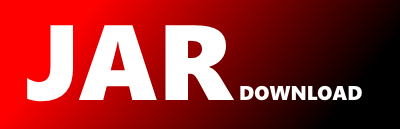
net.oneandone.sushi.util.Lcs Maven / Gradle / Ivy
/**
* Copyright 1&1 Internet AG, https://github.com/1and1/
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package net.oneandone.sushi.util;
import java.util.ArrayList;
import java.util.List;
/** Longest common subsequence. http://en.wikipedia.org/wiki/Diff */
public class Lcs {
private static final List> EMPTY = new ArrayList<>(0);
public static List compute(List vert, List hor) {
List[] previous;
List[] current;
previous = new List[hor.size() + 1];
for (int i = 0; i < previous.length; i++) {
previous[i] = (List) EMPTY;
}
for (T value : vert) {
current = new List[hor.size() + 1];
current[0] = (List) EMPTY;
for (int i = 1; i < current.length; i++) {
if (value.equals(hor.get(i - 1))) {
current[i] = append(previous[i - 1], value);
} else {
current[i] = longest(current[i - 1], previous[i]);
}
}
previous = current;
}
return previous[previous.length - 1];
}
private static List append(List lst, T value) {
List result;
result = new ArrayList<>(lst.size() + 1);
result.addAll(lst);
result.add(value);
return result;
}
private static List longest(List a, List b) {
return new ArrayList<>(a.size() >= b.size() ? a : b);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy