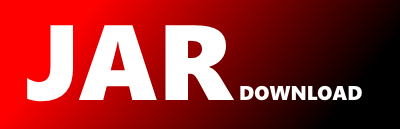
net.officefloor.woof.model.woof.WoofHttpInputToWoofHttpContinuationModel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of officeweb_configuration Show documentation
Show all versions of officeweb_configuration Show documentation
Configuration for WoOF (Web on OfficeFloor)
/*
*
*/
package net.officefloor.woof.model.woof;
import javax.annotation.Generated;
import net.officefloor.model.AbstractModel;
import net.officefloor.model.ConnectionModel;
@Generated("net.officefloor.model.generate.ModelGenerator")
public class WoofHttpInputToWoofHttpContinuationModel extends AbstractModel implements ConnectionModel {
public static enum WoofHttpInputToWoofHttpContinuationEvent {
CHANGE_APPLICATION_PATH, CHANGE_WOOF_HTTP_INPUT, CHANGE_WOOF_HTTP_CONTINUATION
}
/**
* Default constructor.
*/
public WoofHttpInputToWoofHttpContinuationModel() {
}
/**
* Convenience constructor for new non-linked instance.
*
* @param applicationPath Application path.
*/
public WoofHttpInputToWoofHttpContinuationModel(
String applicationPath
) {
this.applicationPath = applicationPath;
}
/**
* Convenience constructor for new non-linked instance allowing XY initialising.
*
* @param applicationPath Application path.
* @param x Horizontal location.
* @param y Vertical location.
*/
public WoofHttpInputToWoofHttpContinuationModel(
String applicationPath
, int x
, int y
) {
this.applicationPath = applicationPath;
this.setX(x);
this.setY(y);
}
/**
* Convenience constructor.
*
* @param applicationPath Application path.
* @param woofHttpInput Woof http input.
* @param woofHttpContinuation Woof http continuation.
*/
public WoofHttpInputToWoofHttpContinuationModel(
String applicationPath
, WoofHttpInputModel woofHttpInput
, WoofHttpContinuationModel woofHttpContinuation
) {
this.applicationPath = applicationPath;
this.woofHttpInput = woofHttpInput;
this.woofHttpContinuation = woofHttpContinuation;
}
/**
* Convenience constructor allowing XY initialising.
*
* @param applicationPath Application path.
* @param woofHttpInput Woof http input.
* @param woofHttpContinuation Woof http continuation.
* @param x Horizontal location.
* @param y Vertical location.
*/
public WoofHttpInputToWoofHttpContinuationModel(
String applicationPath
, WoofHttpInputModel woofHttpInput
, WoofHttpContinuationModel woofHttpContinuation
, int x
, int y
) {
this.applicationPath = applicationPath;
this.woofHttpInput = woofHttpInput;
this.woofHttpContinuation = woofHttpContinuation;
this.setX(x);
this.setY(y);
}
/**
* Application path.
*/
private String applicationPath;
/**
* @return Application path.
*/
public String getApplicationPath() {
return this.applicationPath;
}
/**
* @param applicationPath Application path.
*/
public void setApplicationPath(String applicationPath) {
String oldValue = this.applicationPath;
this.applicationPath = applicationPath;
this.changeField(oldValue, this.applicationPath, WoofHttpInputToWoofHttpContinuationEvent.CHANGE_APPLICATION_PATH);
}
/**
* Woof http input.
*/
private WoofHttpInputModel woofHttpInput;
/**
* @return Woof http input.
*/
public WoofHttpInputModel getWoofHttpInput() {
return this.woofHttpInput;
}
/**
* @param woofHttpInput Woof http input.
*/
public void setWoofHttpInput(WoofHttpInputModel woofHttpInput) {
WoofHttpInputModel oldValue = this.woofHttpInput;
this.woofHttpInput = woofHttpInput;
this.changeField(oldValue, this.woofHttpInput, WoofHttpInputToWoofHttpContinuationEvent.CHANGE_WOOF_HTTP_INPUT);
}
/**
* Woof http continuation.
*/
private WoofHttpContinuationModel woofHttpContinuation;
/**
* @return Woof http continuation.
*/
public WoofHttpContinuationModel getWoofHttpContinuation() {
return this.woofHttpContinuation;
}
/**
* @param woofHttpContinuation Woof http continuation.
*/
public void setWoofHttpContinuation(WoofHttpContinuationModel woofHttpContinuation) {
WoofHttpContinuationModel oldValue = this.woofHttpContinuation;
this.woofHttpContinuation = woofHttpContinuation;
this.changeField(oldValue, this.woofHttpContinuation, WoofHttpInputToWoofHttpContinuationEvent.CHANGE_WOOF_HTTP_CONTINUATION);
}
/**
* @return Indicates if removable.
*/
public boolean isRemovable() {
return true;
}
/**
* Connects to the {@link AbstractModel} instances.
*/
public void connect() {
this.woofHttpInput.setWoofHttpContinuation(this);
this.woofHttpContinuation.addWoofHttpInput(this);
}
/**
* Removes connection to the {@link AbstractModel} instances.
*/
public void remove() {
this.woofHttpInput.setWoofHttpContinuation(null);
this.woofHttpContinuation.removeWoofHttpInput(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy