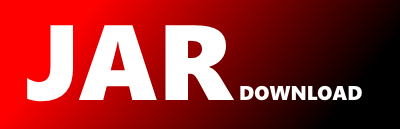
net.objectlab.qalab.m2.util.XmlTransformer Maven / Gradle / Ivy
////////////////////////////////////////////////////////////////////////////////
//
// ObjectLab is sponsoring QALab
//
// Based in London, we are world leaders in the design and development
// of bespoke applications for the Securities Financing markets.
//
// Click here to learn more
// ___ _ _ _ _ _
// / _ \| |__ (_) ___ ___| |_| | __ _| |__
// | | | | '_ \| |/ _ \/ __| __| | / _` | '_ \
// | |_| | |_) | | __/ (__| |_| |__| (_| | |_) |
// \___/|_.__// |\___|\___|\__|_____\__,_|_.__/
// |__/
//
// http://www.ObjectLab.co.uk
// ---------------------------------------------------------------------------
//
//QALab is released under the GNU General Public License.
//
//QALab: Collects QA Statistics from your build over time.
//2005+, ObjectLab Ltd
//
//This library is free software; you can redistribute it and/or
//modify it under the terms of the GNU General Public
//License as published by the Free Software Foundation; either
//version 2.1 of the License, or (at your option) any later version.
//
//This library is distributed in the hope that it will be useful,
//but WITHOUT ANY WARRANTY; without even the implied warranty of
//MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
//General Public License for more details.
//
//You should have received a copy of the GNU General Public
//License along with this library; if not, write to the Free Software
//Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
//
////////////////////////////////////////////////////////////////////////////////
package net.objectlab.qalab.m2.util;
import javax.xml.transform.Source;
import javax.xml.transform.Transformer;
import javax.xml.transform.TransformerFactory;
import javax.xml.transform.TransformerException;
import javax.xml.transform.stream.StreamSource;
import javax.xml.transform.stream.StreamResult;
import java.io.File;
import java.io.InputStream;
import java.util.Map;
import java.util.HashMap;
import java.util.Iterator;
/**
* A simple XML Transformer that is supplied with an XML file, an XSLT
* file and an output file.
* @author Dave Sag.
*/
public class XmlTransformer {
/** the XMl source file. */
private final Source theXML;
/** the XSL source file. */
private final Source theXSLT;
/** the output file. */
private final StreamResult theOutput;
/** the map of parameters. */
private transient Map theParameters;
/**
* Constructor that takes the xml and xslt input stream and output file.
* @param anXml The file containing well formed XML to be transformed.
* @param anXslt The file containing the XSLT that tranforms the XML.
* @param anOutput The file to write the outout to.
*/
public XmlTransformer(final InputStream anXml,
final InputStream anXslt, final File anOutput) {
assert anXml != null : "The supplied XML file was null.";
assert anXslt != null : "The supplied XSLT file was null.";
assert anOutput != null : "The supplied output file was null.";
anOutput.getParentFile().mkdirs();
theXML = new StreamSource(anXml);
theXSLT = new StreamSource(anXslt);
theOutput = new StreamResult(anOutput);
}
/**
* Add an optional XSLT parameter.
* @param aKey The key. a two-part string, the namespace URI enclosed in
* curly braces ({}), followed by the local name. If the name has a
* null URL, the String only contain the local name.
* @param aValue The value.
*/
public final void addParameter(final String aKey, final String aValue) {
if (theParameters == null) {
theParameters = new HashMap();
}
theParameters.put(aKey, aValue);
}
/**
* Performs the XSLT transformation.
* @throws TransformerException if anything went wrong.
*/
public final void transform() throws TransformerException {
final TransformerFactory tf = TransformerFactory.newInstance();
final Transformer t = tf.newTransformer(theXSLT);
// apply any parameters.
if (theParameters != null && !theParameters.isEmpty()) {
for (final Iterator i = theParameters.keySet().iterator();
i.hasNext();) {
final String key = (String) i.next();
t.setParameter(key, theParameters.get(key));
}
}
t.transform(theXML, theOutput);
}
}
/*
* ObjectLab is sponsoring QALab
*
* Based in London, we are world leaders in the design and development
* of bespoke applications for the securities financing markets.
*
* Click here to learn more about us
* ___ _ _ _ _ _
* / _ \| |__ (_) ___ ___| |_| | __ _| |__
* | | | | '_ \| |/ _ \/ __| __| | / _` | '_ \
* | |_| | |_) | | __/ (__| |_| |__| (_| | |_) |
* \___/|_.__// |\___|\___|\__|_____\__,_|_.__/
* |__/
*
* www.ObjectLab.co.uk
*/
© 2015 - 2025 Weber Informatics LLC | Privacy Policy