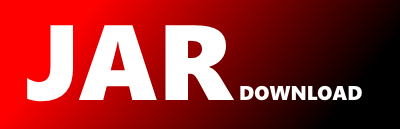
net.objectlab.kit.collections.DefaultMapBuilder Maven / Gradle / Ivy
/**
*
*/
package net.objectlab.kit.collections;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import net.objectlab.kit.util.Pair;
/**
* Inspired by the Google Collection builder.
*
* @author Benoit Xhenseval
*
*/
public class DefaultMapBuilder implements MapBuilder {
private final List> entries = new ArrayList>();
private final String id;
public DefaultMapBuilder(final String id) {
this.id = id;
}
@Override
public String getId() {
return id;
}
/**
* Associates {@code key} with {@code value} in the built map.
*/
@Override
public DefaultMapBuilder put(final K key, final V value) {
entries.add(new Pair(key, value));
return this;
}
/**
* Associates all of the given map's keys and values in the built map.
*
* @throws NullPointerException if any key or value in {@code map} is null
*/
@Override
public DefaultMapBuilder putAll(final Map extends K, ? extends V> map) {
for (final Entry extends K, ? extends V> entry : map.entrySet()) {
put(entry.getKey(), entry.getValue());
}
return this;
}
/**
* Returns a newly-created immutable map.
*
* @throws IllegalArgumentException if duplicate keys were added
* @return
*/
public Map build() {
return fromEntryList(entries);
}
private static Map fromEntryList(final List> entries) {
final int size = entries.size();
if (size == 0) {
return new HashMap();
}
final Map m = new HashMap();
for (final Pair entry : entries) {
m.put(entry.getElement1(), entry.getElement2());
}
return m;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy