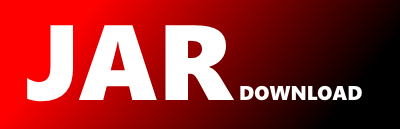
com.artemis.ComponentPool Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of artemis-odb Show documentation
Show all versions of artemis-odb Show documentation
Fork of Artemis Entity System Framework.
package com.artemis;
import java.util.IdentityHashMap;
import java.util.Map;
import com.artemis.utils.Bag;
import com.artemis.utils.reflect.ClassReflection;
import com.artemis.utils.reflect.ReflectionException;
class ComponentPool {
private Bag pools;
ComponentPool() {
pools = new Bag();
}
@SuppressWarnings("unchecked")
T obtain(Class componentClass, ComponentType type)
throws ReflectionException {
Pool pool = getPool(type.getIndex());
return (T)((pool.size() > 0) ? pool.obtain() : ClassReflection.newInstance(componentClass));
}
void free(PooledComponent c, ComponentType type) {
free(c, type.getIndex());
}
void free(PooledComponent c, int typeIndex) {
c.reset();
getPool(typeIndex).free(c);
}
private Pool getPool(int typeIndex) {
Pool pool = pools.safeGet(typeIndex);
if (pool == null) {
pool = new Pool();
pools.set(typeIndex, pool);
}
return pool;
}
private static class Pool {
private final Bag cache = new Bag();
@SuppressWarnings("unchecked")
T obtain() {
return (T)cache.removeLast();
}
int size() {
return cache.size();
}
void free(PooledComponent component) {
cache.add(component);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy