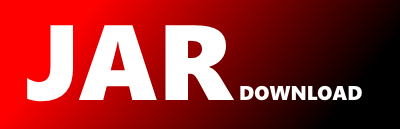
net.objectlab.qalab.m2.BuildStatChartMojo Maven / Gradle / Ivy
The newest version!
////////////////////////////////////////////////////////////////////////////////
//
// ObjectLab is sponsoring QALab
//
// Based in London, we are world leaders in the design and development
// of bespoke applications for the Securities Financing markets.
//
// Click here to learn more
// ___ _ _ _ _ _
// / _ \| |__ (_) ___ ___| |_| | __ _| |__
// | | | | '_ \| |/ _ \/ __| __| | / _` | '_ \
// | |_| | |_) | | __/ (__| |_| |__| (_| | |_) |
// \___/|_.__// |\___|\___|\__|_____\__,_|_.__/
// |__/
//
// http://www.ObjectLab.co.uk
// ---------------------------------------------------------------------------
//
//QALab is released under the GNU General Public License.
//
//QALab: Collects QA Statistics from your build over time.
//2005+, ObjectLab Ltd
//
//This library is free software; you can redistribute it and/or
//modify it under the terms of the GNU General Public
//License as published by the Free Software Foundation; either
//version 2.1 of the License, or (at your option) any later version.
//
//This library is distributed in the hope that it will be useful,
//but WITHOUT ANY WARRANTY; without even the implied warranty of
//MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
//General Public License for more details.
//
//You should have received a copy of the GNU General Public
//License along with this library; if not, write to the Free Software
//Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
//
////////////////////////////////////////////////////////////////////////////////
package net.objectlab.qalab.m2;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
import javax.xml.parsers.ParserConfigurationException;
import javax.xml.parsers.SAXParser;
import javax.xml.parsers.SAXParserFactory;
import net.objectlab.qalab.m2.util.Maven2TaskLogger;
import net.objectlab.qalab.m2.util.Utils;
import net.objectlab.qalab.parser.BuildStatForChartParser;
import org.apache.maven.plugin.AbstractMojo;
import org.apache.maven.plugin.MojoExecutionException;
import org.xml.sax.InputSource;
import org.xml.sax.SAXException;
/**
* Goal which generates the QALab BuildStat chart.
*
* @author Dave Sag.
* @goal chart
* @phase site
*/
public class BuildStatChartMojo extends AbstractMojo {
// ~ Static fields/initializers
// ------------------------------------------------------------------------
/** Default SAX parser. * */
// private static final String DEFAULT_PARSER_NAME = "org.apache.xerces.parsers.SAXParser";
/** Default Chart width. * */
private static final int DEFAULT_WIDTH = 750;
/** Default Chart height. * */
private static final int DEFAULT_HEIGHT = 500;
// ~ Instance fields -------------------------------------------------------
/**
* The QALab properties file.
*
* @parameter expression="${project.basedir}/qalab.xml"
* @required
*/
private File qalabFile = null;
/**
* The directory to put the chart.
*
* @parameter expression="${project.reporting.outputDirectory}/qalab"
* @required
*/
private File toDir = null;
/**
* If true then any debug logging output will be suppressed.
*
* @parameter default-value=false
*/
private boolean quiet = false;
/**
* The Chart width.
*
* @parameter default-value=750
*/
private int width = DEFAULT_WIDTH;
/**
* The Chart height.
*
* @parameter default-value=500
*/
private int height = DEFAULT_HEIGHT;
/**
* If the movingAverage is <= 0 then there is no moving average, otherwise
* it shows the average based on the last n points, where n is the value of
* this field.
*
* @parameter default-value=0
*/
private int movingAverage = 0;
/**
* If true then generate a summary chart only.
*
* @parameter default-value=false
*/
private boolean summaryOnly = false;
/**
* Statistic type, defaulted to
* 'checkstyle,pmd,findbugs,simian,pmd-cpd,cobertura-line,cobertura-branch'.
*
* @parameter default-value="checkstyle,pmd,findbugs,simian,pmd-cpd,cobertura-line,cobertura-branch"
*/
private String types = "checkstyle,pmd,findbugs,simian,pmd-cpd,cobertura-line,cobertura-branch";
/**
* Statistic type to appear on summary chart, defaulted to
* 'checkstyle,pmd,findbugs,simian,pmd-cpd'.
*
* @parameter default-value="checkstyle,pmd,findbugs,simian,pmd-cpd"
*/
private String summaryTypes = "checkstyle,pmd,findbugs,simian,pmd-cpd";
/**
* File prefix for the charts (e.g. cobertura-) Default empty.
*
* @parameter default-value=""
*/
private String filePrefix = "";
/**
* X Axis Title
*
* @parameter default-value="Date"
*/
private String xAxisTitle = "Date";
/**
* Y Axis Title
*
* @parameter default-value="Violation Count / Coverage Percent"
*/
private String yAxisTitle = "Violation Count / Coverage Percent";
/**
* X Axis Title for Summary
*
* @parameter default-value="Date"
*/
private String xAxisSummaryTitle = "Date";
/**
* Y Axis Title for Summary
*
* @parameter default-value="Violation Count"
*/
private String yAxisSummaryTitle = "Violation Count";
/**
* validates the input then generates the charts.
*
* @throws MojoExecutionException
* if anything goes wrong.
*/
public final void execute() throws MojoExecutionException {
// validate the provided parameters
validate();
// Display the files being processed
if (!quiet) {
getLog().info("QALab CHARTS ==> qalabFile='" + qalabFile.getPath() + "'");
getLog().info("toDir='" + toDir.getPath());
getLog().info("width='" + width + "' height='" + height + "' summaryOnly='" + isSummaryOnly() + "'");
getLog().info("Types=" + types);
getLog().info("Summary Types=" + getSummaryTypes());
getLog().info("File Prefix=" + getFilePrefix());
}
generateCharts();
}
/**
* Generate the charts by creating a SAX parser specialised in finding the
* elements of given type and creating charts in a given directory.
*
* @throws MojoExecutionException
* if anything goes wrong.
*/
private void generateCharts() throws MojoExecutionException {
// define a sax stuff...
final BuildStatForChartParser myForChartParser = new BuildStatForChartParser(new Maven2TaskLogger(this));
myForChartParser.setChartHeight(height);
myForChartParser.setChartWidth(width);
myForChartParser.setToDir(toDir.getAbsolutePath() + "/");
myForChartParser.setMovingAverage(movingAverage);
myForChartParser.setSummaryOnly(isSummaryOnly());
myForChartParser.setAcceptedStyle(types);
myForChartParser.setSummaryStyle(getSummaryTypes());
myForChartParser.setQuiet(quiet);
myForChartParser.setFilePrefix(getFilePrefix());
myForChartParser.setXAxisSummaryTitle(xAxisSummaryTitle);
myForChartParser.setXAxisTitle(xAxisTitle);
myForChartParser.setYAxisSummaryTitle(yAxisSummaryTitle);
myForChartParser.setYAxisTitle(yAxisTitle);
try {
SAXParserFactory factory = SAXParserFactory.newInstance();
SAXParser saxParser = factory.newSAXParser();
if (!quiet) {
getLog().info("Parsing " + qalabFile);
}
saxParser.parse(new InputSource(new FileInputStream(qalabFile)), myForChartParser);
} catch (SAXException sex) {
getLog().error(sex.toString());
throw new MojoExecutionException("Error generating charts.", sex);
} catch (FileNotFoundException fnfex) {
getLog().error(fnfex.toString());
throw new MojoExecutionException("Error generating charts.", fnfex);
} catch (IOException ioex) {
getLog().error(ioex.toString());
throw new MojoExecutionException("Error generating charts.", ioex);
} catch (ParserConfigurationException e) {
getLog().error(e.toString());
throw new MojoExecutionException("Error generating charts.", e);
}
}
/**
* Validates the parameters supplied by maven.
*
* @throws MojoExecutionException
* if anything goes wrong.
*/
private void validate() throws MojoExecutionException {
try {
Utils.checkFile(qalabFile, "qalabFile");
} catch (IOException ioex) {
throw new MojoExecutionException(ioex.getMessage(), ioex);
}
if (toDir == null) {
throw new MojoExecutionException("toDir is mandatory");
}
if (toDir.exists() && !toDir.isDirectory()) {
final String message = "toDir must be a directory (" + toDir + ")";
throw new MojoExecutionException(message);
}
if (!toDir.exists()) {
if (!quiet) {
getLog().debug("Creating directory: " + toDir.toString());
}
toDir.mkdir();
}
}
/**
* @return the summaryOnly
*/
public boolean isSummaryOnly() {
return summaryOnly;
}
/**
* @param summaryOnly the summaryOnly to set
*/
public void setSummaryOnly(boolean summaryOnly) {
this.summaryOnly = summaryOnly;
}
/**
* @return the summaryTypes
*/
public String getSummaryTypes() {
return summaryTypes;
}
/**
* @param summaryTypes the summaryTypes to set
*/
public void setSummaryTypes(String summaryTypes) {
this.summaryTypes = summaryTypes;
}
/**
* @return the filePrefix
*/
public String getFilePrefix() {
return filePrefix;
}
/**
* @param filePrefix the filePrefix to set
*/
public void setFilePrefix(String filePrefix) {
this.filePrefix = filePrefix;
}
/**
* @return the height
*/
public int getHeight() {
return height;
}
/**
* @param height the height to set
*/
public void setHeight(int height) {
this.height = height;
}
/**
* @return the movingAverage
*/
public int getMovingAverage() {
return movingAverage;
}
/**
* @param movingAverage the movingAverage to set
*/
public void setMovingAverage(int movingAverage) {
this.movingAverage = movingAverage;
}
/**
* @return the qalabFile
*/
public File getQalabFile() {
return qalabFile;
}
/**
* @param qalabFile the qalabFile to set
*/
public void setQalabFile(File qalabFile) {
this.qalabFile = qalabFile;
}
/**
* @return the quiet
*/
public boolean isQuiet() {
return quiet;
}
/**
* @param quiet the quiet to set
*/
public void setQuiet(boolean quiet) {
this.quiet = quiet;
}
/**
* @return the toDir
*/
public File getToDir() {
return toDir;
}
/**
* @param toDir the toDir to set
*/
public void setToDir(File toDir) {
this.toDir = toDir;
}
/**
* @return the types
*/
public String getTypes() {
return types;
}
/**
* @param types the types to set
*/
public void setTypes(String types) {
this.types = types;
}
/**
* @return the width
*/
public int getWidth() {
return width;
}
/**
* @param width the width to set
*/
public void setWidth(int width) {
this.width = width;
}
public void setXAxisSummaryTitle(String xAxisSummaryTitle) {
this.xAxisSummaryTitle = xAxisSummaryTitle;
}
public void setXAxisTitle(String xAxisTitle) {
this.xAxisTitle = xAxisTitle;
}
public void setYAxisSummaryTitle(String yAxisSummaryTitle) {
this.yAxisSummaryTitle = yAxisSummaryTitle;
}
public void setYAxisTitle(String yAxisTitle) {
this.yAxisTitle = yAxisTitle;
}
}
/*
* ObjectLab is sponsoring QALab
*
* Based in London, we are world leaders in the design and development
* of bespoke applications for the securities financing markets.
*
* Click here to learn more about us
* ___ _ _ _ _ _
* / _ \| |__ (_) ___ ___| |_| | __ _| |__
* | | | | '_ \| |/ _ \/ __| __| | / _` | '_ \
* | |_| | |_) | | __/ (__| |_| |__| (_| | |_) |
* \___/|_.__// |\___|\___|\__|_____\__,_|_.__/
* |__/
*
* www.ObjectLab.co.uk
*/
© 2015 - 2025 Weber Informatics LLC | Privacy Policy