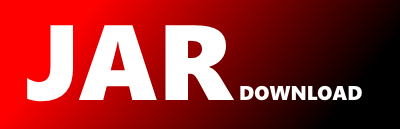
net.officefloor.woof.model.teams.WoofTeamModel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of officeweb_configuration Show documentation
Show all versions of officeweb_configuration Show documentation
Configuration for WoOF (Web on OfficeFloor)
/*
*
*/
package net.officefloor.woof.model.teams;
import java.util.List;
import java.util.LinkedList;
import javax.annotation.Generated;
import net.officefloor.model.AbstractModel;
import net.officefloor.model.ConnectionModel;
import net.officefloor.model.ItemModel;
import net.officefloor.model.RemoveConnectionsAction;
@Generated("net.officefloor.model.generate.ModelGenerator")
public class WoofTeamModel extends AbstractModel implements ItemModel {
public static enum WoofTeamEvent {
CHANGE_TEAM_SIZE, CHANGE_TEAM_SOURCE_CLASS_NAME, CHANGE_QUALIFIER, CHANGE_TYPE, ADD_PROPERTY_SOURCE, REMOVE_PROPERTY_SOURCE, ADD_TYPE_QUALIFICATION, REMOVE_TYPE_QUALIFICATION
}
/**
* Default constructor.
*/
public WoofTeamModel() {
}
/**
* Convenience constructor for new non-linked instance.
*
* @param teamSize Team size.
* @param teamSourceClassName Team source class name.
* @param qualifier Qualifier.
* @param type Type.
*/
public WoofTeamModel(
int teamSize
, String teamSourceClassName
, String qualifier
, String type
) {
this.teamSize = teamSize;
this.teamSourceClassName = teamSourceClassName;
this.qualifier = qualifier;
this.type = type;
}
/**
* Convenience constructor for new non-linked instance allowing XY initialising.
*
* @param teamSize Team size.
* @param teamSourceClassName Team source class name.
* @param qualifier Qualifier.
* @param type Type.
* @param x Horizontal location.
* @param y Vertical location.
*/
public WoofTeamModel(
int teamSize
, String teamSourceClassName
, String qualifier
, String type
, int x
, int y
) {
this.teamSize = teamSize;
this.teamSourceClassName = teamSourceClassName;
this.qualifier = qualifier;
this.type = type;
this.setX(x);
this.setY(y);
}
/**
* Convenience constructor.
*
* @param teamSize Team size.
* @param teamSourceClassName Team source class name.
* @param qualifier Qualifier.
* @param type Type.
* @param propertySource Property source.
* @param typeQualification Type qualification.
*/
public WoofTeamModel(
int teamSize
, String teamSourceClassName
, String qualifier
, String type
, PropertySourceModel[] propertySource
, TypeQualificationModel[] typeQualification
) {
this.teamSize = teamSize;
this.teamSourceClassName = teamSourceClassName;
this.qualifier = qualifier;
this.type = type;
if (propertySource != null) {
for (PropertySourceModel model : propertySource) {
this.propertySource.add(model);
}
}
if (typeQualification != null) {
for (TypeQualificationModel model : typeQualification) {
this.typeQualification.add(model);
}
}
}
/**
* Convenience constructor allowing XY initialising.
*
* @param teamSize Team size.
* @param teamSourceClassName Team source class name.
* @param qualifier Qualifier.
* @param type Type.
* @param propertySource Property source.
* @param typeQualification Type qualification.
* @param x Horizontal location.
* @param y Vertical location.
*/
public WoofTeamModel(
int teamSize
, String teamSourceClassName
, String qualifier
, String type
, PropertySourceModel[] propertySource
, TypeQualificationModel[] typeQualification
, int x
, int y
) {
this.teamSize = teamSize;
this.teamSourceClassName = teamSourceClassName;
this.qualifier = qualifier;
this.type = type;
if (propertySource != null) {
for (PropertySourceModel model : propertySource) {
this.propertySource.add(model);
}
}
if (typeQualification != null) {
for (TypeQualificationModel model : typeQualification) {
this.typeQualification.add(model);
}
}
this.setX(x);
this.setY(y);
}
/**
* Team size.
*/
private int teamSize;
/**
* @return Team size.
*/
public int getTeamSize() {
return this.teamSize;
}
/**
* @param teamSize Team size.
*/
public void setTeamSize(int teamSize) {
int oldValue = this.teamSize;
this.teamSize = teamSize;
this.changeField(oldValue, this.teamSize, WoofTeamEvent.CHANGE_TEAM_SIZE);
}
/**
* Team source class name.
*/
private String teamSourceClassName;
/**
* @return Team source class name.
*/
public String getTeamSourceClassName() {
return this.teamSourceClassName;
}
/**
* @param teamSourceClassName Team source class name.
*/
public void setTeamSourceClassName(String teamSourceClassName) {
String oldValue = this.teamSourceClassName;
this.teamSourceClassName = teamSourceClassName;
this.changeField(oldValue, this.teamSourceClassName, WoofTeamEvent.CHANGE_TEAM_SOURCE_CLASS_NAME);
}
/**
* Qualifier.
*/
private String qualifier;
/**
* @return Qualifier.
*/
public String getQualifier() {
return this.qualifier;
}
/**
* @param qualifier Qualifier.
*/
public void setQualifier(String qualifier) {
String oldValue = this.qualifier;
this.qualifier = qualifier;
this.changeField(oldValue, this.qualifier, WoofTeamEvent.CHANGE_QUALIFIER);
}
/**
* Type.
*/
private String type;
/**
* @return Type.
*/
public String getType() {
return this.type;
}
/**
* @param type Type.
*/
public void setType(String type) {
String oldValue = this.type;
this.type = type;
this.changeField(oldValue, this.type, WoofTeamEvent.CHANGE_TYPE);
}
/**
* Property source.
*/
private List propertySource = new LinkedList();
/**
* @return Property source.
*/
public List getPropertySources() {
return this.propertySource;
}
/**
* @param propertySource Property source.
*/
public void addPropertySource(PropertySourceModel propertySource) {
this.addItemToList(propertySource, this.propertySource, WoofTeamEvent.ADD_PROPERTY_SOURCE);
}
/**
* @param propertySource Property source.
*/
public void removePropertySource(PropertySourceModel propertySource) {
this.removeItemFromList(propertySource, this.propertySource, WoofTeamEvent.REMOVE_PROPERTY_SOURCE);
}
/**
* Type qualification.
*/
private List typeQualification = new LinkedList();
/**
* @return Type qualification.
*/
public List getTypeQualifications() {
return this.typeQualification;
}
/**
* @param typeQualification Type qualification.
*/
public void addTypeQualification(TypeQualificationModel typeQualification) {
this.addItemToList(typeQualification, this.typeQualification, WoofTeamEvent.ADD_TYPE_QUALIFICATION);
}
/**
* @param typeQualification Type qualification.
*/
public void removeTypeQualification(TypeQualificationModel typeQualification) {
this.removeItemFromList(typeQualification, this.typeQualification, WoofTeamEvent.REMOVE_TYPE_QUALIFICATION);
}
/**
* Remove Connections.
*
* @return {@link RemoveConnectionsAction} to remove the {@link ConnectionModel} instances.
*/
public RemoveConnectionsAction removeConnections() {
RemoveConnectionsAction _action = new RemoveConnectionsAction(this);
return _action;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy