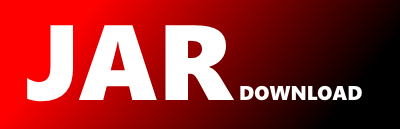
net.nemerosa.ontrack.model.security.RolesService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ontrack-model Show documentation
Show all versions of ontrack-model Show documentation
Ontrack module: ontrack-model
package net.nemerosa.ontrack.model.security;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
/**
* Management of roles and functions.
*
* @see net.nemerosa.ontrack.model.security.GlobalRole
* @see net.nemerosa.ontrack.model.security.ProjectRole
* @see net.nemerosa.ontrack.model.security.GlobalFunction
* @see net.nemerosa.ontrack.model.security.ProjectFunction
*/
public interface RolesService {
/**
* List of all global functions.
*/
List> defaultGlobalFunctions = Arrays.asList(
ProjectCreation.class,
ApplicationManagement.class,
GlobalSettings.class,
AccountManagement.class,
AccountGroupManagement.class,
ProjectList.class
);
/**
* List of read-only global functions.
*/
List> readOnlyGlobalFunctions = Collections.singletonList(
ProjectList.class
);
/**
* List of all project functions.
*/
List> defaultProjectFunctions = Arrays.asList(
ProjectView.class,
ProjectEdit.class,
ProjectAuthorisationMgt.class,
ProjectConfig.class,
ProjectDelete.class,
BranchCreate.class,
BranchEdit.class,
BranchFilterMgt.class,
BranchTemplateMgt.class,
BranchDelete.class,
PromotionLevelCreate.class,
PromotionLevelEdit.class,
PromotionLevelDelete.class,
ValidationStampCreate.class,
ValidationStampEdit.class,
ValidationStampDelete.class,
BuildCreate.class,
BuildConfig.class,
BuildEdit.class,
BuildDelete.class,
ValidationRunCreate.class,
ValidationRunStatusChange.class,
PromotionRunCreate.class,
PromotionRunDelete.class
);
/**
* List of read-only project functions.
*/
List> readOnlyProjectFunctions = Collections.singletonList(
ProjectView.class
);
/**
* List of global roles.
*/
List getGlobalRoles();
/**
* Gets a global role by its identifier
*/
Optional getGlobalRole(String id);
/**
* List of project roles
*/
List getProjectRoles();
/**
* Gets a project role by its identifier
*/
Optional getProjectRole(String id);
/**
* List of all global functions
*/
List> getGlobalFunctions();
/**
* List of all project functions
*/
List> getProjectFunctions();
/**
* Gets a project/role association
*
* @param project Project ID
* @param roleId Role name
* @return Project/role association or {@linkplain java.util.Optional#empty() empty} if the role
* does not exist
*/
Optional getProjectRoleAssociation(int project, String roleId);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy