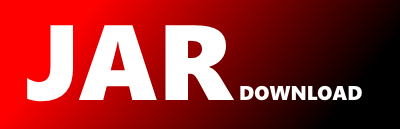
net.officefloor.woof.model.woof.WoofTemplateOutputToWoofSectionInputModel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of officeweb_configuration Show documentation
Show all versions of officeweb_configuration Show documentation
Configuration for WoOF (Web on OfficeFloor)
/*
*
*/
package net.officefloor.woof.model.woof;
import javax.annotation.Generated;
import net.officefloor.model.AbstractModel;
import net.officefloor.model.ConnectionModel;
@Generated("net.officefloor.model.generate.ModelGenerator")
public class WoofTemplateOutputToWoofSectionInputModel extends AbstractModel implements ConnectionModel {
public static enum WoofTemplateOutputToWoofSectionInputEvent {
CHANGE_SECTION_NAME, CHANGE_INPUT_NAME, CHANGE_WOOF_TEMPLATE_OUTPUT, CHANGE_WOOF_SECTION_INPUT
}
/**
* Default constructor.
*/
public WoofTemplateOutputToWoofSectionInputModel() {
}
/**
* Convenience constructor for new non-linked instance.
*
* @param sectionName Section name.
* @param inputName Input name.
*/
public WoofTemplateOutputToWoofSectionInputModel(
String sectionName
, String inputName
) {
this.sectionName = sectionName;
this.inputName = inputName;
}
/**
* Convenience constructor for new non-linked instance allowing XY initialising.
*
* @param sectionName Section name.
* @param inputName Input name.
* @param x Horizontal location.
* @param y Vertical location.
*/
public WoofTemplateOutputToWoofSectionInputModel(
String sectionName
, String inputName
, int x
, int y
) {
this.sectionName = sectionName;
this.inputName = inputName;
this.setX(x);
this.setY(y);
}
/**
* Convenience constructor.
*
* @param sectionName Section name.
* @param inputName Input name.
* @param woofTemplateOutput Woof template output.
* @param woofSectionInput Woof section input.
*/
public WoofTemplateOutputToWoofSectionInputModel(
String sectionName
, String inputName
, WoofTemplateOutputModel woofTemplateOutput
, WoofSectionInputModel woofSectionInput
) {
this.sectionName = sectionName;
this.inputName = inputName;
this.woofTemplateOutput = woofTemplateOutput;
this.woofSectionInput = woofSectionInput;
}
/**
* Convenience constructor allowing XY initialising.
*
* @param sectionName Section name.
* @param inputName Input name.
* @param woofTemplateOutput Woof template output.
* @param woofSectionInput Woof section input.
* @param x Horizontal location.
* @param y Vertical location.
*/
public WoofTemplateOutputToWoofSectionInputModel(
String sectionName
, String inputName
, WoofTemplateOutputModel woofTemplateOutput
, WoofSectionInputModel woofSectionInput
, int x
, int y
) {
this.sectionName = sectionName;
this.inputName = inputName;
this.woofTemplateOutput = woofTemplateOutput;
this.woofSectionInput = woofSectionInput;
this.setX(x);
this.setY(y);
}
/**
* Section name.
*/
private String sectionName;
/**
* @return Section name.
*/
public String getSectionName() {
return this.sectionName;
}
/**
* @param sectionName Section name.
*/
public void setSectionName(String sectionName) {
String oldValue = this.sectionName;
this.sectionName = sectionName;
this.changeField(oldValue, this.sectionName, WoofTemplateOutputToWoofSectionInputEvent.CHANGE_SECTION_NAME);
}
/**
* Input name.
*/
private String inputName;
/**
* @return Input name.
*/
public String getInputName() {
return this.inputName;
}
/**
* @param inputName Input name.
*/
public void setInputName(String inputName) {
String oldValue = this.inputName;
this.inputName = inputName;
this.changeField(oldValue, this.inputName, WoofTemplateOutputToWoofSectionInputEvent.CHANGE_INPUT_NAME);
}
/**
* Woof template output.
*/
private WoofTemplateOutputModel woofTemplateOutput;
/**
* @return Woof template output.
*/
public WoofTemplateOutputModel getWoofTemplateOutput() {
return this.woofTemplateOutput;
}
/**
* @param woofTemplateOutput Woof template output.
*/
public void setWoofTemplateOutput(WoofTemplateOutputModel woofTemplateOutput) {
WoofTemplateOutputModel oldValue = this.woofTemplateOutput;
this.woofTemplateOutput = woofTemplateOutput;
this.changeField(oldValue, this.woofTemplateOutput, WoofTemplateOutputToWoofSectionInputEvent.CHANGE_WOOF_TEMPLATE_OUTPUT);
}
/**
* Woof section input.
*/
private WoofSectionInputModel woofSectionInput;
/**
* @return Woof section input.
*/
public WoofSectionInputModel getWoofSectionInput() {
return this.woofSectionInput;
}
/**
* @param woofSectionInput Woof section input.
*/
public void setWoofSectionInput(WoofSectionInputModel woofSectionInput) {
WoofSectionInputModel oldValue = this.woofSectionInput;
this.woofSectionInput = woofSectionInput;
this.changeField(oldValue, this.woofSectionInput, WoofTemplateOutputToWoofSectionInputEvent.CHANGE_WOOF_SECTION_INPUT);
}
/**
* @return Indicates if removable.
*/
public boolean isRemovable() {
return true;
}
/**
* Connects to the {@link AbstractModel} instances.
*/
public void connect() {
this.woofTemplateOutput.setWoofSectionInput(this);
this.woofSectionInput.addWoofTemplateOutput(this);
}
/**
* Removes connection to the {@link AbstractModel} instances.
*/
public void remove() {
this.woofTemplateOutput.setWoofSectionInput(null);
this.woofSectionInput.removeWoofTemplateOutput(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy