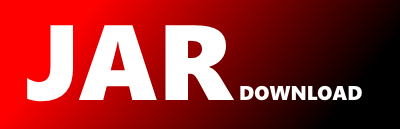
net.nemerosa.ontrack.kdsl.connector.graphql.schema.ValidateWithMetricsMutation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ontrack-kdsl Show documentation
Show all versions of ontrack-kdsl Show documentation
Ontrack module: ontrack-kdsl
// AUTO-GENERATED FILE. DO NOT MODIFY.
//
// This class was automatically generated by Apollo GraphQL plugin from the GraphQL queries it found.
// It should not be modified by hand.
//
package net.nemerosa.ontrack.kdsl.connector.graphql.schema;
import com.apollographql.apollo.api.Input;
import com.apollographql.apollo.api.Mutation;
import com.apollographql.apollo.api.Operation;
import com.apollographql.apollo.api.OperationName;
import com.apollographql.apollo.api.Response;
import com.apollographql.apollo.api.ResponseField;
import com.apollographql.apollo.api.ScalarTypeAdapters;
import com.apollographql.apollo.api.internal.InputFieldMarshaller;
import com.apollographql.apollo.api.internal.InputFieldWriter;
import com.apollographql.apollo.api.internal.OperationRequestBodyComposer;
import com.apollographql.apollo.api.internal.QueryDocumentMinifier;
import com.apollographql.apollo.api.internal.ResponseFieldMapper;
import com.apollographql.apollo.api.internal.ResponseFieldMarshaller;
import com.apollographql.apollo.api.internal.ResponseReader;
import com.apollographql.apollo.api.internal.ResponseWriter;
import com.apollographql.apollo.api.internal.SimpleOperationResponseParser;
import com.apollographql.apollo.api.internal.UnmodifiableMapBuilder;
import com.apollographql.apollo.api.internal.Utils;
import java.io.IOException;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.SuppressWarnings;
import java.util.Arrays;
import java.util.Collections;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import net.nemerosa.ontrack.kdsl.connector.graphql.schema.fragment.PayloadUserErrors;
import net.nemerosa.ontrack.kdsl.connector.graphql.schema.type.MetricsEntryInput;
import okio.Buffer;
import okio.BufferedSource;
import okio.ByteString;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
public final class ValidateWithMetricsMutation implements Mutation {
public static final String OPERATION_ID = "652338a04322df4fb21df22370d6aa3e2559b8dd4066896c19b82ed6379f0e2d";
public static final String QUERY_DOCUMENT = QueryDocumentMinifier.minify(
"mutation ValidateWithMetrics($buildId: Int!, $description: String, $validation: String!, $status: String, $metrics: [MetricsEntryInput!]!) {\n"
+ " validateBuildByIdWithMetrics(input: {id: $buildId, description: $description, validation: $validation, status: $status, metrics: $metrics}) {\n"
+ " __typename\n"
+ " ...PayloadUserErrors\n"
+ " }\n"
+ "}\n"
+ "fragment PayloadUserErrors on Payload {\n"
+ " __typename\n"
+ " errors {\n"
+ " __typename\n"
+ " message\n"
+ " exception\n"
+ " location\n"
+ " }\n"
+ "}"
);
public static final OperationName OPERATION_NAME = new OperationName() {
@Override
public String name() {
return "ValidateWithMetrics";
}
};
private final ValidateWithMetricsMutation.Variables variables;
public ValidateWithMetricsMutation(int buildId, @NotNull Input description,
@NotNull String validation, @NotNull Input status,
@NotNull List metrics) {
Utils.checkNotNull(description, "description == null");
Utils.checkNotNull(validation, "validation == null");
Utils.checkNotNull(status, "status == null");
Utils.checkNotNull(metrics, "metrics == null");
variables = new ValidateWithMetricsMutation.Variables(buildId, description, validation, status, metrics);
}
@Override
public String operationId() {
return OPERATION_ID;
}
@Override
public String queryDocument() {
return QUERY_DOCUMENT;
}
@Override
public ValidateWithMetricsMutation.Data wrapData(ValidateWithMetricsMutation.Data data) {
return data;
}
@Override
public ValidateWithMetricsMutation.Variables variables() {
return variables;
}
@Override
public ResponseFieldMapper responseFieldMapper() {
return new Data.Mapper();
}
public static Builder builder() {
return new Builder();
}
@Override
public OperationName name() {
return OPERATION_NAME;
}
@Override
@NotNull
public Response parse(@NotNull final BufferedSource source,
@NotNull final ScalarTypeAdapters scalarTypeAdapters) throws IOException {
return SimpleOperationResponseParser.parse(source, this, scalarTypeAdapters);
}
@Override
@NotNull
public Response parse(@NotNull final ByteString byteString,
@NotNull final ScalarTypeAdapters scalarTypeAdapters) throws IOException {
return parse(new Buffer().write(byteString), scalarTypeAdapters);
}
@Override
@NotNull
public Response parse(@NotNull final BufferedSource source)
throws IOException {
return parse(source, ScalarTypeAdapters.DEFAULT);
}
@Override
@NotNull
public Response parse(@NotNull final ByteString byteString)
throws IOException {
return parse(byteString, ScalarTypeAdapters.DEFAULT);
}
@Override
@NotNull
public ByteString composeRequestBody(@NotNull final ScalarTypeAdapters scalarTypeAdapters) {
return OperationRequestBodyComposer.compose(this, false, true, scalarTypeAdapters);
}
@NotNull
@Override
public ByteString composeRequestBody() {
return OperationRequestBodyComposer.compose(this, false, true, ScalarTypeAdapters.DEFAULT);
}
@Override
@NotNull
public ByteString composeRequestBody(final boolean autoPersistQueries,
final boolean withQueryDocument, @NotNull final ScalarTypeAdapters scalarTypeAdapters) {
return OperationRequestBodyComposer.compose(this, autoPersistQueries, withQueryDocument, scalarTypeAdapters);
}
public static final class Builder {
private int buildId;
private Input description = Input.absent();
private @NotNull String validation;
private Input status = Input.absent();
private @NotNull List metrics;
Builder() {
}
public Builder buildId(int buildId) {
this.buildId = buildId;
return this;
}
public Builder description(@Nullable String description) {
this.description = Input.fromNullable(description);
return this;
}
public Builder validation(@NotNull String validation) {
this.validation = validation;
return this;
}
public Builder status(@Nullable String status) {
this.status = Input.fromNullable(status);
return this;
}
public Builder metrics(@NotNull List metrics) {
this.metrics = metrics;
return this;
}
public Builder descriptionInput(@NotNull Input description) {
this.description = Utils.checkNotNull(description, "description == null");
return this;
}
public Builder statusInput(@NotNull Input status) {
this.status = Utils.checkNotNull(status, "status == null");
return this;
}
public ValidateWithMetricsMutation build() {
Utils.checkNotNull(validation, "validation == null");
Utils.checkNotNull(metrics, "metrics == null");
return new ValidateWithMetricsMutation(buildId, description, validation, status, metrics);
}
}
public static final class Variables extends Operation.Variables {
private final int buildId;
private final Input description;
private final @NotNull String validation;
private final Input status;
private final @NotNull List metrics;
private final transient Map valueMap = new LinkedHashMap<>();
Variables(int buildId, Input description, @NotNull String validation,
Input status, @NotNull List metrics) {
this.buildId = buildId;
this.description = description;
this.validation = validation;
this.status = status;
this.metrics = metrics;
this.valueMap.put("buildId", buildId);
if (description.defined) {
this.valueMap.put("description", description.value);
}
this.valueMap.put("validation", validation);
if (status.defined) {
this.valueMap.put("status", status.value);
}
this.valueMap.put("metrics", metrics);
}
public int buildId() {
return buildId;
}
public Input description() {
return description;
}
public @NotNull String validation() {
return validation;
}
public Input status() {
return status;
}
public @NotNull List metrics() {
return metrics;
}
@Override
public Map valueMap() {
return Collections.unmodifiableMap(valueMap);
}
@Override
public InputFieldMarshaller marshaller() {
return new InputFieldMarshaller() {
@Override
public void marshal(InputFieldWriter writer) throws IOException {
writer.writeInt("buildId", buildId);
if (description.defined) {
writer.writeString("description", description.value);
}
writer.writeString("validation", validation);
if (status.defined) {
writer.writeString("status", status.value);
}
writer.writeList("metrics", new InputFieldWriter.ListWriter() {
@Override
public void write(InputFieldWriter.ListItemWriter listItemWriter) throws IOException {
for (final MetricsEntryInput $item : metrics) {
listItemWriter.writeObject($item != null ? $item.marshaller() : null);
}
}
});
}
};
}
}
/**
* Data from the response after executing this GraphQL operation
*/
public static class Data implements Operation.Data {
static final ResponseField[] $responseFields = {
ResponseField.forObject("validateBuildByIdWithMetrics", "validateBuildByIdWithMetrics", new UnmodifiableMapBuilder(1)
.put("input", new UnmodifiableMapBuilder(5)
.put("id", new UnmodifiableMapBuilder(2)
.put("kind", "Variable")
.put("variableName", "buildId")
.build())
.put("description", new UnmodifiableMapBuilder(2)
.put("kind", "Variable")
.put("variableName", "description")
.build())
.put("validation", new UnmodifiableMapBuilder(2)
.put("kind", "Variable")
.put("variableName", "validation")
.build())
.put("status", new UnmodifiableMapBuilder(2)
.put("kind", "Variable")
.put("variableName", "status")
.build())
.put("metrics", new UnmodifiableMapBuilder(2)
.put("kind", "Variable")
.put("variableName", "metrics")
.build())
.build())
.build(), true, Collections.emptyList())
};
final @Nullable ValidateBuildByIdWithMetrics validateBuildByIdWithMetrics;
private transient volatile String $toString;
private transient volatile int $hashCode;
private transient volatile boolean $hashCodeMemoized;
public Data(@Nullable ValidateBuildByIdWithMetrics validateBuildByIdWithMetrics) {
this.validateBuildByIdWithMetrics = validateBuildByIdWithMetrics;
}
/**
* Validates a build identified by id with Metrics data
*/
public @Nullable ValidateBuildByIdWithMetrics validateBuildByIdWithMetrics() {
return this.validateBuildByIdWithMetrics;
}
@SuppressWarnings({"rawtypes", "unchecked"})
public ResponseFieldMarshaller marshaller() {
return new ResponseFieldMarshaller() {
@Override
public void marshal(ResponseWriter writer) {
writer.writeObject($responseFields[0], validateBuildByIdWithMetrics != null ? validateBuildByIdWithMetrics.marshaller() : null);
}
};
}
@Override
public String toString() {
if ($toString == null) {
$toString = "Data{"
+ "validateBuildByIdWithMetrics=" + validateBuildByIdWithMetrics
+ "}";
}
return $toString;
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof Data) {
Data that = (Data) o;
return ((this.validateBuildByIdWithMetrics == null) ? (that.validateBuildByIdWithMetrics == null) : this.validateBuildByIdWithMetrics.equals(that.validateBuildByIdWithMetrics));
}
return false;
}
@Override
public int hashCode() {
if (!$hashCodeMemoized) {
int h = 1;
h *= 1000003;
h ^= (validateBuildByIdWithMetrics == null) ? 0 : validateBuildByIdWithMetrics.hashCode();
$hashCode = h;
$hashCodeMemoized = true;
}
return $hashCode;
}
public static final class Mapper implements ResponseFieldMapper {
final ValidateBuildByIdWithMetrics.Mapper validateBuildByIdWithMetricsFieldMapper = new ValidateBuildByIdWithMetrics.Mapper();
@Override
public Data map(ResponseReader reader) {
final ValidateBuildByIdWithMetrics validateBuildByIdWithMetrics = reader.readObject($responseFields[0], new ResponseReader.ObjectReader() {
@Override
public ValidateBuildByIdWithMetrics read(ResponseReader reader) {
return validateBuildByIdWithMetricsFieldMapper.map(reader);
}
});
return new Data(validateBuildByIdWithMetrics);
}
}
}
/**
* Output type for the validateBuildByIdWithMetrics mutation.
*/
public static class ValidateBuildByIdWithMetrics {
static final ResponseField[] $responseFields = {
ResponseField.forString("__typename", "__typename", null, false, Collections.emptyList()),
ResponseField.forString("__typename", "__typename", null, false, Collections.emptyList())
};
final @NotNull String __typename;
private final @NotNull Fragments fragments;
private transient volatile String $toString;
private transient volatile int $hashCode;
private transient volatile boolean $hashCodeMemoized;
public ValidateBuildByIdWithMetrics(@NotNull String __typename, @NotNull Fragments fragments) {
this.__typename = Utils.checkNotNull(__typename, "__typename == null");
this.fragments = Utils.checkNotNull(fragments, "fragments == null");
}
public @NotNull String __typename() {
return this.__typename;
}
public @NotNull Fragments fragments() {
return this.fragments;
}
@SuppressWarnings({"rawtypes", "unchecked"})
public ResponseFieldMarshaller marshaller() {
return new ResponseFieldMarshaller() {
@Override
public void marshal(ResponseWriter writer) {
writer.writeString($responseFields[0], __typename);
fragments.marshaller().marshal(writer);
}
};
}
@Override
public String toString() {
if ($toString == null) {
$toString = "ValidateBuildByIdWithMetrics{"
+ "__typename=" + __typename + ", "
+ "fragments=" + fragments
+ "}";
}
return $toString;
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof ValidateBuildByIdWithMetrics) {
ValidateBuildByIdWithMetrics that = (ValidateBuildByIdWithMetrics) o;
return this.__typename.equals(that.__typename)
&& this.fragments.equals(that.fragments);
}
return false;
}
@Override
public int hashCode() {
if (!$hashCodeMemoized) {
int h = 1;
h *= 1000003;
h ^= __typename.hashCode();
h *= 1000003;
h ^= fragments.hashCode();
$hashCode = h;
$hashCodeMemoized = true;
}
return $hashCode;
}
public static class Fragments {
final @Nullable PayloadUserErrors payloadUserErrors;
private transient volatile String $toString;
private transient volatile int $hashCode;
private transient volatile boolean $hashCodeMemoized;
public Fragments(@Nullable PayloadUserErrors payloadUserErrors) {
this.payloadUserErrors = payloadUserErrors;
}
public @Nullable PayloadUserErrors payloadUserErrors() {
return this.payloadUserErrors;
}
public ResponseFieldMarshaller marshaller() {
return new ResponseFieldMarshaller() {
@Override
public void marshal(ResponseWriter writer) {
final PayloadUserErrors $payloadUserErrors = payloadUserErrors;
if ($payloadUserErrors != null) {
writer.writeFragment($payloadUserErrors.marshaller());
}
}
};
}
@Override
public String toString() {
if ($toString == null) {
$toString = "Fragments{"
+ "payloadUserErrors=" + payloadUserErrors
+ "}";
}
return $toString;
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof Fragments) {
Fragments that = (Fragments) o;
return ((this.payloadUserErrors == null) ? (that.payloadUserErrors == null) : this.payloadUserErrors.equals(that.payloadUserErrors));
}
return false;
}
@Override
public int hashCode() {
if (!$hashCodeMemoized) {
int h = 1;
h *= 1000003;
h ^= (payloadUserErrors == null) ? 0 : payloadUserErrors.hashCode();
$hashCode = h;
$hashCodeMemoized = true;
}
return $hashCode;
}
public static final class Mapper implements ResponseFieldMapper {
static final ResponseField[] $responseFields = {
ResponseField.forFragment("__typename", "__typename", Arrays.asList(
ResponseField.Condition.typeCondition(new String[] {"CheckAutoVersioningPayload", "CreateBranchOrGetPayload", "CreateBranchPayload", "CreateBuildOrGetPayload", "CreateBuildPayload", "CreateGitHubConfigurationPayload", "CreateProjectOrGetPayload", "CreateProjectPayload", "CreatePromotionLevelByIdPayload", "CreatePromotionRunByIdPayload", "CreatePromotionRunPayload", "CreateValidationRunByIdPayload", "CreateValidationRunPayload", "CreateValidationStampByIdPayload", "CreateWebhookPayload", "DeleteBranchGitConfigPropertyByIdPayload", "DeleteBranchGitConfigPropertyPayload", "DeleteBranchMessagePropertyByIdPayload", "DeleteBranchMessagePropertyPayload", "DeleteBranchMetaInfoPropertyByIdPayload", "DeleteBranchMetaInfoPropertyPayload", "DeleteBuildGitCommitPropertyByIdPayload", "DeleteBuildGitCommitPropertyPayload", "DeleteBuildMessagePropertyByIdPayload", "DeleteBuildMessagePropertyPayload", "DeleteBuildMetaInfoPropertyByIdPayload", "DeleteBuildMetaInfoPropertyPayload", "DeleteBuildReleasePropertyByIdPayload", "DeleteBuildReleasePropertyPayload", "DeleteNotificationRecordsPayload", "DeleteProjectAutoPromotionLevelPropertyByIdPayload", "DeleteProjectAutoPromotionLevelPropertyPayload", "DeleteProjectAutoValidationStampPropertyByIdPayload", "DeleteProjectAutoValidationStampPropertyPayload", "DeleteProjectBitbucketCloudConfigurationPropertyByIdPayload", "DeleteProjectBitbucketCloudConfigurationPropertyPayload", "DeleteProjectBitbucketConfigurationPropertyByIdPayload", "DeleteProjectBitbucketConfigurationPropertyPayload", "DeleteProjectGitHubConfigurationPropertyByIdPayload", "DeleteProjectGitHubConfigurationPropertyPayload", "DeleteProjectGitLabConfigurationPropertyByIdPayload", "DeleteProjectGitLabConfigurationPropertyPayload", "DeleteProjectMessagePropertyByIdPayload", "DeleteProjectMessagePropertyPayload", "DeleteProjectMetaInfoPropertyByIdPayload", "DeleteProjectMetaInfoPropertyPayload", "DeleteProjectPayload", "DeleteProjectStalePropertyByIdPayload", "DeleteProjectStalePropertyPayload", "DeletePromotionLevelAutoPromotionPropertyByIdPayload", "DeletePromotionLevelAutoPromotionPropertyPayload", "DeletePromotionLevelMessagePropertyByIdPayload", "DeletePromotionLevelMessagePropertyPayload", "DeletePromotionLevelMetaInfoPropertyByIdPayload", "DeletePromotionLevelMetaInfoPropertyPayload", "DeletePromotionRunMessagePropertyByIdPayload", "DeletePromotionRunMessagePropertyPayload", "DeletePromotionRunMetaInfoPropertyByIdPayload", "DeletePromotionRunMetaInfoPropertyPayload", "DeleteSubscriptionPayload", "DeleteValidationRunMessagePropertyByIdPayload", "DeleteValidationRunMessagePropertyPayload", "DeleteValidationRunMetaInfoPropertyByIdPayload", "DeleteValidationRunMetaInfoPropertyPayload", "DeleteValidationStampMessagePropertyByIdPayload", "DeleteValidationStampMessagePropertyPayload", "DeleteValidationStampMetaInfoPropertyByIdPayload", "DeleteValidationStampMetaInfoPropertyPayload", "DeleteWebhookPayload", "DisableAccountPayload", "DisableProjectPayload", "DisableSubscriptionPayload", "EnableAccountPayload", "EnableProjectPayload", "EnableSubscriptionPayload", "FavouriteProjectPayload", "GitHubCheckAutoVersioningByBuildLabelPayload", "GitHubCheckAutoVersioningByBuildNamePayload", "GitHubCheckAutoVersioningByRunIdPayload", "GitHubIngestionBuildLinksByBuildLabelPayload", "GitHubIngestionBuildLinksByBuildNamePayload", "GitHubIngestionBuildLinksByRunIdPayload", "GitHubIngestionValidateDataByBuildLabelPayload", "GitHubIngestionValidateDataByBuildNamePayload", "GitHubIngestionValidateDataByRunIdPayload", "LinkBuildByIdPayload", "LinkBuildPayload", "LinksBuildPayload", "LockAccountPayload", "ReloadCascPayload", "SetAutoVersioningConfigByNamePayload", "SetAutoVersioningConfigPayload", "SetBranchGitConfigPropertyByIdPayload", "SetBranchGitConfigPropertyPayload", "SetBranchGitHubIngestionConfigPayload", "SetBranchMessagePropertyByIdPayload", "SetBranchMessagePropertyPayload", "SetBranchMetaInfoPropertyByIdPayload", "SetBranchMetaInfoPropertyPayload", "SetBranchPropertyByIdPayload", "SetBranchPropertyPayload", "SetBuildGitCommitPropertyByIdPayload", "SetBuildGitCommitPropertyPayload", "SetBuildMessagePropertyByIdPayload", "SetBuildMessagePropertyPayload", "SetBuildMetaInfoPropertyByIdPayload", "SetBuildMetaInfoPropertyPayload", "SetBuildPropertyByIdPayload", "SetBuildPropertyPayload", "SetBuildReleasePropertyByIdPayload", "SetBuildReleasePropertyPayload", "SetPreferencesPayload", "SetProjectAutoPromotionLevelPropertyByIdPayload", "SetProjectAutoPromotionLevelPropertyPayload", "SetProjectAutoValidationStampPropertyByIdPayload", "SetProjectAutoValidationStampPropertyPayload", "SetProjectBitbucketCloudConfigurationPropertyByIdPayload", "SetProjectBitbucketCloudConfigurationPropertyPayload", "SetProjectBitbucketConfigurationPropertyByIdPayload", "SetProjectBitbucketConfigurationPropertyPayload", "SetProjectGitHubConfigurationPropertyByIdPayload", "SetProjectGitHubConfigurationPropertyPayload", "SetProjectGitLabConfigurationPropertyByIdPayload", "SetProjectGitLabConfigurationPropertyPayload", "SetProjectMessagePropertyByIdPayload", "SetProjectMessagePropertyPayload", "SetProjectMetaInfoPropertyByIdPayload", "SetProjectMetaInfoPropertyPayload", "SetProjectPropertyByIdPayload", "SetProjectPropertyPayload", "SetProjectStalePropertyByIdPayload", "SetProjectStalePropertyPayload", "SetPromotionLevelAutoPromotionPropertyByIdPayload", "SetPromotionLevelAutoPromotionPropertyPayload", "SetPromotionLevelMessagePropertyByIdPayload", "SetPromotionLevelMessagePropertyPayload", "SetPromotionLevelMetaInfoPropertyByIdPayload", "SetPromotionLevelMetaInfoPropertyPayload", "SetPromotionLevelPropertyByIdPayload", "SetPromotionLevelPropertyPayload", "SetPromotionRunMessagePropertyByIdPayload", "SetPromotionRunMessagePropertyPayload", "SetPromotionRunMetaInfoPropertyByIdPayload", "SetPromotionRunMetaInfoPropertyPayload", "SetPromotionRunPropertyByIdPayload", "SetPromotionRunPropertyPayload", "SetValidationRunMessagePropertyByIdPayload", "SetValidationRunMessagePropertyPayload", "SetValidationRunMetaInfoPropertyByIdPayload", "SetValidationRunMetaInfoPropertyPayload", "SetValidationRunPropertyByIdPayload", "SetValidationRunPropertyPayload", "SetValidationStampMessagePropertyByIdPayload", "SetValidationStampMessagePropertyPayload", "SetValidationStampMetaInfoPropertyByIdPayload", "SetValidationStampMetaInfoPropertyPayload", "SetValidationStampPropertyByIdPayload", "SetValidationStampPropertyPayload", "SetupCHMLValidationStampPayload", "SetupMetricsValidationStampPayload", "SetupPercentageValidationStampPayload", "SetupPromotionLevelPayload", "SetupTestSummaryValidationStampPayload", "SetupValidationStampPayload", "SubscribeToEventsPayload", "UnfavouriteProjectPayload", "UnlockAccountPayload", "UpdateProjectPayload", "ValidateBuildByIdWithCHMLPayload", "ValidateBuildByIdWithMetricsPayload", "ValidateBuildByIdWithPercentagePayload", "ValidateBuildByIdWithTestsPayload", "ValidateBuildWithCHMLPayload", "ValidateBuildWithMetricsPayload", "ValidateBuildWithPercentagePayload", "ValidateBuildWithTestsPayload"})
))
};
final PayloadUserErrors.Mapper payloadUserErrorsFieldMapper = new PayloadUserErrors.Mapper();
@Override
public @NotNull Fragments map(ResponseReader reader) {
final PayloadUserErrors payloadUserErrors = reader.readFragment($responseFields[0], new ResponseReader.ObjectReader() {
@Override
public PayloadUserErrors read(ResponseReader reader) {
return payloadUserErrorsFieldMapper.map(reader);
}
});
return new Fragments(payloadUserErrors);
}
}
}
public static final class Mapper implements ResponseFieldMapper {
final Fragments.Mapper fragmentsFieldMapper = new Fragments.Mapper();
@Override
public ValidateBuildByIdWithMetrics map(ResponseReader reader) {
final String __typename = reader.readString($responseFields[0]);
final Fragments fragments = fragmentsFieldMapper.map(reader);
return new ValidateBuildByIdWithMetrics(__typename, fragments);
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy