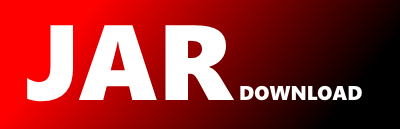
net.nemerosa.ontrack.kdsl.connector.graphql.schema.CreateWebhookMutation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ontrack-kdsl Show documentation
Show all versions of ontrack-kdsl Show documentation
Ontrack module: ontrack-kdsl
// AUTO-GENERATED FILE. DO NOT MODIFY.
//
// This class was automatically generated by Apollo GraphQL plugin from the GraphQL queries it found.
// It should not be modified by hand.
//
package net.nemerosa.ontrack.kdsl.connector.graphql.schema;
import com.apollographql.apollo.api.Mutation;
import com.apollographql.apollo.api.Operation;
import com.apollographql.apollo.api.OperationName;
import com.apollographql.apollo.api.Response;
import com.apollographql.apollo.api.ResponseField;
import com.apollographql.apollo.api.ScalarTypeAdapters;
import com.apollographql.apollo.api.internal.InputFieldMarshaller;
import com.apollographql.apollo.api.internal.InputFieldWriter;
import com.apollographql.apollo.api.internal.OperationRequestBodyComposer;
import com.apollographql.apollo.api.internal.QueryDocumentMinifier;
import com.apollographql.apollo.api.internal.ResponseFieldMapper;
import com.apollographql.apollo.api.internal.ResponseFieldMarshaller;
import com.apollographql.apollo.api.internal.ResponseReader;
import com.apollographql.apollo.api.internal.ResponseWriter;
import com.apollographql.apollo.api.internal.SimpleOperationResponseParser;
import com.apollographql.apollo.api.internal.UnmodifiableMapBuilder;
import com.apollographql.apollo.api.internal.Utils;
import com.fasterxml.jackson.databind.JsonNode;
import java.io.IOException;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.SuppressWarnings;
import java.util.Arrays;
import java.util.Collections;
import java.util.LinkedHashMap;
import java.util.Map;
import net.nemerosa.ontrack.kdsl.connector.graphql.schema.fragment.PayloadUserErrors;
import net.nemerosa.ontrack.kdsl.connector.graphql.schema.fragment.WebhookFragment;
import okio.Buffer;
import okio.BufferedSource;
import okio.ByteString;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
public final class CreateWebhookMutation implements Mutation {
public static final String OPERATION_ID = "e5dc2955bcd5f1a126e79e99ffd97777f65a2fd99690dfb4cbd0b487003a42b7";
public static final String QUERY_DOCUMENT = QueryDocumentMinifier.minify(
"mutation CreateWebhook($name: String!, $enabled: Boolean!, $url: String!, $timeoutSeconds: Long!, $authenticationType: String!, $authenticationConfig: JSON!) {\n"
+ " createWebhook(input: {name: $name, enabled: $enabled, url: $url, timeoutSeconds: $timeoutSeconds, authenticationType: $authenticationType, authenticationConfig: $authenticationConfig}) {\n"
+ " __typename\n"
+ " webhook {\n"
+ " __typename\n"
+ " ...WebhookFragment\n"
+ " }\n"
+ " ...PayloadUserErrors\n"
+ " }\n"
+ "}\n"
+ "fragment PayloadUserErrors on Payload {\n"
+ " __typename\n"
+ " errors {\n"
+ " __typename\n"
+ " message\n"
+ " exception\n"
+ " location\n"
+ " }\n"
+ "}\n"
+ "fragment WebhookFragment on Webhook {\n"
+ " __typename\n"
+ " name\n"
+ " enabled\n"
+ " url\n"
+ " timeoutSeconds\n"
+ " authenticationType\n"
+ "}"
);
public static final OperationName OPERATION_NAME = new OperationName() {
@Override
public String name() {
return "CreateWebhook";
}
};
private final CreateWebhookMutation.Variables variables;
public CreateWebhookMutation(@NotNull String name, boolean enabled, @NotNull String url,
@NotNull Object timeoutSeconds, @NotNull String authenticationType,
@NotNull JsonNode authenticationConfig) {
Utils.checkNotNull(name, "name == null");
Utils.checkNotNull(url, "url == null");
Utils.checkNotNull(timeoutSeconds, "timeoutSeconds == null");
Utils.checkNotNull(authenticationType, "authenticationType == null");
Utils.checkNotNull(authenticationConfig, "authenticationConfig == null");
variables = new CreateWebhookMutation.Variables(name, enabled, url, timeoutSeconds, authenticationType, authenticationConfig);
}
@Override
public String operationId() {
return OPERATION_ID;
}
@Override
public String queryDocument() {
return QUERY_DOCUMENT;
}
@Override
public CreateWebhookMutation.Data wrapData(CreateWebhookMutation.Data data) {
return data;
}
@Override
public CreateWebhookMutation.Variables variables() {
return variables;
}
@Override
public ResponseFieldMapper responseFieldMapper() {
return new Data.Mapper();
}
public static Builder builder() {
return new Builder();
}
@Override
public OperationName name() {
return OPERATION_NAME;
}
@Override
@NotNull
public Response parse(@NotNull final BufferedSource source,
@NotNull final ScalarTypeAdapters scalarTypeAdapters) throws IOException {
return SimpleOperationResponseParser.parse(source, this, scalarTypeAdapters);
}
@Override
@NotNull
public Response parse(@NotNull final ByteString byteString,
@NotNull final ScalarTypeAdapters scalarTypeAdapters) throws IOException {
return parse(new Buffer().write(byteString), scalarTypeAdapters);
}
@Override
@NotNull
public Response parse(@NotNull final BufferedSource source) throws
IOException {
return parse(source, ScalarTypeAdapters.DEFAULT);
}
@Override
@NotNull
public Response parse(@NotNull final ByteString byteString) throws
IOException {
return parse(byteString, ScalarTypeAdapters.DEFAULT);
}
@Override
@NotNull
public ByteString composeRequestBody(@NotNull final ScalarTypeAdapters scalarTypeAdapters) {
return OperationRequestBodyComposer.compose(this, false, true, scalarTypeAdapters);
}
@NotNull
@Override
public ByteString composeRequestBody() {
return OperationRequestBodyComposer.compose(this, false, true, ScalarTypeAdapters.DEFAULT);
}
@Override
@NotNull
public ByteString composeRequestBody(final boolean autoPersistQueries,
final boolean withQueryDocument, @NotNull final ScalarTypeAdapters scalarTypeAdapters) {
return OperationRequestBodyComposer.compose(this, autoPersistQueries, withQueryDocument, scalarTypeAdapters);
}
public static final class Builder {
private @NotNull String name;
private boolean enabled;
private @NotNull String url;
private @NotNull Object timeoutSeconds;
private @NotNull String authenticationType;
private @NotNull JsonNode authenticationConfig;
Builder() {
}
public Builder name(@NotNull String name) {
this.name = name;
return this;
}
public Builder enabled(boolean enabled) {
this.enabled = enabled;
return this;
}
public Builder url(@NotNull String url) {
this.url = url;
return this;
}
public Builder timeoutSeconds(@NotNull Object timeoutSeconds) {
this.timeoutSeconds = timeoutSeconds;
return this;
}
public Builder authenticationType(@NotNull String authenticationType) {
this.authenticationType = authenticationType;
return this;
}
public Builder authenticationConfig(@NotNull JsonNode authenticationConfig) {
this.authenticationConfig = authenticationConfig;
return this;
}
public CreateWebhookMutation build() {
Utils.checkNotNull(name, "name == null");
Utils.checkNotNull(url, "url == null");
Utils.checkNotNull(timeoutSeconds, "timeoutSeconds == null");
Utils.checkNotNull(authenticationType, "authenticationType == null");
Utils.checkNotNull(authenticationConfig, "authenticationConfig == null");
return new CreateWebhookMutation(name, enabled, url, timeoutSeconds, authenticationType, authenticationConfig);
}
}
public static final class Variables extends Operation.Variables {
private final @NotNull String name;
private final boolean enabled;
private final @NotNull String url;
private final @NotNull Object timeoutSeconds;
private final @NotNull String authenticationType;
private final @NotNull JsonNode authenticationConfig;
private final transient Map valueMap = new LinkedHashMap<>();
Variables(@NotNull String name, boolean enabled, @NotNull String url,
@NotNull Object timeoutSeconds, @NotNull String authenticationType,
@NotNull JsonNode authenticationConfig) {
this.name = name;
this.enabled = enabled;
this.url = url;
this.timeoutSeconds = timeoutSeconds;
this.authenticationType = authenticationType;
this.authenticationConfig = authenticationConfig;
this.valueMap.put("name", name);
this.valueMap.put("enabled", enabled);
this.valueMap.put("url", url);
this.valueMap.put("timeoutSeconds", timeoutSeconds);
this.valueMap.put("authenticationType", authenticationType);
this.valueMap.put("authenticationConfig", authenticationConfig);
}
public @NotNull String name() {
return name;
}
public boolean enabled() {
return enabled;
}
public @NotNull String url() {
return url;
}
public @NotNull Object timeoutSeconds() {
return timeoutSeconds;
}
public @NotNull String authenticationType() {
return authenticationType;
}
public @NotNull JsonNode authenticationConfig() {
return authenticationConfig;
}
@Override
public Map valueMap() {
return Collections.unmodifiableMap(valueMap);
}
@Override
public InputFieldMarshaller marshaller() {
return new InputFieldMarshaller() {
@Override
public void marshal(InputFieldWriter writer) throws IOException {
writer.writeString("name", name);
writer.writeBoolean("enabled", enabled);
writer.writeString("url", url);
writer.writeCustom("timeoutSeconds", net.nemerosa.ontrack.kdsl.connector.graphql.schema.type.CustomType.LONG, timeoutSeconds);
writer.writeString("authenticationType", authenticationType);
writer.writeCustom("authenticationConfig", net.nemerosa.ontrack.kdsl.connector.graphql.schema.type.CustomType.JSON, authenticationConfig);
}
};
}
}
/**
* Data from the response after executing this GraphQL operation
*/
public static class Data implements Operation.Data {
static final ResponseField[] $responseFields = {
ResponseField.forObject("createWebhook", "createWebhook", new UnmodifiableMapBuilder(1)
.put("input", new UnmodifiableMapBuilder(6)
.put("name", new UnmodifiableMapBuilder(2)
.put("kind", "Variable")
.put("variableName", "name")
.build())
.put("enabled", new UnmodifiableMapBuilder(2)
.put("kind", "Variable")
.put("variableName", "enabled")
.build())
.put("url", new UnmodifiableMapBuilder(2)
.put("kind", "Variable")
.put("variableName", "url")
.build())
.put("timeoutSeconds", new UnmodifiableMapBuilder(2)
.put("kind", "Variable")
.put("variableName", "timeoutSeconds")
.build())
.put("authenticationType", new UnmodifiableMapBuilder(2)
.put("kind", "Variable")
.put("variableName", "authenticationType")
.build())
.put("authenticationConfig", new UnmodifiableMapBuilder(2)
.put("kind", "Variable")
.put("variableName", "authenticationConfig")
.build())
.build())
.build(), true, Collections.emptyList())
};
final @Nullable CreateWebhook createWebhook;
private transient volatile String $toString;
private transient volatile int $hashCode;
private transient volatile boolean $hashCodeMemoized;
public Data(@Nullable CreateWebhook createWebhook) {
this.createWebhook = createWebhook;
}
/**
* Registers a webhook
*/
public @Nullable CreateWebhook createWebhook() {
return this.createWebhook;
}
@SuppressWarnings({"rawtypes", "unchecked"})
public ResponseFieldMarshaller marshaller() {
return new ResponseFieldMarshaller() {
@Override
public void marshal(ResponseWriter writer) {
writer.writeObject($responseFields[0], createWebhook != null ? createWebhook.marshaller() : null);
}
};
}
@Override
public String toString() {
if ($toString == null) {
$toString = "Data{"
+ "createWebhook=" + createWebhook
+ "}";
}
return $toString;
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof Data) {
Data that = (Data) o;
return ((this.createWebhook == null) ? (that.createWebhook == null) : this.createWebhook.equals(that.createWebhook));
}
return false;
}
@Override
public int hashCode() {
if (!$hashCodeMemoized) {
int h = 1;
h *= 1000003;
h ^= (createWebhook == null) ? 0 : createWebhook.hashCode();
$hashCode = h;
$hashCodeMemoized = true;
}
return $hashCode;
}
public static final class Mapper implements ResponseFieldMapper {
final CreateWebhook.Mapper createWebhookFieldMapper = new CreateWebhook.Mapper();
@Override
public Data map(ResponseReader reader) {
final CreateWebhook createWebhook = reader.readObject($responseFields[0], new ResponseReader.ObjectReader() {
@Override
public CreateWebhook read(ResponseReader reader) {
return createWebhookFieldMapper.map(reader);
}
});
return new Data(createWebhook);
}
}
}
/**
* Output type for the createWebhook mutation.
*/
public static class CreateWebhook {
static final ResponseField[] $responseFields = {
ResponseField.forString("__typename", "__typename", null, false, Collections.emptyList()),
ResponseField.forObject("webhook", "webhook", null, true, Collections.emptyList()),
ResponseField.forString("__typename", "__typename", null, false, Collections.emptyList())
};
final @NotNull String __typename;
final @Nullable Webhook webhook;
private final @NotNull Fragments fragments;
private transient volatile String $toString;
private transient volatile int $hashCode;
private transient volatile boolean $hashCodeMemoized;
public CreateWebhook(@NotNull String __typename, @Nullable Webhook webhook,
@NotNull Fragments fragments) {
this.__typename = Utils.checkNotNull(__typename, "__typename == null");
this.webhook = webhook;
this.fragments = Utils.checkNotNull(fragments, "fragments == null");
}
public @NotNull String __typename() {
return this.__typename;
}
/**
* Registered webhook
*/
public @Nullable Webhook webhook() {
return this.webhook;
}
public @NotNull Fragments fragments() {
return this.fragments;
}
@SuppressWarnings({"rawtypes", "unchecked"})
public ResponseFieldMarshaller marshaller() {
return new ResponseFieldMarshaller() {
@Override
public void marshal(ResponseWriter writer) {
writer.writeString($responseFields[0], __typename);
writer.writeObject($responseFields[1], webhook != null ? webhook.marshaller() : null);
fragments.marshaller().marshal(writer);
}
};
}
@Override
public String toString() {
if ($toString == null) {
$toString = "CreateWebhook{"
+ "__typename=" + __typename + ", "
+ "webhook=" + webhook + ", "
+ "fragments=" + fragments
+ "}";
}
return $toString;
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof CreateWebhook) {
CreateWebhook that = (CreateWebhook) o;
return this.__typename.equals(that.__typename)
&& ((this.webhook == null) ? (that.webhook == null) : this.webhook.equals(that.webhook))
&& this.fragments.equals(that.fragments);
}
return false;
}
@Override
public int hashCode() {
if (!$hashCodeMemoized) {
int h = 1;
h *= 1000003;
h ^= __typename.hashCode();
h *= 1000003;
h ^= (webhook == null) ? 0 : webhook.hashCode();
h *= 1000003;
h ^= fragments.hashCode();
$hashCode = h;
$hashCodeMemoized = true;
}
return $hashCode;
}
public static class Fragments {
final @Nullable PayloadUserErrors payloadUserErrors;
private transient volatile String $toString;
private transient volatile int $hashCode;
private transient volatile boolean $hashCodeMemoized;
public Fragments(@Nullable PayloadUserErrors payloadUserErrors) {
this.payloadUserErrors = payloadUserErrors;
}
public @Nullable PayloadUserErrors payloadUserErrors() {
return this.payloadUserErrors;
}
public ResponseFieldMarshaller marshaller() {
return new ResponseFieldMarshaller() {
@Override
public void marshal(ResponseWriter writer) {
final PayloadUserErrors $payloadUserErrors = payloadUserErrors;
if ($payloadUserErrors != null) {
writer.writeFragment($payloadUserErrors.marshaller());
}
}
};
}
@Override
public String toString() {
if ($toString == null) {
$toString = "Fragments{"
+ "payloadUserErrors=" + payloadUserErrors
+ "}";
}
return $toString;
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof Fragments) {
Fragments that = (Fragments) o;
return ((this.payloadUserErrors == null) ? (that.payloadUserErrors == null) : this.payloadUserErrors.equals(that.payloadUserErrors));
}
return false;
}
@Override
public int hashCode() {
if (!$hashCodeMemoized) {
int h = 1;
h *= 1000003;
h ^= (payloadUserErrors == null) ? 0 : payloadUserErrors.hashCode();
$hashCode = h;
$hashCodeMemoized = true;
}
return $hashCode;
}
public static final class Mapper implements ResponseFieldMapper {
static final ResponseField[] $responseFields = {
ResponseField.forFragment("__typename", "__typename", Arrays.asList(
ResponseField.Condition.typeCondition(new String[] {"CheckAutoVersioningPayload", "CreateBranchOrGetPayload", "CreateBranchPayload", "CreateBuildOrGetPayload", "CreateBuildPayload", "CreateGitHubConfigurationPayload", "CreateProjectOrGetPayload", "CreateProjectPayload", "CreatePromotionLevelByIdPayload", "CreatePromotionRunByIdPayload", "CreatePromotionRunPayload", "CreateValidationRunByIdPayload", "CreateValidationRunPayload", "CreateValidationStampByIdPayload", "CreateWebhookPayload", "DeleteBranchGitConfigPropertyByIdPayload", "DeleteBranchGitConfigPropertyPayload", "DeleteBranchMessagePropertyByIdPayload", "DeleteBranchMessagePropertyPayload", "DeleteBranchMetaInfoPropertyByIdPayload", "DeleteBranchMetaInfoPropertyPayload", "DeleteBuildGitCommitPropertyByIdPayload", "DeleteBuildGitCommitPropertyPayload", "DeleteBuildMessagePropertyByIdPayload", "DeleteBuildMessagePropertyPayload", "DeleteBuildMetaInfoPropertyByIdPayload", "DeleteBuildMetaInfoPropertyPayload", "DeleteBuildReleasePropertyByIdPayload", "DeleteBuildReleasePropertyPayload", "DeleteNotificationRecordsPayload", "DeleteProjectAutoPromotionLevelPropertyByIdPayload", "DeleteProjectAutoPromotionLevelPropertyPayload", "DeleteProjectAutoValidationStampPropertyByIdPayload", "DeleteProjectAutoValidationStampPropertyPayload", "DeleteProjectBitbucketCloudConfigurationPropertyByIdPayload", "DeleteProjectBitbucketCloudConfigurationPropertyPayload", "DeleteProjectBitbucketConfigurationPropertyByIdPayload", "DeleteProjectBitbucketConfigurationPropertyPayload", "DeleteProjectGitHubConfigurationPropertyByIdPayload", "DeleteProjectGitHubConfigurationPropertyPayload", "DeleteProjectGitLabConfigurationPropertyByIdPayload", "DeleteProjectGitLabConfigurationPropertyPayload", "DeleteProjectMessagePropertyByIdPayload", "DeleteProjectMessagePropertyPayload", "DeleteProjectMetaInfoPropertyByIdPayload", "DeleteProjectMetaInfoPropertyPayload", "DeleteProjectPayload", "DeleteProjectStalePropertyByIdPayload", "DeleteProjectStalePropertyPayload", "DeletePromotionLevelAutoPromotionPropertyByIdPayload", "DeletePromotionLevelAutoPromotionPropertyPayload", "DeletePromotionLevelMessagePropertyByIdPayload", "DeletePromotionLevelMessagePropertyPayload", "DeletePromotionLevelMetaInfoPropertyByIdPayload", "DeletePromotionLevelMetaInfoPropertyPayload", "DeletePromotionRunMessagePropertyByIdPayload", "DeletePromotionRunMessagePropertyPayload", "DeletePromotionRunMetaInfoPropertyByIdPayload", "DeletePromotionRunMetaInfoPropertyPayload", "DeleteSubscriptionPayload", "DeleteValidationRunMessagePropertyByIdPayload", "DeleteValidationRunMessagePropertyPayload", "DeleteValidationRunMetaInfoPropertyByIdPayload", "DeleteValidationRunMetaInfoPropertyPayload", "DeleteValidationStampMessagePropertyByIdPayload", "DeleteValidationStampMessagePropertyPayload", "DeleteValidationStampMetaInfoPropertyByIdPayload", "DeleteValidationStampMetaInfoPropertyPayload", "DeleteWebhookPayload", "DisableAccountPayload", "DisableProjectPayload", "DisableSubscriptionPayload", "EnableAccountPayload", "EnableProjectPayload", "EnableSubscriptionPayload", "FavouriteProjectPayload", "GitHubCheckAutoVersioningByBuildLabelPayload", "GitHubCheckAutoVersioningByBuildNamePayload", "GitHubCheckAutoVersioningByRunIdPayload", "GitHubIngestionBuildLinksByBuildLabelPayload", "GitHubIngestionBuildLinksByBuildNamePayload", "GitHubIngestionBuildLinksByRunIdPayload", "GitHubIngestionValidateDataByBuildLabelPayload", "GitHubIngestionValidateDataByBuildNamePayload", "GitHubIngestionValidateDataByRunIdPayload", "LinkBuildByIdPayload", "LinkBuildPayload", "LinksBuildPayload", "LockAccountPayload", "ReloadCascPayload", "SetAutoVersioningConfigByNamePayload", "SetAutoVersioningConfigPayload", "SetBranchGitConfigPropertyByIdPayload", "SetBranchGitConfigPropertyPayload", "SetBranchGitHubIngestionConfigPayload", "SetBranchMessagePropertyByIdPayload", "SetBranchMessagePropertyPayload", "SetBranchMetaInfoPropertyByIdPayload", "SetBranchMetaInfoPropertyPayload", "SetBranchPropertyByIdPayload", "SetBranchPropertyPayload", "SetBuildGitCommitPropertyByIdPayload", "SetBuildGitCommitPropertyPayload", "SetBuildMessagePropertyByIdPayload", "SetBuildMessagePropertyPayload", "SetBuildMetaInfoPropertyByIdPayload", "SetBuildMetaInfoPropertyPayload", "SetBuildPropertyByIdPayload", "SetBuildPropertyPayload", "SetBuildReleasePropertyByIdPayload", "SetBuildReleasePropertyPayload", "SetPreferencesPayload", "SetProjectAutoPromotionLevelPropertyByIdPayload", "SetProjectAutoPromotionLevelPropertyPayload", "SetProjectAutoValidationStampPropertyByIdPayload", "SetProjectAutoValidationStampPropertyPayload", "SetProjectBitbucketCloudConfigurationPropertyByIdPayload", "SetProjectBitbucketCloudConfigurationPropertyPayload", "SetProjectBitbucketConfigurationPropertyByIdPayload", "SetProjectBitbucketConfigurationPropertyPayload", "SetProjectGitHubConfigurationPropertyByIdPayload", "SetProjectGitHubConfigurationPropertyPayload", "SetProjectGitLabConfigurationPropertyByIdPayload", "SetProjectGitLabConfigurationPropertyPayload", "SetProjectMessagePropertyByIdPayload", "SetProjectMessagePropertyPayload", "SetProjectMetaInfoPropertyByIdPayload", "SetProjectMetaInfoPropertyPayload", "SetProjectPropertyByIdPayload", "SetProjectPropertyPayload", "SetProjectStalePropertyByIdPayload", "SetProjectStalePropertyPayload", "SetPromotionLevelAutoPromotionPropertyByIdPayload", "SetPromotionLevelAutoPromotionPropertyPayload", "SetPromotionLevelMessagePropertyByIdPayload", "SetPromotionLevelMessagePropertyPayload", "SetPromotionLevelMetaInfoPropertyByIdPayload", "SetPromotionLevelMetaInfoPropertyPayload", "SetPromotionLevelPropertyByIdPayload", "SetPromotionLevelPropertyPayload", "SetPromotionRunMessagePropertyByIdPayload", "SetPromotionRunMessagePropertyPayload", "SetPromotionRunMetaInfoPropertyByIdPayload", "SetPromotionRunMetaInfoPropertyPayload", "SetPromotionRunPropertyByIdPayload", "SetPromotionRunPropertyPayload", "SetValidationRunMessagePropertyByIdPayload", "SetValidationRunMessagePropertyPayload", "SetValidationRunMetaInfoPropertyByIdPayload", "SetValidationRunMetaInfoPropertyPayload", "SetValidationRunPropertyByIdPayload", "SetValidationRunPropertyPayload", "SetValidationStampMessagePropertyByIdPayload", "SetValidationStampMessagePropertyPayload", "SetValidationStampMetaInfoPropertyByIdPayload", "SetValidationStampMetaInfoPropertyPayload", "SetValidationStampPropertyByIdPayload", "SetValidationStampPropertyPayload", "SetupCHMLValidationStampPayload", "SetupMetricsValidationStampPayload", "SetupPercentageValidationStampPayload", "SetupPromotionLevelPayload", "SetupTestSummaryValidationStampPayload", "SetupValidationStampPayload", "SubscribeToEventsPayload", "UnfavouriteProjectPayload", "UnlockAccountPayload", "UpdateProjectPayload", "ValidateBuildByIdWithCHMLPayload", "ValidateBuildByIdWithMetricsPayload", "ValidateBuildByIdWithPercentagePayload", "ValidateBuildByIdWithTestsPayload", "ValidateBuildWithCHMLPayload", "ValidateBuildWithMetricsPayload", "ValidateBuildWithPercentagePayload", "ValidateBuildWithTestsPayload"})
))
};
final PayloadUserErrors.Mapper payloadUserErrorsFieldMapper = new PayloadUserErrors.Mapper();
@Override
public @NotNull Fragments map(ResponseReader reader) {
final PayloadUserErrors payloadUserErrors = reader.readFragment($responseFields[0], new ResponseReader.ObjectReader() {
@Override
public PayloadUserErrors read(ResponseReader reader) {
return payloadUserErrorsFieldMapper.map(reader);
}
});
return new Fragments(payloadUserErrors);
}
}
}
public static final class Mapper implements ResponseFieldMapper {
final Webhook.Mapper webhookFieldMapper = new Webhook.Mapper();
final Fragments.Mapper fragmentsFieldMapper = new Fragments.Mapper();
@Override
public CreateWebhook map(ResponseReader reader) {
final String __typename = reader.readString($responseFields[0]);
final Webhook webhook = reader.readObject($responseFields[1], new ResponseReader.ObjectReader() {
@Override
public Webhook read(ResponseReader reader) {
return webhookFieldMapper.map(reader);
}
});
final Fragments fragments = fragmentsFieldMapper.map(reader);
return new CreateWebhook(__typename, webhook, fragments);
}
}
}
/**
* Webhook registration
*/
public static class Webhook {
static final ResponseField[] $responseFields = {
ResponseField.forString("__typename", "__typename", null, false, Collections.emptyList()),
ResponseField.forString("__typename", "__typename", null, false, Collections.emptyList())
};
final @NotNull String __typename;
private final @NotNull Fragments fragments;
private transient volatile String $toString;
private transient volatile int $hashCode;
private transient volatile boolean $hashCodeMemoized;
public Webhook(@NotNull String __typename, @NotNull Fragments fragments) {
this.__typename = Utils.checkNotNull(__typename, "__typename == null");
this.fragments = Utils.checkNotNull(fragments, "fragments == null");
}
public @NotNull String __typename() {
return this.__typename;
}
public @NotNull Fragments fragments() {
return this.fragments;
}
@SuppressWarnings({"rawtypes", "unchecked"})
public ResponseFieldMarshaller marshaller() {
return new ResponseFieldMarshaller() {
@Override
public void marshal(ResponseWriter writer) {
writer.writeString($responseFields[0], __typename);
fragments.marshaller().marshal(writer);
}
};
}
@Override
public String toString() {
if ($toString == null) {
$toString = "Webhook{"
+ "__typename=" + __typename + ", "
+ "fragments=" + fragments
+ "}";
}
return $toString;
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof Webhook) {
Webhook that = (Webhook) o;
return this.__typename.equals(that.__typename)
&& this.fragments.equals(that.fragments);
}
return false;
}
@Override
public int hashCode() {
if (!$hashCodeMemoized) {
int h = 1;
h *= 1000003;
h ^= __typename.hashCode();
h *= 1000003;
h ^= fragments.hashCode();
$hashCode = h;
$hashCodeMemoized = true;
}
return $hashCode;
}
public static class Fragments {
final @NotNull WebhookFragment webhookFragment;
private transient volatile String $toString;
private transient volatile int $hashCode;
private transient volatile boolean $hashCodeMemoized;
public Fragments(@NotNull WebhookFragment webhookFragment) {
this.webhookFragment = Utils.checkNotNull(webhookFragment, "webhookFragment == null");
}
public @NotNull WebhookFragment webhookFragment() {
return this.webhookFragment;
}
public ResponseFieldMarshaller marshaller() {
return new ResponseFieldMarshaller() {
@Override
public void marshal(ResponseWriter writer) {
writer.writeFragment(webhookFragment.marshaller());
}
};
}
@Override
public String toString() {
if ($toString == null) {
$toString = "Fragments{"
+ "webhookFragment=" + webhookFragment
+ "}";
}
return $toString;
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof Fragments) {
Fragments that = (Fragments) o;
return this.webhookFragment.equals(that.webhookFragment);
}
return false;
}
@Override
public int hashCode() {
if (!$hashCodeMemoized) {
int h = 1;
h *= 1000003;
h ^= webhookFragment.hashCode();
$hashCode = h;
$hashCodeMemoized = true;
}
return $hashCode;
}
public static final class Mapper implements ResponseFieldMapper {
static final ResponseField[] $responseFields = {
ResponseField.forFragment("__typename", "__typename", Collections.emptyList())
};
final WebhookFragment.Mapper webhookFragmentFieldMapper = new WebhookFragment.Mapper();
@Override
public @NotNull Fragments map(ResponseReader reader) {
final WebhookFragment webhookFragment = reader.readFragment($responseFields[0], new ResponseReader.ObjectReader() {
@Override
public WebhookFragment read(ResponseReader reader) {
return webhookFragmentFieldMapper.map(reader);
}
});
return new Fragments(webhookFragment);
}
}
}
public static final class Mapper implements ResponseFieldMapper {
final Fragments.Mapper fragmentsFieldMapper = new Fragments.Mapper();
@Override
public Webhook map(ResponseReader reader) {
final String __typename = reader.readString($responseFields[0]);
final Fragments fragments = fragmentsFieldMapper.map(reader);
return new Webhook(__typename, fragments);
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy