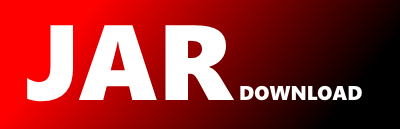
net.nemerosa.ontrack.model.support.RegexMessageAnnotator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ontrack-model Show documentation
Show all versions of ontrack-model Show documentation
Ontrack module: ontrack-model
package net.nemerosa.ontrack.model.support;
import java.util.ArrayList;
import java.util.Collection;
import java.util.function.Function;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class RegexMessageAnnotator extends AbstractMessageAnnotator {
private final Pattern pattern;
private final Function annotationFactory;
public RegexMessageAnnotator(String pattern, Function annotationFactory) {
this(Pattern.compile(pattern), annotationFactory);
}
public RegexMessageAnnotator(Pattern pattern, Function annotationFactory) {
this.pattern = pattern;
this.annotationFactory = annotationFactory;
}
@Override
public Collection annotate(String text) {
Collection annotations = new ArrayList<>();
int start = 0;
Matcher m = pattern.matcher(text);
while (m.find()) {
int mStart = m.start();
int mEnd = m.end();
// Previous section
if (mStart > start) {
String previous = text.substring(start, mStart);
annotations.add(MessageAnnotation.t(previous));
}
// Match
String match = m.group();
MessageAnnotation annotation = annotationFactory.apply(match);
annotations.add(annotation);
// Next
start = mEnd;
}
// End
if (start < text.length() - 1) {
String reminder = text.substring(start);
annotations.add(MessageAnnotation.t(reminder));
}
// OK
return annotations;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy