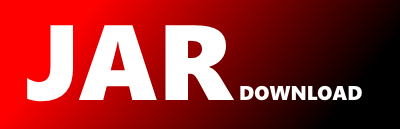
com.artemis.io.EntityReference Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of artemis-odb Show documentation
Show all versions of artemis-odb Show documentation
Fork of Artemis Entity System Framework.
package com.artemis.io;
import com.artemis.Component;
import com.artemis.Entity;
import com.artemis.utils.Bag;
import com.artemis.utils.IntBag;
import com.artemis.utils.reflect.Field;
import com.artemis.utils.reflect.ReflectionException;
class EntityReference {
public final Class> componentType;
public final Field field;
public final FieldType fieldType;
public transient final Bag operations = new Bag();
EntityReference(Class> componentType, Field field) {
this.componentType = componentType;
this.field = field;
this.fieldType = FieldType.resolve(field);
}
void translate(Bag translatedIds) {
for (Component c : operations)
fieldType.translate(c, field, translatedIds);
operations.clear();
}
enum FieldType {
INT {
void translate(Component c, Field field, Bag translatedIds) {
try {
int oldId = ((Integer)field.get(c)).intValue();
field.set(c, translatedIds.get(oldId).id);
} catch (ReflectionException e) {
throw new RuntimeException(e);
}
}
},
INT_BAG {
void translate(Component c, Field field, Bag translatedIds) {
try {
IntBag bag = (IntBag) field.get(c);
for (int i = 0, s = bag.size(); s > i; i++) {
int oldId = bag.get(i);
bag.set(i, translatedIds.get(oldId).id);
}
} catch (ReflectionException e) {
throw new RuntimeException(e);
}
}
},
ENTITY {
void translate(Component c, Field field, Bag translatedIds) {
try {
int oldId = ((Entity) field.get(c)).id;
field.set(c, translatedIds.get(oldId));
} catch (ReflectionException e) {
throw new RuntimeException(e);
}
}
},
ENTITY_BAG {
void translate(Component c, Field field, Bag translatedIds) {
try {
Bag bag = (Bag) field.get(c);
for (int i = 0, s = bag.size(); s > i; i++) {
Entity e = bag.get(i);
bag.set(i, translatedIds.get(e.id));
}
} catch (ReflectionException e) {
throw new RuntimeException(e);
}
}
};
abstract void translate(Component c, Field field, Bag translatedIds);
static FieldType resolve(Field f) {
Class type = f.getType();
if (int.class == type)
return INT;
else if (Entity.class == type)
return ENTITY;
else if (IntBag.class == type)
return INT_BAG;
else if (Bag.class == type)
return ENTITY_BAG;
else
throw new RuntimeException("missing case: " + type.getSimpleName());
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy