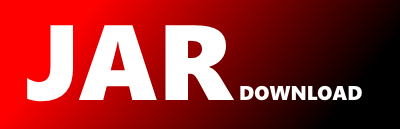
net.nemerosa.ontrack.json.JsonUtils Maven / Gradle / Ivy
package net.nemerosa.ontrack.json;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.node.JsonNodeFactory;
import com.fasterxml.jackson.databind.node.JsonNodeType;
import java.io.IOException;
import java.time.LocalDate;
import java.util.*;
public final class JsonUtils {
private static final JsonNodeFactory factory = JsonNodeFactory.instance;
private static final ObjectMapper mapper = ObjectMapperFactory.create();
private JsonUtils() {
}
public static V parse(JsonNode node, Class type) {
try {
return mapper.treeToValue(node, type);
} catch (JsonProcessingException e) {
throw new JsonParseException(e);
}
}
public static JsonNode format(Object value) {
return mapper.valueToTree(value);
}
public static String toJSONString(Object value) {
try {
return mapper.writeValueAsString(value);
} catch (JsonProcessingException e) {
throw new JsonParseException(e);
}
}
public static ObjectBuilder object() {
return new ObjectBuilder(factory);
}
public static ArrayBuilder array() {
return new ArrayBuilder(factory);
}
public static JsonNode stringArray(Iterable values) {
ArrayBuilder builder = array();
for (String value : values) {
builder.with(text(value));
}
return builder.end();
}
public static JsonNode intArray(int... values) {
ArrayBuilder builder = array();
for (int value : values) {
builder.with(number(value));
}
return builder.end();
}
public static JsonNode stringArray(String... values) {
return stringArray(Arrays.asList(values));
}
public static Map toMap(JsonNode node) throws IOException {
if (node.isObject()) {
Map map = new LinkedHashMap<>();
Iterator fieldNames = node.fieldNames();
while (fieldNames.hasNext()) {
String fieldName = fieldNames.next();
JsonNode fieldNode = node.get(fieldName);
map.put(fieldName, toObject(fieldNode));
}
return map;
} else {
throw new IllegalArgumentException("Can only transform a JSON object into a map");
}
}
public static Object toObject(JsonNode node) throws IOException {
JsonNodeType type = node.getNodeType();
switch (type) {
case ARRAY:
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy