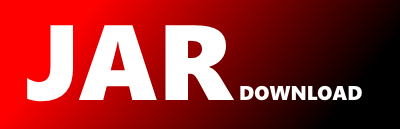
net.nemerosa.ontrack.model.structure.Property Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ontrack-model Show documentation
Show all versions of ontrack-model Show documentation
Ontrack module: ontrack-model
package net.nemerosa.ontrack.model.structure;
import com.fasterxml.jackson.annotation.JsonIgnore;
import lombok.AccessLevel;
import lombok.AllArgsConstructor;
import lombok.Data;
import java.util.Collections;
import java.util.Map;
import java.util.Optional;
/**
* Property value, associated with its type.
*/
@Data
@AllArgsConstructor(access = AccessLevel.PROTECTED)
public class Property {
/**
* Type for this property
*/
@JsonIgnore
private final PropertyType type;
/**
* Value for this property
*/
private final T value;
/**
* Editable status
*/
private final boolean editable;
/**
* Additional decorations
*/
private final Map decorations;
/**
* Descriptor for the property type
*/
public PropertyTypeDescriptor getTypeDescriptor() {
return PropertyTypeDescriptor.of(type);
}
/**
* Empty indicator
*/
public boolean isEmpty() {
return value == null;
}
/**
* List of additional decorations for the property
*/
public Map getDecorations() {
return decorations;
}
/**
* As an option
*/
public Optional option() {
return Optional.ofNullable(value);
}
/**
* Editable property
*/
public Property editable(boolean editable) {
return new Property<>(type, value, editable, decorations);
}
public static Property empty(PropertyType type) {
return new Property<>(type, null, false, Collections.emptyMap());
}
public static Property of(PropertyType type, T value) {
return new Property<>(type, value, false, Collections.emptyMap());
}
public static Property of(PropertyType type, T value, Map decorations) {
return new Property<>(type, value, false, decorations);
}
public boolean containsValue(String propertyValue) {
return value != null && type.containsValue(value, propertyValue);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy