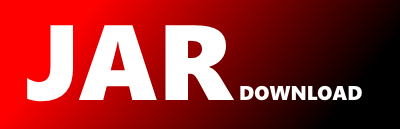
net.openesb.management.jmx.ComponentServiceImpl Maven / Gradle / Ivy
package net.openesb.management.jmx;
import com.sun.jbi.ComponentInfo;
import com.sun.jbi.ComponentQuery;
import com.sun.jbi.ComponentState;
import com.sun.jbi.ServiceUnitInfo;
import com.sun.jbi.framework.ComponentLoggerMBean;
import com.sun.jbi.management.ComponentLifeCycleMBean;
import com.sun.jbi.ui.common.JBIComponentInfo;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import java.util.logging.Level;
import javax.jbi.JBIException;
import javax.management.ObjectName;
import net.openesb.model.api.ComponentDescriptor;
import net.openesb.model.api.JBIComponent;
import net.openesb.model.api.Logger;
import net.openesb.model.api.ServiceUnit;
import net.openesb.model.api.State;
import net.openesb.management.api.ComponentService;
import net.openesb.management.api.ComponentType;
import net.openesb.management.api.ManagementException;
import static net.openesb.management.jmx.AbstractServiceImpl.getLogger;
import net.openesb.management.jmx.utils.ComponentConverter;
import net.openesb.management.jmx.utils.ComponentTypeConverter;
import net.openesb.management.jmx.utils.ServiceUnitConverter;
import net.openesb.management.jmx.utils.StateUtils;
/**
*
* @author David BRASSELY (brasseld at gmail.com)
* @author OpenESB Community
*/
public class ComponentServiceImpl extends AbstractServiceImpl implements ComponentService {
@Override
public Set findComponents(ComponentType type, String state, String sharedLibraryName, String serviceAssemblyName) throws ManagementException {
ComponentState frameworkCompState = ComponentState.UNKNOWN;
if (state != null) {
frameworkCompState = StateUtils.toFrameworkComponentInfoState(state);
}
String slibName = null;
String saName = null;
if (sharedLibraryName != null && sharedLibraryName.trim().length() > 0) {
slibName = sharedLibraryName.trim();
}
if (serviceAssemblyName != null
&& serviceAssemblyName.trim().length() > 0) {
saName = serviceAssemblyName.trim();
}
com.sun.jbi.ComponentType internalComponenType =
ComponentTypeConverter.convert(type);
getLogger().log(Level.FINE, "listComponents: Params : {0}, {1}, {2}, {3}",
new Object[]{internalComponenType, frameworkCompState, slibName, saName});
Collection componentNames;
// there are 4 options with snsId,auId (00,01,10,11)
if (slibName == null && saName == null) {
// 00
componentNames = getComponentNamesWithStatus(internalComponenType,
frameworkCompState);
} else if (slibName == null && saName != null) {
// 01
componentNames = getComponentNamesDependentOnServiceAssembly(
internalComponenType, frameworkCompState, saName);
} else if (slibName != null && saName == null) {
// 10
componentNames = getComponentNamesDependentOnSharedLibrary(
internalComponenType, frameworkCompState, slibName);
} else if (slibName != null && saName != null) {
// 11
componentNames = getComponentNamesDependentOnSharedLibraryAndServiceAssembly(
internalComponenType, frameworkCompState, slibName, saName);
} else {
// not possible.
componentNames = new HashSet();
}
List compsInfo = toUiComponentInfoList(getFrameworkComponentInfoList(componentNames));
Set components = new HashSet(compsInfo.size());
for (JBIComponentInfo compInfo : compsInfo) {
components.add(ComponentConverter.convert(compInfo));
}
return components;
}
@Override
public JBIComponent getComponent(String componentName) throws ManagementException {
checkComponentExists(componentName);
com.sun.jbi.ComponentInfo componentInfo = getComponentInfo(componentName);
JBIComponentInfo uiComponentInfo = toUiComponentInfo(componentInfo);
JBIComponent component = ComponentConverter.convert(uiComponentInfo);
Set sus = new HashSet(
componentInfo.getServiceUnitList().size());
for (ServiceUnitInfo suInfo : componentInfo.getServiceUnitList()) {
ServiceUnit serviceUnit = ServiceUnitConverter.convert(suInfo);
try {
String state = getDeploymentServiceMBean().getServiceUnitState(suInfo.getTargetComponent(), suInfo.getName());
serviceUnit.setState(State.from(state));
} catch (JBIException ex) {
java.util.logging.Logger.getLogger(ComponentServiceImpl.class.getName()).log(Level.SEVERE, null, ex);
}
sus.add(serviceUnit);
}
component.setServiceUnits(sus);
component.setSupportApplicationConfigurations(isAppConfigSupported(componentName));
component.setSupportApplicationVariables(isAppVarsSupported(componentName));
component.setSupportComponentConfigurations(isComponentConfigSupported(componentName));
return component;
}
@Override
public ComponentDescriptor getDescriptor(String componentName) throws ManagementException {
checkComponentExists(componentName);
com.sun.jbi.ComponentInfo retrievedInfo = getComponentInfo(componentName);
JBIComponentInfo jbiComponentInfo = toUiComponentInfo(retrievedInfo);
ComponentDescriptor info = new ComponentDescriptor();
info.setName(retrievedInfo.getName());
info.setDescription(retrievedInfo.getDescription());
if (jbiComponentInfo.getType().equals(JBIComponentInfo.BINDING_TYPE)) {
info.setType(net.openesb.model.api.ComponentType.BINDING_COMPONENT);
} else if (jbiComponentInfo.getType().equals(JBIComponentInfo.ENGINE_TYPE)) {
info.setType(net.openesb.model.api.ComponentType.SERVICE_ENGINE);
} else if (jbiComponentInfo.getType().equals(JBIComponentInfo.SHARED_LIBRARY_TYPE)) {
info.setType(net.openesb.model.api.ComponentType.SHARED_LIBRARY);
}
info.setBootstrapClassLoaderSelfFirst(retrievedInfo.isBootstrapClassLoaderSelfFirst());
info.setBootstrapClassName(retrievedInfo.getBootstrapClassName());
info.setBootstrapClassPathElements(retrievedInfo.getBootstrapClassPathElements());
info.setClassLoaderSelfFirst(retrievedInfo.isClassLoaderSelfFirst());
info.setClassPathElements(retrievedInfo.getClassPathElements());
info.setComponentClassName(retrievedInfo.getComponentClassName());
info.setInstallRoot(retrievedInfo.getInstallRoot());
info.setSharedLibraries(retrievedInfo.getSharedLibraryNames());
info.setWorkspaceRoot(retrievedInfo.getWorkspaceRoot());
info.setBuildNumber(jbiComponentInfo.getBuildNumber());
info.setVersion(jbiComponentInfo.getComponentVersion());
return info;
}
@Override
public String getDescriptorAsXml(String componentName) throws ManagementException {
checkComponentExists(componentName);
com.sun.jbi.ComponentInfo retrievedInfo = getComponentInfo(componentName);
if (retrievedInfo != null) {
return retrievedInfo.getInstallationDescriptor();
}
return null;
}
/**
* list of component names
*
* @return list of component names
* @param frameworkCompType component type
* @param frameworkCompStatus component state
* @param slibName shared library name
*/
@SuppressWarnings("unchecked")
private Collection getComponentNamesDependentOnSharedLibrary(
com.sun.jbi.ComponentType frameworkCompType,
ComponentState frameworkCompStatus, String slibName) {
Set compNameSet = new HashSet(this.getComponentNamesWithStatus(
frameworkCompType, frameworkCompStatus));
Set slibNameDepCompNameSet = new HashSet(
this.getComponentNamesDependentOnSharedLibrary(slibName));
// now retain only ids in the compIdSet that are in compIdSetWithSnsd
compNameSet.retainAll(slibNameDepCompNameSet);
return compNameSet;
}
/**
* list of component names
*
* @return list of component names
* @param frameworkCompType component type
* @param frameworkCompStatus component state
*/
@SuppressWarnings("unchecked")
private Set getComponentNamesWithStatus(com.sun.jbi.ComponentType frameworkCompType,
ComponentState frameworkCompStatus) {
List componentIdList = new ArrayList();
ComponentQuery componentQuery = getEnvironmentContext().getComponentQuery();
if (componentQuery != null) {
if (frameworkCompStatus == ComponentState.UNKNOWN) {
// ANY STATE
componentIdList = componentQuery
.getComponentIds(frameworkCompType);
} else {
componentIdList = componentQuery.getComponentIds(
frameworkCompType, frameworkCompStatus);
}
}
// now retain only ones that has auID deployed.
return new HashSet(componentIdList);
}
/**
* list of component names
*
* @return list of component names
* @param frameworkCompType component type
* @param frameworkCompStatus component state
* @param saName service assembly name
*/
@SuppressWarnings("unchecked")
private Collection getComponentNamesDependentOnServiceAssembly(
com.sun.jbi.ComponentType frameworkCompType,
ComponentState frameworkCompStatus, String saName) {
Set compNameSet = new HashSet(getComponentNamesWithStatus(
frameworkCompType, frameworkCompStatus));
Set saNameDepCompNameSet = new HashSet(
this.getComponentNamesDependentOnServiceAssembly(saName));
// now retain only ids in the compIdSet that are in compIdSetWithAuId
compNameSet.retainAll(saNameDepCompNameSet);
return compNameSet;
}
/**
* list of component names
*
* @return list of component names
* @param saId service assembly name.
*/
@SuppressWarnings("unchecked")
private Collection getComponentNamesDependentOnServiceAssembly(
String saId) {
try {
String[] componentNames = getDeploymentServiceMBean().
getComponentsForDeployedServiceAssembly(saId);
if (componentNames == null) {
componentNames = new String[0];
}
return new HashSet(Arrays.asList(componentNames));
} catch (Exception ex) {
// log exception
getLogger().log(Level.FINE, ex.getMessage(), ex);
// empty set
return new HashSet();
}
}
/**
* list of component names. this method requires non null inputs
*
* @return list of component names.
* @param frameworkCompType component type
* @param frameworkCompStatus component state
* @param slibName shared library name
* @param saName service assembly name
*/
@SuppressWarnings("unchecked")
private Collection getComponentNamesDependentOnSharedLibraryAndServiceAssembly(
com.sun.jbi.ComponentType frameworkCompType,
ComponentState frameworkCompStatus, String slibName, String saName) {
Set compNameSet = new HashSet(this.getComponentNamesWithStatus(
frameworkCompType, frameworkCompStatus));
Set slibNameDepCompNameSet = new HashSet(
this.getComponentNamesDependentOnSharedLibrary(slibName));
Set saNameDepCompNameSet = new HashSet(
this.getComponentNamesDependentOnServiceAssembly(saName));
// intersection of SLIB and SA
slibNameDepCompNameSet.retainAll(saNameDepCompNameSet);
// intersection of type, status, SLIB, SA
compNameSet.retainAll(slibNameDepCompNameSet);
return compNameSet;
}
/**
* framework component info list
*
* @param compNameList list of component names
* @return framework component info list
*/
@SuppressWarnings("unchecked")
private List getFrameworkComponentInfoList(Collection compNameList) {
List compInfoList = new ArrayList();
try {
ComponentQuery componentQuery = getEnvironmentContext().getComponentQuery();
if (componentQuery != null) {
for (String componentName : compNameList) {
ComponentInfo componentInfo =
getComponentInfo(componentName);
if (componentInfo != null) {
compInfoList.add(componentInfo);
}
}
}
} catch (Exception ex) {
// TODO propagate the exception to client.
getLogger().log(Level.FINE, ex.getMessage(), ex);
}
return compInfoList;
}
private ComponentInfo getComponentInfo(String componentName) throws ManagementException {
try {
ComponentQuery componentQuery = getEnvironmentContext().getComponentQuery();
if (componentQuery != null) {
return componentQuery.getComponentInfo(componentName);
}
} catch (Exception ex) {
// TODO propagate the exception to client.
getLogger().log(Level.FINE, ex.getMessage(), ex);
}
return null;
}
@Override
public void start(String componentName) throws ManagementException {
checkComponentExists(componentName);
ComponentLifeCycleMBean compLifecycleMBean = getComponentLifecycleMBean(componentName);
try {
compLifecycleMBean.start();
} catch (JBIException ex) {
String[] args = {componentName};
throw this.createManagementException(
"rest.component.lifecycle.start.error", args, ex);
}
}
@Override
public void stop(String componentName) throws ManagementException {
checkComponentExists(componentName);
ComponentLifeCycleMBean compLifecycleMBean = getComponentLifecycleMBean(componentName);
try {
compLifecycleMBean.stop();
} catch (JBIException ex) {
String[] args = {componentName};
throw this.createManagementException(
"rest.component.lifecycle.stop.error", args, ex);
}
}
@Override
public void shutdown(String componentName, boolean force) throws ManagementException {
checkComponentExists(componentName);
ComponentLifeCycleMBean compLifecycleMBean = getComponentLifecycleMBean(componentName);
try {
compLifecycleMBean.shutDown(force);
} catch (JBIException ex) {
String[] args = {componentName, Boolean.toString(force)};
throw this.createManagementException(
"rest.component.lifecycle.shutdown.error", args, ex);
}
}
@Override
public String install(String cmpZipURL) throws ManagementException {
try {
// 1_ Load the installer
ObjectName installerObjectName = getInstallationServiceMBean().loadNewInstaller(cmpZipURL);
String componentName = getComponentNameFromJmxObjectName(installerObjectName);
// 2_ Launch installation
getComponentInstallerMBean(installerObjectName).install();
return componentName;
} catch (Exception e) {
throw this.createManagementException(e.getMessage());
}
}
@Override
public void uninstall(String componentName, boolean force) throws ManagementException {
try {
// 1_ Load the installer
ObjectName installerObjectName = getInstallationServiceMBean().loadInstaller(componentName, force);
getComponentInstallerMBean(installerObjectName).uninstall(force);
getInstallationServiceMBean().unloadInstaller(componentName, true);
} catch (Exception e) {
throw this.createManagementException(e.getMessage());
}
}
private ComponentLoggerMBean getComponentLoggerMBean(String componentName) throws ManagementException {
// We are in the context of a single instance, so we just have to get back
// the object name for index = 1;
try {
ObjectName[] loggersObjectName = getComponentExtensionMBean(componentName).getLoggerMBeanNames().values().iterator().next();
return getComponentLoggerMBean(loggersObjectName[0]);
} catch (Exception ex) {
String[] args = new String[]{componentName};
throw this.createManagementException(
"rest.component.extension.getComponentLoggerLevels.error", args,
ex);
}
}
@Override
public Set getLoggers(String componentName) throws ManagementException {
checkComponentExists(componentName);
try {
ComponentLoggerMBean mBean = getComponentLoggerMBean(componentName);
String[] loggerNames = mBean.getLoggerNames();
Set loggers = new HashSet(loggerNames.length);
for (String loggerName : loggerNames) {
Logger logger = new Logger();
logger.setName(loggerName);
logger.setDisplayName(mBean.getDisplayName(loggerName));
logger.setLevel(Level.parse(
mBean.getLevel(loggerName)).getName());
logger.setLocalizedLevel(mBean.getLevel(loggerName));
loggers.add(logger);
}
return loggers;
} catch (Exception e) {
throw new ManagementException(e.getMessage());
}
}
@Override
public void setLoggerLevel(String componentName, String loggerName, Level loggerLevel) throws ManagementException {
checkComponentExists(componentName);
try {
ComponentLoggerMBean mBean = getComponentLoggerMBean(componentName);
if (loggerLevel == null) {
mBean.setDefault(loggerName);
} else if (Level.ALL.equals(loggerLevel)) {
mBean.setAll(loggerName);
} else if (Level.CONFIG.equals(loggerLevel)) {
mBean.setConfig(loggerName);
} else if (Level.FINE.equals(loggerLevel)) {
mBean.setFine(loggerName);
} else if (Level.FINER.equals(loggerLevel)) {
mBean.setFiner(loggerName);
} else if (Level.FINEST.equals(loggerLevel)) {
mBean.setFinest(loggerName);
} else if (Level.INFO.equals(loggerLevel)) {
mBean.setInfo(loggerName);
} else if (Level.OFF.equals(loggerLevel)) {
mBean.setOff(loggerName);
} else if (Level.SEVERE.equals(loggerLevel)) {
mBean.setSevere(loggerName);
} else if (Level.WARNING.equals(loggerLevel)) {
mBean.setWarning(loggerName);
}
} catch (Exception e) {
throw new ManagementException(e.getMessage());
}
}
@Override
public void upgrade(String componentName, String cmpZipURL) throws ManagementException {
checkComponentExists(componentName);
try {
getInstallationServiceFacadeMBean().upgradeComponent(componentName, cmpZipURL);
} catch (Exception e) {
throw new ManagementException(e.getMessage());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy