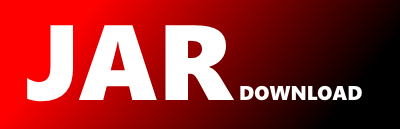
org.reflections8.util.ReflectionsIterables Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of reflections8 Show documentation
Show all versions of reflections8 Show documentation
Reflections8 - a Java runtime metadata analysis without guava on Java 8
package org.reflections8.util;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.NoSuchElementException;
import java.util.Set;
import java.util.function.Function;
/**
* @author aschoerk
*/
public class ReflectionsIterables {
public static boolean isEmpty(Iterable> iterable) {
return iterable == null || !iterable.iterator().hasNext();
}
public static T getOnlyElement(Iterable iterable) {
if (iterable == null)
throw new NoSuchElementException();
final Iterator iterator = iterable.iterator();
if (!iterator.hasNext())
throw new NoSuchElementException();
T res = iterator.next();
if (iterator.hasNext())
throw new IllegalArgumentException();
return res;
}
public static Iterable concat(Iterable extends T> a,
Iterable extends T> b) {
List res = new ArrayList<>();
for (T aEl: a) {
res.add(aEl);
}
for (T bEl: b) {
res.add(bEl);
}
return res;
}
public static Set makeSetOf(Iterable extends T> a) {
HashSet res = new HashSet<>();
for (T aEl: a) {
res.add(aEl);
}
return res;
}
public static boolean contains(Iterable> iterable, Object element) {
for (Object el: iterable) {
if (el.equals(element))
return true;
}
return false;
}
public static Iterable transform(Iterable fromIterable,
Function super F,? extends T> function) {
final ArrayList res = new ArrayList<>();
if (fromIterable != null) {
for (F el : fromIterable) {
res.add(function.apply(el));
}
}
return res;
}
public static Set transformToSet(Iterable fromIterable,
Function super F,? extends T> function) {
Set res = new HashSet<>();
if (fromIterable != null) {
for (F el : fromIterable) {
res.add(function.apply(el));
}
}
return res;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy