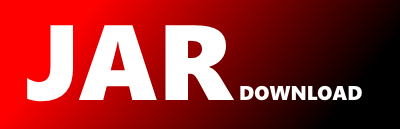
net.nemerosa.ontrack.json.KTJsonUtils.kt Maven / Gradle / Ivy
package net.nemerosa.ontrack.json
import com.fasterxml.jackson.databind.JsonNode
/**
* Parses a string as JSON
*/
fun String.parseAsJson(): JsonNode = JsonUtils.parseAsNode(this)
/**
* Map as JSON
*/
fun jsonOf(vararg pairs: Pair<*, *>) =
mapOf(*pairs).toJson()!!
/**
* Converts any object into JSON, or null if not defined.
*/
fun T?.toJson(): JsonNode? =
JsonUtils.format(this)
/**
* Non-null JSON transformation
*/
fun T.asJson(): JsonNode = JsonUtils.format(this)!!
/**
* Format as a string
*/
fun JsonNode.asJsonString(): String = JsonUtils.toJSONString(this)
/**
* Parses any node into an object.
*/
inline fun JsonNode.parse(): T =
JsonUtils.parse(this, T::class.java)
/**
* Formatting a JSON node as a string
*/
fun JsonNode.format() = JsonUtils.toJSONString(this)
/**
* Parses any node into an object or returns `null` if parsing fails
*/
inline fun JsonNode.parseOrNull(): T? =
try {
parse()
} catch (_: JsonParseException) {
null
}
/**
* Gets a field as enum
*/
inline fun > JsonNode.getEnum(field: String): E? {
val text = path(field).asText()
if (text.isNullOrBlank()) {
return null
} else {
return enumValueOf(text)
}
}
/**
* Gets a field as [Int].
*/
fun JsonNode.getInt(field: String): Int? =
if (has(field)) {
get(field).asInt()
} else {
null
}
/**
* Gets a required field as enum
*/
inline fun > JsonNode.getRequiredEnum(field: String): E =
getEnum(field) ?: throw JsonMissingFieldException(field)
/**
* Checks if a JSON node can be considered as null, either by being `null` itself
* or by being an instance of the [null node][JsonNode.isNull].
*/
fun JsonNode?.isNullOrNullNode() = this == null || this.isNull
© 2015 - 2025 Weber Informatics LLC | Privacy Policy