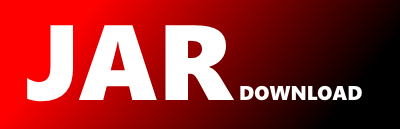
net.nan21.dnet.module.md.activity.business.serviceimpl.CalendarEventService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of nan21.dnet.module.md.business Show documentation
Show all versions of nan21.dnet.module.md.business Show documentation
MD module business layer implementation.
The newest version!
/*
* DNet eBusiness Suite
* Copyright: 2010-2013 Nan21 Electronics SRL. All rights reserved.
* Use is subject to license terms.
*/
package net.nan21.dnet.module.md.activity.business.serviceimpl;
import java.util.List;
import javax.persistence.EntityManager;
import net.nan21.dnet.core.api.session.Session;
import net.nan21.dnet.core.business.service.entity.AbstractEntityService;
import net.nan21.dnet.module.md.activity.business.service.ICalendarEventService;
import net.nan21.dnet.module.md.activity.domain.entity.CalendarEvent;
import net.nan21.dnet.module.md.activity.domain.entity.CalendarEventPriority;
import net.nan21.dnet.module.md.activity.domain.entity.CalendarEventStatus;
import net.nan21.dnet.module.md.bp.domain.entity.BusinessPartner;
import net.nan21.dnet.module.md.bp.domain.entity.Contact;
/**
* Repository functionality for {@link CalendarEvent} domain entity. It contains
* finder methods based on unique keys as well as reference fields.
*
*/
public class CalendarEventService extends AbstractEntityService
implements
ICalendarEventService {
public CalendarEventService() {
super();
}
public CalendarEventService(EntityManager em) {
super();
this.setEntityManager(em);
}
@Override
public Class getEntityClass() {
return CalendarEvent.class;
}
/**
* Find by reference: status
*/
public List findByStatus(CalendarEventStatus status) {
return this.findByStatusId(status.getId());
}
/**
* Find by ID of reference: status.id
*/
public List findByStatusId(Long statusId) {
return (List) this
.getEntityManager()
.createQuery(
"select e from CalendarEvent e where e.clientId = :pClientId and e.status.id = :pStatusId",
CalendarEvent.class)
.setParameter("pClientId", Session.user.get().getClientId())
.setParameter("pStatusId", statusId).getResultList();
}
/**
* Find by reference: priority
*/
public List findByPriority(CalendarEventPriority priority) {
return this.findByPriorityId(priority.getId());
}
/**
* Find by ID of reference: priority.id
*/
public List findByPriorityId(Long priorityId) {
return (List) this
.getEntityManager()
.createQuery(
"select e from CalendarEvent e where e.clientId = :pClientId and e.priority.id = :pPriorityId",
CalendarEvent.class)
.setParameter("pClientId", Session.user.get().getClientId())
.setParameter("pPriorityId", priorityId).getResultList();
}
/**
* Find by reference: bpartner
*/
public List findByBpartner(BusinessPartner bpartner) {
return this.findByBpartnerId(bpartner.getId());
}
/**
* Find by ID of reference: bpartner.id
*/
public List findByBpartnerId(Long bpartnerId) {
return (List) this
.getEntityManager()
.createQuery(
"select e from CalendarEvent e where e.clientId = :pClientId and e.bpartner.id = :pBpartnerId",
CalendarEvent.class)
.setParameter("pClientId", Session.user.get().getClientId())
.setParameter("pBpartnerId", bpartnerId).getResultList();
}
/**
* Find by reference: contact
*/
public List findByContact(Contact contact) {
return this.findByContactId(contact.getId());
}
/**
* Find by ID of reference: contact.id
*/
public List findByContactId(Long contactId) {
return (List) this
.getEntityManager()
.createQuery(
"select e from CalendarEvent e where e.clientId = :pClientId and e.contact.id = :pContactId",
CalendarEvent.class)
.setParameter("pClientId", Session.user.get().getClientId())
.setParameter("pContactId", contactId).getResultList();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy