annotation.PostConstruct;
import javax.annotation.Resource;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.core.annotation.Order;
import org.springframework.stereotype.Service;
import org.springframework.util.StringUtils;
import net.mossol.bot.model.ReplyMessage;
import net.mossol.bot.model.SimpleText;
import net.mossol.bot.service.MatcherService;
import net.mossol.bot.service.MenuServiceHandler;
import net.mossol.bot.model.MenuType;
import com.linecorp.centraldogma.client.Watcher;
@Service
@Order(1)
public class SimpleMatcherServiceImpl implements MatcherService {
private static final Logger logger = LoggerFactory.getLogger(SimpleMatcherServiceImpl.class);
private volatile Map simpleTextContext;
@Resource
private MenuServiceHandler menuServiceHandler;
@Resource
private Watcher
© 2015 - 2024 Weber Informatics LLC | Privacy Policy