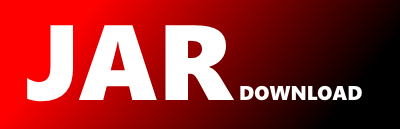
net.objectlab.kit.datecalc.common.ImmutableHolidayCalendar Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of datecalc-common Show documentation
Show all versions of datecalc-common Show documentation
Common Date Calculator Code
/*
* $Id: org.eclipse.jdt.ui.prefs 138 2006-09-10 12:29:15Z marchy $
*
* Copyright 2006 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package net.objectlab.kit.datecalc.common;
import java.util.Set;
/**
* This is an immutable holiday calendar, once given to a DateCalculator, a HolidayCalendar cannot be
* modified, it will throw {@link UnsupportedOperationException}.
*
* @author Benoit Xhenseval
* @author $LastChangedBy: benoitx $
* @version $Revision: 200 $ $Date: 2006-10-10 21:15:58 +0100 (Tue, 10 Oct 2006) $
* @since 1.1.0
*/
public class ImmutableHolidayCalendar implements HolidayCalendar {
private static final long serialVersionUID = 1287613980146071460L;
private HolidayCalendar delegate;
public ImmutableHolidayCalendar(final HolidayCalendar delegate) {
super();
this.delegate = delegate;
}
/**
* @return the early (start) boundary of the holiday range
* @see net.objectlab.kit.datecalc.common.HolidayCalendar#getEarlyBoundary()
*/
public E getEarlyBoundary() {
return delegate.getEarlyBoundary();
}
/**
* @return the set of holidays
* @see net.objectlab.kit.datecalc.common.HolidayCalendar#getHolidays()
*/
public Set getHolidays() {
return delegate.getHolidays();
}
/**
* @return the late (end) boundary of the holiday range
* @see net.objectlab.kit.datecalc.common.HolidayCalendar#getLateBoundary()
*/
public E getLateBoundary() {
return delegate.getLateBoundary();
}
/**
* @param earlyBoundary
* @see net.objectlab.kit.datecalc.common.HolidayCalendar#setEarlyBoundary(java.lang.Object)
* @throws UnsupportedOperationException You cannot modify the early boundary, you need to use a new HolidayCalendar.
*/
public void setEarlyBoundary(final E earlyBoundary) {
throw new UnsupportedOperationException("You cannot modify the early boundary, you need to use a new HolidayCalendar.");
}
/**
* @param holidays
* @see net.objectlab.kit.datecalc.common.HolidayCalendar#setHolidays(java.util.Set)
* @throws UnsupportedOperationException("You cannot modify the holidays, you need to use a new HolidayCalendar.");
*/
public void setHolidays(final Set holidays) {
throw new UnsupportedOperationException("You cannot modify the holidays, you need to use a new HolidayCalendar.");
}
/**
* @param lateBoundary
* @see net.objectlab.kit.datecalc.common.HolidayCalendar#setLateBoundary(java.lang.Object)
* @throws UnsupportedOperationException You cannot modify the late boundary, you need to use a new HolidayCalendar.
*/
public void setLateBoundary(final E lateBoundary) {
throw new UnsupportedOperationException("You cannot modify the early boundary, you need to use a new HolidayCalendar.");
}
/**
* @param date
* @return
* @see net.objectlab.kit.datecalc.common.HolidayCalendar#isHoliday(java.lang.Object)
*/
public boolean isHoliday(final E date) {
return delegate.isHoliday(date);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy