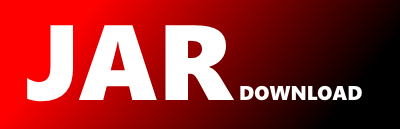
net.officefloor.woof.model.objects.WoofFlowModel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of officeweb_configuration Show documentation
Show all versions of officeweb_configuration Show documentation
Configuration for WoOF (Web on OfficeFloor)
/*
*
*/
package net.officefloor.woof.model.objects;
import javax.annotation.Generated;
import net.officefloor.model.AbstractModel;
import net.officefloor.model.ConnectionModel;
import net.officefloor.model.ItemModel;
import net.officefloor.model.RemoveConnectionsAction;
@Generated("net.officefloor.model.generate.ModelGenerator")
public class WoofFlowModel extends AbstractModel implements ItemModel {
public static enum WoofFlowEvent {
CHANGE_NAME, CHANGE_SECTION, CHANGE_INPUT
}
/**
* Default constructor.
*/
public WoofFlowModel() {
}
/**
* Convenience constructor.
*
* @param name Name.
* @param section Section.
* @param input Input.
*/
public WoofFlowModel(
String name
, String section
, String input
) {
this.name = name;
this.section = section;
this.input = input;
}
/**
* Convenience constructor allowing XY initialising.
*
* @param name Name.
* @param section Section.
* @param input Input.
* @param x Horizontal location.
* @param y Vertical location.
*/
public WoofFlowModel(
String name
, String section
, String input
, int x
, int y
) {
this.name = name;
this.section = section;
this.input = input;
this.setX(x);
this.setY(y);
}
/**
* Name.
*/
private String name;
/**
* @return Name.
*/
public String getName() {
return this.name;
}
/**
* @param name Name.
*/
public void setName(String name) {
String oldValue = this.name;
this.name = name;
this.changeField(oldValue, this.name, WoofFlowEvent.CHANGE_NAME);
}
/**
* Section.
*/
private String section;
/**
* @return Section.
*/
public String getSection() {
return this.section;
}
/**
* @param section Section.
*/
public void setSection(String section) {
String oldValue = this.section;
this.section = section;
this.changeField(oldValue, this.section, WoofFlowEvent.CHANGE_SECTION);
}
/**
* Input.
*/
private String input;
/**
* @return Input.
*/
public String getInput() {
return this.input;
}
/**
* @param input Input.
*/
public void setInput(String input) {
String oldValue = this.input;
this.input = input;
this.changeField(oldValue, this.input, WoofFlowEvent.CHANGE_INPUT);
}
/**
* Remove Connections.
*
* @return {@link RemoveConnectionsAction} to remove the {@link ConnectionModel} instances.
*/
public RemoveConnectionsAction removeConnections() {
RemoveConnectionsAction _action = new RemoveConnectionsAction(this);
return _action;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy