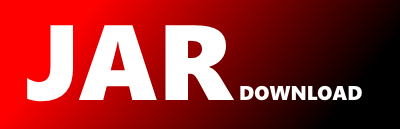
net.nemerosa.ontrack.model.structure.SearchIndexService.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ontrack-model Show documentation
Show all versions of ontrack-model Show documentation
Ontrack module: ontrack-model
package net.nemerosa.ontrack.model.structure
import com.fasterxml.jackson.databind.JsonNode
import net.nemerosa.ontrack.model.search.SearchQuery
/**
* This service is used to manage the search indexes when they are available.
*/
interface SearchIndexService {
/**
* Are search indexes available?
*/
val searchIndexesAvailable: Boolean
fun initIndex(indexer: SearchIndexer)
fun index(indexer: SearchIndexer)
fun resetIndex(indexer: SearchIndexer, reindex: Boolean): Boolean
fun createSearchIndex(indexer: SearchIndexer, item: T)
fun updateSearchIndex(indexer: SearchIndexer, item: T)
fun deleteSearchIndex(indexer: SearchIndexer, id: String)
fun batchSearchIndex(indexer: SearchIndexer, items: Collection, mode: BatchIndexMode): BatchIndexResults
fun query(indexer: SearchIndexer, size: Int, query: SearchQuery, handler: (source: JsonNode) -> Unit)
}
/**
* Batch indexing mode
*/
enum class BatchIndexMode {
/**
* If ID already exists, leaves as-is
*/
KEEP,
/**
* If ID already exists, replaces it
*/
UPDATE
}
/**
* Results of batch indexing
*/
data class BatchIndexResults(
val added: Int,
val updated: Int,
val kept: Int,
val deleted: Int
) {
companion object {
val NONE = BatchIndexResults(added = 0, updated = 0, kept = 0, deleted = 0)
val KEEP = BatchIndexResults(added = 0, updated = 0, kept = 1, deleted = 0)
val UPDATE = BatchIndexResults(added = 0, updated = 1, kept = 0, deleted = 0)
val ADD = BatchIndexResults(added = 1, updated = 0, kept = 0, deleted = 0)
}
operator fun plus(other: BatchIndexResults) =
BatchIndexResults(
added = added + other.added,
updated = updated + other.updated,
kept = kept + other.kept,
deleted = deleted + other.deleted
)
}
/**
* Utility extension accepting any kind of ID.
*/
fun SearchIndexService.deleteSearchIndex(indexer: SearchIndexer, id: Any) =
deleteSearchIndex(indexer, id.toString())
© 2015 - 2025 Weber Informatics LLC | Privacy Policy