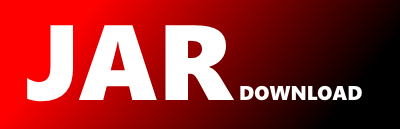
net.officefloor.web.state.HttpApplicationStateManagedObjectSource Maven / Gradle / Ivy
/*
* OfficeFloor - http://www.officefloor.net
* Copyright (C) 2005-2018 Daniel Sagenschneider
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see .
*/
package net.officefloor.web.state;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
import net.officefloor.frame.api.build.None;
import net.officefloor.frame.api.managedobject.ManagedObject;
import net.officefloor.frame.api.managedobject.source.ManagedObjectSource;
import net.officefloor.frame.api.managedobject.source.impl.AbstractManagedObjectSource;
import net.officefloor.frame.api.source.PrivateSource;
import net.officefloor.server.http.HttpException;
import net.officefloor.server.http.ServerHttpConnection;
import net.officefloor.web.route.WebRouter;
/**
* {@link ManagedObjectSource} for the {@link HttpRequestState}.
*
* @author Daniel Sagenschneider
*/
@PrivateSource
public class HttpApplicationStateManagedObjectSource extends AbstractManagedObjectSource
implements ManagedObject, HttpApplicationState {
/**
* Context path.
*/
private final String contextPath;
/**
* Attributes.
*/
private final Map attributes = new ConcurrentHashMap<>();
/**
* Instantiate.
*
* @param contextPath
* Context path.
*/
public HttpApplicationStateManagedObjectSource(String contextPath) {
this.contextPath = contextPath;
}
/*
* =================== ManagedObjectSource ==========================
*/
@Override
protected void loadSpecification(SpecificationContext context) {
// No properties required
}
@Override
protected void loadMetaData(MetaDataContext context) throws Exception {
context.setObjectClass(HttpApplicationState.class);
}
@Override
protected ManagedObject getManagedObject() throws Throwable {
return this;
}
/*
* ====================== ManagedObject ===========================
*/
@Override
public Object getObject() throws Throwable {
return this;
}
/*
* ==================== HttpApplicationState ==========================
*/
@Override
public String getContextPath() {
return this.contextPath;
}
@Override
public String createApplicationClientUrl(boolean isSecure, String path, ServerHttpConnection connection) {
// Create the application path
path = this.createApplicationClientPath(path);
// Determine if appropriately secure
if (connection.isSecure() == isSecure) {
// Relative path
return path;
} else {
// Full path back to server
return connection.getServerLocation().createClientUrl(isSecure, path);
}
}
@Override
public String createApplicationClientPath(String path) {
// Create the application path
if (this.contextPath != null) {
path = this.contextPath + path;
}
// Return the path
return path;
}
@Override
public String extractApplicationPath(ServerHttpConnection connection) throws HttpException {
return WebRouter.transformToApplicationCanonicalPath(connection.getRequest().getUri(), this.contextPath);
}
@Override
public Object getAttribute(String name) {
return HttpApplicationStateManagedObjectSource.this.attributes.get(name);
}
@Override
public Iterator getAttributeNames() {
// Create copy of names (stops concurrency issues)
List names = new ArrayList(this.attributes.keySet());
return names.iterator();
}
@Override
public void setAttribute(String name, Object object) {
this.attributes.put(name, object);
}
@Override
public void removeAttribute(String name) {
this.attributes.remove(name);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy