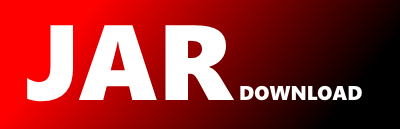
com.artemis.managers.PlayerManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of artemis-odb Show documentation
Show all versions of artemis-odb Show documentation
Fork of Artemis Entity System Framework.
package com.artemis.managers;
import java.util.HashMap;
import java.util.Map;
import com.artemis.Entity;
import com.artemis.Manager;
import com.artemis.utils.Bag;
import com.artemis.utils.ImmutableBag;
/**
* You may sometimes want to specify to which player an entity belongs to.
*
* An entity can only belong to a single player at a time.
*
*
* @author Arni Arent
*/
public class PlayerManager extends Manager {
/** All players mapped to entities as key. */
private final Map playerByEntity;
/** All entities that are mapped to a player, with the player as key. */
private final Map> entitiesByPlayer;
/**
* Creates a new PlayerManager instance.
*/
public PlayerManager() {
playerByEntity = new HashMap();
entitiesByPlayer = new HashMap>();
}
/**
* Associate the entity with the specified player.
*
* Each entity may only be assoctiated with one player at a time.
*
*
* @param e
* the entity to associate
* @param player
* the player to associtate to the entity with
*/
public void setPlayer(Entity e, String player) {
playerByEntity.put(e, player);
Bag entities = entitiesByPlayer.get(player);
if(entities == null) {
entities = new Bag();
entitiesByPlayer.put(player, entities);
}
entities.add(e);
}
/**
* Get all entities belonging to a player.
*
* @param player
* the player
*
* @return a bag containing all entities belonging to the player
*/
public ImmutableBag getEntitiesOfPlayer(String player) {
Bag entities = entitiesByPlayer.get(player);
if(entities == null) {
entities = new Bag();
}
return entities;
}
/**
* Remove the association of an entity with a player.
*
* @param e
* the entity to remove
*/
public void removeFromPlayer(Entity e) {
String player = playerByEntity.get(e);
if(player != null) {
Bag entities = entitiesByPlayer.get(player);
if(entities != null) {
entities.remove(e);
}
}
}
/**
* Get the player an entity is associated with.
*
* @param e
* the entity to get the player for
*
* @return the player
*/
public String getPlayer(Entity e) {
return playerByEntity.get(e);
}
/**
* Deleted entities are removed from their player.
*
* @param e
* the deleted entity
*/
@Override
public void deleted(Entity e) {
removeFromPlayer(e);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy