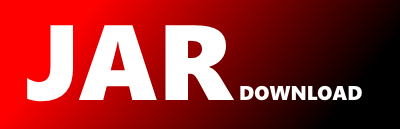
org.reflections8.util.HashSetMultimap Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of reflections8 Show documentation
Show all versions of reflections8 Show documentation
Reflections8 - a Java runtime metadata analysis without guava on Java 8
package org.reflections8.util;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Map;
import java.util.Set;
import java.util.function.Supplier;
/**
* Helper class used to avoid guava
*
* @author aschoerk
*/
public class HashSetMultimap extends HashMap> implements SetMultimap {
private static final long serialVersionUID = 140511307437539771L;
private transient final Supplier> setSupplier;
public HashSetMultimap() {
setSupplier = () -> new HashSet<>();
}
public HashSetMultimap(Supplier> setSupplier) {
this.setSupplier = setSupplier;
}
@Override
public boolean putSingle(T key, V value) {
Set vs = super.get(key);
if (vs != null) {
return vs.add(value);
} else {
Set setValue = setSupplier.get();
setValue.add(value);
super.put(key, setValue);
return true;
}
}
@Override
public void putAllSingles(SetMultimap m) {
for (T key: m.keySet()) {
Set val = m.get(key);
Set vs = super.get(key);
if(vs != null) {
if (val != null) {
vs.addAll(val);
}
}
else {
if (val == null) {
super.put(key, null);
} else {
Set setValue = setSupplier.get();
if(val != null)
setValue.addAll(m.get(key));
super.put(key, setValue);
}
}
}
}
@Override
public boolean removeSingle(Object key, V value) {
Set vs = super.get(key);
if (vs == null)
return false;
else {
boolean res = vs.remove(value);
if (vs.isEmpty()) {
super.remove(key);
}
return res;
}
}
@Override
public Collection flatValues() {
ArrayList res = new ArrayList<>();
for (Set s: values()) {
res.addAll(s);
}
return res;
}
@Override
public Set flatValuesAsSet() {
HashSet res = new HashSet<>();
for (Set s: values()) {
res.addAll(s);
}
return res;
}
@Override
public Map> asMap() {
Map> result = new HashMap<>();
for (Entry> e: entrySet()) {
if (e.getValue() != null && !e.getValue().isEmpty())
result.put(e.getKey(), e.getValue());
}
return result;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy