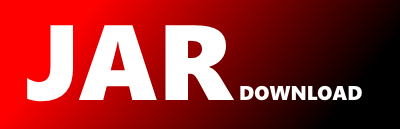
net.officefloor.woof.model.resources.WoofResourceSecurityModel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of officeweb_configuration Show documentation
Show all versions of officeweb_configuration Show documentation
Configuration for WoOF (Web on OfficeFloor)
/*
*
*/
package net.officefloor.woof.model.resources;
import java.util.List;
import java.util.LinkedList;
import javax.annotation.Generated;
import net.officefloor.model.AbstractModel;
import net.officefloor.model.ConnectionModel;
import net.officefloor.model.ItemModel;
import net.officefloor.model.RemoveConnectionsAction;
@Generated("net.officefloor.model.generate.ModelGenerator")
public class WoofResourceSecurityModel extends AbstractModel implements ItemModel {
public static enum WoofResourceSecurityEvent {
CHANGE_HTTP_SECURITY_NAME, ADD_ROLE, REMOVE_ROLE, ADD_REQUIRED_ROLE, REMOVE_REQUIRED_ROLE
}
/**
* Default constructor.
*/
public WoofResourceSecurityModel() {
}
/**
* Convenience constructor.
*
* @param httpSecurityName Http security name.
* @param role Role.
* @param requiredRole Required role.
*/
public WoofResourceSecurityModel(
String httpSecurityName
, String[] role
, String[] requiredRole
) {
this.httpSecurityName = httpSecurityName;
if (role != null) {
for (String model : role) {
this.role.add(model);
}
}
if (requiredRole != null) {
for (String model : requiredRole) {
this.requiredRole.add(model);
}
}
}
/**
* Convenience constructor allowing XY initialising.
*
* @param httpSecurityName Http security name.
* @param role Role.
* @param requiredRole Required role.
* @param x Horizontal location.
* @param y Vertical location.
*/
public WoofResourceSecurityModel(
String httpSecurityName
, String[] role
, String[] requiredRole
, int x
, int y
) {
this.httpSecurityName = httpSecurityName;
if (role != null) {
for (String model : role) {
this.role.add(model);
}
}
if (requiredRole != null) {
for (String model : requiredRole) {
this.requiredRole.add(model);
}
}
this.setX(x);
this.setY(y);
}
/**
* Http security name.
*/
private String httpSecurityName;
/**
* @return Http security name.
*/
public String getHttpSecurityName() {
return this.httpSecurityName;
}
/**
* @param httpSecurityName Http security name.
*/
public void setHttpSecurityName(String httpSecurityName) {
String oldValue = this.httpSecurityName;
this.httpSecurityName = httpSecurityName;
this.changeField(oldValue, this.httpSecurityName, WoofResourceSecurityEvent.CHANGE_HTTP_SECURITY_NAME);
}
/**
* Role.
*/
private List role = new LinkedList();
/**
* @return Role.
*/
public List getRoles() {
return this.role;
}
/**
* @param role Role.
*/
public void addRole(String role) {
this.addItemToList(role, this.role, WoofResourceSecurityEvent.ADD_ROLE);
}
/**
* @param role Role.
*/
public void removeRole(String role) {
this.removeItemFromList(role, this.role, WoofResourceSecurityEvent.REMOVE_ROLE);
}
/**
* Required role.
*/
private List requiredRole = new LinkedList();
/**
* @return Required role.
*/
public List getRequiredRoles() {
return this.requiredRole;
}
/**
* @param requiredRole Required role.
*/
public void addRequiredRole(String requiredRole) {
this.addItemToList(requiredRole, this.requiredRole, WoofResourceSecurityEvent.ADD_REQUIRED_ROLE);
}
/**
* @param requiredRole Required role.
*/
public void removeRequiredRole(String requiredRole) {
this.removeItemFromList(requiredRole, this.requiredRole, WoofResourceSecurityEvent.REMOVE_REQUIRED_ROLE);
}
/**
* Remove Connections.
*
* @return {@link RemoveConnectionsAction} to remove the {@link ConnectionModel} instances.
*/
public RemoveConnectionsAction removeConnections() {
RemoveConnectionsAction _action = new RemoveConnectionsAction(this);
return _action;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy