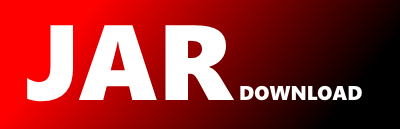
net.named_data.jndn.sync.SyncStateProto Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: sync-state-proto.proto
package net.named_data.jndn.sync;
public final class SyncStateProto {
private SyncStateProto() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
}
public interface SyncStateOrBuilder extends
// @@protoc_insertion_point(interface_extends:net.named_data.jndn.sync.SyncState)
com.google.protobuf.MessageOrBuilder {
/**
* required string name = 1;
*/
boolean hasName();
/**
* required string name = 1;
*/
java.lang.String getName();
/**
* required string name = 1;
*/
com.google.protobuf.ByteString
getNameBytes();
/**
* required .net.named_data.jndn.sync.SyncState.ActionType type = 2;
*/
boolean hasType();
/**
* required .net.named_data.jndn.sync.SyncState.ActionType type = 2;
*/
net.named_data.jndn.sync.SyncStateProto.SyncState.ActionType getType();
/**
* optional .net.named_data.jndn.sync.SyncState.SeqNo seqno = 3;
*/
boolean hasSeqno();
/**
* optional .net.named_data.jndn.sync.SyncState.SeqNo seqno = 3;
*/
net.named_data.jndn.sync.SyncStateProto.SyncState.SeqNo getSeqno();
/**
* optional .net.named_data.jndn.sync.SyncState.SeqNo seqno = 3;
*/
net.named_data.jndn.sync.SyncStateProto.SyncState.SeqNoOrBuilder getSeqnoOrBuilder();
/**
* optional bytes application_info = 4;
*/
boolean hasApplicationInfo();
/**
* optional bytes application_info = 4;
*/
com.google.protobuf.ByteString getApplicationInfo();
}
/**
* Protobuf type {@code net.named_data.jndn.sync.SyncState}
*/
public static final class SyncState extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:net.named_data.jndn.sync.SyncState)
SyncStateOrBuilder {
// Use SyncState.newBuilder() to construct.
private SyncState(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private SyncState(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final SyncState defaultInstance;
public static SyncState getDefaultInstance() {
return defaultInstance;
}
public SyncState getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private SyncState(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
name_ = bs;
break;
}
case 16: {
int rawValue = input.readEnum();
net.named_data.jndn.sync.SyncStateProto.SyncState.ActionType value = net.named_data.jndn.sync.SyncStateProto.SyncState.ActionType.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(2, rawValue);
} else {
bitField0_ |= 0x00000002;
type_ = value;
}
break;
}
case 26: {
net.named_data.jndn.sync.SyncStateProto.SyncState.SeqNo.Builder subBuilder = null;
if (((bitField0_ & 0x00000004) == 0x00000004)) {
subBuilder = seqno_.toBuilder();
}
seqno_ = input.readMessage(net.named_data.jndn.sync.SyncStateProto.SyncState.SeqNo.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(seqno_);
seqno_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000004;
break;
}
case 34: {
bitField0_ |= 0x00000008;
applicationInfo_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return net.named_data.jndn.sync.SyncStateProto.internal_static_net_named_data_jndn_sync_SyncState_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return net.named_data.jndn.sync.SyncStateProto.internal_static_net_named_data_jndn_sync_SyncState_fieldAccessorTable
.ensureFieldAccessorsInitialized(
net.named_data.jndn.sync.SyncStateProto.SyncState.class, net.named_data.jndn.sync.SyncStateProto.SyncState.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public SyncState parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new SyncState(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
/**
* Protobuf enum {@code net.named_data.jndn.sync.SyncState.ActionType}
*/
public enum ActionType
implements com.google.protobuf.ProtocolMessageEnum {
/**
* UPDATE = 0;
*/
UPDATE(0, 0),
/**
* DELETE = 1;
*/
DELETE(1, 1),
/**
* OTHER = 2;
*/
OTHER(2, 2),
;
/**
* UPDATE = 0;
*/
public static final int UPDATE_VALUE = 0;
/**
* DELETE = 1;
*/
public static final int DELETE_VALUE = 1;
/**
* OTHER = 2;
*/
public static final int OTHER_VALUE = 2;
public final int getNumber() { return value; }
public static ActionType valueOf(int value) {
switch (value) {
case 0: return UPDATE;
case 1: return DELETE;
case 2: return OTHER;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static com.google.protobuf.Internal.EnumLiteMap
internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public ActionType findValueByNumber(int number) {
return ActionType.valueOf(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(index);
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return net.named_data.jndn.sync.SyncStateProto.SyncState.getDescriptor().getEnumTypes().get(0);
}
private static final ActionType[] VALUES = values();
public static ActionType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int index;
private final int value;
private ActionType(int index, int value) {
this.index = index;
this.value = value;
}
// @@protoc_insertion_point(enum_scope:net.named_data.jndn.sync.SyncState.ActionType)
}
public interface SeqNoOrBuilder extends
// @@protoc_insertion_point(interface_extends:net.named_data.jndn.sync.SyncState.SeqNo)
com.google.protobuf.MessageOrBuilder {
/**
* required uint64 seq = 1;
*/
boolean hasSeq();
/**
* required uint64 seq = 1;
*/
long getSeq();
/**
* required uint64 session = 2;
*/
boolean hasSession();
/**
* required uint64 session = 2;
*/
long getSession();
}
/**
* Protobuf type {@code net.named_data.jndn.sync.SyncState.SeqNo}
*/
public static final class SeqNo extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:net.named_data.jndn.sync.SyncState.SeqNo)
SeqNoOrBuilder {
// Use SeqNo.newBuilder() to construct.
private SeqNo(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private SeqNo(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final SeqNo defaultInstance;
public static SeqNo getDefaultInstance() {
return defaultInstance;
}
public SeqNo getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private SeqNo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
seq_ = input.readUInt64();
break;
}
case 16: {
bitField0_ |= 0x00000002;
session_ = input.readUInt64();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return net.named_data.jndn.sync.SyncStateProto.internal_static_net_named_data_jndn_sync_SyncState_SeqNo_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return net.named_data.jndn.sync.SyncStateProto.internal_static_net_named_data_jndn_sync_SyncState_SeqNo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
net.named_data.jndn.sync.SyncStateProto.SyncState.SeqNo.class, net.named_data.jndn.sync.SyncStateProto.SyncState.SeqNo.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public SeqNo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new SeqNo(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
public static final int SEQ_FIELD_NUMBER = 1;
private long seq_;
/**
* required uint64 seq = 1;
*/
public boolean hasSeq() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required uint64 seq = 1;
*/
public long getSeq() {
return seq_;
}
public static final int SESSION_FIELD_NUMBER = 2;
private long session_;
/**
* required uint64 session = 2;
*/
public boolean hasSession() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required uint64 session = 2;
*/
public long getSession() {
return session_;
}
private void initFields() {
seq_ = 0L;
session_ = 0L;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasSeq()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasSession()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeUInt64(1, seq_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeUInt64(2, session_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(1, seq_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(2, session_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static net.named_data.jndn.sync.SyncStateProto.SyncState.SeqNo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static net.named_data.jndn.sync.SyncStateProto.SyncState.SeqNo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static net.named_data.jndn.sync.SyncStateProto.SyncState.SeqNo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static net.named_data.jndn.sync.SyncStateProto.SyncState.SeqNo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static net.named_data.jndn.sync.SyncStateProto.SyncState.SeqNo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static net.named_data.jndn.sync.SyncStateProto.SyncState.SeqNo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static net.named_data.jndn.sync.SyncStateProto.SyncState.SeqNo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static net.named_data.jndn.sync.SyncStateProto.SyncState.SeqNo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static net.named_data.jndn.sync.SyncStateProto.SyncState.SeqNo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static net.named_data.jndn.sync.SyncStateProto.SyncState.SeqNo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(net.named_data.jndn.sync.SyncStateProto.SyncState.SeqNo prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code net.named_data.jndn.sync.SyncState.SeqNo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:net.named_data.jndn.sync.SyncState.SeqNo)
net.named_data.jndn.sync.SyncStateProto.SyncState.SeqNoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return net.named_data.jndn.sync.SyncStateProto.internal_static_net_named_data_jndn_sync_SyncState_SeqNo_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return net.named_data.jndn.sync.SyncStateProto.internal_static_net_named_data_jndn_sync_SyncState_SeqNo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
net.named_data.jndn.sync.SyncStateProto.SyncState.SeqNo.class, net.named_data.jndn.sync.SyncStateProto.SyncState.SeqNo.Builder.class);
}
// Construct using net.named_data.jndn.sync.SyncStateProto.SyncState.SeqNo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
seq_ = 0L;
bitField0_ = (bitField0_ & ~0x00000001);
session_ = 0L;
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return net.named_data.jndn.sync.SyncStateProto.internal_static_net_named_data_jndn_sync_SyncState_SeqNo_descriptor;
}
public net.named_data.jndn.sync.SyncStateProto.SyncState.SeqNo getDefaultInstanceForType() {
return net.named_data.jndn.sync.SyncStateProto.SyncState.SeqNo.getDefaultInstance();
}
public net.named_data.jndn.sync.SyncStateProto.SyncState.SeqNo build() {
net.named_data.jndn.sync.SyncStateProto.SyncState.SeqNo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public net.named_data.jndn.sync.SyncStateProto.SyncState.SeqNo buildPartial() {
net.named_data.jndn.sync.SyncStateProto.SyncState.SeqNo result = new net.named_data.jndn.sync.SyncStateProto.SyncState.SeqNo(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.seq_ = seq_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.session_ = session_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof net.named_data.jndn.sync.SyncStateProto.SyncState.SeqNo) {
return mergeFrom((net.named_data.jndn.sync.SyncStateProto.SyncState.SeqNo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(net.named_data.jndn.sync.SyncStateProto.SyncState.SeqNo other) {
if (other == net.named_data.jndn.sync.SyncStateProto.SyncState.SeqNo.getDefaultInstance()) return this;
if (other.hasSeq()) {
setSeq(other.getSeq());
}
if (other.hasSession()) {
setSession(other.getSession());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasSeq()) {
return false;
}
if (!hasSession()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
net.named_data.jndn.sync.SyncStateProto.SyncState.SeqNo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (net.named_data.jndn.sync.SyncStateProto.SyncState.SeqNo) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private long seq_ ;
/**
* required uint64 seq = 1;
*/
public boolean hasSeq() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required uint64 seq = 1;
*/
public long getSeq() {
return seq_;
}
/**
* required uint64 seq = 1;
*/
public Builder setSeq(long value) {
bitField0_ |= 0x00000001;
seq_ = value;
onChanged();
return this;
}
/**
* required uint64 seq = 1;
*/
public Builder clearSeq() {
bitField0_ = (bitField0_ & ~0x00000001);
seq_ = 0L;
onChanged();
return this;
}
private long session_ ;
/**
* required uint64 session = 2;
*/
public boolean hasSession() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required uint64 session = 2;
*/
public long getSession() {
return session_;
}
/**
* required uint64 session = 2;
*/
public Builder setSession(long value) {
bitField0_ |= 0x00000002;
session_ = value;
onChanged();
return this;
}
/**
* required uint64 session = 2;
*/
public Builder clearSession() {
bitField0_ = (bitField0_ & ~0x00000002);
session_ = 0L;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:net.named_data.jndn.sync.SyncState.SeqNo)
}
static {
defaultInstance = new SeqNo(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:net.named_data.jndn.sync.SyncState.SeqNo)
}
private int bitField0_;
public static final int NAME_FIELD_NUMBER = 1;
private java.lang.Object name_;
/**
* required string name = 1;
*/
public boolean hasName() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string name = 1;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
}
}
/**
* required string name = 1;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TYPE_FIELD_NUMBER = 2;
private net.named_data.jndn.sync.SyncStateProto.SyncState.ActionType type_;
/**
* required .net.named_data.jndn.sync.SyncState.ActionType type = 2;
*/
public boolean hasType() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required .net.named_data.jndn.sync.SyncState.ActionType type = 2;
*/
public net.named_data.jndn.sync.SyncStateProto.SyncState.ActionType getType() {
return type_;
}
public static final int SEQNO_FIELD_NUMBER = 3;
private net.named_data.jndn.sync.SyncStateProto.SyncState.SeqNo seqno_;
/**
* optional .net.named_data.jndn.sync.SyncState.SeqNo seqno = 3;
*/
public boolean hasSeqno() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional .net.named_data.jndn.sync.SyncState.SeqNo seqno = 3;
*/
public net.named_data.jndn.sync.SyncStateProto.SyncState.SeqNo getSeqno() {
return seqno_;
}
/**
* optional .net.named_data.jndn.sync.SyncState.SeqNo seqno = 3;
*/
public net.named_data.jndn.sync.SyncStateProto.SyncState.SeqNoOrBuilder getSeqnoOrBuilder() {
return seqno_;
}
public static final int APPLICATION_INFO_FIELD_NUMBER = 4;
private com.google.protobuf.ByteString applicationInfo_;
/**
* optional bytes application_info = 4;
*/
public boolean hasApplicationInfo() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional bytes application_info = 4;
*/
public com.google.protobuf.ByteString getApplicationInfo() {
return applicationInfo_;
}
private void initFields() {
name_ = "";
type_ = net.named_data.jndn.sync.SyncStateProto.SyncState.ActionType.UPDATE;
seqno_ = net.named_data.jndn.sync.SyncStateProto.SyncState.SeqNo.getDefaultInstance();
applicationInfo_ = com.google.protobuf.ByteString.EMPTY;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasName()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasType()) {
memoizedIsInitialized = 0;
return false;
}
if (hasSeqno()) {
if (!getSeqno().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, getNameBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeEnum(2, type_.getNumber());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeMessage(3, seqno_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeBytes(4, applicationInfo_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, getNameBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, type_.getNumber());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, seqno_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, applicationInfo_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static net.named_data.jndn.sync.SyncStateProto.SyncState parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static net.named_data.jndn.sync.SyncStateProto.SyncState parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static net.named_data.jndn.sync.SyncStateProto.SyncState parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static net.named_data.jndn.sync.SyncStateProto.SyncState parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static net.named_data.jndn.sync.SyncStateProto.SyncState parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static net.named_data.jndn.sync.SyncStateProto.SyncState parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static net.named_data.jndn.sync.SyncStateProto.SyncState parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static net.named_data.jndn.sync.SyncStateProto.SyncState parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static net.named_data.jndn.sync.SyncStateProto.SyncState parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static net.named_data.jndn.sync.SyncStateProto.SyncState parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(net.named_data.jndn.sync.SyncStateProto.SyncState prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code net.named_data.jndn.sync.SyncState}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:net.named_data.jndn.sync.SyncState)
net.named_data.jndn.sync.SyncStateProto.SyncStateOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return net.named_data.jndn.sync.SyncStateProto.internal_static_net_named_data_jndn_sync_SyncState_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return net.named_data.jndn.sync.SyncStateProto.internal_static_net_named_data_jndn_sync_SyncState_fieldAccessorTable
.ensureFieldAccessorsInitialized(
net.named_data.jndn.sync.SyncStateProto.SyncState.class, net.named_data.jndn.sync.SyncStateProto.SyncState.Builder.class);
}
// Construct using net.named_data.jndn.sync.SyncStateProto.SyncState.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getSeqnoFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
name_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
type_ = net.named_data.jndn.sync.SyncStateProto.SyncState.ActionType.UPDATE;
bitField0_ = (bitField0_ & ~0x00000002);
if (seqnoBuilder_ == null) {
seqno_ = net.named_data.jndn.sync.SyncStateProto.SyncState.SeqNo.getDefaultInstance();
} else {
seqnoBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
applicationInfo_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000008);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return net.named_data.jndn.sync.SyncStateProto.internal_static_net_named_data_jndn_sync_SyncState_descriptor;
}
public net.named_data.jndn.sync.SyncStateProto.SyncState getDefaultInstanceForType() {
return net.named_data.jndn.sync.SyncStateProto.SyncState.getDefaultInstance();
}
public net.named_data.jndn.sync.SyncStateProto.SyncState build() {
net.named_data.jndn.sync.SyncStateProto.SyncState result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public net.named_data.jndn.sync.SyncStateProto.SyncState buildPartial() {
net.named_data.jndn.sync.SyncStateProto.SyncState result = new net.named_data.jndn.sync.SyncStateProto.SyncState(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.name_ = name_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.type_ = type_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
if (seqnoBuilder_ == null) {
result.seqno_ = seqno_;
} else {
result.seqno_ = seqnoBuilder_.build();
}
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.applicationInfo_ = applicationInfo_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof net.named_data.jndn.sync.SyncStateProto.SyncState) {
return mergeFrom((net.named_data.jndn.sync.SyncStateProto.SyncState)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(net.named_data.jndn.sync.SyncStateProto.SyncState other) {
if (other == net.named_data.jndn.sync.SyncStateProto.SyncState.getDefaultInstance()) return this;
if (other.hasName()) {
bitField0_ |= 0x00000001;
name_ = other.name_;
onChanged();
}
if (other.hasType()) {
setType(other.getType());
}
if (other.hasSeqno()) {
mergeSeqno(other.getSeqno());
}
if (other.hasApplicationInfo()) {
setApplicationInfo(other.getApplicationInfo());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasName()) {
return false;
}
if (!hasType()) {
return false;
}
if (hasSeqno()) {
if (!getSeqno().isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
net.named_data.jndn.sync.SyncStateProto.SyncState parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (net.named_data.jndn.sync.SyncStateProto.SyncState) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object name_ = "";
/**
* required string name = 1;
*/
public boolean hasName() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string name = 1;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string name = 1;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string name = 1;
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
name_ = value;
onChanged();
return this;
}
/**
* required string name = 1;
*/
public Builder clearName() {
bitField0_ = (bitField0_ & ~0x00000001);
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
* required string name = 1;
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
name_ = value;
onChanged();
return this;
}
private net.named_data.jndn.sync.SyncStateProto.SyncState.ActionType type_ = net.named_data.jndn.sync.SyncStateProto.SyncState.ActionType.UPDATE;
/**
* required .net.named_data.jndn.sync.SyncState.ActionType type = 2;
*/
public boolean hasType() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required .net.named_data.jndn.sync.SyncState.ActionType type = 2;
*/
public net.named_data.jndn.sync.SyncStateProto.SyncState.ActionType getType() {
return type_;
}
/**
* required .net.named_data.jndn.sync.SyncState.ActionType type = 2;
*/
public Builder setType(net.named_data.jndn.sync.SyncStateProto.SyncState.ActionType value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
type_ = value;
onChanged();
return this;
}
/**
* required .net.named_data.jndn.sync.SyncState.ActionType type = 2;
*/
public Builder clearType() {
bitField0_ = (bitField0_ & ~0x00000002);
type_ = net.named_data.jndn.sync.SyncStateProto.SyncState.ActionType.UPDATE;
onChanged();
return this;
}
private net.named_data.jndn.sync.SyncStateProto.SyncState.SeqNo seqno_ = net.named_data.jndn.sync.SyncStateProto.SyncState.SeqNo.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
net.named_data.jndn.sync.SyncStateProto.SyncState.SeqNo, net.named_data.jndn.sync.SyncStateProto.SyncState.SeqNo.Builder, net.named_data.jndn.sync.SyncStateProto.SyncState.SeqNoOrBuilder> seqnoBuilder_;
/**
* optional .net.named_data.jndn.sync.SyncState.SeqNo seqno = 3;
*/
public boolean hasSeqno() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional .net.named_data.jndn.sync.SyncState.SeqNo seqno = 3;
*/
public net.named_data.jndn.sync.SyncStateProto.SyncState.SeqNo getSeqno() {
if (seqnoBuilder_ == null) {
return seqno_;
} else {
return seqnoBuilder_.getMessage();
}
}
/**
* optional .net.named_data.jndn.sync.SyncState.SeqNo seqno = 3;
*/
public Builder setSeqno(net.named_data.jndn.sync.SyncStateProto.SyncState.SeqNo value) {
if (seqnoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
seqno_ = value;
onChanged();
} else {
seqnoBuilder_.setMessage(value);
}
bitField0_ |= 0x00000004;
return this;
}
/**
* optional .net.named_data.jndn.sync.SyncState.SeqNo seqno = 3;
*/
public Builder setSeqno(
net.named_data.jndn.sync.SyncStateProto.SyncState.SeqNo.Builder builderForValue) {
if (seqnoBuilder_ == null) {
seqno_ = builderForValue.build();
onChanged();
} else {
seqnoBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000004;
return this;
}
/**
* optional .net.named_data.jndn.sync.SyncState.SeqNo seqno = 3;
*/
public Builder mergeSeqno(net.named_data.jndn.sync.SyncStateProto.SyncState.SeqNo value) {
if (seqnoBuilder_ == null) {
if (((bitField0_ & 0x00000004) == 0x00000004) &&
seqno_ != net.named_data.jndn.sync.SyncStateProto.SyncState.SeqNo.getDefaultInstance()) {
seqno_ =
net.named_data.jndn.sync.SyncStateProto.SyncState.SeqNo.newBuilder(seqno_).mergeFrom(value).buildPartial();
} else {
seqno_ = value;
}
onChanged();
} else {
seqnoBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000004;
return this;
}
/**
* optional .net.named_data.jndn.sync.SyncState.SeqNo seqno = 3;
*/
public Builder clearSeqno() {
if (seqnoBuilder_ == null) {
seqno_ = net.named_data.jndn.sync.SyncStateProto.SyncState.SeqNo.getDefaultInstance();
onChanged();
} else {
seqnoBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
/**
* optional .net.named_data.jndn.sync.SyncState.SeqNo seqno = 3;
*/
public net.named_data.jndn.sync.SyncStateProto.SyncState.SeqNo.Builder getSeqnoBuilder() {
bitField0_ |= 0x00000004;
onChanged();
return getSeqnoFieldBuilder().getBuilder();
}
/**
* optional .net.named_data.jndn.sync.SyncState.SeqNo seqno = 3;
*/
public net.named_data.jndn.sync.SyncStateProto.SyncState.SeqNoOrBuilder getSeqnoOrBuilder() {
if (seqnoBuilder_ != null) {
return seqnoBuilder_.getMessageOrBuilder();
} else {
return seqno_;
}
}
/**
* optional .net.named_data.jndn.sync.SyncState.SeqNo seqno = 3;
*/
private com.google.protobuf.SingleFieldBuilder<
net.named_data.jndn.sync.SyncStateProto.SyncState.SeqNo, net.named_data.jndn.sync.SyncStateProto.SyncState.SeqNo.Builder, net.named_data.jndn.sync.SyncStateProto.SyncState.SeqNoOrBuilder>
getSeqnoFieldBuilder() {
if (seqnoBuilder_ == null) {
seqnoBuilder_ = new com.google.protobuf.SingleFieldBuilder<
net.named_data.jndn.sync.SyncStateProto.SyncState.SeqNo, net.named_data.jndn.sync.SyncStateProto.SyncState.SeqNo.Builder, net.named_data.jndn.sync.SyncStateProto.SyncState.SeqNoOrBuilder>(
getSeqno(),
getParentForChildren(),
isClean());
seqno_ = null;
}
return seqnoBuilder_;
}
private com.google.protobuf.ByteString applicationInfo_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes application_info = 4;
*/
public boolean hasApplicationInfo() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional bytes application_info = 4;
*/
public com.google.protobuf.ByteString getApplicationInfo() {
return applicationInfo_;
}
/**
* optional bytes application_info = 4;
*/
public Builder setApplicationInfo(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
applicationInfo_ = value;
onChanged();
return this;
}
/**
* optional bytes application_info = 4;
*/
public Builder clearApplicationInfo() {
bitField0_ = (bitField0_ & ~0x00000008);
applicationInfo_ = getDefaultInstance().getApplicationInfo();
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:net.named_data.jndn.sync.SyncState)
}
static {
defaultInstance = new SyncState(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:net.named_data.jndn.sync.SyncState)
}
public interface SyncStateMsgOrBuilder extends
// @@protoc_insertion_point(interface_extends:net.named_data.jndn.sync.SyncStateMsg)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .net.named_data.jndn.sync.SyncState ss = 1;
*/
java.util.List
getSsList();
/**
* repeated .net.named_data.jndn.sync.SyncState ss = 1;
*/
net.named_data.jndn.sync.SyncStateProto.SyncState getSs(int index);
/**
* repeated .net.named_data.jndn.sync.SyncState ss = 1;
*/
int getSsCount();
/**
* repeated .net.named_data.jndn.sync.SyncState ss = 1;
*/
java.util.List extends net.named_data.jndn.sync.SyncStateProto.SyncStateOrBuilder>
getSsOrBuilderList();
/**
* repeated .net.named_data.jndn.sync.SyncState ss = 1;
*/
net.named_data.jndn.sync.SyncStateProto.SyncStateOrBuilder getSsOrBuilder(
int index);
}
/**
* Protobuf type {@code net.named_data.jndn.sync.SyncStateMsg}
*/
public static final class SyncStateMsg extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:net.named_data.jndn.sync.SyncStateMsg)
SyncStateMsgOrBuilder {
// Use SyncStateMsg.newBuilder() to construct.
private SyncStateMsg(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private SyncStateMsg(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final SyncStateMsg defaultInstance;
public static SyncStateMsg getDefaultInstance() {
return defaultInstance;
}
public SyncStateMsg getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private SyncStateMsg(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
ss_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
ss_.add(input.readMessage(net.named_data.jndn.sync.SyncStateProto.SyncState.PARSER, extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
ss_ = java.util.Collections.unmodifiableList(ss_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return net.named_data.jndn.sync.SyncStateProto.internal_static_net_named_data_jndn_sync_SyncStateMsg_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return net.named_data.jndn.sync.SyncStateProto.internal_static_net_named_data_jndn_sync_SyncStateMsg_fieldAccessorTable
.ensureFieldAccessorsInitialized(
net.named_data.jndn.sync.SyncStateProto.SyncStateMsg.class, net.named_data.jndn.sync.SyncStateProto.SyncStateMsg.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public SyncStateMsg parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new SyncStateMsg(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public static final int SS_FIELD_NUMBER = 1;
private java.util.List ss_;
/**
* repeated .net.named_data.jndn.sync.SyncState ss = 1;
*/
public java.util.List getSsList() {
return ss_;
}
/**
* repeated .net.named_data.jndn.sync.SyncState ss = 1;
*/
public java.util.List extends net.named_data.jndn.sync.SyncStateProto.SyncStateOrBuilder>
getSsOrBuilderList() {
return ss_;
}
/**
* repeated .net.named_data.jndn.sync.SyncState ss = 1;
*/
public int getSsCount() {
return ss_.size();
}
/**
* repeated .net.named_data.jndn.sync.SyncState ss = 1;
*/
public net.named_data.jndn.sync.SyncStateProto.SyncState getSs(int index) {
return ss_.get(index);
}
/**
* repeated .net.named_data.jndn.sync.SyncState ss = 1;
*/
public net.named_data.jndn.sync.SyncStateProto.SyncStateOrBuilder getSsOrBuilder(
int index) {
return ss_.get(index);
}
private void initFields() {
ss_ = java.util.Collections.emptyList();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
for (int i = 0; i < getSsCount(); i++) {
if (!getSs(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
for (int i = 0; i < ss_.size(); i++) {
output.writeMessage(1, ss_.get(i));
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < ss_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, ss_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static net.named_data.jndn.sync.SyncStateProto.SyncStateMsg parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static net.named_data.jndn.sync.SyncStateProto.SyncStateMsg parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static net.named_data.jndn.sync.SyncStateProto.SyncStateMsg parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static net.named_data.jndn.sync.SyncStateProto.SyncStateMsg parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static net.named_data.jndn.sync.SyncStateProto.SyncStateMsg parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static net.named_data.jndn.sync.SyncStateProto.SyncStateMsg parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static net.named_data.jndn.sync.SyncStateProto.SyncStateMsg parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static net.named_data.jndn.sync.SyncStateProto.SyncStateMsg parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static net.named_data.jndn.sync.SyncStateProto.SyncStateMsg parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static net.named_data.jndn.sync.SyncStateProto.SyncStateMsg parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(net.named_data.jndn.sync.SyncStateProto.SyncStateMsg prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code net.named_data.jndn.sync.SyncStateMsg}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:net.named_data.jndn.sync.SyncStateMsg)
net.named_data.jndn.sync.SyncStateProto.SyncStateMsgOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return net.named_data.jndn.sync.SyncStateProto.internal_static_net_named_data_jndn_sync_SyncStateMsg_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return net.named_data.jndn.sync.SyncStateProto.internal_static_net_named_data_jndn_sync_SyncStateMsg_fieldAccessorTable
.ensureFieldAccessorsInitialized(
net.named_data.jndn.sync.SyncStateProto.SyncStateMsg.class, net.named_data.jndn.sync.SyncStateProto.SyncStateMsg.Builder.class);
}
// Construct using net.named_data.jndn.sync.SyncStateProto.SyncStateMsg.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getSsFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
if (ssBuilder_ == null) {
ss_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ssBuilder_.clear();
}
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return net.named_data.jndn.sync.SyncStateProto.internal_static_net_named_data_jndn_sync_SyncStateMsg_descriptor;
}
public net.named_data.jndn.sync.SyncStateProto.SyncStateMsg getDefaultInstanceForType() {
return net.named_data.jndn.sync.SyncStateProto.SyncStateMsg.getDefaultInstance();
}
public net.named_data.jndn.sync.SyncStateProto.SyncStateMsg build() {
net.named_data.jndn.sync.SyncStateProto.SyncStateMsg result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public net.named_data.jndn.sync.SyncStateProto.SyncStateMsg buildPartial() {
net.named_data.jndn.sync.SyncStateProto.SyncStateMsg result = new net.named_data.jndn.sync.SyncStateProto.SyncStateMsg(this);
int from_bitField0_ = bitField0_;
if (ssBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
ss_ = java.util.Collections.unmodifiableList(ss_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.ss_ = ss_;
} else {
result.ss_ = ssBuilder_.build();
}
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof net.named_data.jndn.sync.SyncStateProto.SyncStateMsg) {
return mergeFrom((net.named_data.jndn.sync.SyncStateProto.SyncStateMsg)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(net.named_data.jndn.sync.SyncStateProto.SyncStateMsg other) {
if (other == net.named_data.jndn.sync.SyncStateProto.SyncStateMsg.getDefaultInstance()) return this;
if (ssBuilder_ == null) {
if (!other.ss_.isEmpty()) {
if (ss_.isEmpty()) {
ss_ = other.ss_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureSsIsMutable();
ss_.addAll(other.ss_);
}
onChanged();
}
} else {
if (!other.ss_.isEmpty()) {
if (ssBuilder_.isEmpty()) {
ssBuilder_.dispose();
ssBuilder_ = null;
ss_ = other.ss_;
bitField0_ = (bitField0_ & ~0x00000001);
ssBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getSsFieldBuilder() : null;
} else {
ssBuilder_.addAllMessages(other.ss_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
for (int i = 0; i < getSsCount(); i++) {
if (!getSs(i).isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
net.named_data.jndn.sync.SyncStateProto.SyncStateMsg parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (net.named_data.jndn.sync.SyncStateProto.SyncStateMsg) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List ss_ =
java.util.Collections.emptyList();
private void ensureSsIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
ss_ = new java.util.ArrayList(ss_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
net.named_data.jndn.sync.SyncStateProto.SyncState, net.named_data.jndn.sync.SyncStateProto.SyncState.Builder, net.named_data.jndn.sync.SyncStateProto.SyncStateOrBuilder> ssBuilder_;
/**
* repeated .net.named_data.jndn.sync.SyncState ss = 1;
*/
public java.util.List getSsList() {
if (ssBuilder_ == null) {
return java.util.Collections.unmodifiableList(ss_);
} else {
return ssBuilder_.getMessageList();
}
}
/**
* repeated .net.named_data.jndn.sync.SyncState ss = 1;
*/
public int getSsCount() {
if (ssBuilder_ == null) {
return ss_.size();
} else {
return ssBuilder_.getCount();
}
}
/**
* repeated .net.named_data.jndn.sync.SyncState ss = 1;
*/
public net.named_data.jndn.sync.SyncStateProto.SyncState getSs(int index) {
if (ssBuilder_ == null) {
return ss_.get(index);
} else {
return ssBuilder_.getMessage(index);
}
}
/**
* repeated .net.named_data.jndn.sync.SyncState ss = 1;
*/
public Builder setSs(
int index, net.named_data.jndn.sync.SyncStateProto.SyncState value) {
if (ssBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureSsIsMutable();
ss_.set(index, value);
onChanged();
} else {
ssBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .net.named_data.jndn.sync.SyncState ss = 1;
*/
public Builder setSs(
int index, net.named_data.jndn.sync.SyncStateProto.SyncState.Builder builderForValue) {
if (ssBuilder_ == null) {
ensureSsIsMutable();
ss_.set(index, builderForValue.build());
onChanged();
} else {
ssBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .net.named_data.jndn.sync.SyncState ss = 1;
*/
public Builder addSs(net.named_data.jndn.sync.SyncStateProto.SyncState value) {
if (ssBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureSsIsMutable();
ss_.add(value);
onChanged();
} else {
ssBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .net.named_data.jndn.sync.SyncState ss = 1;
*/
public Builder addSs(
int index, net.named_data.jndn.sync.SyncStateProto.SyncState value) {
if (ssBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureSsIsMutable();
ss_.add(index, value);
onChanged();
} else {
ssBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .net.named_data.jndn.sync.SyncState ss = 1;
*/
public Builder addSs(
net.named_data.jndn.sync.SyncStateProto.SyncState.Builder builderForValue) {
if (ssBuilder_ == null) {
ensureSsIsMutable();
ss_.add(builderForValue.build());
onChanged();
} else {
ssBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .net.named_data.jndn.sync.SyncState ss = 1;
*/
public Builder addSs(
int index, net.named_data.jndn.sync.SyncStateProto.SyncState.Builder builderForValue) {
if (ssBuilder_ == null) {
ensureSsIsMutable();
ss_.add(index, builderForValue.build());
onChanged();
} else {
ssBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .net.named_data.jndn.sync.SyncState ss = 1;
*/
public Builder addAllSs(
java.lang.Iterable extends net.named_data.jndn.sync.SyncStateProto.SyncState> values) {
if (ssBuilder_ == null) {
ensureSsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, ss_);
onChanged();
} else {
ssBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .net.named_data.jndn.sync.SyncState ss = 1;
*/
public Builder clearSs() {
if (ssBuilder_ == null) {
ss_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
ssBuilder_.clear();
}
return this;
}
/**
* repeated .net.named_data.jndn.sync.SyncState ss = 1;
*/
public Builder removeSs(int index) {
if (ssBuilder_ == null) {
ensureSsIsMutable();
ss_.remove(index);
onChanged();
} else {
ssBuilder_.remove(index);
}
return this;
}
/**
* repeated .net.named_data.jndn.sync.SyncState ss = 1;
*/
public net.named_data.jndn.sync.SyncStateProto.SyncState.Builder getSsBuilder(
int index) {
return getSsFieldBuilder().getBuilder(index);
}
/**
* repeated .net.named_data.jndn.sync.SyncState ss = 1;
*/
public net.named_data.jndn.sync.SyncStateProto.SyncStateOrBuilder getSsOrBuilder(
int index) {
if (ssBuilder_ == null) {
return ss_.get(index); } else {
return ssBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .net.named_data.jndn.sync.SyncState ss = 1;
*/
public java.util.List extends net.named_data.jndn.sync.SyncStateProto.SyncStateOrBuilder>
getSsOrBuilderList() {
if (ssBuilder_ != null) {
return ssBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(ss_);
}
}
/**
* repeated .net.named_data.jndn.sync.SyncState ss = 1;
*/
public net.named_data.jndn.sync.SyncStateProto.SyncState.Builder addSsBuilder() {
return getSsFieldBuilder().addBuilder(
net.named_data.jndn.sync.SyncStateProto.SyncState.getDefaultInstance());
}
/**
* repeated .net.named_data.jndn.sync.SyncState ss = 1;
*/
public net.named_data.jndn.sync.SyncStateProto.SyncState.Builder addSsBuilder(
int index) {
return getSsFieldBuilder().addBuilder(
index, net.named_data.jndn.sync.SyncStateProto.SyncState.getDefaultInstance());
}
/**
* repeated .net.named_data.jndn.sync.SyncState ss = 1;
*/
public java.util.List
getSsBuilderList() {
return getSsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
net.named_data.jndn.sync.SyncStateProto.SyncState, net.named_data.jndn.sync.SyncStateProto.SyncState.Builder, net.named_data.jndn.sync.SyncStateProto.SyncStateOrBuilder>
getSsFieldBuilder() {
if (ssBuilder_ == null) {
ssBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
net.named_data.jndn.sync.SyncStateProto.SyncState, net.named_data.jndn.sync.SyncStateProto.SyncState.Builder, net.named_data.jndn.sync.SyncStateProto.SyncStateOrBuilder>(
ss_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
ss_ = null;
}
return ssBuilder_;
}
// @@protoc_insertion_point(builder_scope:net.named_data.jndn.sync.SyncStateMsg)
}
static {
defaultInstance = new SyncStateMsg(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:net.named_data.jndn.sync.SyncStateMsg)
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_net_named_data_jndn_sync_SyncState_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_net_named_data_jndn_sync_SyncState_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_net_named_data_jndn_sync_SyncState_SeqNo_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_net_named_data_jndn_sync_SyncState_SeqNo_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_net_named_data_jndn_sync_SyncStateMsg_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_net_named_data_jndn_sync_SyncStateMsg_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\026sync-state-proto.proto\022\030net.named_data" +
".jndn.sync\"\203\002\n\tSyncState\022\014\n\004name\030\001 \002(\t\022<" +
"\n\004type\030\002 \002(\0162..net.named_data.jndn.sync." +
"SyncState.ActionType\0228\n\005seqno\030\003 \001(\0132).ne" +
"t.named_data.jndn.sync.SyncState.SeqNo\022\030" +
"\n\020application_info\030\004 \001(\014\032%\n\005SeqNo\022\013\n\003seq" +
"\030\001 \002(\004\022\017\n\007session\030\002 \002(\004\"/\n\nActionType\022\n\n" +
"\006UPDATE\020\000\022\n\n\006DELETE\020\001\022\t\n\005OTHER\020\002\"?\n\014Sync" +
"StateMsg\022/\n\002ss\030\001 \003(\0132#.net.named_data.jn" +
"dn.sync.SyncState"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor. InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
}, assigner);
internal_static_net_named_data_jndn_sync_SyncState_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_net_named_data_jndn_sync_SyncState_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_net_named_data_jndn_sync_SyncState_descriptor,
new java.lang.String[] { "Name", "Type", "Seqno", "ApplicationInfo", });
internal_static_net_named_data_jndn_sync_SyncState_SeqNo_descriptor =
internal_static_net_named_data_jndn_sync_SyncState_descriptor.getNestedTypes().get(0);
internal_static_net_named_data_jndn_sync_SyncState_SeqNo_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_net_named_data_jndn_sync_SyncState_SeqNo_descriptor,
new java.lang.String[] { "Seq", "Session", });
internal_static_net_named_data_jndn_sync_SyncStateMsg_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_net_named_data_jndn_sync_SyncStateMsg_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_net_named_data_jndn_sync_SyncStateMsg_descriptor,
new java.lang.String[] { "Ss", });
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy