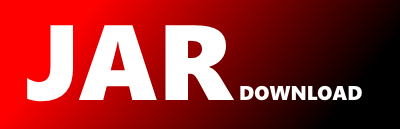
net.openesb.management.jmx.StatisticsServiceImpl Maven / Gradle / Ivy
package net.openesb.management.jmx;
import java.util.Collections;
import java.util.Date;
import java.util.HashMap;
import java.util.Map;
import java.util.logging.Level;
import javax.management.openmbean.CompositeData;
import javax.management.openmbean.CompositeType;
import javax.management.openmbean.OpenDataException;
import net.openesb.model.api.ConsumingEndpointStatistics;
import net.openesb.model.api.EndpointStatistics;
import net.openesb.model.api.ProvidingEndpointStatistics;
import net.openesb.model.api.Statistic;
import net.openesb.model.api.metric.Metric;
import net.openesb.management.api.ManagementException;
import net.openesb.management.api.StatisticsService;
/**
*
* @author David BRASSELY (brasseld at gmail.com)
* @author OpenESB Community
*/
public class StatisticsServiceImpl extends AbstractServiceImpl implements StatisticsService {
/**
* attr. name for last restart time in component statistics mbean
*/
static String COMPONENT_LAST_RESTART_TIME_ATTR = "LastRestartTime";
/**
* item name for active endpoints in component statistics data
*/
static String COMPONENT_ACTIVE_ENDPOINTS = "ActiveEndpoints";
/**
* item name for number of received requests in component statistics data
*/
static String COMPONENT_RECEIVE_REQUEST = "ReceiveRequest";
/**
* item name for number of sent requests in component statistics data
*/
static String COMPONENT_SEND_REQUEST = "SendRequest";
/**
* item name for number of received replies in component statistics data
*/
static String COMPONENT_RECEIVE_REPLY = "ReceiveReply";
/**
* item name for number of sent replies in component statistics data
*/
static String COMPONENT_SEND_REPLY = "SendReply";
/**
* item name for number of received DONEs in component statistics data
*/
static String COMPONENT_RECEIVE_DONE = "ReceiveDONE";
/**
* item name for number of sent DONEs requests in component statistics data
*/
static String COMPONENT_SEND_DONE = "SendDONE";
/**
* item name for number of received faults in component statistics data
*/
static String COMPONENT_RECEIVE_FAULT = "ReceiveFault";
/**
* item name for number of sent faults in component statistics data
*/
static String COMPONENT_SEND_FAULT = "SendFault";
/**
* item name for number of received errors in component statistics data
*/
static String COMPONENT_RECEIVE_ERROR = "ReceiveERROR";
/**
* item name for number of sent errors in component statistics data
*/
static String COMPONENT_SEND_ERROR = "SendERROR";
/**
* item name for number of active exchangesin component statistics data
*/
static String COMPONENT_ACTIVE_EXCHANGE = "ActiveExchanges";
/**
* item name for number of active exchangesin component statistics data
*/
static String COMPONENT_ACTIVE_EXCHANGE_MAX = "MaxActiveExchanges";
/**
* item name for number of active exchangesin component statistics data
*/
static String COMPONENT_QUEUED_EXCHANGE = "QueuedExchanges";
/**
* item name for number of active exchangesin component statistics data
*/
static String COMPONENT_QUEUED_EXCHANGE_MAX = "MaxQueuedExchanges";
/**
* item name for response time in component statistics data
*/
static String COMPONENT_RESPONSE_TIME = "ResponseTimeAvg (ns)";
/**
* item name for component time in component statistics data
*/
static String COMPONENT_COMPONENT_TIME = "ComponentTimeAvg (ns)";
/**
* item name for channel time in component statistics data
*/
static String COMPONENT_CHANNEL_TIME = "ChannelTimeAvg (ns)";
/**
* item name for nmr time in component statistics data
*/
static String COMPONENT_NMR_TIME = "NMRTimeAvg (ns)";
/**
* item name for owning component in endpoint statistics data
*/
static String OWNING_COMPONENT = "OwningChannel";
/**
* item name for activation time stamp in endpoint statistics data
*/
static String PROVIDER_ACTIVATION_TIMESTAMP = "ActivationTimestamp";
/**
* item name for status time in endpoint statistics data
*/
static String COMPONENT_STATUS_TIME = "StatusTimeAvg (ns)";
/**
* endpoint stats items - provider specific used to find out if the endpoint
* is a provider
*/
static String[] ENDPOINT_STATS_PROVIDER_ITEM_NAMES = {
PROVIDER_ACTIVATION_TIMESTAMP,
COMPONENT_RECEIVE_REQUEST,
COMPONENT_SEND_REPLY,};
@Override
public Map getEndpointStatistics(String endpointName) throws ManagementException {
CompositeData epDataStats = getMessageServiceStatisticsMBean().getEndpointStatistics(endpointName);
boolean isProvider = true;
try {
epDataStats.getAll(ENDPOINT_STATS_PROVIDER_ITEM_NAMES);
} catch (javax.management.openmbean.InvalidKeyException invalidKeyException) {
getLogger().log(Level.FINE, "All provider items are not present, could be consuming endpoint");
isProvider = false;
}
return convert(epDataStats);
/*
EndpointStatistics stats = null;
if (isProvider) {
stats = composeProviderEndpointStats(epDataStats);
} else {
stats = composeConsumerEndpointStats(epDataStats);
}
stats.setOwningComponent((String) epDataStats.get(OWNING_COMPONENT));
stats.setActiveExchanges((Long) epDataStats.get(COMPONENT_ACTIVE_EXCHANGE));
stats.setReceivedDones((Long) epDataStats.get(COMPONENT_RECEIVE_DONE));
stats.setSentDones((Long) epDataStats.get(COMPONENT_SEND_DONE));
stats.setReceivedFaults((Long) epDataStats.get(COMPONENT_RECEIVE_FAULT));
stats.setSentFaults((Long) epDataStats.get(COMPONENT_SEND_FAULT));
stats.setReceivedErrors((Long) epDataStats.get(COMPONENT_RECEIVE_ERROR));
stats.setSentErrors((Long) epDataStats.get(COMPONENT_SEND_ERROR));
return stats;
*/
}
@Override
public Map getComponentStatistics(String componentName) throws ManagementException {
checkComponentExists(componentName);
CompositeData compData =
getMessageServiceStatisticsMBean()
.getDeliveryChannelStatistics(componentName);
return convert(compData);
}
@Override
public Map getServiceAssemblyStatistics(String serviceAssemblyName) throws ManagementException {
try {
CompositeData assemblyData = getDeploymentServiceStatisticsMBean()
.getServiceAssemblyStatistics(serviceAssemblyName);
return convert(assemblyData);
} catch (OpenDataException ode) {
}
return null;
}
private Map convert(CompositeData compositeData) {
CompositeType compositeType = compositeData.getCompositeType();
Map metrics = new HashMap(
compositeType.keySet().size());
for (String statKey : compositeType.keySet()) {
Object value = compositeData.get(statKey);
if (value instanceof CompositeData) {
value = convert((CompositeData) value);
}
String description = compositeType.getDescription(statKey);
metrics.put(statKey, new Statistic
© 2015 - 2025 Weber Informatics LLC | Privacy Policy