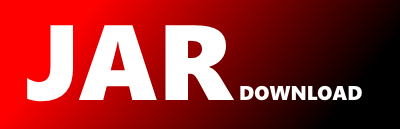
net.nemerosa.ontrack.model.security.SecurityService.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ontrack-model Show documentation
Show all versions of ontrack-model Show documentation
Ontrack module: ontrack-model
package net.nemerosa.ontrack.model.security
import net.nemerosa.ontrack.model.structure.ProjectEntity
import net.nemerosa.ontrack.model.structure.Signature
import java.util.*
import kotlin.reflect.KClass
interface SecurityService {
/**
* Checks that the current user is authenticated
*/
fun checkAuthenticated()
fun checkGlobalFunction(fn: Class)
fun isGlobalFunctionGranted(fn: Class): Boolean
fun checkProjectFunction(projectId: Int, fn: Class)
fun checkProjectFunction(entity: ProjectEntity, fn: Class) {
checkProjectFunction(entity.projectId(), fn)
}
fun isProjectFunctionGranted(projectId: Int, fn: Class): Boolean
fun isProjectFunctionGranted(entity: ProjectEntity, fn: Class): Boolean {
return isProjectFunctionGranted(entity.projectId(), fn)
}
/**
* List of [project functions][ProjectFunction] which are automatically assigned to authenticated users.
*/
val autoProjectFunctions: Set>
/**
* Returns the current logged account or `null` if none is logged.
*/
val currentAccount: OntrackAuthenticatedUser?
/**
* Is the current user logged?
*/
val isLogged: Boolean
get() = currentAccount != null
/**
* Returns the current logged account as an option
*
*/
@get:Deprecated("Use getCurrentAccount directly and check for null")
val account: Optional
get() = Optional.ofNullable(currentAccount)
val currentSignature: Signature
/**
* Performs a call as admin. This is mainly by internal code to access parts of the application
* which are usually protected from the current context, but that need to be accessed locally.
*
* @param supplier Call to perform in a protected context
* @param Type of data to get back
* @return A new supplier running in a new security context
*/
fun runAsAdmin(supplier: () -> T): () -> T
fun asAdmin(supplier: () -> T): T
// For Java compatibility
fun asAdmin(code: Runnable): Unit = asAdmin {
code.run()
}
/**
* In some asynchronous operations, we need to run a task with the same credentials that initiated the operation.
* This method creates a wrapping supplier that holds the initial security context.
*
* @param fn Call to perform in a protected context
* @param Type of data to get back
* @return A new function running in a new security context
*/
fun runner(fn: (T) -> R): (T) -> R
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy