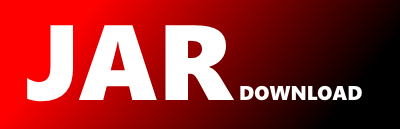
net.nemerosa.ontrack.kdsl.connector.graphql.schema.PromotionLevelGetPropertyQuery Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ontrack-kdsl Show documentation
Show all versions of ontrack-kdsl Show documentation
Ontrack module: ontrack-kdsl
// AUTO-GENERATED FILE. DO NOT MODIFY.
//
// This class was automatically generated by Apollo GraphQL plugin from the GraphQL queries it found.
// It should not be modified by hand.
//
package net.nemerosa.ontrack.kdsl.connector.graphql.schema;
import com.apollographql.apollo.api.Operation;
import com.apollographql.apollo.api.OperationName;
import com.apollographql.apollo.api.Query;
import com.apollographql.apollo.api.Response;
import com.apollographql.apollo.api.ResponseField;
import com.apollographql.apollo.api.ScalarTypeAdapters;
import com.apollographql.apollo.api.internal.InputFieldMarshaller;
import com.apollographql.apollo.api.internal.InputFieldWriter;
import com.apollographql.apollo.api.internal.OperationRequestBodyComposer;
import com.apollographql.apollo.api.internal.QueryDocumentMinifier;
import com.apollographql.apollo.api.internal.ResponseFieldMapper;
import com.apollographql.apollo.api.internal.ResponseFieldMarshaller;
import com.apollographql.apollo.api.internal.ResponseReader;
import com.apollographql.apollo.api.internal.ResponseWriter;
import com.apollographql.apollo.api.internal.SimpleOperationResponseParser;
import com.apollographql.apollo.api.internal.UnmodifiableMapBuilder;
import com.apollographql.apollo.api.internal.Utils;
import com.fasterxml.jackson.databind.JsonNode;
import java.io.IOException;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.SuppressWarnings;
import java.util.Collections;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import net.nemerosa.ontrack.kdsl.connector.graphql.schema.type.CustomType;
import okio.Buffer;
import okio.BufferedSource;
import okio.ByteString;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
public final class PromotionLevelGetPropertyQuery implements Query {
public static final String OPERATION_ID = "4c3fcb5c2315d8b7c664255c26889bf3e2e1cf50b05bdc22ddbcacdae38c7f4e";
public static final String QUERY_DOCUMENT = QueryDocumentMinifier.minify(
"query PromotionLevelGetProperty($id: Int!, $type: String!) {\n"
+ " promotionLevel(id: $id) {\n"
+ " __typename\n"
+ " properties(type: $type) {\n"
+ " __typename\n"
+ " value\n"
+ " }\n"
+ " }\n"
+ "}"
);
public static final OperationName OPERATION_NAME = new OperationName() {
@Override
public String name() {
return "PromotionLevelGetProperty";
}
};
private final PromotionLevelGetPropertyQuery.Variables variables;
public PromotionLevelGetPropertyQuery(int id, @NotNull String type) {
Utils.checkNotNull(type, "type == null");
variables = new PromotionLevelGetPropertyQuery.Variables(id, type);
}
@Override
public String operationId() {
return OPERATION_ID;
}
@Override
public String queryDocument() {
return QUERY_DOCUMENT;
}
@Override
public PromotionLevelGetPropertyQuery.Data wrapData(PromotionLevelGetPropertyQuery.Data data) {
return data;
}
@Override
public PromotionLevelGetPropertyQuery.Variables variables() {
return variables;
}
@Override
public ResponseFieldMapper responseFieldMapper() {
return new Data.Mapper();
}
public static Builder builder() {
return new Builder();
}
@Override
public OperationName name() {
return OPERATION_NAME;
}
@Override
@NotNull
public Response parse(@NotNull final BufferedSource source,
@NotNull final ScalarTypeAdapters scalarTypeAdapters) throws IOException {
return SimpleOperationResponseParser.parse(source, this, scalarTypeAdapters);
}
@Override
@NotNull
public Response parse(@NotNull final ByteString byteString,
@NotNull final ScalarTypeAdapters scalarTypeAdapters) throws IOException {
return parse(new Buffer().write(byteString), scalarTypeAdapters);
}
@Override
@NotNull
public Response parse(@NotNull final BufferedSource source)
throws IOException {
return parse(source, ScalarTypeAdapters.DEFAULT);
}
@Override
@NotNull
public Response parse(@NotNull final ByteString byteString)
throws IOException {
return parse(byteString, ScalarTypeAdapters.DEFAULT);
}
@Override
@NotNull
public ByteString composeRequestBody(@NotNull final ScalarTypeAdapters scalarTypeAdapters) {
return OperationRequestBodyComposer.compose(this, false, true, scalarTypeAdapters);
}
@NotNull
@Override
public ByteString composeRequestBody() {
return OperationRequestBodyComposer.compose(this, false, true, ScalarTypeAdapters.DEFAULT);
}
@Override
@NotNull
public ByteString composeRequestBody(final boolean autoPersistQueries,
final boolean withQueryDocument, @NotNull final ScalarTypeAdapters scalarTypeAdapters) {
return OperationRequestBodyComposer.compose(this, autoPersistQueries, withQueryDocument, scalarTypeAdapters);
}
public static final class Builder {
private int id;
private @NotNull String type;
Builder() {
}
public Builder id(int id) {
this.id = id;
return this;
}
public Builder type(@NotNull String type) {
this.type = type;
return this;
}
public PromotionLevelGetPropertyQuery build() {
Utils.checkNotNull(type, "type == null");
return new PromotionLevelGetPropertyQuery(id, type);
}
}
public static final class Variables extends Operation.Variables {
private final int id;
private final @NotNull String type;
private final transient Map valueMap = new LinkedHashMap<>();
Variables(int id, @NotNull String type) {
this.id = id;
this.type = type;
this.valueMap.put("id", id);
this.valueMap.put("type", type);
}
public int id() {
return id;
}
public @NotNull String type() {
return type;
}
@Override
public Map valueMap() {
return Collections.unmodifiableMap(valueMap);
}
@Override
public InputFieldMarshaller marshaller() {
return new InputFieldMarshaller() {
@Override
public void marshal(InputFieldWriter writer) throws IOException {
writer.writeInt("id", id);
writer.writeString("type", type);
}
};
}
}
/**
* Data from the response after executing this GraphQL operation
*/
public static class Data implements Operation.Data {
static final ResponseField[] $responseFields = {
ResponseField.forObject("promotionLevel", "promotionLevel", new UnmodifiableMapBuilder(1)
.put("id", new UnmodifiableMapBuilder(2)
.put("kind", "Variable")
.put("variableName", "id")
.build())
.build(), true, Collections.emptyList())
};
final @Nullable PromotionLevel promotionLevel;
private transient volatile String $toString;
private transient volatile int $hashCode;
private transient volatile boolean $hashCodeMemoized;
public Data(@Nullable PromotionLevel promotionLevel) {
this.promotionLevel = promotionLevel;
}
public @Nullable PromotionLevel promotionLevel() {
return this.promotionLevel;
}
@SuppressWarnings({"rawtypes", "unchecked"})
public ResponseFieldMarshaller marshaller() {
return new ResponseFieldMarshaller() {
@Override
public void marshal(ResponseWriter writer) {
writer.writeObject($responseFields[0], promotionLevel != null ? promotionLevel.marshaller() : null);
}
};
}
@Override
public String toString() {
if ($toString == null) {
$toString = "Data{"
+ "promotionLevel=" + promotionLevel
+ "}";
}
return $toString;
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof Data) {
Data that = (Data) o;
return ((this.promotionLevel == null) ? (that.promotionLevel == null) : this.promotionLevel.equals(that.promotionLevel));
}
return false;
}
@Override
public int hashCode() {
if (!$hashCodeMemoized) {
int h = 1;
h *= 1000003;
h ^= (promotionLevel == null) ? 0 : promotionLevel.hashCode();
$hashCode = h;
$hashCodeMemoized = true;
}
return $hashCode;
}
public static final class Mapper implements ResponseFieldMapper {
final PromotionLevel.Mapper promotionLevelFieldMapper = new PromotionLevel.Mapper();
@Override
public Data map(ResponseReader reader) {
final PromotionLevel promotionLevel = reader.readObject($responseFields[0], new ResponseReader.ObjectReader() {
@Override
public PromotionLevel read(ResponseReader reader) {
return promotionLevelFieldMapper.map(reader);
}
});
return new Data(promotionLevel);
}
}
}
public static class PromotionLevel {
static final ResponseField[] $responseFields = {
ResponseField.forString("__typename", "__typename", null, false, Collections.emptyList()),
ResponseField.forList("properties", "properties", new UnmodifiableMapBuilder(1)
.put("type", new UnmodifiableMapBuilder(2)
.put("kind", "Variable")
.put("variableName", "type")
.build())
.build(), false, Collections.emptyList())
};
final @NotNull String __typename;
final @NotNull List properties;
private transient volatile String $toString;
private transient volatile int $hashCode;
private transient volatile boolean $hashCodeMemoized;
public PromotionLevel(@NotNull String __typename, @NotNull List properties) {
this.__typename = Utils.checkNotNull(__typename, "__typename == null");
this.properties = Utils.checkNotNull(properties, "properties == null");
}
public @NotNull String __typename() {
return this.__typename;
}
/**
* List of properties
*/
public @NotNull List properties() {
return this.properties;
}
@SuppressWarnings({"rawtypes", "unchecked"})
public ResponseFieldMarshaller marshaller() {
return new ResponseFieldMarshaller() {
@Override
public void marshal(ResponseWriter writer) {
writer.writeString($responseFields[0], __typename);
writer.writeList($responseFields[1], properties, new ResponseWriter.ListWriter() {
@Override
public void write(List items, ResponseWriter.ListItemWriter listItemWriter) {
for (Object item : items) {
listItemWriter.writeObject(((Property) item).marshaller());
}
}
});
}
};
}
@Override
public String toString() {
if ($toString == null) {
$toString = "PromotionLevel{"
+ "__typename=" + __typename + ", "
+ "properties=" + properties
+ "}";
}
return $toString;
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof PromotionLevel) {
PromotionLevel that = (PromotionLevel) o;
return this.__typename.equals(that.__typename)
&& this.properties.equals(that.properties);
}
return false;
}
@Override
public int hashCode() {
if (!$hashCodeMemoized) {
int h = 1;
h *= 1000003;
h ^= __typename.hashCode();
h *= 1000003;
h ^= properties.hashCode();
$hashCode = h;
$hashCodeMemoized = true;
}
return $hashCode;
}
public static final class Mapper implements ResponseFieldMapper {
final Property.Mapper propertyFieldMapper = new Property.Mapper();
@Override
public PromotionLevel map(ResponseReader reader) {
final String __typename = reader.readString($responseFields[0]);
final List properties = reader.readList($responseFields[1], new ResponseReader.ListReader() {
@Override
public Property read(ResponseReader.ListItemReader listItemReader) {
return listItemReader.readObject(new ResponseReader.ObjectReader() {
@Override
public Property read(ResponseReader reader) {
return propertyFieldMapper.map(reader);
}
});
}
});
return new PromotionLevel(__typename, properties);
}
}
}
public static class Property {
static final ResponseField[] $responseFields = {
ResponseField.forString("__typename", "__typename", null, false, Collections.emptyList()),
ResponseField.forCustomType("value", "value", null, true, CustomType.JSON, Collections.emptyList())
};
final @NotNull String __typename;
final @Nullable JsonNode value;
private transient volatile String $toString;
private transient volatile int $hashCode;
private transient volatile boolean $hashCodeMemoized;
public Property(@NotNull String __typename, @Nullable JsonNode value) {
this.__typename = Utils.checkNotNull(__typename, "__typename == null");
this.value = value;
}
public @NotNull String __typename() {
return this.__typename;
}
/**
* JSON representation of the value
*/
public @Nullable JsonNode value() {
return this.value;
}
@SuppressWarnings({"rawtypes", "unchecked"})
public ResponseFieldMarshaller marshaller() {
return new ResponseFieldMarshaller() {
@Override
public void marshal(ResponseWriter writer) {
writer.writeString($responseFields[0], __typename);
writer.writeCustom((ResponseField.CustomTypeField) $responseFields[1], value);
}
};
}
@Override
public String toString() {
if ($toString == null) {
$toString = "Property{"
+ "__typename=" + __typename + ", "
+ "value=" + value
+ "}";
}
return $toString;
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof Property) {
Property that = (Property) o;
return this.__typename.equals(that.__typename)
&& ((this.value == null) ? (that.value == null) : this.value.equals(that.value));
}
return false;
}
@Override
public int hashCode() {
if (!$hashCodeMemoized) {
int h = 1;
h *= 1000003;
h ^= __typename.hashCode();
h *= 1000003;
h ^= (value == null) ? 0 : value.hashCode();
$hashCode = h;
$hashCodeMemoized = true;
}
return $hashCode;
}
public static final class Mapper implements ResponseFieldMapper {
@Override
public Property map(ResponseReader reader) {
final String __typename = reader.readString($responseFields[0]);
final JsonNode value = reader.readCustomType((ResponseField.CustomTypeField) $responseFields[1]);
return new Property(__typename, value);
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy