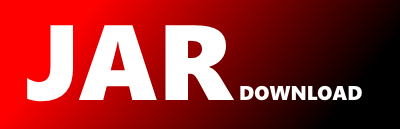
net.officefloor.web.HttpPathFactoryImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of officeweb Show documentation
Show all versions of officeweb Show documentation
OfficeFloor plug-in for Web
/*
* OfficeFloor - http://www.officefloor.net
* Copyright (C) 2005-2018 Daniel Sagenschneider
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see .
*/
package net.officefloor.web;
import net.officefloor.server.http.HttpException;
import net.officefloor.web.build.HttpPathFactory;
import net.officefloor.web.value.retrieve.ValueRetriever;
/**
* Factory to create the HTTP path.
*
* @author Daniel Sagenschneider
*/
public class HttpPathFactoryImpl implements HttpPathFactory {
/**
* Segment of the path.
*/
public static abstract class Segment {
/**
* Writes the values.
*
* @param values
* Values.
* @param target
* {@link Appendable}.
* @throws Exception
* Failure in writing value.
*/
protected abstract void write(T values, Appendable target) throws Exception;
}
/**
* Static {@link Segment}.
*/
public static class StaticSegment extends Segment {
/**
* Static path.
*/
private final String staticPath;
/**
* Instantiate.
*
* @param staticPath
* Static path.
*/
public StaticSegment(String staticPath) {
this.staticPath = staticPath;
}
/*
* =================== Segment ===================
*/
@Override
protected void write(Object values, Appendable target) throws Exception {
target.append(this.staticPath);
}
}
/**
* Parameter {@link Segment}.
*/
public static class ParameterSegment extends Segment {
/**
* Property name to obtain value.
*/
private final String propertyName;
/**
* {@link ValueRetriever} to obtain the value.
*/
private final ValueRetriever valueRetriever;
/**
* Instantiate.
*
* @param propertyName
* Property name to obtain value.
* @param valueRetriever
* {@link ValueRetriever} to obtain the value.
*/
public ParameterSegment(String propertyName, ValueRetriever valueRetriever) {
this.propertyName = propertyName;
this.valueRetriever = valueRetriever;
}
/*
* =================== Segment ===================
*/
@Override
protected void write(T values, Appendable target) throws Exception {
// Obtain the parameter value
Object value = this.valueRetriever.retrieveValue(values, this.propertyName);
if (value == null) {
value = "";
}
// Write the value
target.append(value.toString());
}
}
/**
* {@link ThreadLocal} {@link StringBuilder}.
*/
private static ThreadLocal stringBuilder = new ThreadLocal() {
@Override
protected StringBuilder initialValue() {
return new StringBuilder();
}
};
/**
* Type to obtain values.
*/
private final Class valuesType;
/**
* {@link Segment} instances to create the path.
*/
private final Segment[] segments;
/**
* Instantiate.
*
* @param valuesType
* Type to obtain values.
* @param segments
* {@link Segment} instances.
*/
public HttpPathFactoryImpl(Class valuesType, Segment[] segments) {
this.valuesType = valuesType;
this.segments = segments;
}
/*
* ==================== HttpPathFactory ====================
*/
@Override
public Class getValuesType() {
return this.valuesType;
}
@Override
public String createApplicationClientPath(T values) throws HttpException {
try {
// Obtain the string builder to create the path
StringBuilder buffer = stringBuilder.get();
buffer.setLength(0);
// Generate the path
for (int i = 0; i < this.segments.length; i++) {
Segment segment = this.segments[i];
segment.write(values, buffer);
}
// Return the path
return buffer.toString();
} catch (Exception ex) {
throw new HttpException(ex);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy