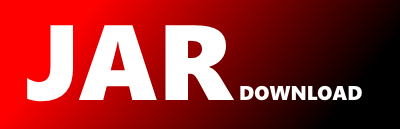
net.officefloor.woof.model.objects.WoofManagedObjectModel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of officeweb_configuration Show documentation
Show all versions of officeweb_configuration Show documentation
Configuration for WoOF (Web on OfficeFloor)
/*
*
*/
package net.officefloor.woof.model.objects;
import java.util.List;
import java.util.LinkedList;
import javax.annotation.Generated;
import net.officefloor.model.AbstractModel;
import net.officefloor.model.ConnectionModel;
import net.officefloor.model.ItemModel;
import net.officefloor.model.RemoveConnectionsAction;
@Generated("net.officefloor.model.generate.ModelGenerator")
public class WoofManagedObjectModel extends AbstractModel implements ItemModel, WoofObjectSourceModel {
public static enum WoofManagedObjectEvent {
CHANGE_MANAGED_OBJECT_SOURCE_CLASS_NAME, CHANGE_CLASS_MANAGED_OBJECT_SOURCE_CLASS, CHANGE_TIMEOUT, CHANGE_QUALIFIER, CHANGE_TYPE, CHANGE_SCOPE, CHANGE_POOL, ADD_PROPERTY_SOURCE, REMOVE_PROPERTY_SOURCE, ADD_TYPE_QUALIFICATION, REMOVE_TYPE_QUALIFICATION, ADD_FLOW, REMOVE_FLOW, ADD_DEPENDENCY, REMOVE_DEPENDENCY
}
/**
* Default constructor.
*/
public WoofManagedObjectModel() {
}
/**
* Convenience constructor for new non-linked instance.
*
* @param managedObjectSourceClassName Managed object source class name.
* @param classManagedObjectSourceClass Class managed object source class.
* @param timeout Timeout.
* @param qualifier Qualifier.
* @param type Type.
* @param scope Scope.
*/
public WoofManagedObjectModel(
String managedObjectSourceClassName
, String classManagedObjectSourceClass
, long timeout
, String qualifier
, String type
, String scope
) {
this.managedObjectSourceClassName = managedObjectSourceClassName;
this.classManagedObjectSourceClass = classManagedObjectSourceClass;
this.timeout = timeout;
this.qualifier = qualifier;
this.type = type;
this.scope = scope;
}
/**
* Convenience constructor for new non-linked instance allowing XY initialising.
*
* @param managedObjectSourceClassName Managed object source class name.
* @param classManagedObjectSourceClass Class managed object source class.
* @param timeout Timeout.
* @param qualifier Qualifier.
* @param type Type.
* @param scope Scope.
* @param x Horizontal location.
* @param y Vertical location.
*/
public WoofManagedObjectModel(
String managedObjectSourceClassName
, String classManagedObjectSourceClass
, long timeout
, String qualifier
, String type
, String scope
, int x
, int y
) {
this.managedObjectSourceClassName = managedObjectSourceClassName;
this.classManagedObjectSourceClass = classManagedObjectSourceClass;
this.timeout = timeout;
this.qualifier = qualifier;
this.type = type;
this.scope = scope;
this.setX(x);
this.setY(y);
}
/**
* Convenience constructor.
*
* @param managedObjectSourceClassName Managed object source class name.
* @param classManagedObjectSourceClass Class managed object source class.
* @param timeout Timeout.
* @param qualifier Qualifier.
* @param type Type.
* @param scope Scope.
* @param pool Pool.
* @param propertySource Property source.
* @param typeQualification Type qualification.
* @param flow Flow.
* @param dependency Dependency.
*/
public WoofManagedObjectModel(
String managedObjectSourceClassName
, String classManagedObjectSourceClass
, long timeout
, String qualifier
, String type
, String scope
, WoofPoolModel pool
, PropertySourceModel[] propertySource
, TypeQualificationModel[] typeQualification
, WoofFlowModel[] flow
, WoofDependencyModel[] dependency
) {
this.managedObjectSourceClassName = managedObjectSourceClassName;
this.classManagedObjectSourceClass = classManagedObjectSourceClass;
this.timeout = timeout;
this.qualifier = qualifier;
this.type = type;
this.scope = scope;
this.pool = pool;
if (propertySource != null) {
for (PropertySourceModel model : propertySource) {
this.propertySource.add(model);
}
}
if (typeQualification != null) {
for (TypeQualificationModel model : typeQualification) {
this.typeQualification.add(model);
}
}
if (flow != null) {
for (WoofFlowModel model : flow) {
this.flow.add(model);
}
}
if (dependency != null) {
for (WoofDependencyModel model : dependency) {
this.dependency.add(model);
}
}
}
/**
* Convenience constructor allowing XY initialising.
*
* @param managedObjectSourceClassName Managed object source class name.
* @param classManagedObjectSourceClass Class managed object source class.
* @param timeout Timeout.
* @param qualifier Qualifier.
* @param type Type.
* @param scope Scope.
* @param pool Pool.
* @param propertySource Property source.
* @param typeQualification Type qualification.
* @param flow Flow.
* @param dependency Dependency.
* @param x Horizontal location.
* @param y Vertical location.
*/
public WoofManagedObjectModel(
String managedObjectSourceClassName
, String classManagedObjectSourceClass
, long timeout
, String qualifier
, String type
, String scope
, WoofPoolModel pool
, PropertySourceModel[] propertySource
, TypeQualificationModel[] typeQualification
, WoofFlowModel[] flow
, WoofDependencyModel[] dependency
, int x
, int y
) {
this.managedObjectSourceClassName = managedObjectSourceClassName;
this.classManagedObjectSourceClass = classManagedObjectSourceClass;
this.timeout = timeout;
this.qualifier = qualifier;
this.type = type;
this.scope = scope;
this.pool = pool;
if (propertySource != null) {
for (PropertySourceModel model : propertySource) {
this.propertySource.add(model);
}
}
if (typeQualification != null) {
for (TypeQualificationModel model : typeQualification) {
this.typeQualification.add(model);
}
}
if (flow != null) {
for (WoofFlowModel model : flow) {
this.flow.add(model);
}
}
if (dependency != null) {
for (WoofDependencyModel model : dependency) {
this.dependency.add(model);
}
}
this.setX(x);
this.setY(y);
}
/**
* Managed object source class name.
*/
private String managedObjectSourceClassName;
/**
* @return Managed object source class name.
*/
public String getManagedObjectSourceClassName() {
return this.managedObjectSourceClassName;
}
/**
* @param managedObjectSourceClassName Managed object source class name.
*/
public void setManagedObjectSourceClassName(String managedObjectSourceClassName) {
String oldValue = this.managedObjectSourceClassName;
this.managedObjectSourceClassName = managedObjectSourceClassName;
this.changeField(oldValue, this.managedObjectSourceClassName, WoofManagedObjectEvent.CHANGE_MANAGED_OBJECT_SOURCE_CLASS_NAME);
}
/**
* Class managed object source class.
*/
private String classManagedObjectSourceClass;
/**
* @return Class managed object source class.
*/
public String getClassManagedObjectSourceClass() {
return this.classManagedObjectSourceClass;
}
/**
* @param classManagedObjectSourceClass Class managed object source class.
*/
public void setClassManagedObjectSourceClass(String classManagedObjectSourceClass) {
String oldValue = this.classManagedObjectSourceClass;
this.classManagedObjectSourceClass = classManagedObjectSourceClass;
this.changeField(oldValue, this.classManagedObjectSourceClass, WoofManagedObjectEvent.CHANGE_CLASS_MANAGED_OBJECT_SOURCE_CLASS);
}
/**
* Timeout.
*/
private long timeout;
/**
* @return Timeout.
*/
public long getTimeout() {
return this.timeout;
}
/**
* @param timeout Timeout.
*/
public void setTimeout(long timeout) {
long oldValue = this.timeout;
this.timeout = timeout;
this.changeField(oldValue, this.timeout, WoofManagedObjectEvent.CHANGE_TIMEOUT);
}
/**
* Qualifier.
*/
private String qualifier;
/**
* @return Qualifier.
*/
public String getQualifier() {
return this.qualifier;
}
/**
* @param qualifier Qualifier.
*/
public void setQualifier(String qualifier) {
String oldValue = this.qualifier;
this.qualifier = qualifier;
this.changeField(oldValue, this.qualifier, WoofManagedObjectEvent.CHANGE_QUALIFIER);
}
/**
* Type.
*/
private String type;
/**
* @return Type.
*/
public String getType() {
return this.type;
}
/**
* @param type Type.
*/
public void setType(String type) {
String oldValue = this.type;
this.type = type;
this.changeField(oldValue, this.type, WoofManagedObjectEvent.CHANGE_TYPE);
}
/**
* Scope.
*/
private String scope;
/**
* @return Scope.
*/
public String getScope() {
return this.scope;
}
/**
* @param scope Scope.
*/
public void setScope(String scope) {
String oldValue = this.scope;
this.scope = scope;
this.changeField(oldValue, this.scope, WoofManagedObjectEvent.CHANGE_SCOPE);
}
/**
* Pool.
*/
private WoofPoolModel pool;
/**
* @return Pool.
*/
public WoofPoolModel getPool() {
return this.pool;
}
/**
* @param pool Pool.
*/
public void setPool(WoofPoolModel pool) {
WoofPoolModel oldValue = this.pool;
this.pool = pool;
this.changeField(oldValue, this.pool, WoofManagedObjectEvent.CHANGE_POOL);
}
/**
* Property source.
*/
private List propertySource = new LinkedList();
/**
* @return Property source.
*/
public List getPropertySources() {
return this.propertySource;
}
/**
* @param propertySource Property source.
*/
public void addPropertySource(PropertySourceModel propertySource) {
this.addItemToList(propertySource, this.propertySource, WoofManagedObjectEvent.ADD_PROPERTY_SOURCE);
}
/**
* @param propertySource Property source.
*/
public void removePropertySource(PropertySourceModel propertySource) {
this.removeItemFromList(propertySource, this.propertySource, WoofManagedObjectEvent.REMOVE_PROPERTY_SOURCE);
}
/**
* Type qualification.
*/
private List typeQualification = new LinkedList();
/**
* @return Type qualification.
*/
public List getTypeQualifications() {
return this.typeQualification;
}
/**
* @param typeQualification Type qualification.
*/
public void addTypeQualification(TypeQualificationModel typeQualification) {
this.addItemToList(typeQualification, this.typeQualification, WoofManagedObjectEvent.ADD_TYPE_QUALIFICATION);
}
/**
* @param typeQualification Type qualification.
*/
public void removeTypeQualification(TypeQualificationModel typeQualification) {
this.removeItemFromList(typeQualification, this.typeQualification, WoofManagedObjectEvent.REMOVE_TYPE_QUALIFICATION);
}
/**
* Flow.
*/
private List flow = new LinkedList();
/**
* @return Flow.
*/
public List getFlows() {
return this.flow;
}
/**
* @param flow Flow.
*/
public void addFlow(WoofFlowModel flow) {
this.addItemToList(flow, this.flow, WoofManagedObjectEvent.ADD_FLOW);
}
/**
* @param flow Flow.
*/
public void removeFlow(WoofFlowModel flow) {
this.removeItemFromList(flow, this.flow, WoofManagedObjectEvent.REMOVE_FLOW);
}
/**
* Dependency.
*/
private List dependency = new LinkedList();
/**
* @return Dependency.
*/
public List getDependencies() {
return this.dependency;
}
/**
* @param dependency Dependency.
*/
public void addDependency(WoofDependencyModel dependency) {
this.addItemToList(dependency, this.dependency, WoofManagedObjectEvent.ADD_DEPENDENCY);
}
/**
* @param dependency Dependency.
*/
public void removeDependency(WoofDependencyModel dependency) {
this.removeItemFromList(dependency, this.dependency, WoofManagedObjectEvent.REMOVE_DEPENDENCY);
}
/**
* Remove Connections.
*
* @return {@link RemoveConnectionsAction} to remove the {@link ConnectionModel} instances.
*/
public RemoveConnectionsAction removeConnections() {
RemoveConnectionsAction _action = new RemoveConnectionsAction(this);
return _action;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy