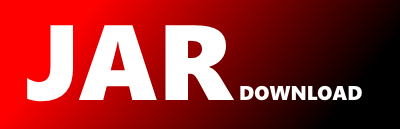
net.openesb.management.jmx.SharedLibraryServiceImpl Maven / Gradle / Ivy
package net.openesb.management.jmx;
import com.sun.jbi.ComponentInfo;
import com.sun.jbi.ComponentQuery;
import com.sun.jbi.ComponentType;
import com.sun.jbi.ui.common.JBIComponentInfo;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import java.util.logging.Level;
import net.openesb.model.api.ComponentDescriptor;
import net.openesb.model.api.SharedLibrary;
import net.openesb.management.api.ManagementException;
import net.openesb.management.api.SharedLibraryService;
import net.openesb.management.jmx.utils.SharedLibraryConverter;
/**
*
* @author David BRASSELY (brasseld at gmail.com)
* @author OpenESB Community
*/
public class SharedLibraryServiceImpl extends AbstractServiceImpl implements SharedLibraryService {
@Override
public Set findSharedLibraries(String componentName) throws ManagementException {
if (componentName != null && ! componentName.trim().isEmpty()) {
checkComponentExists(componentName);
}
List frameworkCompInfoList = this
.getFrameworkComponentInfoListForSharedLibraries(componentName);
List uiCompInfoList = this.toUiComponentInfoList(frameworkCompInfoList);
Set libraries = new HashSet(uiCompInfoList.size());
for (JBIComponentInfo compInfo : uiCompInfoList) {
libraries.add(SharedLibraryConverter.convert(compInfo));
}
return libraries;
}
@Override
public String getDescriptorAsXml(String sharedLibraryName) throws ManagementException {
checkSharedLibraryExists(sharedLibraryName);
ComponentQuery componentQuery = getEnvironmentContext().getComponentQuery();
if (componentQuery != null) {
com.sun.jbi.ComponentInfo retrievedInfo =
componentQuery.getSharedLibraryInfo(sharedLibraryName);
return retrievedInfo.getInstallationDescriptor();
}
return null;
}
private void checkSharedLibraryExists(String sharedLibraryName) {
ComponentQuery componentQuery = getEnvironmentContext().getComponentQuery();
com.sun.jbi.ComponentInfo componentInfo = null;
if (componentQuery != null) {
componentInfo = componentQuery.getSharedLibraryInfo(sharedLibraryName);
}
if (componentInfo == null) {
throw this.createComponentNotFoundException(
"rest.library.id.does.not.exist",
new String[]{sharedLibraryName});
}
}
/**
* this methods check for valid inputs and take only a valid inputs. for
* example slib, saName both can be null
*
* @param componentName name
* @throws com.sun.jbi.ui.common.ManagementRemoteException on error
* @return list of componentInfo objects
*/
@SuppressWarnings("unchecked")
private List getFrameworkComponentInfoListForSharedLibraries(
String componentName)
throws ManagementException {
String compName = null;
if (componentName != null && componentName.trim().length() > 0) {
compName = componentName.trim();
}
getLogger().log(Level.FINE, "getFrameworkComponentInfoForSharedLibraries: Params : {0}",
componentName);
List slibNames = new ArrayList();
ComponentQuery componentQuery = null;
if (compName == null) {
getLogger().log(Level.FINE, "Listing All Shared Libraries in the system");
componentQuery = this.getEnvironmentContext().getComponentQuery();
if (componentQuery != null) {
slibNames = componentQuery
.getComponentIds(ComponentType.SHARED_LIBRARY);
}
} else {
getLogger().log(Level.FINE, "Listing Shared Libraries for the component {0}", compName);
componentQuery = this.getEnvironmentContext().getComponentQuery();
if (componentQuery != null) {
ComponentInfo componentInfo = componentQuery
.getComponentInfo(compName);
if (componentInfo == null) {
Exception exception = new Exception("ui.mbean.component.id.does.not.exist");
throw new ManagementException(exception);
}
slibNames = componentInfo.getSharedLibraryNames();
}
}
return this.getFrameworkComponentInfoListForSharedLibraryNames(slibNames);
}
@Override
public String install(String slZipURL) throws ManagementException {
try {
return getInstallationServiceMBean().installSharedLibrary(slZipURL);
} catch (Exception e) {
throw this.createManagementException(e.getMessage());
}
}
@Override
public void uninstall(String sharedLibraryName) throws ManagementException {
checkSharedLibraryExists(sharedLibraryName);
try {
getInstallationServiceMBean().uninstallSharedLibrary(sharedLibraryName);
} catch (Exception e) {
throw this.createManagementException(e.getMessage());
}
}
@Override
public SharedLibrary getSharedLibrary(String sharedLibraryName) throws ManagementException {
checkSharedLibraryExists(sharedLibraryName);
Set libraries = findSharedLibraries(null);
for (SharedLibrary library : libraries) {
if (library.getName().equalsIgnoreCase(sharedLibraryName)) {
library.setComponentDependencies(
new HashSet(getComponentNamesDependentOnSharedLibrary(sharedLibraryName)));
return library;
}
}
return null;
}
@Override
public ComponentDescriptor getDescriptor(String sharedLibraryName) throws ManagementException {
checkSharedLibraryExists(sharedLibraryName);
ComponentQuery componentQuery = getEnvironmentContext().getComponentQuery();
if (componentQuery != null) {
com.sun.jbi.ComponentInfo retrievedInfo =
componentQuery.getSharedLibraryInfo(sharedLibraryName);
net.openesb.model.api.ComponentDescriptor info = new net.openesb.model.api.ComponentDescriptor();
info.setName(retrievedInfo.getName());
info.setDescription(retrievedInfo.getDescription());
info.setBootstrapClassLoaderSelfFirst(retrievedInfo.isBootstrapClassLoaderSelfFirst());
info.setBootstrapClassName(retrievedInfo.getBootstrapClassName());
info.setBootstrapClassPathElements(retrievedInfo.getBootstrapClassPathElements());
info.setClassLoaderSelfFirst(retrievedInfo.isClassLoaderSelfFirst());
info.setClassPathElements(retrievedInfo.getClassPathElements());
info.setComponentClassName(retrievedInfo.getComponentClassName());
info.setInstallRoot(retrievedInfo.getInstallRoot());
info.setSharedLibraries(retrievedInfo.getSharedLibraryNames());
info.setWorkspaceRoot(retrievedInfo.getWorkspaceRoot());
return info;
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy