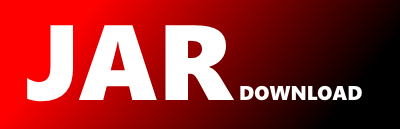
net.nemerosa.ontrack.kdsl.connector.graphql.schema.SearchQuery Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ontrack-kdsl Show documentation
Show all versions of ontrack-kdsl Show documentation
Ontrack module: ontrack-kdsl
// AUTO-GENERATED FILE. DO NOT MODIFY.
//
// This class was automatically generated by Apollo GraphQL plugin from the GraphQL queries it found.
// It should not be modified by hand.
//
package net.nemerosa.ontrack.kdsl.connector.graphql.schema;
import com.apollographql.apollo.api.Operation;
import com.apollographql.apollo.api.OperationName;
import com.apollographql.apollo.api.Query;
import com.apollographql.apollo.api.Response;
import com.apollographql.apollo.api.ResponseField;
import com.apollographql.apollo.api.ScalarTypeAdapters;
import com.apollographql.apollo.api.internal.InputFieldMarshaller;
import com.apollographql.apollo.api.internal.InputFieldWriter;
import com.apollographql.apollo.api.internal.OperationRequestBodyComposer;
import com.apollographql.apollo.api.internal.QueryDocumentMinifier;
import com.apollographql.apollo.api.internal.ResponseFieldMapper;
import com.apollographql.apollo.api.internal.ResponseFieldMarshaller;
import com.apollographql.apollo.api.internal.ResponseReader;
import com.apollographql.apollo.api.internal.ResponseWriter;
import com.apollographql.apollo.api.internal.SimpleOperationResponseParser;
import com.apollographql.apollo.api.internal.UnmodifiableMapBuilder;
import com.apollographql.apollo.api.internal.Utils;
import java.io.IOException;
import java.lang.Double;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.SuppressWarnings;
import java.util.Collections;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import net.nemerosa.ontrack.kdsl.connector.graphql.schema.fragment.PageInfoContent;
import okio.Buffer;
import okio.BufferedSource;
import okio.ByteString;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
public final class SearchQuery implements Query {
public static final String OPERATION_ID = "5ace273f2d0f0eddfb332f25199bc6a79b4d1ea7c5058b65cd562bd33b2535dc";
public static final String QUERY_DOCUMENT = QueryDocumentMinifier.minify(
"query Search($token: String!, $size: Int!) {\n"
+ " search(token: $token, size: $size) {\n"
+ " __typename\n"
+ " pageInfo {\n"
+ " __typename\n"
+ " ...PageInfoContent\n"
+ " }\n"
+ " pageItems {\n"
+ " __typename\n"
+ " type {\n"
+ " __typename\n"
+ " id\n"
+ " name\n"
+ " description\n"
+ " feature {\n"
+ " __typename\n"
+ " id\n"
+ " }\n"
+ " }\n"
+ " title\n"
+ " description\n"
+ " accuracy\n"
+ " }\n"
+ " }\n"
+ "}\n"
+ "fragment PageInfoContent on PageInfo {\n"
+ " __typename\n"
+ " totalSize\n"
+ " currentOffset\n"
+ " currentSize\n"
+ " previousPage {\n"
+ " __typename\n"
+ " offset\n"
+ " size\n"
+ " }\n"
+ " nextPage {\n"
+ " __typename\n"
+ " offset\n"
+ " size\n"
+ " }\n"
+ " pageTotal\n"
+ " pageIndex\n"
+ "}"
);
public static final OperationName OPERATION_NAME = new OperationName() {
@Override
public String name() {
return "Search";
}
};
private final SearchQuery.Variables variables;
public SearchQuery(@NotNull String token, int size) {
Utils.checkNotNull(token, "token == null");
variables = new SearchQuery.Variables(token, size);
}
@Override
public String operationId() {
return OPERATION_ID;
}
@Override
public String queryDocument() {
return QUERY_DOCUMENT;
}
@Override
public SearchQuery.Data wrapData(SearchQuery.Data data) {
return data;
}
@Override
public SearchQuery.Variables variables() {
return variables;
}
@Override
public ResponseFieldMapper responseFieldMapper() {
return new Data.Mapper();
}
public static Builder builder() {
return new Builder();
}
@Override
public OperationName name() {
return OPERATION_NAME;
}
@Override
@NotNull
public Response parse(@NotNull final BufferedSource source,
@NotNull final ScalarTypeAdapters scalarTypeAdapters) throws IOException {
return SimpleOperationResponseParser.parse(source, this, scalarTypeAdapters);
}
@Override
@NotNull
public Response parse(@NotNull final ByteString byteString,
@NotNull final ScalarTypeAdapters scalarTypeAdapters) throws IOException {
return parse(new Buffer().write(byteString), scalarTypeAdapters);
}
@Override
@NotNull
public Response parse(@NotNull final BufferedSource source) throws IOException {
return parse(source, ScalarTypeAdapters.DEFAULT);
}
@Override
@NotNull
public Response parse(@NotNull final ByteString byteString) throws IOException {
return parse(byteString, ScalarTypeAdapters.DEFAULT);
}
@Override
@NotNull
public ByteString composeRequestBody(@NotNull final ScalarTypeAdapters scalarTypeAdapters) {
return OperationRequestBodyComposer.compose(this, false, true, scalarTypeAdapters);
}
@NotNull
@Override
public ByteString composeRequestBody() {
return OperationRequestBodyComposer.compose(this, false, true, ScalarTypeAdapters.DEFAULT);
}
@Override
@NotNull
public ByteString composeRequestBody(final boolean autoPersistQueries,
final boolean withQueryDocument, @NotNull final ScalarTypeAdapters scalarTypeAdapters) {
return OperationRequestBodyComposer.compose(this, autoPersistQueries, withQueryDocument, scalarTypeAdapters);
}
public static final class Builder {
private @NotNull String token;
private int size;
Builder() {
}
public Builder token(@NotNull String token) {
this.token = token;
return this;
}
public Builder size(int size) {
this.size = size;
return this;
}
public SearchQuery build() {
Utils.checkNotNull(token, "token == null");
return new SearchQuery(token, size);
}
}
public static final class Variables extends Operation.Variables {
private final @NotNull String token;
private final int size;
private final transient Map valueMap = new LinkedHashMap<>();
Variables(@NotNull String token, int size) {
this.token = token;
this.size = size;
this.valueMap.put("token", token);
this.valueMap.put("size", size);
}
public @NotNull String token() {
return token;
}
public int size() {
return size;
}
@Override
public Map valueMap() {
return Collections.unmodifiableMap(valueMap);
}
@Override
public InputFieldMarshaller marshaller() {
return new InputFieldMarshaller() {
@Override
public void marshal(InputFieldWriter writer) throws IOException {
writer.writeString("token", token);
writer.writeInt("size", size);
}
};
}
}
/**
* Data from the response after executing this GraphQL operation
*/
public static class Data implements Operation.Data {
static final ResponseField[] $responseFields = {
ResponseField.forObject("search", "search", new UnmodifiableMapBuilder(2)
.put("token", new UnmodifiableMapBuilder(2)
.put("kind", "Variable")
.put("variableName", "token")
.build())
.put("size", new UnmodifiableMapBuilder(2)
.put("kind", "Variable")
.put("variableName", "size")
.build())
.build(), true, Collections.emptyList())
};
final @Nullable Search search;
private transient volatile String $toString;
private transient volatile int $hashCode;
private transient volatile boolean $hashCodeMemoized;
public Data(@Nullable Search search) {
this.search = search;
}
/**
* Performs a search in Ontrack
*/
public @Nullable Search search() {
return this.search;
}
@SuppressWarnings({"rawtypes", "unchecked"})
public ResponseFieldMarshaller marshaller() {
return new ResponseFieldMarshaller() {
@Override
public void marshal(ResponseWriter writer) {
writer.writeObject($responseFields[0], search != null ? search.marshaller() : null);
}
};
}
@Override
public String toString() {
if ($toString == null) {
$toString = "Data{"
+ "search=" + search
+ "}";
}
return $toString;
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof Data) {
Data that = (Data) o;
return ((this.search == null) ? (that.search == null) : this.search.equals(that.search));
}
return false;
}
@Override
public int hashCode() {
if (!$hashCodeMemoized) {
int h = 1;
h *= 1000003;
h ^= (search == null) ? 0 : search.hashCode();
$hashCode = h;
$hashCodeMemoized = true;
}
return $hashCode;
}
public static final class Mapper implements ResponseFieldMapper {
final Search.Mapper searchFieldMapper = new Search.Mapper();
@Override
public Data map(ResponseReader reader) {
final Search search = reader.readObject($responseFields[0], new ResponseReader.ObjectReader() {
@Override
public Search read(ResponseReader reader) {
return searchFieldMapper.map(reader);
}
});
return new Data(search);
}
}
}
public static class Search {
static final ResponseField[] $responseFields = {
ResponseField.forString("__typename", "__typename", null, false, Collections.emptyList()),
ResponseField.forObject("pageInfo", "pageInfo", null, true, Collections.emptyList()),
ResponseField.forList("pageItems", "pageItems", null, false, Collections.emptyList())
};
final @NotNull String __typename;
final @Nullable PageInfo pageInfo;
final @NotNull List pageItems;
private transient volatile String $toString;
private transient volatile int $hashCode;
private transient volatile boolean $hashCodeMemoized;
public Search(@NotNull String __typename, @Nullable PageInfo pageInfo,
@NotNull List pageItems) {
this.__typename = Utils.checkNotNull(__typename, "__typename == null");
this.pageInfo = pageInfo;
this.pageItems = Utils.checkNotNull(pageItems, "pageItems == null");
}
public @NotNull String __typename() {
return this.__typename;
}
/**
* Information about the current page
*/
public @Nullable PageInfo pageInfo() {
return this.pageInfo;
}
/**
* Items in the current page
*/
public @NotNull List pageItems() {
return this.pageItems;
}
@SuppressWarnings({"rawtypes", "unchecked"})
public ResponseFieldMarshaller marshaller() {
return new ResponseFieldMarshaller() {
@Override
public void marshal(ResponseWriter writer) {
writer.writeString($responseFields[0], __typename);
writer.writeObject($responseFields[1], pageInfo != null ? pageInfo.marshaller() : null);
writer.writeList($responseFields[2], pageItems, new ResponseWriter.ListWriter() {
@Override
public void write(List items, ResponseWriter.ListItemWriter listItemWriter) {
for (Object item : items) {
listItemWriter.writeObject(((PageItem) item).marshaller());
}
}
});
}
};
}
@Override
public String toString() {
if ($toString == null) {
$toString = "Search{"
+ "__typename=" + __typename + ", "
+ "pageInfo=" + pageInfo + ", "
+ "pageItems=" + pageItems
+ "}";
}
return $toString;
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof Search) {
Search that = (Search) o;
return this.__typename.equals(that.__typename)
&& ((this.pageInfo == null) ? (that.pageInfo == null) : this.pageInfo.equals(that.pageInfo))
&& this.pageItems.equals(that.pageItems);
}
return false;
}
@Override
public int hashCode() {
if (!$hashCodeMemoized) {
int h = 1;
h *= 1000003;
h ^= __typename.hashCode();
h *= 1000003;
h ^= (pageInfo == null) ? 0 : pageInfo.hashCode();
h *= 1000003;
h ^= pageItems.hashCode();
$hashCode = h;
$hashCodeMemoized = true;
}
return $hashCode;
}
public static final class Mapper implements ResponseFieldMapper {
final PageInfo.Mapper pageInfoFieldMapper = new PageInfo.Mapper();
final PageItem.Mapper pageItemFieldMapper = new PageItem.Mapper();
@Override
public Search map(ResponseReader reader) {
final String __typename = reader.readString($responseFields[0]);
final PageInfo pageInfo = reader.readObject($responseFields[1], new ResponseReader.ObjectReader() {
@Override
public PageInfo read(ResponseReader reader) {
return pageInfoFieldMapper.map(reader);
}
});
final List pageItems = reader.readList($responseFields[2], new ResponseReader.ListReader() {
@Override
public PageItem read(ResponseReader.ListItemReader listItemReader) {
return listItemReader.readObject(new ResponseReader.ObjectReader() {
@Override
public PageItem read(ResponseReader reader) {
return pageItemFieldMapper.map(reader);
}
});
}
});
return new Search(__typename, pageInfo, pageItems);
}
}
}
public static class PageInfo {
static final ResponseField[] $responseFields = {
ResponseField.forString("__typename", "__typename", null, false, Collections.emptyList()),
ResponseField.forString("__typename", "__typename", null, false, Collections.emptyList())
};
final @NotNull String __typename;
private final @NotNull Fragments fragments;
private transient volatile String $toString;
private transient volatile int $hashCode;
private transient volatile boolean $hashCodeMemoized;
public PageInfo(@NotNull String __typename, @NotNull Fragments fragments) {
this.__typename = Utils.checkNotNull(__typename, "__typename == null");
this.fragments = Utils.checkNotNull(fragments, "fragments == null");
}
public @NotNull String __typename() {
return this.__typename;
}
public @NotNull Fragments fragments() {
return this.fragments;
}
@SuppressWarnings({"rawtypes", "unchecked"})
public ResponseFieldMarshaller marshaller() {
return new ResponseFieldMarshaller() {
@Override
public void marshal(ResponseWriter writer) {
writer.writeString($responseFields[0], __typename);
fragments.marshaller().marshal(writer);
}
};
}
@Override
public String toString() {
if ($toString == null) {
$toString = "PageInfo{"
+ "__typename=" + __typename + ", "
+ "fragments=" + fragments
+ "}";
}
return $toString;
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof PageInfo) {
PageInfo that = (PageInfo) o;
return this.__typename.equals(that.__typename)
&& this.fragments.equals(that.fragments);
}
return false;
}
@Override
public int hashCode() {
if (!$hashCodeMemoized) {
int h = 1;
h *= 1000003;
h ^= __typename.hashCode();
h *= 1000003;
h ^= fragments.hashCode();
$hashCode = h;
$hashCodeMemoized = true;
}
return $hashCode;
}
public static class Fragments {
final @NotNull PageInfoContent pageInfoContent;
private transient volatile String $toString;
private transient volatile int $hashCode;
private transient volatile boolean $hashCodeMemoized;
public Fragments(@NotNull PageInfoContent pageInfoContent) {
this.pageInfoContent = Utils.checkNotNull(pageInfoContent, "pageInfoContent == null");
}
public @NotNull PageInfoContent pageInfoContent() {
return this.pageInfoContent;
}
public ResponseFieldMarshaller marshaller() {
return new ResponseFieldMarshaller() {
@Override
public void marshal(ResponseWriter writer) {
writer.writeFragment(pageInfoContent.marshaller());
}
};
}
@Override
public String toString() {
if ($toString == null) {
$toString = "Fragments{"
+ "pageInfoContent=" + pageInfoContent
+ "}";
}
return $toString;
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof Fragments) {
Fragments that = (Fragments) o;
return this.pageInfoContent.equals(that.pageInfoContent);
}
return false;
}
@Override
public int hashCode() {
if (!$hashCodeMemoized) {
int h = 1;
h *= 1000003;
h ^= pageInfoContent.hashCode();
$hashCode = h;
$hashCodeMemoized = true;
}
return $hashCode;
}
public static final class Mapper implements ResponseFieldMapper {
static final ResponseField[] $responseFields = {
ResponseField.forFragment("__typename", "__typename", Collections.emptyList())
};
final PageInfoContent.Mapper pageInfoContentFieldMapper = new PageInfoContent.Mapper();
@Override
public @NotNull Fragments map(ResponseReader reader) {
final PageInfoContent pageInfoContent = reader.readFragment($responseFields[0], new ResponseReader.ObjectReader() {
@Override
public PageInfoContent read(ResponseReader reader) {
return pageInfoContentFieldMapper.map(reader);
}
});
return new Fragments(pageInfoContent);
}
}
}
public static final class Mapper implements ResponseFieldMapper {
final Fragments.Mapper fragmentsFieldMapper = new Fragments.Mapper();
@Override
public PageInfo map(ResponseReader reader) {
final String __typename = reader.readString($responseFields[0]);
final Fragments fragments = fragmentsFieldMapper.map(reader);
return new PageInfo(__typename, fragments);
}
}
}
/**
* Search result
*/
public static class PageItem {
static final ResponseField[] $responseFields = {
ResponseField.forString("__typename", "__typename", null, false, Collections.emptyList()),
ResponseField.forObject("type", "type", null, true, Collections.emptyList()),
ResponseField.forString("title", "title", null, true, Collections.emptyList()),
ResponseField.forString("description", "description", null, true, Collections.emptyList()),
ResponseField.forDouble("accuracy", "accuracy", null, true, Collections.emptyList())
};
final @NotNull String __typename;
final @Nullable Type type;
final @Nullable String title;
final @Nullable String description;
final @Nullable Double accuracy;
private transient volatile String $toString;
private transient volatile int $hashCode;
private transient volatile boolean $hashCodeMemoized;
public PageItem(@NotNull String __typename, @Nullable Type type, @Nullable String title,
@Nullable String description, @Nullable Double accuracy) {
this.__typename = Utils.checkNotNull(__typename, "__typename == null");
this.type = type;
this.title = title;
this.description = description;
this.accuracy = accuracy;
}
public @NotNull String __typename() {
return this.__typename;
}
/**
* Type of result
*/
public @Nullable Type type() {
return this.type;
}
/**
* Short title
*/
public @Nullable String title() {
return this.title;
}
/**
* Description linked to the item being found
*/
public @Nullable String description() {
return this.description;
}
/**
* Score for the search
*/
public @Nullable Double accuracy() {
return this.accuracy;
}
@SuppressWarnings({"rawtypes", "unchecked"})
public ResponseFieldMarshaller marshaller() {
return new ResponseFieldMarshaller() {
@Override
public void marshal(ResponseWriter writer) {
writer.writeString($responseFields[0], __typename);
writer.writeObject($responseFields[1], type != null ? type.marshaller() : null);
writer.writeString($responseFields[2], title);
writer.writeString($responseFields[3], description);
writer.writeDouble($responseFields[4], accuracy);
}
};
}
@Override
public String toString() {
if ($toString == null) {
$toString = "PageItem{"
+ "__typename=" + __typename + ", "
+ "type=" + type + ", "
+ "title=" + title + ", "
+ "description=" + description + ", "
+ "accuracy=" + accuracy
+ "}";
}
return $toString;
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof PageItem) {
PageItem that = (PageItem) o;
return this.__typename.equals(that.__typename)
&& ((this.type == null) ? (that.type == null) : this.type.equals(that.type))
&& ((this.title == null) ? (that.title == null) : this.title.equals(that.title))
&& ((this.description == null) ? (that.description == null) : this.description.equals(that.description))
&& ((this.accuracy == null) ? (that.accuracy == null) : this.accuracy.equals(that.accuracy));
}
return false;
}
@Override
public int hashCode() {
if (!$hashCodeMemoized) {
int h = 1;
h *= 1000003;
h ^= __typename.hashCode();
h *= 1000003;
h ^= (type == null) ? 0 : type.hashCode();
h *= 1000003;
h ^= (title == null) ? 0 : title.hashCode();
h *= 1000003;
h ^= (description == null) ? 0 : description.hashCode();
h *= 1000003;
h ^= (accuracy == null) ? 0 : accuracy.hashCode();
$hashCode = h;
$hashCodeMemoized = true;
}
return $hashCode;
}
public static final class Mapper implements ResponseFieldMapper {
final Type.Mapper typeFieldMapper = new Type.Mapper();
@Override
public PageItem map(ResponseReader reader) {
final String __typename = reader.readString($responseFields[0]);
final Type type = reader.readObject($responseFields[1], new ResponseReader.ObjectReader() {
@Override
public Type read(ResponseReader reader) {
return typeFieldMapper.map(reader);
}
});
final String title = reader.readString($responseFields[2]);
final String description = reader.readString($responseFields[3]);
final Double accuracy = reader.readDouble($responseFields[4]);
return new PageItem(__typename, type, title, description, accuracy);
}
}
}
/**
* Type of search result
*/
public static class Type {
static final ResponseField[] $responseFields = {
ResponseField.forString("__typename", "__typename", null, false, Collections.emptyList()),
ResponseField.forString("id", "id", null, true, Collections.emptyList()),
ResponseField.forString("name", "name", null, true, Collections.emptyList()),
ResponseField.forString("description", "description", null, true, Collections.emptyList()),
ResponseField.forObject("feature", "feature", null, true, Collections.emptyList())
};
final @NotNull String __typename;
final @Nullable String id;
final @Nullable String name;
final @Nullable String description;
final @Nullable Feature feature;
private transient volatile String $toString;
private transient volatile int $hashCode;
private transient volatile boolean $hashCodeMemoized;
public Type(@NotNull String __typename, @Nullable String id, @Nullable String name,
@Nullable String description, @Nullable Feature feature) {
this.__typename = Utils.checkNotNull(__typename, "__typename == null");
this.id = id;
this.name = name;
this.description = description;
this.feature = feature;
}
public @NotNull String __typename() {
return this.__typename;
}
/**
* ID for the type of search result
*/
public @Nullable String id() {
return this.id;
}
/**
* Display name for the search result
*/
public @Nullable String name() {
return this.name;
}
/**
* Short help text explaining the format of the token
*/
public @Nullable String description() {
return this.description;
}
/**
* Associated feature
*/
public @Nullable Feature feature() {
return this.feature;
}
@SuppressWarnings({"rawtypes", "unchecked"})
public ResponseFieldMarshaller marshaller() {
return new ResponseFieldMarshaller() {
@Override
public void marshal(ResponseWriter writer) {
writer.writeString($responseFields[0], __typename);
writer.writeString($responseFields[1], id);
writer.writeString($responseFields[2], name);
writer.writeString($responseFields[3], description);
writer.writeObject($responseFields[4], feature != null ? feature.marshaller() : null);
}
};
}
@Override
public String toString() {
if ($toString == null) {
$toString = "Type{"
+ "__typename=" + __typename + ", "
+ "id=" + id + ", "
+ "name=" + name + ", "
+ "description=" + description + ", "
+ "feature=" + feature
+ "}";
}
return $toString;
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof Type) {
Type that = (Type) o;
return this.__typename.equals(that.__typename)
&& ((this.id == null) ? (that.id == null) : this.id.equals(that.id))
&& ((this.name == null) ? (that.name == null) : this.name.equals(that.name))
&& ((this.description == null) ? (that.description == null) : this.description.equals(that.description))
&& ((this.feature == null) ? (that.feature == null) : this.feature.equals(that.feature));
}
return false;
}
@Override
public int hashCode() {
if (!$hashCodeMemoized) {
int h = 1;
h *= 1000003;
h ^= __typename.hashCode();
h *= 1000003;
h ^= (id == null) ? 0 : id.hashCode();
h *= 1000003;
h ^= (name == null) ? 0 : name.hashCode();
h *= 1000003;
h ^= (description == null) ? 0 : description.hashCode();
h *= 1000003;
h ^= (feature == null) ? 0 : feature.hashCode();
$hashCode = h;
$hashCodeMemoized = true;
}
return $hashCode;
}
public static final class Mapper implements ResponseFieldMapper {
final Feature.Mapper featureFieldMapper = new Feature.Mapper();
@Override
public Type map(ResponseReader reader) {
final String __typename = reader.readString($responseFields[0]);
final String id = reader.readString($responseFields[1]);
final String name = reader.readString($responseFields[2]);
final String description = reader.readString($responseFields[3]);
final Feature feature = reader.readObject($responseFields[4], new ResponseReader.ObjectReader() {
@Override
public Feature read(ResponseReader reader) {
return featureFieldMapper.map(reader);
}
});
return new Type(__typename, id, name, description, feature);
}
}
}
public static class Feature {
static final ResponseField[] $responseFields = {
ResponseField.forString("__typename", "__typename", null, false, Collections.emptyList()),
ResponseField.forString("id", "id", null, true, Collections.emptyList())
};
final @NotNull String __typename;
final @Nullable String id;
private transient volatile String $toString;
private transient volatile int $hashCode;
private transient volatile boolean $hashCodeMemoized;
public Feature(@NotNull String __typename, @Nullable String id) {
this.__typename = Utils.checkNotNull(__typename, "__typename == null");
this.id = id;
}
public @NotNull String __typename() {
return this.__typename;
}
/**
* Feature ID
*/
public @Nullable String id() {
return this.id;
}
@SuppressWarnings({"rawtypes", "unchecked"})
public ResponseFieldMarshaller marshaller() {
return new ResponseFieldMarshaller() {
@Override
public void marshal(ResponseWriter writer) {
writer.writeString($responseFields[0], __typename);
writer.writeString($responseFields[1], id);
}
};
}
@Override
public String toString() {
if ($toString == null) {
$toString = "Feature{"
+ "__typename=" + __typename + ", "
+ "id=" + id
+ "}";
}
return $toString;
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof Feature) {
Feature that = (Feature) o;
return this.__typename.equals(that.__typename)
&& ((this.id == null) ? (that.id == null) : this.id.equals(that.id));
}
return false;
}
@Override
public int hashCode() {
if (!$hashCodeMemoized) {
int h = 1;
h *= 1000003;
h ^= __typename.hashCode();
h *= 1000003;
h ^= (id == null) ? 0 : id.hashCode();
$hashCode = h;
$hashCodeMemoized = true;
}
return $hashCode;
}
public static final class Mapper implements ResponseFieldMapper {
@Override
public Feature map(ResponseReader reader) {
final String __typename = reader.readString($responseFields[0]);
final String id = reader.readString($responseFields[1]);
return new Feature(__typename, id);
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy