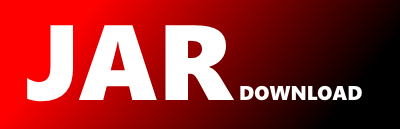
net.obvj.confectory.DataFetchStrategy Maven / Gradle / Ivy
Show all versions of confectory-core Show documentation
/*
* Copyright 2021 obvj.net
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package net.obvj.confectory;
import java.util.Collection;
import java.util.Collections;
import java.util.Map;
import java.util.Set;
import java.util.stream.Stream;
import org.apache.commons.lang3.StringUtils;
import net.obvj.confectory.util.ConfigurationComparator;
/**
* Enumerates the supported data-fetch strategies for use with a
* {@code ConfigurationContainer}.
*
* @author oswaldo.bapvic.jr (Oswaldo Junior)
* @author FernandoNSC (Fernando Tiannamen)
* @since 0.1.0
*
* @see ConfigurationContainer
*/
public enum DataFetchStrategy
{
/**
* Retrieves sorted data from {@code Configuration} objects declared
* with a specific namespace.
*
* If no namespace is specified during data fetch, only {@link Configuration}
* objects without a namespace defined will be searched.
*/
STRICT
{
@Override
protected Stream> getConfigurationStream(String namespace,
Map>> configMap)
{
return STRICT_UNSORTED.getConfigurationStream(namespace, configMap).sorted(new ConfigurationComparator());
}
},
/**
* Retrieves unsorted data from {@code Configuration} objects declared
* with a specific namespace.
*
* If no namespace is specified during data fetch, only {@link Configuration}
* objects without a namespace defined will be searched.
*
* @since 0.2.0
*/
STRICT_UNSORTED
{
@Override
protected Stream> getConfigurationStream(String namespace,
Map>> configMap) {
return configMap.getOrDefault(parseNamespace(namespace), Collections.emptySet()).stream();
}
},
/**
* Retrieves sorted data from all {@code Configuration} objects
* regardless of their namespaces when no namespace specified during data
* fetch.
*/
LENIENT
{
@Override
protected Stream> getConfigurationStream(String namespace,
Map>> configMap)
{
if (StringUtils.isEmpty(namespace))
{
// Flatten and re-order the configuration list
return LENIENT_UNSORTED.getConfigurationStream(namespace, configMap)
.sorted(new ConfigurationComparator());
}
return STRICT.getConfigurationStream(namespace, configMap);
}
},
/**
* Retrieves unsorted data from all {@code Configuration} objects
* regardless of their namespaces when no namespace specified during data
* fetch.
*
* @since 0.2.0
*/
LENIENT_UNSORTED
{
@Override
protected Stream> getConfigurationStream(String namespace,
Map>> configMap)
{
if (StringUtils.isEmpty(namespace))
{
// Flatten and re-order the configuration list
return configMap.values().stream()
.flatMap(Collection::stream);
}
return STRICT_UNSORTED.getConfigurationStream(namespace, configMap);
}
};
/**
* Retrieves a stream of sorted {@link Configuration} objects based on the specified
* {@code namespace}.
*
* The {@link Configuration} objects may be sorted by precedence (highest to lowest).
*
* @param namespace the namespace to be searched
* @param configMap the source map
* @return a stream of {@link Configuration} objects according to the selected strategy,
* never {@code null}
*/
protected abstract Stream> getConfigurationStream(String namespace,
Map>> configMap);
/**
* Returns either the passed namespace, or a default value, if the passed argument is
* empty or null
*
* @param namespace the namespace to the checked
* @return the passed namespace, or the value of
* {@link ConfigurationContainer#DEFAULT_NAMESPACE}, never {@code null}
*/
private static String parseNamespace(String namespace)
{
return StringUtils.defaultString(namespace, ConfigurationContainer.DEFAULT_NAMESPACE);
}
}