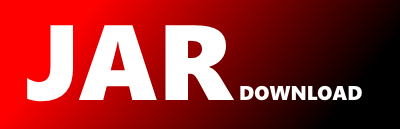
net.openesb.management.jmx.AbstractServiceImpl Maven / Gradle / Ivy
package net.openesb.management.jmx;
import com.sun.esb.management.common.ManagementRemoteException;
import com.sun.jbi.ComponentInfo;
import com.sun.jbi.ComponentQuery;
import com.sun.jbi.ComponentState;
import com.sun.jbi.ComponentType;
import com.sun.jbi.EnvironmentContext;
import com.sun.jbi.framework.ComponentLoggerMBean;
import com.sun.jbi.management.ComponentConfiguration;
import com.sun.jbi.management.ComponentExtensionMBean;
import com.sun.jbi.management.ComponentLifeCycleMBean;
import com.sun.jbi.management.DeploymentServiceMBean;
import com.sun.jbi.management.InstallationServiceMBean;
import com.sun.jbi.management.InstallerMBean;
import com.sun.jbi.management.MBeanNames;
import com.sun.jbi.management.message.ComponentTaskResult;
import com.sun.jbi.management.message.ExceptionInfo;
import com.sun.jbi.management.message.JbiTask;
import com.sun.jbi.management.message.TaskResultDetailsElement;
import com.sun.jbi.management.message.TaskStatusMsg;
import com.sun.jbi.management.system.AdminServiceMBean;
import com.sun.jbi.management.system.ConfigurationServiceMBean;
import com.sun.jbi.management.system.DeploymentServiceStatisticsMBean;
import com.sun.jbi.management.system.MessageServiceMBean;
import com.sun.jbi.messaging.MessageServiceStatisticsMBean;
import com.sun.jbi.platform.PlatformContext;
import com.sun.jbi.ui.common.JBIComponentInfo;
import com.sun.jbi.ui.common.JBIJMXObjectNames;
import com.sun.jbi.ui.common.ServiceAssemblyInfo;
import com.sun.jbi.ui.common.ServiceUnitInfo;
import com.sun.jbi.util.ComponentConfigurationHelper;
import java.io.StringReader;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.logging.Level;
import java.util.logging.Logger;
import javax.jbi.JBIException;
import javax.management.Attribute;
import javax.management.AttributeList;
import javax.management.InstanceNotFoundException;
import javax.management.MBeanAttributeInfo;
import javax.management.MBeanException;
import javax.management.MBeanInfo;
import javax.management.MBeanServer;
import javax.management.MBeanServerConnection;
import javax.management.MBeanServerInvocationHandler;
import javax.management.MalformedObjectNameException;
import javax.management.ObjectName;
import javax.management.ReflectionException;
import javax.management.RuntimeMBeanException;
import javax.management.RuntimeOperationsException;
import javax.xml.bind.JAXBContext;
import javax.xml.bind.Unmarshaller;
import javax.xml.namespace.NamespaceContext;
import javax.xml.transform.stream.StreamSource;
import javax.xml.xpath.XPath;
import javax.xml.xpath.XPathConstants;
import javax.xml.xpath.XPathFactory;
import net.openesb.model.api.State;
import net.openesb.model.api.manage.ComponentTask;
import net.openesb.model.api.manage.FrameworkTask;
import net.openesb.model.api.manage.Task;
import net.openesb.model.api.manage.TaskResult;
import net.openesb.management.api.ComponentNotFoundException;
import net.openesb.management.api.ManagementException;
import net.openesb.management.jmx.utils.I18NBundle;
import net.openesb.management.jmx.utils.StateUtils;
import org.w3c.dom.NamedNodeMap;
import org.w3c.dom.Node;
import org.xml.sax.InputSource;
/**
*
* @author David BRASSELY (brasseld at gmail.com)
* @author OpenESB Community
*/
public abstract class AbstractServiceImpl {
private static Logger sLogger = null;
private static DeploymentServiceMBean deploymentService;
private static DeploymentServiceStatisticsMBean deploymentServiceStatistics;
private static InstallationServiceMBean installationService;
private static InstallationServiceMBean installationServiceFacade;
private static MessageServiceMBean messageService;
private static ConfigurationServiceMBean configurationService;
private static MessageServiceStatisticsMBean messageServiceStatistics;
private static AdminServiceMBean adminService;
private static com.sun.jbi.management.AdminServiceMBean adminServiceExtension;
protected ComponentConfigurationHelper componentConfigurationHelper = new ComponentConfigurationHelper();
private JAXBContext mJaxbContext;
private JAXBContext mJbiDescriptorContext;
/**
* i18n
*/
private static I18NBundle I18NBUNDLE = null;
/**
* Get the logger for the REST services.
*
* @return java.util.Logger is the logger
*/
public static Logger getLogger() {
// late binding.
if (sLogger == null) {
sLogger = Logger.getLogger("net.openesb.management.jmx");
}
return sLogger;
}
/**
* @return the platform context
*/
protected PlatformContext getPlatformContext() {
PlatformContext platformContext = null;
EnvironmentContext context = getEnvironmentContext();
if (context != null) {
platformContext = context.getPlatformContext();
}
return platformContext;
}
/**
* @return the EnvironmentContext
*/
protected EnvironmentContext getEnvironmentContext() {
return com.sun.jbi.framework.EnvironmentContext.getInstance();
}
/**
* Provides access to the platform's MBean server.
*
* @return platform MBean server.
*/
protected MBeanServer getPlatformContextMBeanServer() {
return this.getPlatformContext().getMBeanServer();
}
/**
* Get the Target Name. If the instance is not a clustered instance then the
* target name is the instance name. If the instance is part of a cluster
* then the target name is the cluster name.
*
* @return the target name.
*/
protected String getPlatformContextTargetName() {
return this.getPlatformContext().getTargetName();
}
protected synchronized AdminServiceMBean getAdminServiceMBean() {
if (adminService == null) {
ObjectName adminServiceObjectName = getEnvironmentContext().getMBeanNames().getSystemServiceMBeanName(
MBeanNames.SERVICE_NAME_ADMIN_SERVICE,
MBeanNames.CONTROL_TYPE_ADMIN_SERVICE);
adminService = MBeanServerInvocationHandler.newProxyInstance(getPlatformContextMBeanServer(),
adminServiceObjectName, AdminServiceMBean.class, false);
}
return adminService;
}
protected synchronized com.sun.jbi.management.AdminServiceMBean getAdminServiceExtensionMBean() {
if (adminServiceExtension == null) {
ObjectName adminServiceObjectName = getEnvironmentContext().getMBeanNames().getSystemServiceMBeanName(
MBeanNames.ServiceName.AdminService,
MBeanNames.ServiceType.Admin, getEnvironmentContext().getPlatformContext().getTargetName());
adminServiceExtension = MBeanServerInvocationHandler.newProxyInstance(getPlatformContextMBeanServer(),
adminServiceObjectName, com.sun.jbi.management.AdminServiceMBean.class, false);
}
return adminServiceExtension;
}
protected synchronized DeploymentServiceMBean getDeploymentServiceMBean() {
if (deploymentService == null) {
ObjectName deployServiceObjectName = getEnvironmentContext().getMBeanNames().getSystemServiceMBeanName(
MBeanNames.ServiceName.DeploymentService,
MBeanNames.ServiceType.Deployment,
getEnvironmentContext().getPlatformContext().getInstanceName());
deploymentService = MBeanServerInvocationHandler.newProxyInstance(getPlatformContextMBeanServer(),
deployServiceObjectName, DeploymentServiceMBean.class, false);
}
return deploymentService;
}
protected synchronized DeploymentServiceStatisticsMBean getDeploymentServiceStatisticsMBean() {
if (deploymentServiceStatistics == null) {
ObjectName deployServiceStatisticsObjectName = getEnvironmentContext().getMBeanNames().getSystemServiceMBeanName(
MBeanNames.SERVICE_NAME_DEPLOY_SERVICE,
MBeanNames.CONTROL_TYPE_STATISTICS,
getEnvironmentContext().getPlatformContext().getInstanceName());
deploymentServiceStatistics = MBeanServerInvocationHandler.newProxyInstance(getPlatformContextMBeanServer(),
deployServiceStatisticsObjectName, DeploymentServiceStatisticsMBean.class, false);
}
return deploymentServiceStatistics;
}
protected synchronized InstallationServiceMBean getInstallationServiceMBean() {
if (installationService == null) {
ObjectName installServiceObjectName = getEnvironmentContext().getMBeanNames().getSystemServiceMBeanName(
MBeanNames.ServiceName.InstallationService,
MBeanNames.ServiceType.Installation, getEnvironmentContext().getPlatformContext().getTargetName());
installationService = MBeanServerInvocationHandler.newProxyInstance(getPlatformContextMBeanServer(),
installServiceObjectName, InstallationServiceMBean.class, false);
}
return installationService;
}
protected synchronized InstallationServiceMBean getInstallationServiceFacadeMBean() {
if (installationServiceFacade == null) {
ObjectName installServiceObjectName = getEnvironmentContext().getMBeanNames().getSystemServiceMBeanName(
MBeanNames.SERVICE_NAME_INSTALL_SERVICE,
MBeanNames.CONTROL_TYPE_INSTALL_SERVICE);
installationServiceFacade = MBeanServerInvocationHandler.newProxyInstance(getPlatformContextMBeanServer(),
installServiceObjectName, InstallationServiceMBean.class, false);
}
return installationServiceFacade;
}
protected synchronized MessageServiceMBean getMessageServiceMBean() {
if (messageService == null) {
ObjectName messageServiceObjectName = getEnvironmentContext().getMBeanNames().getSystemServiceMBeanName(
MBeanNames.SERVICE_NAME_MESSAGE_SERVICE,
MBeanNames.CONTROL_TYPE_MESSAGE_SERVICE);
messageService = MBeanServerInvocationHandler.newProxyInstance(getPlatformContextMBeanServer(),
messageServiceObjectName, MessageServiceMBean.class, false);
}
return messageService;
}
protected synchronized MessageServiceStatisticsMBean getMessageServiceStatisticsMBean() {
if (messageServiceStatistics == null) {
ObjectName messageStatisticsServiceObjectName = getEnvironmentContext().getMBeanNames().getSystemServiceMBeanName(
MBeanNames.SERVICE_NAME_MESSAGE_SERVICE,
MBeanNames.CONTROL_TYPE_STATISTICS);
messageServiceStatistics = MBeanServerInvocationHandler.newProxyInstance(getPlatformContextMBeanServer(),
messageStatisticsServiceObjectName, MessageServiceStatisticsMBean.class, false);
}
return messageServiceStatistics;
}
protected synchronized ConfigurationServiceMBean getConfigurationServiceMBean() {
if (configurationService == null) {
ObjectName configurationServiceObjectName = getEnvironmentContext().getMBeanNames().
getSystemServiceMBeanName(
MBeanNames.ServiceName.ConfigurationService,
MBeanNames.ServiceType.Logger,
getEnvironmentContext().getPlatformContext().getTargetName());
configurationService = MBeanServerInvocationHandler.newProxyInstance(getPlatformContextMBeanServer(),
configurationServiceObjectName, ConfigurationServiceMBean.class, false);
}
return configurationService;
}
protected ComponentLifeCycleMBean getComponentLifecycleMBean(String component)
throws ManagementException {
ObjectName componentLifecycleObjectName = getComponentLifeCycleMBeanObjectName(component);
return MBeanServerInvocationHandler.newProxyInstance(getPlatformContextMBeanServer(),
componentLifecycleObjectName, ComponentLifeCycleMBean.class, false);
}
protected ComponentExtensionMBean getComponentExtensionMBean(String component)
throws ManagementException {
ObjectName componentExtensionObjectName = getComponentExtensionMBeanObjectName(component);
return MBeanServerInvocationHandler.newProxyInstance(getPlatformContextMBeanServer(),
componentExtensionObjectName, ComponentExtensionMBean.class, false);
}
protected ComponentConfiguration getComponentConfigurationMBean(String component) throws ManagementException {
// try {
ObjectName componentConfigurationObjectName = getComponentConfigurationMBeanObjectName(component);
return MBeanServerInvocationHandler.newProxyInstance(getPlatformContextMBeanServer(),
componentConfigurationObjectName, ComponentConfiguration.class, false);
// } catch (ManagementRemoteException mre) {
// String[] args = new String[]{component};
// throw this.createManagementException(
// "rest.component.configuration.mbean.not.found.error",
// args, mre);
// }
}
protected InstallerMBean getComponentInstallerMBean(ObjectName componentInstallerObjectName)
throws ManagementRemoteException {
return MBeanServerInvocationHandler.newProxyInstance(getPlatformContextMBeanServer(),
componentInstallerObjectName, InstallerMBean.class, false);
}
protected ComponentLoggerMBean getComponentLoggerMBean(ObjectName componentLoggerObjectName)
throws ManagementRemoteException {
return MBeanServerInvocationHandler.newProxyInstance(getPlatformContextMBeanServer(),
componentLoggerObjectName, ComponentLoggerMBean.class, false);
}
protected ObjectName[] getSystemLoggerMBeans(String instanceName) {
com.sun.jbi.management.MBeanNames mbn = getEnvironmentContext().getMBeanNames();
String tmp = mbn.getJmxDomainName();
tmp += ":" + com.sun.jbi.management.MBeanNames.INSTANCE_NAME_KEY + "=" + instanceName;
tmp += "," + com.sun.jbi.management.MBeanNames.COMPONENT_TYPE_KEY + "=" + com.sun.jbi.management.MBeanNames.COMPONENT_TYPE_SYSTEM;
tmp += "," + com.sun.jbi.management.MBeanNames.CONTROL_TYPE_KEY + "=" + com.sun.jbi.management.MBeanNames.CONTROL_TYPE_LOGGER;
//wildcard goes at the end:
tmp += ",*";
//exec the query:
ObjectName mbeanPattern = null;
try {
mbeanPattern = new ObjectName(tmp);
} catch (javax.management.MalformedObjectNameException mex) {
return new ObjectName[0];
}
java.util.Set resultSet = getEnvironmentContext().getMBeanServer().queryNames(mbeanPattern, null);
ObjectName[] names = new ObjectName[0];
if (!resultSet.isEmpty()) {
names = (ObjectName[]) resultSet.toArray(names);
} else {
getLogger().log(Level.FINE, "Logger MBeans with ObjectName pattern {0} not found.", tmp);
}
return names;
}
/**
*
*/
private synchronized JAXBContext getJaxbContext()
throws Exception {
if (mJaxbContext == null) {
ClassLoader cl =
Class.forName(
"com.sun.jbi.management.message.JbiTaskResult").
getClassLoader();
mJaxbContext = JAXBContext.newInstance("com.sun.jbi.management.message", cl);
}
return mJaxbContext;
}
/**
*
*/
protected synchronized JAXBContext getJbiDescriptorContext()
throws Exception {
if (mJbiDescriptorContext == null) {
ClassLoader cl =
Class.forName(
"com.sun.jbi.management.descriptor.Jbi").
getClassLoader();
mJbiDescriptorContext = JAXBContext.newInstance("com.sun.jbi.management.descriptor", cl);
}
return mJbiDescriptorContext;
}
private Object unmarshal(StreamSource input)
throws Exception {
Unmarshaller reader = getJaxbContext().createUnmarshaller();
return reader.unmarshal(input);
}
protected Task getTaskFromString(String component, String jbiTaskMsg) throws
Exception {
JbiTask jbiTask = getJbiTaskFromString(jbiTaskMsg);
// Now convert JbiTask into a Management Task
Task task = new Task();
task.setComponent(component);
// Framework
FrameworkTask fwkTask = new FrameworkTask();
fwkTask.setResult(convert(
jbiTask.getJbiTaskResult().
getFrmwkTaskResult().
getFrmwkTaskResultDetails().
getTaskResultDetails()));
task.setFrameworkTask(fwkTask);
// Components
for (ComponentTaskResult compTaskResult : jbiTask.getJbiTaskResult().getComponentTaskResult()) {
ComponentTask compTask = new ComponentTask();
compTask.setComponent(compTaskResult.getComponentName());
compTask.setResult(convert(
compTaskResult.
getComponentTaskResultDetails().
getTaskResultDetails()));
task.getComponentsTask().add(compTask);
}
return task;
}
private TaskResult convert(TaskResultDetailsElement details) {
TaskResult result = new TaskResult();
result.setTaskId(details.getTaskId());
result.setTaskResult(TaskResult.TaskResultType.convert(details.getTaskResult()));
result.setMessageType(TaskResult.MessageType.convert(details.getMessageType()));
if (details.getTaskStatusMsg() != null) {
List messages = new ArrayList(details.getTaskStatusMsg().size());
for (TaskStatusMsg msg : details.getTaskStatusMsg()) {
messages.add(
msg.getMsgLocInfo().getLocToken()
+ " : " + msg.getMsgLocInfo().getLocMessage());
}
result.setTaskStatusMsg(messages);
}
if (details.getExceptionInfo() != null) {
List messages = new ArrayList(details.getExceptionInfo().size());
for (ExceptionInfo error : details.getExceptionInfo()) {
messages.add(
error.getMsgLocInfo().getLocToken()
+ " : " + error.getMsgLocInfo().getLocMessage());
}
result.setErrorMsg(messages);
}
return result;
}
/**
* Get the JbiTask Message JAXB object from the message string version
*/
private JbiTask getJbiTaskFromString(String jbiTaskMsg)
throws Exception {
StringBuilder strBuf = new StringBuilder(jbiTaskMsg);
return (JbiTask) unmarshal(new StreamSource(
new java.io.StringReader(strBuf.toString())));
}
/**
* finds the component id property in the object name
*
* @param jmxObjectName jmx obj name
* @return componentId of the component if embbeded in object name else
* null.
*/
protected String getComponentNameFromJmxObjectName(ObjectName jmxObjectName) {
String componentName = null;
try {
// TODO change COMPONENT_ID_KEY to Component Name when mgmt api
// changes.
componentName = ((ObjectName) jmxObjectName)
.getKeyProperty(JBIJMXObjectNames.COMPONENT_ID_KEY);
} catch (NullPointerException nullEx) {
componentName = null;
}
return componentName;
}
/**
* checks the component name in the registry
*
* @return true if component exists else false.
* @param componentName name of the component
*/
protected boolean isExistingComponent(String componentName) {
List list = new ArrayList();
ComponentQuery componentQuery = getEnvironmentContext().getComponentQuery();
if (componentQuery != null) {
list = componentQuery
.getComponentIds(ComponentType.BINDINGS_AND_ENGINES);
}
return list.contains(componentName);
}
/**
* returns the ObjectName for the lifecycle Mbean of this component.
*
* @return the ObjectName for the lifecycle Mbean.
* @param componentName of a binding or engine component.
* @throws ManagementRemoteException on error
*/
@SuppressWarnings("unchecked")
private ObjectName getComponentLifeCycleMBeanObjectName(
String componentName)
throws ManagementException {
getLogger().log(Level.FINE, "Get Component Lifecycle MBean Name for component {0}", componentName);
ObjectName lifecycleObjectNamePattern = null;
try {
lifecycleObjectNamePattern = JBIJMXObjectNames
.getComponentLifeCycleMBeanObjectNamePattern(componentName,
this.getPlatformContextTargetName());
} catch (MalformedObjectNameException ex) {
String[] args = new String[]{componentName, this.getPlatformContextTargetName()};
throw this.createManagementException(
"rest.component.lifecycle.mbean.bad.name",
args, ex);
}
MBeanServer mbeanServer = this.getPlatformContextMBeanServer();
Set objectNames = mbeanServer.queryNames(lifecycleObjectNamePattern,
null);
if (objectNames.isEmpty()) {
String[] args = new String[]{componentName, this.getPlatformContextTargetName()};
throw this.createManagementException(
"rest.component.lifecycle.mbean.not.found.with.query",
args, null);
}
if (objectNames.size() > 1) {
String[] args = new String[]{componentName, this.getPlatformContextTargetName()};
throw this.createManagementException(
"rest.component.lifecycle.multiple.mbeans.found.with.query",
args, null);
}
ObjectName lifecyleObjectName = (ObjectName) objectNames.iterator()
.next();
getLogger().log(Level.FINE, "LifecyleMBean : {0}", lifecyleObjectName);
return lifecyleObjectName;
}
/**
* returns the ObjectName for the extension Mbean of this component.
*
* @return the ObjectName for the extension Mbean.
* @param componentName of a binding or engine component.
* @throws ManagementRemoteException on error
*/
@SuppressWarnings("unchecked")
private ObjectName getComponentExtensionMBeanObjectName(
String componentName)
throws ManagementException {
getLogger().log(Level.FINE, "Get Component Extension MBean Name for component {0}", componentName);
ObjectName componentExtensionMBean = getAdminServiceExtensionMBean().
getComponentExtensionFacadeMBean(componentName);
getLogger().log(Level.FINE, "ExtensionMBean : {0}", componentExtensionMBean);
return componentExtensionMBean;
}
/**
* returns the ObjectName for the configuration Mbean of this component.
*
* @return the ObjectName for the configuration Mbean.
* @param componentName of a binding or engine component.
* @throws ManagementRemoteException on error
*/
@SuppressWarnings("unchecked")
protected ObjectName getComponentConfigurationMBeanObjectName(
String componentName)
throws ManagementException {
getLogger().log(Level.FINE, "Get Component Configuration MBean Name for component {0}", componentName);
ComponentExtensionMBean extensionMBean = this.getComponentExtensionMBean(componentName);
ObjectName configurationObjectName;
try {
configurationObjectName = extensionMBean.getComponentConfigurationFacadeMBeanName(this.getPlatformContextTargetName());
} catch (JBIException ex) {
String[] args = new String[]{componentName};
throw this.createManagementException(
"rest.component.config.mbean.not.found.error",
args, ex);
}
getLogger().log(Level.FINE, "ConfigurationMBean : {0}", configurationObjectName);
return configurationObjectName;
}
public Map getComponentConfigurationProperties(String componentName) throws ManagementException {
ObjectName configurationObjectName = getComponentConfigurationMBeanObjectName(componentName);
Map properties = new HashMap();
try {
MBeanInfo mbeanInfo = this.getPlatformContextMBeanServer().getMBeanInfo(configurationObjectName);
MBeanAttributeInfo[] mbeanAttrInfoArray = mbeanInfo.getAttributes();
for (MBeanAttributeInfo attributeInfo : mbeanAttrInfoArray) {
String key = attributeInfo.getName();
if (key != null) {
Object value = null;
try {
value = this.getPlatformContextMBeanServer().getAttribute(configurationObjectName, key);
} catch (Exception ex) {
String[] args = new String[]{componentName, configurationObjectName.toString()};
throw this.createManagementException(
"rest.component.config.mbean.error.get.attrs.error",
args, ex);
}
if (value != null) {
// Use getApplicationVariables to get application
// variable values
if (key.equals("ApplicationVariables")) {
continue;
}
// Use getApplicationConfigurations to get application
// configuration values
if (key.equals("ApplicationConfigurations")) {
continue;
}
properties.put(key, value);
}
}
}
} catch (Exception ex) {
String[] args = new String[]{componentName, configurationObjectName.toString()};
throw this.createManagementException(
"rest.component.config.mbean.error.get.attrs.error",
args, ex);
}
return properties;
}
protected boolean isAppVarsSupported(String componentName) throws ManagementException {
getLogger().log(Level.FINE, "isAppVarsSupported({0})", componentName);
try {
ObjectName componentConfigMBean = getComponentConfigurationMBeanObjectName(componentName);
boolean isAppVarsSupported = (Boolean) invokeMBeanOperation(componentConfigMBean, "isAppVarsSupported", new Object[]{}, new String[]{});
getLogger().log(Level.FINE, "isAppVarsSupported(): result = {0}",
isAppVarsSupported);
return isAppVarsSupported;
} catch (Exception ex) {
String[] args = new String[]{componentName};
throw this.createManagementException(
"rest.component.config.mbean.not.found.error",
args, ex);
}
}
protected boolean isAppConfigSupported(String componentName) throws ManagementException {
getLogger().log(Level.FINE, "isAppConfigSupported({0})", componentName);
ObjectName componentConfigMBean = getComponentConfigurationMBeanObjectName(componentName);
try {
boolean isAppConfigSupported = (Boolean) invokeMBeanOperation(componentConfigMBean, "isAppConfigSupported", new Object[]{}, new String[]{});
getLogger().log(Level.FINE, "isAppConfigSupported(): result = {0}",
isAppConfigSupported);
return isAppConfigSupported;
} catch (Exception ex) {
String[] args = new String[]{componentName};
throw this.createManagementException(
"rest.component.config.mbean.not.found.error",
args, ex);
}
}
protected boolean isComponentConfigSupported(String componentName) throws ManagementException {
getLogger().log(Level.FINE, "isComponentConfigSupported({0})", componentName);
try {
ObjectName componentConfigMBean = getComponentConfigurationMBeanObjectName(componentName);
boolean isComponentConfigSupported = (Boolean) invokeMBeanOperation(componentConfigMBean, "isComponentConfigSupported", new Object[]{}, new String[]{});
getLogger().log(Level.FINE, "isComponentConfigSupported(): result = {0}",
isComponentConfigSupported);
return isComponentConfigSupported;
} catch (Exception ex) {
String[] args = new String[]{componentName};
throw this.createManagementException(
"rest.component.config.mbean.not.found.error",
args, ex);
}
}
/**
* set the specified attribute values on the mbean attributes
*
* @param attrList list of attributes
* @param objectName object name
* @return management message response from setConfigurationAttributes
* @throws ManagementRemoteException on user error
*/
protected String setMBeanConfigAttributes(ObjectName objectName,
AttributeList attrList) throws ManagementRemoteException {
MBeanServer mbeanServer = getPlatformContextMBeanServer();
return this.setMBeanConfigAttributes(mbeanServer, objectName, attrList);
}
/**
* invokes the operation on mbean
*
* @return Attribute List of the mbean to set
* @param objectName mbean name
* @param params a name value pair Map object contains the attribute name
* and its value.
* @throws ManagementRemoteException on user error
*/
@SuppressWarnings("unchecked")
protected AttributeList constructMBeanAttributes(ObjectName objectName,
Map params) throws ManagementRemoteException {
MBeanServer mbeanServer = getPlatformContextMBeanServer();
AttributeList attrList = new AttributeList();
try {
MBeanInfo mbeanInfo = mbeanServer.getMBeanInfo(objectName);
MBeanAttributeInfo[] mbeanAttrInfoArray = mbeanInfo.getAttributes();
Map attribInfoMap = new HashMap();
for (int i = 0; i < mbeanAttrInfoArray.length; ++i) {
MBeanAttributeInfo attrInfo = mbeanAttrInfoArray[i];
attribInfoMap.put(attrInfo.getName(), attrInfo);
}
for (Iterator itr = params.keySet().iterator(); itr.hasNext();) {
String attrName = (String) itr.next();
Object attrValueObj = params.get(attrName);
Attribute attr = new Attribute(attrName, attrValueObj);
attrList.add(attr);
}
} catch (InstanceNotFoundException notFoundEx) {
throw new ManagementRemoteException(notFoundEx);
} catch (ReflectionException rEx) {
throw new ManagementRemoteException(rEx);
} catch (RuntimeMBeanException rtEx) {
throw ManagementRemoteException.filterJmxExceptions(rtEx);
} catch (RuntimeOperationsException rtOpEx) {
throw ManagementRemoteException.filterJmxExceptions(rtOpEx);
} catch (Exception ex) {
throw ManagementRemoteException.filterJmxExceptions(ex);
}
return attrList;
}
/**
* set the specified attribute values on the mbean attributes
*
* @param MBeanServer the MBean server to use
* @param attrList list of attributes
* @param objectName object name
* @return management message response from setConfigurationAttributes
* @throws ManagementRemoteException on user error
*/
protected String setMBeanConfigAttributes(
MBeanServerConnection mbeanServer, ObjectName objectName,
AttributeList attrList) throws ManagementRemoteException {
try {
return ((String) mbeanServer.invoke(objectName,
"setConfigurationAttributes", new Object[]{attrList},
new String[]{"javax.management.AttributeList"}));
} catch (InstanceNotFoundException notFoundEx) {
throw new ManagementRemoteException(notFoundEx);
} catch (ReflectionException rEx) {
throw new ManagementRemoteException(rEx);
} catch (RuntimeMBeanException rtEx) {
throw ManagementRemoteException.filterJmxExceptions(rtEx);
} catch (RuntimeOperationsException rtOpEx) {
throw ManagementRemoteException.filterJmxExceptions(rtOpEx);
} catch (Exception ex) {
throw ManagementRemoteException.filterJmxExceptions(ex);
}
}
/**
* invokes the operation on mbean
*
* @return result object
* @param objectName object name
* @param operationName operation name
* @throws ManagementRemoteException on user error
*/
protected Object invokeMBeanOperation(ObjectName objectName,
String operationName) throws ManagementRemoteException {
Object[] params = new Object[0];
String[] signature = new String[0];
return invokeMBeanOperation(objectName, operationName, params,
signature);
}
/**
* invokes the operation on mbean
*
* @param objectName object name
* @param operationName operation name
* @param params parameters
* @param signature signature of the parameters
* @return result object
* @throws ManagementRemoteException on user error
*/
protected Object invokeMBeanOperation(ObjectName objectName,
String operationName, Object[] params, String[] signature)
throws ManagementRemoteException {
// TODO: Add error code and message to each exception
MBeanServer mbeanServer = getPlatformContextMBeanServer();
Object result = null;
try {
result = mbeanServer.invoke(objectName, operationName, params,
signature);
} catch (InstanceNotFoundException notFoundEx) {
throw new ManagementRemoteException(notFoundEx);
} catch (ReflectionException rEx) {
throw new ManagementRemoteException(rEx);
} catch (MBeanException mbeanEx) {
throw ManagementRemoteException.filterJmxExceptions(mbeanEx);
} catch (RuntimeMBeanException rtEx) {
throw ManagementRemoteException.filterJmxExceptions(rtEx);
} catch (RuntimeOperationsException rtOpEx) {
throw ManagementRemoteException.filterJmxExceptions(rtOpEx);
} catch (Exception ex) {
throw ManagementRemoteException.filterJmxExceptions(ex);
}
return result;
}
/**
* Return the "verb" (the setXXX MBean operation) for the given log level
*
* @param logLevel log level to set logger to
* @return a MBean operation to set the logger to that level
*/
protected String setLevel(Level logLevel) {
if (null == logLevel) {
return "setDefault";
}
if (Level.OFF.equals(logLevel)) {
return "setOff";
}
if (Level.SEVERE.equals(logLevel)) {
return "setSevere";
}
if (Level.WARNING.equals(logLevel)) {
return "setWarning";
}
if (Level.INFO.equals(logLevel)) {
return "setInfo";
}
if (Level.CONFIG.equals(logLevel)) {
return "setConfig";
}
if (Level.FINE.equals(logLevel)) {
return "setFine";
}
if (Level.FINER.equals(logLevel)) {
return "setFiner";
}
if (Level.FINEST.equals(logLevel)) {
return "setFinest";
}
if (Level.ALL.equals(logLevel)) {
return "setAll";
}
// should never reach this statement
return "setDefault";
}
/**
* update state in service assembly info
*
* @param saInfo service assembly info
* @throws com.sun.jbi.ui.common.ManagementRemoteException on error
*/
protected void updateServiceAssemblyInfoState(ServiceAssemblyInfo saInfo) {
String saName = saInfo.getName();
String frameworkState = StateUtils.FRAMEWORK_SA_ANY_STATE;
try {
frameworkState = getDeploymentServiceMBean().getState(saName);
} catch (Exception ex) {
getLogger().log(Level.FINE, "issue 30: state invalid: {0}", ex.getMessage());
frameworkState = StateUtils.FRAMEWORK_SU_UNKNOWN_STATE;
}
getLogger().log(Level.FINE, "Framework State = {0} for Service Assembly = {1}",
new Object[]{frameworkState, saName});
String uiState = StateUtils.toUiServiceAssemblyState(frameworkState);
saInfo.setState(uiState);
}
/**
* update the state in each service unit info in service assembly info
*
* @param saInfo service assembly info object
* @throws com.sun.jbi.ui.common.ManagementRemoteException on error
*/
@SuppressWarnings("unchecked")
protected void updateEachServiceUnitInfoStateInServiceAssemblyInfo(
ServiceAssemblyInfo saInfo) {
List list = saInfo.getServiceUnitInfoList();
if (list == null) {
return;
}
for (Iterator itr = list.iterator(); itr.hasNext();) {
ServiceUnitInfo suInfo = (ServiceUnitInfo) itr.next();
updateServiceUnitInfoState(suInfo);
}
}
/**
* updates the state in the service unit info
*
* @param suInfo service unit info object
*/
protected void updateServiceUnitInfoState(ServiceUnitInfo suInfo) {
suInfo.setState(ServiceUnitInfo.UNKNOWN_STATE);
String compName = suInfo.getDeployedOn();
String suName = suInfo.getName();
try {
String frameworkState =
getDeploymentServiceMBean().getServiceUnitState(compName, suName);
getLogger().log(Level.FINE, "Framework State = {0} for Service UNIT {1}", new Object[]{frameworkState, suName});
String uiState = StateUtils.toUiServiceUnitState(frameworkState);
suInfo.setState(uiState);
} catch (Exception ex) {
// ignore the exception and log it
/*
log(getI18NBundle().getMessage(
"ui.mbean.exception.getting.service.unit.state", suName,
compName, ex.getMessage()));
*/
getLogger().log(Level.FINE, ex.getMessage(), ex);
}
}
/**
* list of component infos
*
* @return list of component infos
* @param slibNameList shared library names
*/
@SuppressWarnings("unchecked")
protected List getFrameworkComponentInfoListForSharedLibraryNames(
Collection slibNameList) {
ArrayList compInfoList = new ArrayList();
try {
ComponentQuery componentQuery = this.getEnvironmentContext().getComponentQuery();
if (componentQuery != null) {
for (Iterator itr = slibNameList.iterator(); itr.hasNext();) {
ComponentInfo componentInfo = componentQuery
.getSharedLibraryInfo((String) itr.next());
if (componentInfo != null) {
compInfoList.add(componentInfo);
}
}
}
} catch (Exception ex) {
// TODO propagate the exception to client.
getLogger().log(Level.FINE, ex.getMessage(), ex);
}
return compInfoList;
}
/**
* list of component names
*
* @return list of component names
* @param slibName shared library name.
*/
@SuppressWarnings("unchecked")
protected Collection getComponentNamesDependentOnSharedLibrary(
String slibName) {
List componentNames = new ArrayList();
try {
ComponentQuery componentQuery = getEnvironmentContext().getComponentQuery();
if (componentQuery != null) {
componentNames = componentQuery
.getDependentComponentIds(slibName);
}
} catch (Exception ex) {
// log exception
getLogger().log(Level.FINE, ex.getMessage(), ex);
}
return componentNames;
}
protected void checkComponentExists(String componentName) throws ManagementException {
ComponentQuery componentQuery = getEnvironmentContext().getComponentQuery();
com.sun.jbi.ComponentInfo componentInfo = null;
if (componentQuery != null) {
componentInfo = componentQuery.getComponentInfo(componentName);
}
if (componentInfo == null) {
throw this.createComponentNotFoundException(
"rest.component.id.does.not.exist",
new String[]{componentName});
}
}
/**
* Creates a management message string and populates the exception
*
* @param bundleKey
* @param args of Strings
* @param sourceException - the source exception to propagate
* @return Exception object created with a valid XML Management Message
*/
protected ManagementException createManagementException(String bundleKey,
String[] args, Exception sourceException) {
String message = getI18NBundle().getMessage(bundleKey, args);
if (sourceException != null) {
getLogger().log(Level.SEVERE, message, sourceException);
} else {
getLogger().log(Level.SEVERE, message);
}
return new ManagementException(message, sourceException);
}
protected ManagementException createManagementException(String bundleKey,
String[] args) {
return createManagementException(bundleKey, args, null);
}
protected ManagementException createManagementException(String taskString) {
try {
return new ManagementException(getTaskFromString(null, taskString));
} catch (Exception ex) {
return new ManagementException(ex.getMessage());
}
}
protected ComponentNotFoundException createComponentNotFoundException(String bundleKey,
String[] args) {
String message = getI18NBundle().getMessage(bundleKey, args);
getLogger().log(Level.SEVERE, message);
return new ComponentNotFoundException(message);
}
/**
* gives the I18N bundle
*
* @return I18NBundle object
*/
protected static I18NBundle getI18NBundle() {
// lazy initialize the JBI Client
if (I18NBUNDLE == null) {
I18NBUNDLE = new I18NBundle("net.openesb.management.jmx");
}
return I18NBUNDLE;
}
/**
* XPath query for new component version
*/
protected static final String COMPONENT_VERSION_XPATH_QUERY =
"/jbi:jbi/jbi:component/jbi:identification/identification:VersionInfo";
/**
* XPath query for new component version
*/
protected static final String SHARED_LIBRARY_VERSION_XPATH_QUERY =
"/jbi:jbi/jbi:shared-library/jbi:identification/identification:VersionInfo";
/**
* namespace for Component version info node
*/
protected static final String COMPONENT_VERSION_NS =
"http://www.sun.com/jbi/descriptor/identification";
/**
* namespace for Component version info node
*/
protected static final String COMPONENT_VERSION_NS_NEW =
"http://www.sun.com/jbi/descriptor/identification/v1.0";
/**
* convert to ui component info list
*
* @return ui component info list
* @param frameworkCompList framework component info list
*/
@SuppressWarnings("unchecked")
protected List toUiComponentInfoList(List frameworkCompList) throws ManagementException {
List uiCompList = new ArrayList(frameworkCompList.size());
for (ComponentInfo frameworkCompInfo : frameworkCompList) {
JBIComponentInfo uiCompInfo = toUiComponentInfo(frameworkCompInfo);
uiCompList.add(uiCompInfo);
}
return uiCompList;
}
/**
* return component info object
*
* @return object
* @param frameworkCompInfo framework component info
*/
protected JBIComponentInfo toUiComponentInfo(
ComponentInfo frameworkCompInfo) throws ManagementException {
String state = JBIComponentInfo.UNKNOWN_STATE;
String componentName = frameworkCompInfo.getName();
String componentDescription = frameworkCompInfo.getDescription();
ComponentState componentStatus = ComponentState.UNKNOWN;
ComponentType componentType = frameworkCompInfo.getComponentType();
// Figure out the component state
if ((false == ComponentType.BINDING.equals(componentType))
&& (false == ComponentType.ENGINE.equals(componentType))
&& (false == ComponentType.BINDINGS_AND_ENGINES
.equals(componentType))) {
componentStatus = frameworkCompInfo.getStatus();
state = StateUtils.toUiComponentInfoState(componentStatus);
} else {
try {
state = getComponentLifecycleMBean(componentName).getCurrentState();
} catch (ManagementException ex) {
// do not rethrow it. The state is already defaulted to
// JBIComponentInfo.UNKNOWN_STATE
componentStatus = ComponentState.UNKNOWN;
state = JBIComponentInfo.UNKNOWN_STATE;
}
}
if (true == StateUtils.RUNNING_STATE.equals(state)) {
state = JBIComponentInfo.STARTED_STATE;
}
String type = StateUtils.toUiComponentInfoType(componentType);
// Get descripor string to greb version and build numbers out
String componentVersion = State.UNKNOWN.name();
String buildNumber = State.UNKNOWN.name();
try {
String descriptorXml = frameworkCompInfo.getInstallationDescriptor();
InputSource inputSource = new InputSource(new StringReader(descriptorXml));
String queryString = "";
if (ComponentType.BINDING.equals(componentType)
|| ComponentType.ENGINE.equals(componentType)
|| ComponentType.BINDINGS_AND_ENGINES.equals(componentType)) {
queryString = COMPONENT_VERSION_XPATH_QUERY;
} else if (ComponentType.SHARED_LIBRARY.equals(componentType)) {
queryString = SHARED_LIBRARY_VERSION_XPATH_QUERY;
}
XPathFactory factory = XPathFactory.newInstance();
XPath xpath = factory.newXPath();
xpath.setNamespaceContext(new IdentificationNSContext());
Node rtnNode = (Node) xpath.evaluate(queryString,
inputSource,
XPathConstants.NODE);
if (rtnNode != null) {
NamedNodeMap attrs = rtnNode.getAttributes();
Node verAttr = attrs.getNamedItem("specification-version");
if (verAttr != null) {
componentVersion = verAttr.getNodeValue();
}
Node buildAttr = attrs.getNamedItem("build-number");
if (buildAttr != null) {
buildNumber = buildAttr.getNodeValue();
}
} else {
// Try the new version attr name
inputSource = new InputSource(new StringReader(descriptorXml));
xpath.setNamespaceContext(new IdentificationNewNSContext());
rtnNode = (Node) xpath.evaluate(queryString,
inputSource,
XPathConstants.NODE);
if (rtnNode != null) {
NamedNodeMap attrs = rtnNode.getAttributes();
Node verAttr = attrs.getNamedItem("component-version");
if (verAttr != null) {
componentVersion = verAttr.getNodeValue();
}
Node buildAttr = attrs.getNamedItem("build-number");
if (buildAttr != null) {
buildNumber = buildAttr.getNodeValue();
}
}
}
} catch (Exception exp) {
String[] args = {""};
Exception exception = new Exception("ui.mbean.install.config.mbean.error.get.attrs.error");
throw new ManagementException(exception);
}
JBIComponentInfo compInfo = new JBIComponentInfo(type, // Integer.toString(comp.getComponentType()),
state, // Integer.toString(comp.getStatus()),
componentName, componentDescription);
compInfo.setComponentVersion(componentVersion);
compInfo.setBuildNumber(buildNumber);
return compInfo;
}
/*
* Inner class defined for component identification namespace
*/
class IdentificationNSContext implements NamespaceContext {
/**
* This method maps the prefix jbi and config to the right URIs
*/
public String getNamespaceURI(String prefix) {
if (prefix.equals("jbi")) {
return "http://java.sun.com/xml/ns/jbi";
} else if (prefix.equals("identification")) {
return COMPONENT_VERSION_NS;
} else {
return "http://java.sun.com/xml/ns/jbi";
}
}
public String getPrefix(String uri) {
throw new UnsupportedOperationException();
}
public Iterator getPrefixes(String uri) {
throw new UnsupportedOperationException();
}
}
/*
* Inner class defined for component identification namespace
*/
class IdentificationNewNSContext implements NamespaceContext {
/**
* This method maps the prefix jbi and config to the right URIs
*/
public String getNamespaceURI(String prefix) {
if (prefix.equals("jbi")) {
return "http://java.sun.com/xml/ns/jbi";
} else if (prefix.equals("identification")) {
return COMPONENT_VERSION_NS_NEW;
} else {
return "http://java.sun.com/xml/ns/jbi";
}
}
public String getPrefix(String uri) {
throw new UnsupportedOperationException();
}
public Iterator getPrefixes(String uri) {
throw new UnsupportedOperationException();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy