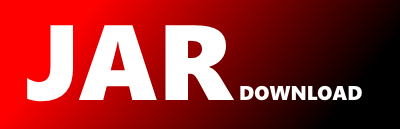
net.openesb.management.jmx.ConfigurationServiceImpl Maven / Gradle / Ivy
package net.openesb.management.jmx;
import java.io.StringReader;
import java.lang.reflect.UndeclaredThrowableException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Properties;
import java.util.Set;
import java.util.logging.Level;
import javax.management.AttributeList;
import javax.management.MBeanException;
import javax.management.ObjectName;
import javax.management.openmbean.CompositeData;
import javax.management.openmbean.TabularData;
import javax.xml.bind.JAXBContext;
import javax.xml.bind.Unmarshaller;
import net.openesb.model.api.ApplicationConfiguration;
import net.openesb.model.api.ApplicationVariable;
import net.openesb.model.api.ComponentConfiguration;
import net.openesb.model.api.Configuration;
import net.openesb.management.api.ConfigurationService;
import net.openesb.management.api.ManagementException;
import static net.openesb.management.jmx.AbstractServiceImpl.getLogger;
/**
*
* @author David BRASSELY (brasseld at gmail.com)
* @author OpenESB Community
*/
public class ConfigurationServiceImpl extends AbstractServiceImpl implements ConfigurationService {
@Override
public Set getComponentConfiguration(String componentName) throws ManagementException {
checkComponentExists(componentName);
if (!isComponentConfigSupported(componentName)) {
throw this.createManagementException(
"rest.component.config.not.supported",
new String[]{componentName}, null);
}
try {
Map configurations = getComponentConfigurationProperties(componentName);
Set structuredConfigurations = new HashSet(configurations.size());
for (Map.Entry config : configurations.entrySet()) {
structuredConfigurations.add(
new ComponentConfiguration(
config.getKey(), config.getValue()));
}
return structuredConfigurations;
} catch (Exception ex) {
getLogger().log(Level.SEVERE, null, ex);
throw new ManagementException(ex.getMessage());
}
}
@Override
public Set getApplicationVariables(String componentName) throws ManagementException {
checkComponentExists(componentName);
if (!isAppVarsSupported(componentName)) {
throw this.createManagementException(
"rest.component.app.vars.not.supported",
new String[]{componentName}, null);
}
try {
Map variables = retrieveApplicationVariables(componentName);
Set structVariables = new HashSet(variables.size());
for (Map.Entry variable : variables.entrySet()) {
structVariables.add(
new ApplicationVariable(
variable.getKey(),
componentConfigurationHelper.getAppVarValue(variable.getValue()),
componentConfigurationHelper.getAppVarType(variable.getValue(), "string")));
}
return structVariables;
} catch (Exception ex) {
getLogger().log(Level.SEVERE, null, ex);
throw new ManagementException(ex.getMessage());
}
}
private Map retrieveApplicationVariables(String component) throws Exception {
com.sun.jbi.management.ComponentConfiguration config = getComponentConfigurationMBean(component);
TabularData datas = config.getApplicationVariables();
Properties props = componentConfigurationHelper.convertToApplicationVariablesProperties(datas);
return new HashMap(props);
}
@Override
public void updateComponentConfiguration(String componentName, Set configurations) throws ManagementException {
checkComponentExists(componentName);
if (!isComponentConfigSupported(componentName)) {
throw this.createManagementException(
"rest.component.config.not.supported",
new String[]{componentName}, null);
}
Set oldConfigurations = getComponentConfiguration(componentName);
Set newConfigurations = new HashSet(oldConfigurations.size());
newConfigurations.addAll(configurations);
newConfigurations.addAll(oldConfigurations);
try {
ObjectName ojbName = getComponentConfigurationMBeanObjectName(componentName);
AttributeList attrs = constructMBeanAttributes(ojbName, convert(newConfigurations));
setMBeanConfigAttributes(ojbName, attrs);
} catch (Exception ex) {
getLogger().log(Level.SEVERE, null, ex);
throw new ManagementException(ex.getMessage());
}
}
private Map convert(Set configurations) {
Map map = new HashMap(configurations.size());
for (ComponentConfiguration config : configurations) {
map.put(config.getName(), config.getValue());
}
return map;
}
@Override
public void updateApplicationVariable(String componentName, Set appVariables) throws ManagementException {
checkComponentExists(componentName);
getLogger().log(Level.FINE, "updateApplicationVariable({0},{1})",
new Object[]{componentName, appVariables});
if (!isAppVarsSupported(componentName)) {
throw this.createManagementException(
"rest.component.app.vars.not.supported",
new String[]{componentName}, null);
}
//TODO: check component started
com.sun.jbi.management.ComponentConfiguration configurationMBean =
getComponentConfigurationMBean(componentName);
if (appVariables != null) {
for (ApplicationVariable appVariable : appVariables) {
try {
configurationMBean.setApplicationVariable(
appVariable.getName(),
convertApplicationVariable(appVariable));
} catch (Exception ex) {
String[] args = {appVariable.getName(), componentName};
if (ex instanceof UndeclaredThrowableException) {
ex = (Exception)((UndeclaredThrowableException)ex).getUndeclaredThrowable();
}
throw this.createManagementException(
"rest.component.set.app.vars.error", args, ex);
}
}
}
}
@Override
public void addApplicationVariable(String componentName, Set appVariables) throws ManagementException {
checkComponentExists(componentName);
getLogger().log(Level.FINE, "addApplicationVariable({0},{1})",
new Object[]{componentName, appVariables});
if (!isAppVarsSupported(componentName)) {
throw this.createManagementException(
"rest.component.app.vars.not.supported",
new String[]{componentName}, null);
}
//TODO: check component started
com.sun.jbi.management.ComponentConfiguration configurationMBean =
getComponentConfigurationMBean(componentName);
if (appVariables != null) {
for (ApplicationVariable appVariable : appVariables) {
try {
configurationMBean.addApplicationVariable(
appVariable.getName(),
convertApplicationVariable(appVariable));
} catch (Exception ex) {
String[] args = {appVariable.getName(), componentName};
if (ex instanceof UndeclaredThrowableException) {
ex = (Exception)((UndeclaredThrowableException)ex).getUndeclaredThrowable();
}
throw this.createManagementException(
"rest.component.add.app.vars.error", args, ex);
}
}
}
}
private CompositeData convertApplicationVariable(ApplicationVariable appVariable) {
try {
return componentConfigurationHelper.createApplicationVariableComposite(appVariable.getName(), appVariable.getValue(), appVariable.getType());
} catch (Exception ex) {
getLogger().log(Level.SEVERE, "convertApplicationVariable(" + appVariable + ")", ex);
return null;
}
}
@Override
public void deleteApplicationVariables(String componentName, String[] appVariableNames) throws ManagementException {
checkComponentExists(componentName);
getLogger().log(Level.FINE, "deleteApplicationVariables({0},{1})",
new Object[]{componentName, appVariableNames});
if (!isAppVarsSupported(componentName)) {
throw this.createManagementException(
"rest.component.app.vars.not.supported",
new String[]{componentName}, null);
}
//TODO: check component started
com.sun.jbi.management.ComponentConfiguration configurationMBean =
getComponentConfigurationMBean(componentName);
if (appVariableNames != null) {
for (String appVariableName : appVariableNames) {
try {
configurationMBean.deleteApplicationVariable(appVariableName);
} catch (Exception ex) {
String[] args = {appVariableName, componentName};
throw this.createManagementException(
"rest.component.del.app.vars.error", args, ex);
}
}
}
}
@Override
public Configuration getConfigurationSchema(String componentName) throws ManagementException {
checkComponentExists(componentName);
//TODO: check component started
com.sun.jbi.management.ComponentConfiguration configurationMBean =
getComponentConfigurationMBean(componentName);
try {
String schema = configurationMBean.retrieveConfigurationDisplayData();
JAXBContext jaxbContext = JAXBContext.newInstance(Configuration.class);
Unmarshaller unmarshaller = jaxbContext.createUnmarshaller();
StringReader reader = new StringReader(schema);
return (Configuration) unmarshaller.unmarshal(reader);
} catch (Exception e) {
throw new ManagementException("error : " + e.getMessage());
}
}
private Map retrieveApplicationConfigurations(String component) throws Exception {
com.sun.jbi.management.ComponentConfiguration config = getComponentConfigurationMBean(component);
TabularData datas = config.getApplicationConfigurations();
return getApplicationConfigurationsMap(datas);
}
/**
* Convert a application configuration TabularData to a Map keyed by the
* "configurationName". The value is the application configuration
* represented as properties.
*
* @return a Map of application configuration properties
*/
private Map getApplicationConfigurationsMap(
TabularData td) {
Map configMap = new HashMap();
Set configKeys = td.keySet();
if (configKeys != null) {
for (Object configKey : configKeys) {
List keyList = (List) configKey;
String[] index = new String[keyList.size()];
index = (String[]) keyList.toArray(index);
CompositeData cd = td.get(index);
Properties configProps = this.componentConfigurationHelper
.convertCompositeDataToProperties(cd);
configMap.put(index[0], configProps);
}
}
return configMap;
}
@Override
public Set getApplicationConfigurations(String componentName) throws ManagementException {
checkComponentExists(componentName);
if (!isAppConfigSupported(componentName)) {
throw this.createManagementException(
"rest.component.app.config.not.supported",
new String[]{componentName}, null);
}
try {
Map configurations = retrieveApplicationConfigurations(componentName);
return configurations.keySet();
} catch (Exception ex) {
getLogger().log(Level.SEVERE, null, ex);
throw new ManagementException(ex.getMessage());
}
}
@Override
public ApplicationConfiguration getApplicationConfiguration(String componentName, String configurationName) throws ManagementException {
checkComponentExists(componentName);
if (!isAppConfigSupported(componentName)) {
throw this.createManagementException(
"rest.component.app.config.not.supported",
new String[]{componentName}, null);
}
Map configurationsMap = null;
try {
configurationsMap = retrieveApplicationConfigurations(componentName);
} catch (Exception ex) {
getLogger().log(Level.SEVERE, null, ex);
throw new ManagementException(ex.getMessage());
}
Properties appConfigurationProp = configurationsMap.get(configurationName);
if (appConfigurationProp != null) {
ApplicationConfiguration applicationConfiguration = new ApplicationConfiguration(configurationName);
Set configurations = new HashSet(appConfigurationProp.size());
for (final Map.Entry
© 2015 - 2025 Weber Informatics LLC | Privacy Policy