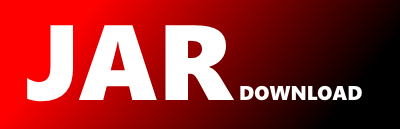
net.minecraftforge.gdi.annotations.DSLProperty.groovy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of groovydslimprover Show documentation
Show all versions of groovydslimprover Show documentation
Groovy Compiler Plugin to improve building Groovy based DSLs, like those used in Gradle.
The newest version!
/*
* Copyright (c) Forge Development LLC and contributors
* SPDX-License-Identifier: LGPL-2.1-only
*/
package net.minecraftforge.gdi.annotations
import net.minecraftforge.gdi.transformer.DSLPropertyTransformer
import org.codehaus.groovy.transform.GroovyASTTransformationClass
import org.gradle.api.Action
import org.gradle.api.NamedDomainObjectContainer
import org.gradle.api.Project
import org.gradle.api.file.*
import org.gradle.api.provider.*
import java.lang.annotation.*
/**
* Annotate an abstract method of a groovy interface in order to generate DSL methods for the property.
* Methods with {@link Action} or {@link Closure} parameters will be generated only if the property
* {@link DSLProperty#isConfigurable() is configurable} (which needs to be explicitly declared) or if its type implements {@link org.gradle.util.Configurable}.
* This annotation will generate the following methods based on the return type of the method:
*
*
* Property Type
* Generated Methods
*
*
* {@link Property}
*
*
* - {@code $propertyName(T)}
* - {@code $propertyName(Action
)} - the action will be executed on the provider's value, and if it doesn't have a value, one will be created using the {@linkplain DSLProperty#factory() factory}
* - {@code $propertyName(@DelegatesTo(T.class) Closure
)} - same behaviour as the action
* - {@code $propertyName(T, Action
)} - the action will be executed on the given value, which will be then {@linkplain org.gradle.api.provider.Property#set(java.lang.Object) set} as the provider's value
* - {@code $propertyName(T, @DelegatesTo(T.class) Closure
)} - same behaviour as the action
*
*
*
*
* any {@link HasMultipleValues} ({@link ListProperty}, {@link SetProperty})
*
*
* - {@code $singularName(T)} - the given value will be added to the list
* - {@code $propertyName(T...)} - the given values will be added to the list
* - {@code $propertyName(Iterable extends T>)} - the given values will be added to the list
* - {@code $singularName(T, Action
)} - the action will be executed on the given value, which will be then added to the list
* - {@code $singularName(T, @DelegatesTo(T.class) Closure
)} - same behaviour as the action
*
* The methods below are generated only if a {@linkplain DSLProperty#factory() factory} is supplied:
*
* - {@code $singularName(Action
)} - the action will be executed on an object created with the factory, which will be then added to the list
* - {@code $singularName(@DelegatesTo(T.class) Closure
)} - same behaviour as the action
*
*
*
*
* {@link MapProperty}
*
*
* - {@code $singularName(K, V)} - the given value will be added to the map, at the given key
* - {@code $singularName(K, V, Action
)} - the action will be executed on the given value, which will be then added to the map at the given key
* - {@code $singularName(K, V, @DelegatesTo(V.class) Closure
)} - same behaviour as the action
* - {@code $propertyName(Map
)} - calls {@link MapProperty#putAll(java.util.Map)}
* - {@code $propertyName(CommonAncestor...)} - computes a key-value mapping from the vararg array (expecting an array such as [k1, v1, k2, v2, ...], with an even number of elements, and of the correct key and value types), and calls {@link MapProperty#put(java.lang.Object, java.lang.Object)} with each pair; the array type is determined by the first common type in the inheritance tree of both the key and the value, or {@link Object} otherwise
*
* The methods below are generated only if a {@linkplain DSLProperty#factory() factory} is supplied:
*
* - {@code $singularName(K, Action
)} - the action will be executed on an object created with the factory, which will be then added to the map at the given key
* - {@code $singularName(K, @DelegatesTo(V.class) Closure
)} - same behaviour as the action
*
*
*
*
* {@link NamedDomainObjectContainer}
*
*
* - {@code $singularName(String, Action
)} - calls {@link NamedDomainObjectContainer#register(java.lang.String, Action)}
* - {@code $singularName(K, @DelegatesTo(V.class) Closure
)} - calls {@link NamedDomainObjectContainer#register(java.lang.String, Action)}
* - {@code $propertyName(@DelegatesTo(NamedDomainObjectContainer.class) Closure
)} - calls {@link NamedDomainObjectContainer#register(java.lang.String, Action)}
*
*
*
*
*
* {@link DirectoryProperty} - requires a {@link ProjectGetter}
*
*
* - {@code $propertyName(Directory)} - calls {@link DirectoryProperty#value(Directory)}
* - {@code $propertyName(File)} - calls {@link DirectoryProperty#set(java.io.File)}
* - {@code $propertyName(Object)} - calls {@link DirectoryProperty#set(java.io.File)} with a file got using {@link Project#file(java.lang.Object)}
*
*
*
*
* {@link RegularFileProperty} - requires a {@link ProjectGetter}
*
*
* - {@code $propertyName(RegularFile)} - calls {@link RegularFileProperty#value(RegularFile)}
* - {@code $propertyName(File)} - calls {@link RegularFileProperty#set(java.io.File)}
* - {@code $propertyName(Object)} - calls {@link RegularFileProperty#set(java.io.File)} with a file got using {@link Project#file(java.lang.Object)}
*
*
*
*
* {@link ConfigurableFileCollection}
*
*
* - {@code $singularName(Object)} - calls {@link ConfigurableFileCollection#from(java.lang.Object ...)}
* - {@code $propertyName(Object...)} - calls {@link ConfigurableFileCollection#from(java.lang.Object ...)}
*
*
*
*
*/
@Documented
@Target(ElementType.METHOD)
@Retention(RetentionPolicy.CLASS)
@GroovyASTTransformationClass(classes = DSLPropertyTransformer)
@interface DSLProperty {
/**
* The name of the property. If not specified this will be the method name without
* {@code get} and {@link org.codehaus.groovy.runtime.StringGroovyMethods#uncapitalize(java.lang.CharSequence) uncapitalized}.
*/
String propertyName() default ''
/**
* The singular name of the property, used by map or collection methods adding a single element.
* If not specified it will be computed from the {@link #propertyName()} using {@link net.minecraftforge.gdi.transformer.Unpluralizer#unpluralize(java.lang.String)}.
*/
String singularName() default ''
/**
* The factory used to create instances of the property type, when applicable.
*/
Class factory() default Closure.class
/**
* If this property is configurable.
* While the default value is {@code true}, it will only be considered when it is explicitly defined.
*/
boolean isConfigurable() default true
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy