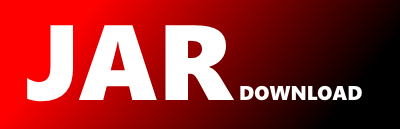
net.nemerosa.ontrack.kdsl.connector.graphql.schema.WebhookDeliveriesQuery Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ontrack-kdsl Show documentation
Show all versions of ontrack-kdsl Show documentation
Ontrack module: ontrack-kdsl
// AUTO-GENERATED FILE. DO NOT MODIFY.
//
// This class was automatically generated by Apollo GraphQL plugin from the GraphQL queries it found.
// It should not be modified by hand.
//
package net.nemerosa.ontrack.kdsl.connector.graphql.schema;
import com.apollographql.apollo.api.Input;
import com.apollographql.apollo.api.Operation;
import com.apollographql.apollo.api.OperationName;
import com.apollographql.apollo.api.Query;
import com.apollographql.apollo.api.ResponseField;
import com.apollographql.apollo.api.ScalarTypeAdapters;
import com.apollographql.apollo.api.internal.InputFieldMarshaller;
import com.apollographql.apollo.api.internal.InputFieldWriter;
import com.apollographql.apollo.api.internal.OperationRequestBodyComposer;
import com.apollographql.apollo.api.internal.QueryDocumentMinifier;
import com.apollographql.apollo.api.internal.ResponseFieldMapper;
import com.apollographql.apollo.api.internal.ResponseFieldMarshaller;
import com.apollographql.apollo.api.internal.ResponseReader;
import com.apollographql.apollo.api.internal.ResponseWriter;
import com.apollographql.apollo.api.internal.SimpleOperationResponseParser;
import com.apollographql.apollo.api.internal.UnmodifiableMapBuilder;
import com.apollographql.apollo.api.internal.Utils;
import java.io.IOException;
import java.lang.Integer;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.SuppressWarnings;
import java.time.LocalDateTime;
import java.util.Collections;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.UUID;
import net.nemerosa.ontrack.kdsl.connector.graphql.schema.fragment.PageInfoContent;
import net.nemerosa.ontrack.kdsl.connector.graphql.schema.type.CustomType;
import okio.Buffer;
import okio.BufferedSource;
import okio.ByteString;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
public final class WebhookDeliveriesQuery implements Query {
public static final String OPERATION_ID = "da50168f6ce4b390825886c531a8c3a2b97938631a796b2ac1f8c10c92e342fa";
public static final String QUERY_DOCUMENT = QueryDocumentMinifier.minify(
"query WebhookDeliveries($offset: Int = 0, $size: Int = 10, $webhook: String!) {\n"
+ " webhooks(name: $webhook) {\n"
+ " __typename\n"
+ " exchanges(offset: $offset, size: $size, filter: {webhook: $webhook}) {\n"
+ " __typename\n"
+ " pageInfo {\n"
+ " __typename\n"
+ " ...PageInfoContent\n"
+ " }\n"
+ " pageItems {\n"
+ " __typename\n"
+ " uuid\n"
+ " webhook\n"
+ " request {\n"
+ " __typename\n"
+ " timestamp\n"
+ " type\n"
+ " payload\n"
+ " }\n"
+ " response {\n"
+ " __typename\n"
+ " timestamp\n"
+ " code\n"
+ " payload\n"
+ " }\n"
+ " stack\n"
+ " }\n"
+ " }\n"
+ " }\n"
+ "}\n"
+ "fragment PageInfoContent on PageInfo {\n"
+ " __typename\n"
+ " totalSize\n"
+ " currentOffset\n"
+ " currentSize\n"
+ " previousPage {\n"
+ " __typename\n"
+ " offset\n"
+ " size\n"
+ " }\n"
+ " nextPage {\n"
+ " __typename\n"
+ " offset\n"
+ " size\n"
+ " }\n"
+ " pageTotal\n"
+ " pageIndex\n"
+ "}"
);
public static final OperationName OPERATION_NAME = new OperationName() {
@Override
public String name() {
return "WebhookDeliveries";
}
};
private final WebhookDeliveriesQuery.Variables variables;
public WebhookDeliveriesQuery(@NotNull Input offset, @NotNull Input size,
@NotNull String webhook) {
Utils.checkNotNull(offset, "offset == null");
Utils.checkNotNull(size, "size == null");
Utils.checkNotNull(webhook, "webhook == null");
variables = new WebhookDeliveriesQuery.Variables(offset, size, webhook);
}
@Override
public String operationId() {
return OPERATION_ID;
}
@Override
public String queryDocument() {
return QUERY_DOCUMENT;
}
@Override
public WebhookDeliveriesQuery.Data wrapData(WebhookDeliveriesQuery.Data data) {
return data;
}
@Override
public WebhookDeliveriesQuery.Variables variables() {
return variables;
}
@Override
public ResponseFieldMapper responseFieldMapper() {
return new Data.Mapper();
}
public static Builder builder() {
return new Builder();
}
@Override
public OperationName name() {
return OPERATION_NAME;
}
@Override
@NotNull
public com.apollographql.apollo.api.Response parse(@NotNull final BufferedSource source,
@NotNull final ScalarTypeAdapters scalarTypeAdapters) throws IOException {
return SimpleOperationResponseParser.parse(source, this, scalarTypeAdapters);
}
@Override
@NotNull
public com.apollographql.apollo.api.Response parse(@NotNull final ByteString byteString,
@NotNull final ScalarTypeAdapters scalarTypeAdapters) throws IOException {
return parse(new Buffer().write(byteString), scalarTypeAdapters);
}
@Override
@NotNull
public com.apollographql.apollo.api.Response parse(@NotNull final BufferedSource source)
throws IOException {
return parse(source, ScalarTypeAdapters.DEFAULT);
}
@Override
@NotNull
public com.apollographql.apollo.api.Response parse(@NotNull final ByteString byteString)
throws IOException {
return parse(byteString, ScalarTypeAdapters.DEFAULT);
}
@Override
@NotNull
public ByteString composeRequestBody(@NotNull final ScalarTypeAdapters scalarTypeAdapters) {
return OperationRequestBodyComposer.compose(this, false, true, scalarTypeAdapters);
}
@NotNull
@Override
public ByteString composeRequestBody() {
return OperationRequestBodyComposer.compose(this, false, true, ScalarTypeAdapters.DEFAULT);
}
@Override
@NotNull
public ByteString composeRequestBody(final boolean autoPersistQueries,
final boolean withQueryDocument, @NotNull final ScalarTypeAdapters scalarTypeAdapters) {
return OperationRequestBodyComposer.compose(this, autoPersistQueries, withQueryDocument, scalarTypeAdapters);
}
public static final class Builder {
private Input offset = Input.absent();
private Input size = Input.absent();
private @NotNull String webhook;
Builder() {
}
public Builder offset(@Nullable Integer offset) {
this.offset = Input.fromNullable(offset);
return this;
}
public Builder size(@Nullable Integer size) {
this.size = Input.fromNullable(size);
return this;
}
public Builder webhook(@NotNull String webhook) {
this.webhook = webhook;
return this;
}
public Builder offsetInput(@NotNull Input offset) {
this.offset = Utils.checkNotNull(offset, "offset == null");
return this;
}
public Builder sizeInput(@NotNull Input size) {
this.size = Utils.checkNotNull(size, "size == null");
return this;
}
public WebhookDeliveriesQuery build() {
Utils.checkNotNull(webhook, "webhook == null");
return new WebhookDeliveriesQuery(offset, size, webhook);
}
}
public static final class Variables extends Operation.Variables {
private final Input offset;
private final Input size;
private final @NotNull String webhook;
private final transient Map valueMap = new LinkedHashMap<>();
Variables(Input offset, Input size, @NotNull String webhook) {
this.offset = offset;
this.size = size;
this.webhook = webhook;
if (offset.defined) {
this.valueMap.put("offset", offset.value);
}
if (size.defined) {
this.valueMap.put("size", size.value);
}
this.valueMap.put("webhook", webhook);
}
public Input offset() {
return offset;
}
public Input size() {
return size;
}
public @NotNull String webhook() {
return webhook;
}
@Override
public Map valueMap() {
return Collections.unmodifiableMap(valueMap);
}
@Override
public InputFieldMarshaller marshaller() {
return new InputFieldMarshaller() {
@Override
public void marshal(InputFieldWriter writer) throws IOException {
if (offset.defined) {
writer.writeInt("offset", offset.value);
}
if (size.defined) {
writer.writeInt("size", size.value);
}
writer.writeString("webhook", webhook);
}
};
}
}
/**
* Data from the response after executing this GraphQL operation
*/
public static class Data implements Operation.Data {
static final ResponseField[] $responseFields = {
ResponseField.forList("webhooks", "webhooks", new UnmodifiableMapBuilder(1)
.put("name", new UnmodifiableMapBuilder(2)
.put("kind", "Variable")
.put("variableName", "webhook")
.build())
.build(), false, Collections.emptyList())
};
final @NotNull List webhooks;
private transient volatile String $toString;
private transient volatile int $hashCode;
private transient volatile boolean $hashCodeMemoized;
public Data(@NotNull List webhooks) {
this.webhooks = Utils.checkNotNull(webhooks, "webhooks == null");
}
/**
* List of registred webhooks
*/
public @NotNull List webhooks() {
return this.webhooks;
}
@SuppressWarnings({"rawtypes", "unchecked"})
public ResponseFieldMarshaller marshaller() {
return new ResponseFieldMarshaller() {
@Override
public void marshal(ResponseWriter writer) {
writer.writeList($responseFields[0], webhooks, new ResponseWriter.ListWriter() {
@Override
public void write(List items, ResponseWriter.ListItemWriter listItemWriter) {
for (Object item : items) {
listItemWriter.writeObject(((Webhook) item).marshaller());
}
}
});
}
};
}
@Override
public String toString() {
if ($toString == null) {
$toString = "Data{"
+ "webhooks=" + webhooks
+ "}";
}
return $toString;
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof Data) {
Data that = (Data) o;
return this.webhooks.equals(that.webhooks);
}
return false;
}
@Override
public int hashCode() {
if (!$hashCodeMemoized) {
int h = 1;
h *= 1000003;
h ^= webhooks.hashCode();
$hashCode = h;
$hashCodeMemoized = true;
}
return $hashCode;
}
public static final class Mapper implements ResponseFieldMapper {
final Webhook.Mapper webhookFieldMapper = new Webhook.Mapper();
@Override
public Data map(ResponseReader reader) {
final List webhooks = reader.readList($responseFields[0], new ResponseReader.ListReader() {
@Override
public Webhook read(ResponseReader.ListItemReader listItemReader) {
return listItemReader.readObject(new ResponseReader.ObjectReader() {
@Override
public Webhook read(ResponseReader reader) {
return webhookFieldMapper.map(reader);
}
});
}
});
return new Data(webhooks);
}
}
}
/**
* Webhook registration
*/
public static class Webhook {
static final ResponseField[] $responseFields = {
ResponseField.forString("__typename", "__typename", null, false, Collections.emptyList()),
ResponseField.forObject("exchanges", "exchanges", new UnmodifiableMapBuilder(3)
.put("offset", new UnmodifiableMapBuilder(2)
.put("kind", "Variable")
.put("variableName", "offset")
.build())
.put("size", new UnmodifiableMapBuilder(2)
.put("kind", "Variable")
.put("variableName", "size")
.build())
.put("filter", new UnmodifiableMapBuilder(1)
.put("webhook", new UnmodifiableMapBuilder(2)
.put("kind", "Variable")
.put("variableName", "webhook")
.build())
.build())
.build(), true, Collections.emptyList())
};
final @NotNull String __typename;
final @Nullable Exchanges exchanges;
private transient volatile String $toString;
private transient volatile int $hashCode;
private transient volatile boolean $hashCodeMemoized;
public Webhook(@NotNull String __typename, @Nullable Exchanges exchanges) {
this.__typename = Utils.checkNotNull(__typename, "__typename == null");
this.exchanges = exchanges;
}
public @NotNull String __typename() {
return this.__typename;
}
/**
* Exchanges for this webhook
*/
public @Nullable Exchanges exchanges() {
return this.exchanges;
}
@SuppressWarnings({"rawtypes", "unchecked"})
public ResponseFieldMarshaller marshaller() {
return new ResponseFieldMarshaller() {
@Override
public void marshal(ResponseWriter writer) {
writer.writeString($responseFields[0], __typename);
writer.writeObject($responseFields[1], exchanges != null ? exchanges.marshaller() : null);
}
};
}
@Override
public String toString() {
if ($toString == null) {
$toString = "Webhook{"
+ "__typename=" + __typename + ", "
+ "exchanges=" + exchanges
+ "}";
}
return $toString;
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof Webhook) {
Webhook that = (Webhook) o;
return this.__typename.equals(that.__typename)
&& ((this.exchanges == null) ? (that.exchanges == null) : this.exchanges.equals(that.exchanges));
}
return false;
}
@Override
public int hashCode() {
if (!$hashCodeMemoized) {
int h = 1;
h *= 1000003;
h ^= __typename.hashCode();
h *= 1000003;
h ^= (exchanges == null) ? 0 : exchanges.hashCode();
$hashCode = h;
$hashCodeMemoized = true;
}
return $hashCode;
}
public static final class Mapper implements ResponseFieldMapper {
final Exchanges.Mapper exchangesFieldMapper = new Exchanges.Mapper();
@Override
public Webhook map(ResponseReader reader) {
final String __typename = reader.readString($responseFields[0]);
final Exchanges exchanges = reader.readObject($responseFields[1], new ResponseReader.ObjectReader() {
@Override
public Exchanges read(ResponseReader reader) {
return exchangesFieldMapper.map(reader);
}
});
return new Webhook(__typename, exchanges);
}
}
}
public static class Exchanges {
static final ResponseField[] $responseFields = {
ResponseField.forString("__typename", "__typename", null, false, Collections.emptyList()),
ResponseField.forObject("pageInfo", "pageInfo", null, true, Collections.emptyList()),
ResponseField.forList("pageItems", "pageItems", null, false, Collections.emptyList())
};
final @NotNull String __typename;
final @Nullable PageInfo pageInfo;
final @NotNull List pageItems;
private transient volatile String $toString;
private transient volatile int $hashCode;
private transient volatile boolean $hashCodeMemoized;
public Exchanges(@NotNull String __typename, @Nullable PageInfo pageInfo,
@NotNull List pageItems) {
this.__typename = Utils.checkNotNull(__typename, "__typename == null");
this.pageInfo = pageInfo;
this.pageItems = Utils.checkNotNull(pageItems, "pageItems == null");
}
public @NotNull String __typename() {
return this.__typename;
}
/**
* Information about the current page
*/
public @Nullable PageInfo pageInfo() {
return this.pageInfo;
}
/**
* Items in the current page
*/
public @NotNull List pageItems() {
return this.pageItems;
}
@SuppressWarnings({"rawtypes", "unchecked"})
public ResponseFieldMarshaller marshaller() {
return new ResponseFieldMarshaller() {
@Override
public void marshal(ResponseWriter writer) {
writer.writeString($responseFields[0], __typename);
writer.writeObject($responseFields[1], pageInfo != null ? pageInfo.marshaller() : null);
writer.writeList($responseFields[2], pageItems, new ResponseWriter.ListWriter() {
@Override
public void write(List items, ResponseWriter.ListItemWriter listItemWriter) {
for (Object item : items) {
listItemWriter.writeObject(((PageItem) item).marshaller());
}
}
});
}
};
}
@Override
public String toString() {
if ($toString == null) {
$toString = "Exchanges{"
+ "__typename=" + __typename + ", "
+ "pageInfo=" + pageInfo + ", "
+ "pageItems=" + pageItems
+ "}";
}
return $toString;
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof Exchanges) {
Exchanges that = (Exchanges) o;
return this.__typename.equals(that.__typename)
&& ((this.pageInfo == null) ? (that.pageInfo == null) : this.pageInfo.equals(that.pageInfo))
&& this.pageItems.equals(that.pageItems);
}
return false;
}
@Override
public int hashCode() {
if (!$hashCodeMemoized) {
int h = 1;
h *= 1000003;
h ^= __typename.hashCode();
h *= 1000003;
h ^= (pageInfo == null) ? 0 : pageInfo.hashCode();
h *= 1000003;
h ^= pageItems.hashCode();
$hashCode = h;
$hashCodeMemoized = true;
}
return $hashCode;
}
public static final class Mapper implements ResponseFieldMapper {
final PageInfo.Mapper pageInfoFieldMapper = new PageInfo.Mapper();
final PageItem.Mapper pageItemFieldMapper = new PageItem.Mapper();
@Override
public Exchanges map(ResponseReader reader) {
final String __typename = reader.readString($responseFields[0]);
final PageInfo pageInfo = reader.readObject($responseFields[1], new ResponseReader.ObjectReader() {
@Override
public PageInfo read(ResponseReader reader) {
return pageInfoFieldMapper.map(reader);
}
});
final List pageItems = reader.readList($responseFields[2], new ResponseReader.ListReader() {
@Override
public PageItem read(ResponseReader.ListItemReader listItemReader) {
return listItemReader.readObject(new ResponseReader.ObjectReader() {
@Override
public PageItem read(ResponseReader reader) {
return pageItemFieldMapper.map(reader);
}
});
}
});
return new Exchanges(__typename, pageInfo, pageItems);
}
}
}
public static class PageInfo {
static final ResponseField[] $responseFields = {
ResponseField.forString("__typename", "__typename", null, false, Collections.emptyList()),
ResponseField.forString("__typename", "__typename", null, false, Collections.emptyList())
};
final @NotNull String __typename;
private final @NotNull Fragments fragments;
private transient volatile String $toString;
private transient volatile int $hashCode;
private transient volatile boolean $hashCodeMemoized;
public PageInfo(@NotNull String __typename, @NotNull Fragments fragments) {
this.__typename = Utils.checkNotNull(__typename, "__typename == null");
this.fragments = Utils.checkNotNull(fragments, "fragments == null");
}
public @NotNull String __typename() {
return this.__typename;
}
public @NotNull Fragments fragments() {
return this.fragments;
}
@SuppressWarnings({"rawtypes", "unchecked"})
public ResponseFieldMarshaller marshaller() {
return new ResponseFieldMarshaller() {
@Override
public void marshal(ResponseWriter writer) {
writer.writeString($responseFields[0], __typename);
fragments.marshaller().marshal(writer);
}
};
}
@Override
public String toString() {
if ($toString == null) {
$toString = "PageInfo{"
+ "__typename=" + __typename + ", "
+ "fragments=" + fragments
+ "}";
}
return $toString;
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof PageInfo) {
PageInfo that = (PageInfo) o;
return this.__typename.equals(that.__typename)
&& this.fragments.equals(that.fragments);
}
return false;
}
@Override
public int hashCode() {
if (!$hashCodeMemoized) {
int h = 1;
h *= 1000003;
h ^= __typename.hashCode();
h *= 1000003;
h ^= fragments.hashCode();
$hashCode = h;
$hashCodeMemoized = true;
}
return $hashCode;
}
public static class Fragments {
final @NotNull PageInfoContent pageInfoContent;
private transient volatile String $toString;
private transient volatile int $hashCode;
private transient volatile boolean $hashCodeMemoized;
public Fragments(@NotNull PageInfoContent pageInfoContent) {
this.pageInfoContent = Utils.checkNotNull(pageInfoContent, "pageInfoContent == null");
}
public @NotNull PageInfoContent pageInfoContent() {
return this.pageInfoContent;
}
public ResponseFieldMarshaller marshaller() {
return new ResponseFieldMarshaller() {
@Override
public void marshal(ResponseWriter writer) {
writer.writeFragment(pageInfoContent.marshaller());
}
};
}
@Override
public String toString() {
if ($toString == null) {
$toString = "Fragments{"
+ "pageInfoContent=" + pageInfoContent
+ "}";
}
return $toString;
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof Fragments) {
Fragments that = (Fragments) o;
return this.pageInfoContent.equals(that.pageInfoContent);
}
return false;
}
@Override
public int hashCode() {
if (!$hashCodeMemoized) {
int h = 1;
h *= 1000003;
h ^= pageInfoContent.hashCode();
$hashCode = h;
$hashCodeMemoized = true;
}
return $hashCode;
}
public static final class Mapper implements ResponseFieldMapper {
static final ResponseField[] $responseFields = {
ResponseField.forFragment("__typename", "__typename", Collections.emptyList())
};
final PageInfoContent.Mapper pageInfoContentFieldMapper = new PageInfoContent.Mapper();
@Override
public @NotNull Fragments map(ResponseReader reader) {
final PageInfoContent pageInfoContent = reader.readFragment($responseFields[0], new ResponseReader.ObjectReader() {
@Override
public PageInfoContent read(ResponseReader reader) {
return pageInfoContentFieldMapper.map(reader);
}
});
return new Fragments(pageInfoContent);
}
}
}
public static final class Mapper implements ResponseFieldMapper {
final Fragments.Mapper fragmentsFieldMapper = new Fragments.Mapper();
@Override
public PageInfo map(ResponseReader reader) {
final String __typename = reader.readString($responseFields[0]);
final Fragments fragments = fragmentsFieldMapper.map(reader);
return new PageInfo(__typename, fragments);
}
}
}
/**
* Webhook exchange
*/
public static class PageItem {
static final ResponseField[] $responseFields = {
ResponseField.forString("__typename", "__typename", null, false, Collections.emptyList()),
ResponseField.forCustomType("uuid", "uuid", null, false, CustomType.UUID, Collections.emptyList()),
ResponseField.forString("webhook", "webhook", null, false, Collections.emptyList()),
ResponseField.forObject("request", "request", null, false, Collections.emptyList()),
ResponseField.forObject("response", "response", null, true, Collections.emptyList()),
ResponseField.forString("stack", "stack", null, true, Collections.emptyList())
};
final @NotNull String __typename;
final @NotNull UUID uuid;
final @NotNull String webhook;
final @NotNull Request request;
final @Nullable Response response;
final @Nullable String stack;
private transient volatile String $toString;
private transient volatile int $hashCode;
private transient volatile boolean $hashCodeMemoized;
public PageItem(@NotNull String __typename, @NotNull UUID uuid, @NotNull String webhook,
@NotNull Request request, @Nullable Response response, @Nullable String stack) {
this.__typename = Utils.checkNotNull(__typename, "__typename == null");
this.uuid = Utils.checkNotNull(uuid, "uuid == null");
this.webhook = Utils.checkNotNull(webhook, "webhook == null");
this.request = Utils.checkNotNull(request, "request == null");
this.response = response;
this.stack = stack;
}
public @NotNull String __typename() {
return this.__typename;
}
/**
* Unique ID for the exchange
*/
public @NotNull UUID uuid() {
return this.uuid;
}
/**
* Webhook name
*/
public @NotNull String webhook() {
return this.webhook;
}
/**
* Request sent to the webhook
*/
public @NotNull Request request() {
return this.request;
}
/**
* Response received from the webhook
*/
public @Nullable Response response() {
return this.response;
}
/**
* Error raised during the exchange
*/
public @Nullable String stack() {
return this.stack;
}
@SuppressWarnings({"rawtypes", "unchecked"})
public ResponseFieldMarshaller marshaller() {
return new ResponseFieldMarshaller() {
@Override
public void marshal(ResponseWriter writer) {
writer.writeString($responseFields[0], __typename);
writer.writeCustom((ResponseField.CustomTypeField) $responseFields[1], uuid);
writer.writeString($responseFields[2], webhook);
writer.writeObject($responseFields[3], request.marshaller());
writer.writeObject($responseFields[4], response != null ? response.marshaller() : null);
writer.writeString($responseFields[5], stack);
}
};
}
@Override
public String toString() {
if ($toString == null) {
$toString = "PageItem{"
+ "__typename=" + __typename + ", "
+ "uuid=" + uuid + ", "
+ "webhook=" + webhook + ", "
+ "request=" + request + ", "
+ "response=" + response + ", "
+ "stack=" + stack
+ "}";
}
return $toString;
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof PageItem) {
PageItem that = (PageItem) o;
return this.__typename.equals(that.__typename)
&& this.uuid.equals(that.uuid)
&& this.webhook.equals(that.webhook)
&& this.request.equals(that.request)
&& ((this.response == null) ? (that.response == null) : this.response.equals(that.response))
&& ((this.stack == null) ? (that.stack == null) : this.stack.equals(that.stack));
}
return false;
}
@Override
public int hashCode() {
if (!$hashCodeMemoized) {
int h = 1;
h *= 1000003;
h ^= __typename.hashCode();
h *= 1000003;
h ^= uuid.hashCode();
h *= 1000003;
h ^= webhook.hashCode();
h *= 1000003;
h ^= request.hashCode();
h *= 1000003;
h ^= (response == null) ? 0 : response.hashCode();
h *= 1000003;
h ^= (stack == null) ? 0 : stack.hashCode();
$hashCode = h;
$hashCodeMemoized = true;
}
return $hashCode;
}
public static final class Mapper implements ResponseFieldMapper {
final Request.Mapper requestFieldMapper = new Request.Mapper();
final Response.Mapper responseFieldMapper = new Response.Mapper();
@Override
public PageItem map(ResponseReader reader) {
final String __typename = reader.readString($responseFields[0]);
final UUID uuid = reader.readCustomType((ResponseField.CustomTypeField) $responseFields[1]);
final String webhook = reader.readString($responseFields[2]);
final Request request = reader.readObject($responseFields[3], new ResponseReader.ObjectReader() {
@Override
public Request read(ResponseReader reader) {
return requestFieldMapper.map(reader);
}
});
final Response response = reader.readObject($responseFields[4], new ResponseReader.ObjectReader() {
@Override
public Response read(ResponseReader reader) {
return responseFieldMapper.map(reader);
}
});
final String stack = reader.readString($responseFields[5]);
return new PageItem(__typename, uuid, webhook, request, response, stack);
}
}
}
/**
* Request sent to the webhook
*/
public static class Request {
static final ResponseField[] $responseFields = {
ResponseField.forString("__typename", "__typename", null, false, Collections.emptyList()),
ResponseField.forCustomType("timestamp", "timestamp", null, false, CustomType.LOCALDATETIME, Collections.emptyList()),
ResponseField.forString("type", "type", null, false, Collections.emptyList()),
ResponseField.forString("payload", "payload", null, false, Collections.emptyList())
};
final @NotNull String __typename;
final @NotNull LocalDateTime timestamp;
final @NotNull String type;
final @NotNull String payload;
private transient volatile String $toString;
private transient volatile int $hashCode;
private transient volatile boolean $hashCodeMemoized;
public Request(@NotNull String __typename, @NotNull LocalDateTime timestamp,
@NotNull String type, @NotNull String payload) {
this.__typename = Utils.checkNotNull(__typename, "__typename == null");
this.timestamp = Utils.checkNotNull(timestamp, "timestamp == null");
this.type = Utils.checkNotNull(type, "type == null");
this.payload = Utils.checkNotNull(payload, "payload == null");
}
public @NotNull String __typename() {
return this.__typename;
}
/**
* Request timestamp
*/
public @NotNull LocalDateTime timestamp() {
return this.timestamp;
}
/**
* Type of payload
*/
public @NotNull String type() {
return this.type;
}
/**
* Payload sent to the webhook
*/
public @NotNull String payload() {
return this.payload;
}
@SuppressWarnings({"rawtypes", "unchecked"})
public ResponseFieldMarshaller marshaller() {
return new ResponseFieldMarshaller() {
@Override
public void marshal(ResponseWriter writer) {
writer.writeString($responseFields[0], __typename);
writer.writeCustom((ResponseField.CustomTypeField) $responseFields[1], timestamp);
writer.writeString($responseFields[2], type);
writer.writeString($responseFields[3], payload);
}
};
}
@Override
public String toString() {
if ($toString == null) {
$toString = "Request{"
+ "__typename=" + __typename + ", "
+ "timestamp=" + timestamp + ", "
+ "type=" + type + ", "
+ "payload=" + payload
+ "}";
}
return $toString;
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof Request) {
Request that = (Request) o;
return this.__typename.equals(that.__typename)
&& this.timestamp.equals(that.timestamp)
&& this.type.equals(that.type)
&& this.payload.equals(that.payload);
}
return false;
}
@Override
public int hashCode() {
if (!$hashCodeMemoized) {
int h = 1;
h *= 1000003;
h ^= __typename.hashCode();
h *= 1000003;
h ^= timestamp.hashCode();
h *= 1000003;
h ^= type.hashCode();
h *= 1000003;
h ^= payload.hashCode();
$hashCode = h;
$hashCodeMemoized = true;
}
return $hashCode;
}
public static final class Mapper implements ResponseFieldMapper {
@Override
public Request map(ResponseReader reader) {
final String __typename = reader.readString($responseFields[0]);
final LocalDateTime timestamp = reader.readCustomType((ResponseField.CustomTypeField) $responseFields[1]);
final String type = reader.readString($responseFields[2]);
final String payload = reader.readString($responseFields[3]);
return new Request(__typename, timestamp, type, payload);
}
}
}
/**
* Response received from the webhook
*/
public static class Response {
static final ResponseField[] $responseFields = {
ResponseField.forString("__typename", "__typename", null, false, Collections.emptyList()),
ResponseField.forCustomType("timestamp", "timestamp", null, false, CustomType.LOCALDATETIME, Collections.emptyList()),
ResponseField.forInt("code", "code", null, false, Collections.emptyList()),
ResponseField.forString("payload", "payload", null, false, Collections.emptyList())
};
final @NotNull String __typename;
final @NotNull LocalDateTime timestamp;
final int code;
final @NotNull String payload;
private transient volatile String $toString;
private transient volatile int $hashCode;
private transient volatile boolean $hashCodeMemoized;
public Response(@NotNull String __typename, @NotNull LocalDateTime timestamp, int code,
@NotNull String payload) {
this.__typename = Utils.checkNotNull(__typename, "__typename == null");
this.timestamp = Utils.checkNotNull(timestamp, "timestamp == null");
this.code = code;
this.payload = Utils.checkNotNull(payload, "payload == null");
}
public @NotNull String __typename() {
return this.__typename;
}
/**
* Response timestamp
*/
public @NotNull LocalDateTime timestamp() {
return this.timestamp;
}
/**
* Response HTTP status code
*/
public int code() {
return this.code;
}
/**
* Response payload
*/
public @NotNull String payload() {
return this.payload;
}
@SuppressWarnings({"rawtypes", "unchecked"})
public ResponseFieldMarshaller marshaller() {
return new ResponseFieldMarshaller() {
@Override
public void marshal(ResponseWriter writer) {
writer.writeString($responseFields[0], __typename);
writer.writeCustom((ResponseField.CustomTypeField) $responseFields[1], timestamp);
writer.writeInt($responseFields[2], code);
writer.writeString($responseFields[3], payload);
}
};
}
@Override
public String toString() {
if ($toString == null) {
$toString = "Response{"
+ "__typename=" + __typename + ", "
+ "timestamp=" + timestamp + ", "
+ "code=" + code + ", "
+ "payload=" + payload
+ "}";
}
return $toString;
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof Response) {
Response that = (Response) o;
return this.__typename.equals(that.__typename)
&& this.timestamp.equals(that.timestamp)
&& this.code == that.code
&& this.payload.equals(that.payload);
}
return false;
}
@Override
public int hashCode() {
if (!$hashCodeMemoized) {
int h = 1;
h *= 1000003;
h ^= __typename.hashCode();
h *= 1000003;
h ^= timestamp.hashCode();
h *= 1000003;
h ^= code;
h *= 1000003;
h ^= payload.hashCode();
$hashCode = h;
$hashCodeMemoized = true;
}
return $hashCode;
}
public static final class Mapper implements ResponseFieldMapper {
@Override
public Response map(ResponseReader reader) {
final String __typename = reader.readString($responseFields[0]);
final LocalDateTime timestamp = reader.readCustomType((ResponseField.CustomTypeField) $responseFields[1]);
final int code = reader.readInt($responseFields[2]);
final String payload = reader.readString($responseFields[3]);
return new Response(__typename, timestamp, code, payload);
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy