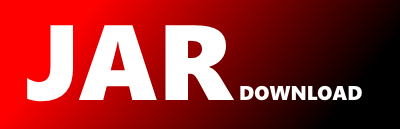
net.officefloor.woof.model.woof.WoofSectionInputModel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of officeweb_configuration Show documentation
Show all versions of officeweb_configuration Show documentation
Configuration for WoOF (Web on OfficeFloor)
/*
*
*/
package net.officefloor.woof.model.woof;
import java.util.List;
import java.util.LinkedList;
import javax.annotation.Generated;
import net.officefloor.model.AbstractModel;
import net.officefloor.model.ConnectionModel;
import net.officefloor.model.ItemModel;
import net.officefloor.model.RemoveConnectionsAction;
@Generated("net.officefloor.model.generate.ModelGenerator")
public class WoofSectionInputModel extends AbstractModel implements ItemModel {
public static enum WoofSectionInputEvent {
CHANGE_WOOF_SECTION_INPUT_NAME, CHANGE_PARAMETER_TYPE, ADD_WOOF_TEMPLATE_OUTPUT, REMOVE_WOOF_TEMPLATE_OUTPUT, ADD_WOOF_SECURITY_OUTPUT, REMOVE_WOOF_SECURITY_OUTPUT, ADD_WOOF_SECTION_OUTPUT, REMOVE_WOOF_SECTION_OUTPUT, ADD_WOOF_PROCEDURE_OUTPUT, REMOVE_WOOF_PROCEDURE_OUTPUT, ADD_WOOF_PROCEDURE_NEXT, REMOVE_WOOF_PROCEDURE_NEXT, ADD_WOOF_EXCEPTION, REMOVE_WOOF_EXCEPTION, ADD_WOOF_HTTP_CONTINUATION, REMOVE_WOOF_HTTP_CONTINUATION, ADD_WOOF_HTTP_INPUT, REMOVE_WOOF_HTTP_INPUT, ADD_WOOF_START, REMOVE_WOOF_START
}
/**
* Default constructor.
*/
public WoofSectionInputModel() {
}
/**
* Convenience constructor for new non-linked instance.
*
* @param woofSectionInputName Woof section input name.
* @param parameterType Parameter type.
*/
public WoofSectionInputModel(
String woofSectionInputName
, String parameterType
) {
this.woofSectionInputName = woofSectionInputName;
this.parameterType = parameterType;
}
/**
* Convenience constructor for new non-linked instance allowing XY initialising.
*
* @param woofSectionInputName Woof section input name.
* @param parameterType Parameter type.
* @param x Horizontal location.
* @param y Vertical location.
*/
public WoofSectionInputModel(
String woofSectionInputName
, String parameterType
, int x
, int y
) {
this.woofSectionInputName = woofSectionInputName;
this.parameterType = parameterType;
this.setX(x);
this.setY(y);
}
/**
* Convenience constructor.
*
* @param woofSectionInputName Woof section input name.
* @param parameterType Parameter type.
* @param woofTemplateOutput Woof template output.
* @param woofSecurityOutput Woof security output.
* @param woofSectionOutput Woof section output.
* @param woofProcedureOutput Woof procedure output.
* @param woofProcedureNext Woof procedure next.
* @param woofException Woof exception.
* @param woofHttpContinuation Woof http continuation.
* @param woofHttpInput Woof http input.
* @param woofStart Woof start.
*/
public WoofSectionInputModel(
String woofSectionInputName
, String parameterType
, WoofTemplateOutputToWoofSectionInputModel[] woofTemplateOutput
, WoofSecurityOutputToWoofSectionInputModel[] woofSecurityOutput
, WoofSectionOutputToWoofSectionInputModel[] woofSectionOutput
, WoofProcedureOutputToWoofSectionInputModel[] woofProcedureOutput
, WoofProcedureNextToWoofSectionInputModel[] woofProcedureNext
, WoofExceptionToWoofSectionInputModel[] woofException
, WoofHttpContinuationToWoofSectionInputModel[] woofHttpContinuation
, WoofHttpInputToWoofSectionInputModel[] woofHttpInput
, WoofStartToWoofSectionInputModel[] woofStart
) {
this.woofSectionInputName = woofSectionInputName;
this.parameterType = parameterType;
if (woofTemplateOutput != null) {
for (WoofTemplateOutputToWoofSectionInputModel model : woofTemplateOutput) {
this.woofTemplateOutput.add(model);
}
}
if (woofSecurityOutput != null) {
for (WoofSecurityOutputToWoofSectionInputModel model : woofSecurityOutput) {
this.woofSecurityOutput.add(model);
}
}
if (woofSectionOutput != null) {
for (WoofSectionOutputToWoofSectionInputModel model : woofSectionOutput) {
this.woofSectionOutput.add(model);
}
}
if (woofProcedureOutput != null) {
for (WoofProcedureOutputToWoofSectionInputModel model : woofProcedureOutput) {
this.woofProcedureOutput.add(model);
}
}
if (woofProcedureNext != null) {
for (WoofProcedureNextToWoofSectionInputModel model : woofProcedureNext) {
this.woofProcedureNext.add(model);
}
}
if (woofException != null) {
for (WoofExceptionToWoofSectionInputModel model : woofException) {
this.woofException.add(model);
}
}
if (woofHttpContinuation != null) {
for (WoofHttpContinuationToWoofSectionInputModel model : woofHttpContinuation) {
this.woofHttpContinuation.add(model);
}
}
if (woofHttpInput != null) {
for (WoofHttpInputToWoofSectionInputModel model : woofHttpInput) {
this.woofHttpInput.add(model);
}
}
if (woofStart != null) {
for (WoofStartToWoofSectionInputModel model : woofStart) {
this.woofStart.add(model);
}
}
}
/**
* Convenience constructor allowing XY initialising.
*
* @param woofSectionInputName Woof section input name.
* @param parameterType Parameter type.
* @param woofTemplateOutput Woof template output.
* @param woofSecurityOutput Woof security output.
* @param woofSectionOutput Woof section output.
* @param woofProcedureOutput Woof procedure output.
* @param woofProcedureNext Woof procedure next.
* @param woofException Woof exception.
* @param woofHttpContinuation Woof http continuation.
* @param woofHttpInput Woof http input.
* @param woofStart Woof start.
* @param x Horizontal location.
* @param y Vertical location.
*/
public WoofSectionInputModel(
String woofSectionInputName
, String parameterType
, WoofTemplateOutputToWoofSectionInputModel[] woofTemplateOutput
, WoofSecurityOutputToWoofSectionInputModel[] woofSecurityOutput
, WoofSectionOutputToWoofSectionInputModel[] woofSectionOutput
, WoofProcedureOutputToWoofSectionInputModel[] woofProcedureOutput
, WoofProcedureNextToWoofSectionInputModel[] woofProcedureNext
, WoofExceptionToWoofSectionInputModel[] woofException
, WoofHttpContinuationToWoofSectionInputModel[] woofHttpContinuation
, WoofHttpInputToWoofSectionInputModel[] woofHttpInput
, WoofStartToWoofSectionInputModel[] woofStart
, int x
, int y
) {
this.woofSectionInputName = woofSectionInputName;
this.parameterType = parameterType;
if (woofTemplateOutput != null) {
for (WoofTemplateOutputToWoofSectionInputModel model : woofTemplateOutput) {
this.woofTemplateOutput.add(model);
}
}
if (woofSecurityOutput != null) {
for (WoofSecurityOutputToWoofSectionInputModel model : woofSecurityOutput) {
this.woofSecurityOutput.add(model);
}
}
if (woofSectionOutput != null) {
for (WoofSectionOutputToWoofSectionInputModel model : woofSectionOutput) {
this.woofSectionOutput.add(model);
}
}
if (woofProcedureOutput != null) {
for (WoofProcedureOutputToWoofSectionInputModel model : woofProcedureOutput) {
this.woofProcedureOutput.add(model);
}
}
if (woofProcedureNext != null) {
for (WoofProcedureNextToWoofSectionInputModel model : woofProcedureNext) {
this.woofProcedureNext.add(model);
}
}
if (woofException != null) {
for (WoofExceptionToWoofSectionInputModel model : woofException) {
this.woofException.add(model);
}
}
if (woofHttpContinuation != null) {
for (WoofHttpContinuationToWoofSectionInputModel model : woofHttpContinuation) {
this.woofHttpContinuation.add(model);
}
}
if (woofHttpInput != null) {
for (WoofHttpInputToWoofSectionInputModel model : woofHttpInput) {
this.woofHttpInput.add(model);
}
}
if (woofStart != null) {
for (WoofStartToWoofSectionInputModel model : woofStart) {
this.woofStart.add(model);
}
}
this.setX(x);
this.setY(y);
}
/**
* Woof section input name.
*/
private String woofSectionInputName;
/**
* @return Woof section input name.
*/
public String getWoofSectionInputName() {
return this.woofSectionInputName;
}
/**
* @param woofSectionInputName Woof section input name.
*/
public void setWoofSectionInputName(String woofSectionInputName) {
String oldValue = this.woofSectionInputName;
this.woofSectionInputName = woofSectionInputName;
this.changeField(oldValue, this.woofSectionInputName, WoofSectionInputEvent.CHANGE_WOOF_SECTION_INPUT_NAME);
}
/**
* Parameter type.
*/
private String parameterType;
/**
* @return Parameter type.
*/
public String getParameterType() {
return this.parameterType;
}
/**
* @param parameterType Parameter type.
*/
public void setParameterType(String parameterType) {
String oldValue = this.parameterType;
this.parameterType = parameterType;
this.changeField(oldValue, this.parameterType, WoofSectionInputEvent.CHANGE_PARAMETER_TYPE);
}
/**
* Woof template output.
*/
private List woofTemplateOutput = new LinkedList();
/**
* @return Woof template output.
*/
public List getWoofTemplateOutputs() {
return this.woofTemplateOutput;
}
/**
* @param woofTemplateOutput Woof template output.
*/
public void addWoofTemplateOutput(WoofTemplateOutputToWoofSectionInputModel woofTemplateOutput) {
this.addItemToList(woofTemplateOutput, this.woofTemplateOutput, WoofSectionInputEvent.ADD_WOOF_TEMPLATE_OUTPUT);
}
/**
* @param woofTemplateOutput Woof template output.
*/
public void removeWoofTemplateOutput(WoofTemplateOutputToWoofSectionInputModel woofTemplateOutput) {
this.removeItemFromList(woofTemplateOutput, this.woofTemplateOutput, WoofSectionInputEvent.REMOVE_WOOF_TEMPLATE_OUTPUT);
}
/**
* Woof security output.
*/
private List woofSecurityOutput = new LinkedList();
/**
* @return Woof security output.
*/
public List getWoofSecurityOutputs() {
return this.woofSecurityOutput;
}
/**
* @param woofSecurityOutput Woof security output.
*/
public void addWoofSecurityOutput(WoofSecurityOutputToWoofSectionInputModel woofSecurityOutput) {
this.addItemToList(woofSecurityOutput, this.woofSecurityOutput, WoofSectionInputEvent.ADD_WOOF_SECURITY_OUTPUT);
}
/**
* @param woofSecurityOutput Woof security output.
*/
public void removeWoofSecurityOutput(WoofSecurityOutputToWoofSectionInputModel woofSecurityOutput) {
this.removeItemFromList(woofSecurityOutput, this.woofSecurityOutput, WoofSectionInputEvent.REMOVE_WOOF_SECURITY_OUTPUT);
}
/**
* Woof section output.
*/
private List woofSectionOutput = new LinkedList();
/**
* @return Woof section output.
*/
public List getWoofSectionOutputs() {
return this.woofSectionOutput;
}
/**
* @param woofSectionOutput Woof section output.
*/
public void addWoofSectionOutput(WoofSectionOutputToWoofSectionInputModel woofSectionOutput) {
this.addItemToList(woofSectionOutput, this.woofSectionOutput, WoofSectionInputEvent.ADD_WOOF_SECTION_OUTPUT);
}
/**
* @param woofSectionOutput Woof section output.
*/
public void removeWoofSectionOutput(WoofSectionOutputToWoofSectionInputModel woofSectionOutput) {
this.removeItemFromList(woofSectionOutput, this.woofSectionOutput, WoofSectionInputEvent.REMOVE_WOOF_SECTION_OUTPUT);
}
/**
* Woof procedure output.
*/
private List woofProcedureOutput = new LinkedList();
/**
* @return Woof procedure output.
*/
public List getWoofProcedureOutputs() {
return this.woofProcedureOutput;
}
/**
* @param woofProcedureOutput Woof procedure output.
*/
public void addWoofProcedureOutput(WoofProcedureOutputToWoofSectionInputModel woofProcedureOutput) {
this.addItemToList(woofProcedureOutput, this.woofProcedureOutput, WoofSectionInputEvent.ADD_WOOF_PROCEDURE_OUTPUT);
}
/**
* @param woofProcedureOutput Woof procedure output.
*/
public void removeWoofProcedureOutput(WoofProcedureOutputToWoofSectionInputModel woofProcedureOutput) {
this.removeItemFromList(woofProcedureOutput, this.woofProcedureOutput, WoofSectionInputEvent.REMOVE_WOOF_PROCEDURE_OUTPUT);
}
/**
* Woof procedure next.
*/
private List woofProcedureNext = new LinkedList();
/**
* @return Woof procedure next.
*/
public List getWoofProcedureNexts() {
return this.woofProcedureNext;
}
/**
* @param woofProcedureNext Woof procedure next.
*/
public void addWoofProcedureNext(WoofProcedureNextToWoofSectionInputModel woofProcedureNext) {
this.addItemToList(woofProcedureNext, this.woofProcedureNext, WoofSectionInputEvent.ADD_WOOF_PROCEDURE_NEXT);
}
/**
* @param woofProcedureNext Woof procedure next.
*/
public void removeWoofProcedureNext(WoofProcedureNextToWoofSectionInputModel woofProcedureNext) {
this.removeItemFromList(woofProcedureNext, this.woofProcedureNext, WoofSectionInputEvent.REMOVE_WOOF_PROCEDURE_NEXT);
}
/**
* Woof exception.
*/
private List woofException = new LinkedList();
/**
* @return Woof exception.
*/
public List getWoofExceptions() {
return this.woofException;
}
/**
* @param woofException Woof exception.
*/
public void addWoofException(WoofExceptionToWoofSectionInputModel woofException) {
this.addItemToList(woofException, this.woofException, WoofSectionInputEvent.ADD_WOOF_EXCEPTION);
}
/**
* @param woofException Woof exception.
*/
public void removeWoofException(WoofExceptionToWoofSectionInputModel woofException) {
this.removeItemFromList(woofException, this.woofException, WoofSectionInputEvent.REMOVE_WOOF_EXCEPTION);
}
/**
* Woof http continuation.
*/
private List woofHttpContinuation = new LinkedList();
/**
* @return Woof http continuation.
*/
public List getWoofHttpContinuations() {
return this.woofHttpContinuation;
}
/**
* @param woofHttpContinuation Woof http continuation.
*/
public void addWoofHttpContinuation(WoofHttpContinuationToWoofSectionInputModel woofHttpContinuation) {
this.addItemToList(woofHttpContinuation, this.woofHttpContinuation, WoofSectionInputEvent.ADD_WOOF_HTTP_CONTINUATION);
}
/**
* @param woofHttpContinuation Woof http continuation.
*/
public void removeWoofHttpContinuation(WoofHttpContinuationToWoofSectionInputModel woofHttpContinuation) {
this.removeItemFromList(woofHttpContinuation, this.woofHttpContinuation, WoofSectionInputEvent.REMOVE_WOOF_HTTP_CONTINUATION);
}
/**
* Woof http input.
*/
private List woofHttpInput = new LinkedList();
/**
* @return Woof http input.
*/
public List getWoofHttpInputs() {
return this.woofHttpInput;
}
/**
* @param woofHttpInput Woof http input.
*/
public void addWoofHttpInput(WoofHttpInputToWoofSectionInputModel woofHttpInput) {
this.addItemToList(woofHttpInput, this.woofHttpInput, WoofSectionInputEvent.ADD_WOOF_HTTP_INPUT);
}
/**
* @param woofHttpInput Woof http input.
*/
public void removeWoofHttpInput(WoofHttpInputToWoofSectionInputModel woofHttpInput) {
this.removeItemFromList(woofHttpInput, this.woofHttpInput, WoofSectionInputEvent.REMOVE_WOOF_HTTP_INPUT);
}
/**
* Woof start.
*/
private List woofStart = new LinkedList();
/**
* @return Woof start.
*/
public List getWoofStarts() {
return this.woofStart;
}
/**
* @param woofStart Woof start.
*/
public void addWoofStart(WoofStartToWoofSectionInputModel woofStart) {
this.addItemToList(woofStart, this.woofStart, WoofSectionInputEvent.ADD_WOOF_START);
}
/**
* @param woofStart Woof start.
*/
public void removeWoofStart(WoofStartToWoofSectionInputModel woofStart) {
this.removeItemFromList(woofStart, this.woofStart, WoofSectionInputEvent.REMOVE_WOOF_START);
}
/**
* Remove Connections.
*
* @return {@link RemoveConnectionsAction} to remove the {@link ConnectionModel} instances.
*/
public RemoveConnectionsAction removeConnections() {
RemoveConnectionsAction _action = new RemoveConnectionsAction(this);
_action.disconnect(this.woofTemplateOutput);
_action.disconnect(this.woofSecurityOutput);
_action.disconnect(this.woofSectionOutput);
_action.disconnect(this.woofProcedureOutput);
_action.disconnect(this.woofProcedureNext);
_action.disconnect(this.woofException);
_action.disconnect(this.woofHttpContinuation);
_action.disconnect(this.woofHttpInput);
_action.disconnect(this.woofStart);
return _action;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy