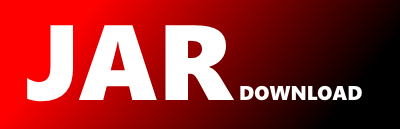
net.objectlab.qalab.m2.BuildStatMoversMojo Maven / Gradle / Ivy
////////////////////////////////////////////////////////////////////////////////
//
// ObjectLab is sponsoring QALab
//
// Based in London, we are world leaders in the design and development
// of bespoke applications for the Securities Financing markets.
//
// Click here to learn more
// ___ _ _ _ _ _
// / _ \| |__ (_) ___ ___| |_| | __ _| |__
// | | | | '_ \| |/ _ \/ __| __| | / _` | '_ \
// | |_| | |_) | | __/ (__| |_| |__| (_| | |_) |
// \___/|_.__// |\___|\___|\__|_____\__,_|_.__/
// |__/
//
// http://www.ObjectLab.co.uk
// ---------------------------------------------------------------------------
//
//QALab is released under the GNU General Public License.
//
//QALab: Collects QA Statistics from your build over time.
//2005+, ObjectLab Ltd
//
//This library is free software; you can redistribute it and/or
//modify it under the terms of the GNU General Public
//License as published by the Free Software Foundation; either
//version 2.1 of the License, or (at your option) any later version.
//
//This library is distributed in the hope that it will be useful,
//but WITHOUT ANY WARRANTY; without even the implied warranty of
//MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
//General Public License for more details.
//
//You should have received a copy of the GNU General Public
//License along with this library; if not, write to the Free Software
//Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
//
////////////////////////////////////////////////////////////////////////////////
package net.objectlab.qalab.m2;
import net.objectlab.qalab.parser.BuildStatMoverHandler;
import net.objectlab.qalab.util.QALabTags;
import net.objectlab.qalab.util.TaskLogger;
import net.objectlab.qalab.m2.util.Utils;
import net.objectlab.qalab.m2.util.Maven2TaskLogger;
import org.apache.maven.plugin.AbstractMojo;
import org.apache.maven.plugin.MojoExecutionException;
import org.xml.sax.InputSource;
import org.xml.sax.SAXException;
import javax.xml.parsers.ParserConfigurationException;
import java.io.File;
import java.io.FileReader;
import java.io.FileWriter;
import java.io.IOException;
import java.text.ParseException;
/**
* Goal to create the mover XML file given the correct parameters. The movers
* report shows what has changed over the last "x" hours.
*
* @author Dave Sag.
* @goal movers
* @phase deploy
*/
public class BuildStatMoversMojo extends AbstractMojo {
// ~ Static final fields ---------------------------------------------------
/** The size of the string based on ISO 8601 date format. */
private static final int MIN_DATE_SIZE = 18;
/**
* The qalabFile, typically qalab.xml.
*
* @parameter expression="${project.basedir}/qalab.xml"
*/
private File qalabFile = null;
/**
* The number of hours to define the time window from now.
*
* @parameter default-value="54"
*/
private String startTimeHoursOffset = null;
/**
* Statistic types to be taken into account
* (checkstyle|findbugs|pmd|simian|pmd-cpd|cobertura-line|cobertura-branch).
* Or comma separated combinations. Default is checkstyle, pmd and findbugs. Please note
* that there is no point of adding pmd-cpd or Simian as no statistics are
* kept at file level for those.
*
* @parameter default-value="checkstyle,pmd,findbugs,cobertura-line,cobertura-branch"
*/
private String types = "checkstyle,pmd,findbugs,cobertura-line,cobertura-branch";
/**
* The output XML file with ups and downs.
*
* @parameter expression="${project.build.directory}/qalab/qalab-movers.xml"
*/
private File qalabMoversOutputFile;
/**
* If true then any debug logging output will be suppressed.
*
* @parameter default-value=false
*/
private boolean quiet = false;
/**
* if true, adjust for weekend ie add 48h if start time falls on a weekend.
*
* @parameter default-value=true
*/
private boolean weekendAdjustment = true;
/**
* Start Time Window (instead of using startTimeHoursOffset).
* "parameter
*/
private String startTimeWindow = null;
/**
* End Time Window (instead of assuming "now").
* "parameter
*/
private String endTimeWindow = null;
/**
* Method invoked by the maven 2 framework to execute the compilation of
* statistics movers withing the time window.
*
* @throws MojoExecutionException
* if anything went wrong.
*/
public final void execute() throws MojoExecutionException {
validate();
if (!quiet) {
getLog().info("QALAB Movers ==> qalabFile='" + qalabFile.getPath());
getLog().info("type=" + types);
getLog().info("output=" + qalabMoversOutputFile.getPath());
getLog().info("adjustForWeekend=" + weekendAdjustment);
getLog().info("startTimeOffset=" + startTimeHoursOffset);
getLog().info("startTimeWindow=" + startTimeWindow);
getLog().info("endTimeWindow=" + endTimeWindow);
}
try {
final TaskLogger logger = new Maven2TaskLogger(this);
final BuildStatMoverHandler handler = new BuildStatMoverHandler(new InputSource(new FileReader(qalabFile)), types,
new FileWriter(qalabMoversOutputFile), weekendAdjustment, logger, quiet);
if (startTimeWindow != null) {
handler.setStartTimeWindow(QALabTags.DEFAULT_DATETIME_FORMAT.parse(fixTime(startTimeWindow)));
}
if (endTimeWindow != null) {
handler.setEndTimeWindow(QALabTags.DEFAULT_DATETIME_FORMAT.parse(fixTime(endTimeWindow)));
}
if (startTimeHoursOffset != null) {
handler.setOffsetHours(startTimeHoursOffset);
}
handler.process();
} catch (ParserConfigurationException pcex) {
getLog().error(pcex.toString());
} catch (SAXException sex) {
getLog().error(sex.toString());
} catch (IOException ioex) {
getLog().error(ioex.toString());
} catch (ParseException pex) {
throw new MojoExecutionException("Date Parse issue", pex);
}
}
/**
* Do some basic parameter validation.
*
* @throws MojoExecutionException
* if anything goes wrong.
*/
private void validate() throws MojoExecutionException {
try {
Utils.checkFile(qalabFile, "qalabFile");
} catch (IOException ioex) {
throw new MojoExecutionException(ioex.getMessage(), ioex);
}
if ((startTimeHoursOffset == null) && (startTimeWindow == null)) {
throw new MojoExecutionException("Please set startTimeHoursOffset or startTimeWindow.");
}
if ((startTimeHoursOffset != null) && (startTimeWindow != null)) {
throw new MojoExecutionException("Use startTimeHoursOffset OR startTimeWindow, not both.");
}
final File dir = qalabMoversOutputFile.getParentFile();
if (!dir.exists()) {
dir.mkdirs();
}
}
/**
* @param aTime
* The time string to adjust.
* @return the adjusted time string - now with full hh:mm:ss
*/
private String fixTime(final String aTime) {
String result = aTime.trim();
if (result.length() < MIN_DATE_SIZE) {
result += " 00:00:00";
}
return result;
}
/**
* @return the endTimeWindow
*/
public String getEndTimeWindow() {
return endTimeWindow;
}
/**
* @param endTimeWindow the endTimeWindow to set
*/
public void setEndTimeWindow(String endTimeWindow) {
this.endTimeWindow = endTimeWindow;
}
/**
* @return the qalabFile
*/
public File getQalabFile() {
return qalabFile;
}
/**
* @param qalabFile the qalabFile to set
*/
public void setQalabFile(File qalabFile) {
this.qalabFile = qalabFile;
}
/**
* @return the qalabMoversOutputFile
*/
public File getQalabMoversOutputFile() {
return qalabMoversOutputFile;
}
/**
* @param qalabMoversOutputFile the qalabMoversOutputFile to set
*/
public void setQalabMoversOutputFile(File qalabMoversOutputFile) {
this.qalabMoversOutputFile = qalabMoversOutputFile;
}
/**
* @return the quiet
*/
public boolean isQuiet() {
return quiet;
}
/**
* @param quiet the quiet to set
*/
public void setQuiet(boolean quiet) {
this.quiet = quiet;
}
/**
* @return the startTimeHoursOffset
*/
public String getStartTimeHoursOffset() {
return startTimeHoursOffset;
}
/**
* @param startTimeHoursOffset the startTimeHoursOffset to set
*/
public void setStartTimeHoursOffset(String startTimeHoursOffset) {
this.startTimeHoursOffset = startTimeHoursOffset;
}
/**
* @return the startTimeWindow
*/
public String getStartTimeWindow() {
return startTimeWindow;
}
/**
* @param startTimeWindow the startTimeWindow to set
*/
public void setStartTimeWindow(String startTimeWindow) {
this.startTimeWindow = startTimeWindow;
}
/**
* @return the types
*/
public String getTypes() {
return types;
}
/**
* @param types the types to set
*/
public void setTypes(String types) {
this.types = types;
}
/**
* @return the weekendAdjustment
*/
public boolean isWeekendAdjustment() {
return weekendAdjustment;
}
/**
* @param weekendAdjustment the weekendAdjustment to set
*/
public void setWeekendAdjustment(boolean weekendAdjustment) {
this.weekendAdjustment = weekendAdjustment;
}
}
/*
* ObjectLab is sponsoring QALab
*
* Based in London, we are world leaders in the design and development
* of bespoke applications for the securities financing markets.
*
* Click here to learn more about us
* ___ _ _ _ _ _
* / _ \| |__ (_) ___ ___| |_| | __ _| |__
* | | | | '_ \| |/ _ \/ __| __| | / _` | '_ \
* | |_| | |_) | | __/ (__| |_| |__| (_| | |_) |
* \___/|_.__// |\___|\___|\__|_____\__,_|_.__/
* |__/
*
* www.ObjectLab.co.uk
*/
© 2015 - 2025 Weber Informatics LLC | Privacy Policy