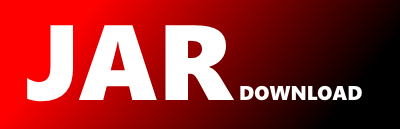
net.officefloor.web.build.WebArchitect Maven / Gradle / Ivy
Show all versions of officeweb Show documentation
/*
* OfficeFloor - http://www.officefloor.net
* Copyright (C) 2005-2018 Daniel Sagenschneider
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see .
*/
package net.officefloor.web.build;
import java.lang.annotation.Annotation;
import java.util.ServiceLoader;
import net.officefloor.compile.spi.office.OfficeArchitect;
import net.officefloor.compile.spi.office.OfficeFlowSinkNode;
import net.officefloor.compile.spi.office.OfficeFlowSourceNode;
import net.officefloor.compile.spi.office.OfficeManagedObject;
import net.officefloor.compile.spi.office.OfficeSection;
import net.officefloor.compile.spi.office.OfficeSectionInput;
import net.officefloor.server.http.HttpMethod;
import net.officefloor.server.http.HttpRequest;
import net.officefloor.server.http.ServerHttpConnection;
import net.officefloor.web.HttpObject;
import net.officefloor.web.accept.AcceptNegotiator;
import net.officefloor.web.session.HttpSession;
import net.officefloor.web.state.HttpApplicationState;
import net.officefloor.web.state.HttpRequestState;
/**
* Web configuration extensions for the {@link OfficeArchitect}.
*
* @author Daniel Sagenschneider
*/
public abstract interface WebArchitect {
/**
* Name of the {@link OfficeSection} that handles the {@link HttpRequest}
* instances.
*/
static String HANDLER_SECTION_NAME = "HANDLE_HTTP_SECTION";
/**
* Name of the {@link OfficeSectionInput} that handles the {@link HttpRequest}
* instances.
*/
static String HANDLER_INPUT_NAME = "HANDLE_HTTP_INPUT";
/**
*
* Manually adds a {@link HttpObjectParserFactory}.
*
* Typically these should be configured via {@link ServiceLoader}, so can be
* plugged in as required.
*
* @param objectParserFactory
* {@link HttpObjectParserFactory}.
*/
void addHttpObjectParser(HttpObjectParserFactory objectParserFactory);
/**
*
* Adds another {@link Class} as an alias for the {@link HttpObject} annotation.
*
* As code generators are likely to be used for the HTTP objects, it is not
* always possible to generate the {@link Class} annotated with
* {@link HttpObject}. This allows another {@link Annotation} to indicate the
* parameter object is a HTTP object.
*
* @param httpObjectAnnotationAliasClass
* Alias {@link Annotation} {@link Class} for {@link HttpObject}.
* @param acceptedContentTypes
* Listing of the content-type
values accepted. May be
* empty array to allow supporting all available
* content-type
{@link HttpObjectParser} instances
* available.
*/
void addHttpObjectAnnotationAlias(Class> httpObjectAnnotationAliasClass, String... acceptedContentTypes);
/**
* Adds a {@link HttpObjectResponderFactory}.
*
* @param objectResponderFactory
* {@link HttpObjectResponderFactory}.
*/
void addHttpObjectResponder(HttpObjectResponderFactory objectResponderFactory);
/**
* Adds an object to be lazily created and stored within the
* {@link HttpApplicationState}.
*
* @param objectClass
* Class of the object.
* @param bindName
* Name to bind the object within the {@link HttpApplicationState}.
* @return {@link OfficeManagedObject}.
*/
OfficeManagedObject addHttpApplicationObject(Class> objectClass, String bindName);
/**
*
* Adds an object to be lazily created and stored within the
* {@link HttpApplicationState}.
*
* The bound name is arbitrarily chosen but will be unique for the object.
*
* @param objectClass
* Class of the object.
* @return {@link OfficeManagedObject}.
*/
OfficeManagedObject addHttpApplicationObject(Class> objectClass);
/**
* Adds an object to be lazily created and stored within the
* {@link HttpSession}.
*
* @param objectClass
* Class of the object.
* @param bindName
* Name to bind the object within the {@link HttpSession}.
* @return {@link OfficeManagedObject}.
*/
OfficeManagedObject addHttpSessionObject(Class> objectClass, String bindName);
/**
*
* Adds an object to be lazily created and stored within the
* {@link HttpSession}.
*
* The bound name is arbitrarily chosen but will be unique for the object.
*
* @param objectClass
* Class of the object.
* @return {@link OfficeManagedObject}.
*/
OfficeManagedObject addHttpSessionObject(Class> objectClass);
/**
* Adds an object to be lazily created and stored within the
* {@link HttpRequestState}.
*
* @param objectClass
* Class of the object.
* @param isLoadParameters
* Indicates whether to load the HTTP parameters to instantiated
* objects.
* @param bindName
* Name to bind the object within the {@link HttpRequestState}.
* @return {@link OfficeManagedObject}.
*/
OfficeManagedObject addHttpRequestObject(Class> objectClass, boolean isLoadParameters, String bindName);
/**
*
* Adds an object to be lazily created and stored within the
* {@link HttpRequestState}.
*
* The bound name is arbitrarily chosen but will be unique for the object.
*
* @param objectClass
* Class of the object.
* @param isLoadParameters
* Indicates whether to load the HTTP parameters to instantiated
* objects.
* @return {@link OfficeManagedObject}.
*/
OfficeManagedObject addHttpRequestObject(Class> objectClass, boolean isLoadParameters);
/**
* Adds a HTTP argument.
*
* @param parameterName
* Name of the parameter.
* @param location
* {@link HttpValueLocation} to obtain the argument value. May be
* null
to obtain from anywhere on the
* {@link HttpRequest}.
* @return {@link OfficeManagedObject}.
*/
OfficeManagedObject addHttpArgument(String parameterName, HttpValueLocation location);
/**
* Adds a HTTP {@link Object} to be parsed from the {@link HttpRequest}.
*
* @param objectClass
* Class of the object.
* @param acceptedContentTypes
* Listing of the content-type
values accepted. May be
* empty array to allow supporting all available
* content-type
{@link HttpObjectParser} instances
* available.
* @return {@link OfficeManagedObject}.
*/
OfficeManagedObject addHttpObject(Class> objectClass, String... acceptedContentTypes);
/**
* Indicates if the path contains path parameters.
*
* @param path
* Path.
* @return true
if path contains parameters.
*/
boolean isPathParameters(String path);
/**
* Creates a {@link HttpUrlContinuation} into the web application. This will
* always be a {@link HttpMethod#GET} due to redirection required for the
* {@link HttpUrlContinuation}.
*
* @param isSecure
* Indicates if secure connection required.
* @param applicationPath
* Application path to be linked.
* @return {@link HttpUrlContinuation}.
*/
HttpUrlContinuation getHttpInput(boolean isSecure, String applicationPath);
/**
* Creates a {@link HttpInput} into the application.
*
* @param isSecure
* Indicates if secure connection required.
* @param httpMethodName
* Name of the {@link HttpMethod}.
* @param applicationPath
* URL path of the application to be linked.
* @return {@link HttpInput}.
*/
HttpInput getHttpInput(boolean isSecure, String httpMethodName, String applicationPath);
/**
*
* Runs the {@link ServerHttpConnection} through routing again.
*
* Typically, this is used on importing previous state into the
* {@link ServerHttpConnection} and then have it serviced.
*
* @param flowSourceNode
* {@link OfficeFlowSourceNode} to trigger re-routing the
* {@link ServerHttpConnection}.
*/
void reroute(OfficeFlowSourceNode flowSourceNode);
/**
*
* Intercepts all {@link HttpRequest} instances before servicing. Multiple
* intercepts may be configured, with them executed in the order they are added.
*
* This allows, for example, logging all requests to the web application.
*
* @param flowSinkNode
* {@link OfficeFlowSinkNode} to handle intercepting the
* {@link HttpRequest}.
* @param flowSourceNode
* {@link OfficeFlowSourceNode} to continue servicing the
* {@link HttpRequest}.
*/
void intercept(OfficeFlowSinkNode flowSinkNode, OfficeFlowSourceNode flowSourceNode);
/**
*
* Chains a {@link OfficeSectionInput} to the end of the servicing chain to
* handle a {@link HttpRequest}. Multiple chained services may be added, with
* them executed in the order they are added.
*
* The {@link WebArchitect} functionality is always the first in the chain to
* attempt to service the {@link HttpRequest}. This allows, for example, adding
* a chained servicer for serving resources from a file system.
*
* @param flowSinkNode
* {@link OfficeFlowSinkNode} to handle the {@link HttpRequest}.
* @param notHandledOutput
* {@link OfficeFlowSourceNode} should this servicer not handle the
* {@link HttpRequest}. May be null
if handles all
* {@link HttpRequest} instances (any services chained after this
* will therefore not be used).
*/
void chainServicer(OfficeFlowSinkNode flowSinkNode, OfficeFlowSourceNode notHandledOutput);
/**
* Creates the {@link AcceptNegotiatorBuilder} to build an
* {@link AcceptNegotiator}.
*
* @param
* Handler type.
* @return {@link AcceptNegotiatorBuilder} to build an {@link AcceptNegotiator}.
*/
AcceptNegotiatorBuilder createAcceptNegotiator();
/**
* Informs the {@link OfficeArchitect} of the web architect. This is to be
* invoked once all web architecture is configured.
*/
void informOfficeArchitect();
}