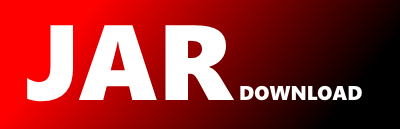
net.nitrado.api.order.PartPricing Maven / Gradle / Ivy
package net.nitrado.api.order;
import net.nitrado.api.Nitrapi;
import net.nitrado.api.common.exceptions.NitrapiErrorException;
import net.nitrado.api.common.http.Parameter;
import java.util.HashMap;
import java.util.Map;
public class PartPricing extends Pricing {
HashMap parts = new HashMap();
public PartPricing(Nitrapi nitrapi, int locationId) {
super(nitrapi, locationId);
}
public void addPart(String part, int value) {
parts.put(part, value);
}
public Part[] getParts() {
return getPrices().getParts();
}
@Override
public int getPrice(int service, int rentalTime) {
PriceList prices = getPrices(service);
int totalPrice = 0;
for (Part part : prices.getParts()) {
if (!parts.containsKey(part.getType())) { throw new NitrapiErrorException("No amount selected for " + part.getType()); }
int amount = parts.get(part.getType());
if (amount < part.getMinCount()) { throw new NitrapiErrorException("The amount " + amount + " of type " + part.getType() + " is too small."); }
if (amount > part.getMaxCount()) { throw new NitrapiErrorException("The amount " + amount + " of type " + part.getType() + " is too big."); }
int localPrice = -1;
for ( PartRentalOption rentalOption :part.getRentalTimes()) {
if (rentalOption.getHours() == rentalTime) {
for (Price price:rentalOption.getPrices()) {
if (price.getCount() == amount) {
localPrice = price.getPrice();
break;
}
}
}
if (localPrice != -1) { break; }
}
if (localPrice == -1) {
throw new NitrapiErrorException("No valid price found for part " + part.getType());
}
totalPrice += localPrice;
}
totalPrice -=prices.getAdvice();
return totalPrice;
}
@Override
public void orderService(int rentalTime) {
int ADD_PARAMS = 3;
Part[] priceParts = getParts();
Parameter[] parameters = new Parameter[priceParts.length + additionals.size()+ ADD_PARAMS];
parameters[0] = new Parameter("price", getPrice(rentalTime));
parameters[1] = new Parameter("rental_time", rentalTime);
parameters[2] = new Parameter("location", locationId);
int j = ADD_PARAMS;
for (int i = 0; i < priceParts.length; i++) {
Part part = getParts()[i];
parameters[j] = new Parameter("parts["+part.getName()+"]", parts.get(part.getName()));
j++;
}
for (Map.Entry entry : additionals.entrySet()) {
parameters[j] = new Parameter("additionals[" + entry.getKey() + "]", entry.getValue());
j++;
}
nitrapi.dataPost("order/order/"+product, parameters);
}
@Override
public void switchService(int service, int rentalTime) {
int ADD_PARAMS = 5;
Part[] priceParts = getParts();
Parameter[] parameters = new Parameter[priceParts.length + additionals.size()+ ADD_PARAMS];
parameters[0] = new Parameter("price", getSwitchPrice(service, rentalTime));
parameters[1] = new Parameter("rental_time", rentalTime);
parameters[2] = new Parameter("location", locationId);
parameters[3] = new Parameter("method", "switch");
parameters[4] = new Parameter("service_id", service);
int j = ADD_PARAMS;
for (int i = 0; i < priceParts.length; i++) {
Part part = getParts()[i];
parameters[j] = new Parameter("parts["+part.getName()+"]", parts.get(part.getName()));
j++;
}
for (Map.Entry entry : additionals.entrySet()) {
parameters[j] = new Parameter("additionals[" + entry.getKey() + "]", entry.getValue());
j++;
}
nitrapi.dataPost("order/order/"+product, parameters);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy