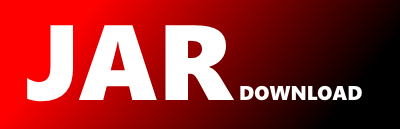
net.nemerosa.ontrack.model.structure.PropertyService.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ontrack-model Show documentation
Show all versions of ontrack-model Show documentation
Ontrack module: ontrack-model
package net.nemerosa.ontrack.model.structure
import com.fasterxml.jackson.databind.JsonNode
import net.nemerosa.ontrack.model.Ack
import net.nemerosa.ontrack.model.exceptions.PropertyTypeNotFoundException
import net.nemerosa.ontrack.model.form.Form
import java.util.function.BiFunction
import java.util.function.Function
import java.util.function.Predicate
import kotlin.reflect.KClass
/**
* Management of properties.
*/
interface PropertyService {
/**
* List of all property types
*/
val propertyTypes: List>
/**
* Gets a property type using its name
*
* @param propertyTypeName Fully qualified name of the property type
* @param Type of property
* @return Property type
* @throws PropertyTypeNotFoundException If not found
*/
@Throws(PropertyTypeNotFoundException::class)
fun getPropertyTypeByName(propertyTypeName: String): PropertyType
/**
* List of property values for a given entity and for the current user.
*
* @param entity Entity
* @return List of properties for this entity
*/
fun getProperties(entity: ProjectEntity): List>
/**
* Gets the edition form for a given property for an entity. The content of the form may be filled or not,
* according to the fact if the property is actually set for this entity or not. If the property is not
* opened for edition, the call could be rejected with an authorization exception.
*
* @param entity Entity to get the edition form for
* @param propertyTypeName Fully qualified name of the property to get the form for
* @return An edition form to be used by the client
*/
fun getPropertyEditionForm(entity: ProjectEntity, propertyTypeName: String): Form
/**
* Gets the value for a given property for an entity. If the property is not set, a non-null
* [net.nemerosa.ontrack.model.structure.Property] is returned but is marked as
* [empty][net.nemerosa.ontrack.model.structure.Property.isEmpty].
* If the property is not opened for viewing, the call could be rejected with an
* authorization exception.
*
* @param entity Entity to get the edition form for
* @param propertyTypeName Fully qualified name of the property to get the property for
* @return A response that defines the property
*/
fun getProperty(entity: ProjectEntity, propertyTypeName: String): Property
/**
* Same than [.getProperty] but using the class of
* the property type.
*
* @param entity Entity to get the edition form for
* @param propertyTypeClass Class of the property type to get the property for
* @return A response that defines the property
*/
fun getProperty(entity: ProjectEntity, propertyTypeClass: Class>): Property
/**
* Edits the value of a property.
*
* @param entity Entity to edit
* @param propertyTypeName Fully qualified name of the property to edit
* @param data Raw JSON data for the property value
*/
fun editProperty(entity: ProjectEntity, propertyTypeName: String, data: JsonNode): Ack
/**
* Edits the value of a property.
*
* @param entity Entity to edit
* @param propertyType The type of the property to edit
* @param data Property value
*/
fun editProperty(entity: ProjectEntity, propertyType: Class>, data: T): Ack
/**
* Deletes the value of a property.
*
* @param entity Type of the entity to edit
* @param propertyTypeName Fully qualified name of the property to delete
*/
fun deleteProperty(entity: ProjectEntity, propertyTypeName: String): Ack
/**
* Deletes the value of a property.
*
* @param entity Type of the entity to edit
* @param propertyType Class of the property to delete
*/
fun deleteProperty(entity: ProjectEntity, propertyType: Class>): Ack =
deleteProperty(entity, propertyType.name)
/**
* Searches for all entities with the corresponding property value.
*/
fun searchWithPropertyValue(
propertyTypeClass: Class>,
entityLoader: BiFunction,
predicate: Predicate
): Collection
/**
* Finds an item using its search key.
*/
fun findBuildByBranchAndSearchkey(branchId: ID, propertyType: Class>, searchKey: String): ID?
/**
* Finds a list of entities based on their type, a property and a search key.
*/
fun findByEntityTypeAndSearchkey(entityType: ProjectEntityType, propertyType: Class>, searchKey: String): List
/**
* Tests if a property is defined.
*/
fun hasProperty(entity: ProjectEntity, propertyTypeClass: Class>): Boolean
/**
* Copy/clones the `property` into the `targetEntity` after applying the replacement function.
*
* @param sourceEntity Owner of the property to copy
* @param property Property to copy
* @param targetEntity Entity to associate the new property with
* @param replacementFn Replacement function for textual values
* @param Type of the property
*/
fun copyProperty(sourceEntity: ProjectEntity, property: Property, targetEntity: ProjectEntity, replacementFn: Function)
/**
* Loops over all the properties of a given type.
*/
fun forEachEntityWithProperty(propertyTypeClass: KClass>, consumer: (ProjectEntityID, T) -> Unit)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy