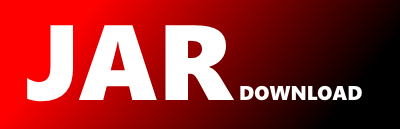
net.openesb.rest.api.resources.ComponentResource Maven / Gradle / Ivy
The newest version!
package net.openesb.rest.api.resources;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.ObjectMapper;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import java.util.Map;
import java.util.logging.Level;
import javax.inject.Inject;
import javax.ws.rs.Consumes;
import javax.ws.rs.DELETE;
import javax.ws.rs.DefaultValue;
import javax.ws.rs.GET;
import javax.ws.rs.POST;
import javax.ws.rs.PUT;
import javax.ws.rs.Path;
import javax.ws.rs.Produces;
import javax.ws.rs.QueryParam;
import javax.ws.rs.container.ResourceContext;
import javax.ws.rs.core.Context;
import javax.ws.rs.core.MediaType;
import javax.ws.rs.core.Response;
import net.openesb.model.api.ComponentDescriptor;
import net.openesb.model.api.Configuration;
import net.openesb.model.api.JBIComponent;
import net.openesb.model.api.metric.Metric;
import net.openesb.management.api.ComponentService;
import net.openesb.management.api.ConfigurationService;
import net.openesb.management.api.ManagementException;
import net.openesb.management.api.StatisticsService;
import net.openesb.rest.api.annotation.RequiresAuthentication;
import net.openesb.rest.api.json.MetricsModule;
import org.glassfish.jersey.media.multipart.FormDataContentDisposition;
import org.glassfish.jersey.media.multipart.FormDataParam;
/**
*
* @author David BRASSELY (brasseld at gmail.com)
* @author OpenESB Community
*/
@RequiresAuthentication
public class ComponentResource extends AbstractResource {
private static final ObjectMapper mapper = new ObjectMapper().registerModules(
new MetricsModule());
@Inject
private ComponentService componentService;
@Inject
private StatisticsService statisticsService;
@Inject
private ConfigurationService configurationService;
@Inject
private ResourceContext resourceContext;
private final String componentName;
public ComponentResource(String componentName) {
this.componentName = componentName;
}
@GET
@Produces(MediaType.APPLICATION_JSON)
public JBIComponent getComponent() throws ManagementException {
return componentService.getComponent(componentName);
}
@GET
@Path("descriptor")
@Produces(MediaType.APPLICATION_JSON)
public ComponentDescriptor getComponentDescriptor() throws ManagementException {
return componentService.getDescriptor(componentName);
}
@GET
@Path("descriptor")
@Produces(MediaType.APPLICATION_XML)
public String getComponentDescriptorAsXML() throws ManagementException {
return componentService.getDescriptorAsXml(componentName);
}
@DELETE
public Response uninstall(@DefaultValue("true") @QueryParam("force") boolean force) throws ManagementException {
componentService.uninstall(componentName, force);
return Response.ok().build();
}
@PUT
@Consumes(MediaType.MULTIPART_FORM_DATA)
public Response upgrade(@FormDataParam("component") InputStream is,
@FormDataParam("component") FormDataContentDisposition fileDisposition) throws ManagementException {
File compArchive = null;
try {
compArchive = createTemporaryFile(is, fileDisposition.getFileName());
} catch (Exception e) {
getLogger().log(Level.SEVERE, "I/O errors while uploading the component archive.", e);
return Response.serverError().build();
} finally {
if (is != null) {
try {
is.close();
} catch (IOException ex) {
getLogger().log(Level.SEVERE, null, ex);
}
}
}
if (compArchive != null) {
componentService.upgrade(componentName, compArchive.toURI().toString());
return Response.ok().build();
}
return Response.serverError().build();
}
@POST
public Response doLifecycleAction(@QueryParam("action") LifecycleActionParam action,
@DefaultValue("false") @QueryParam("force") boolean force) throws ManagementException {
getLogger().log(Level.FINE, "Do lifecycle action {0} for component {1}",
new Object[]{action.getAction(), componentName});
switch (action.getAction()) {
case START:
componentService.start(componentName);
break;
case STOP:
componentService.stop(componentName);
break;
case SHUTDOWN:
componentService.shutdown(componentName, force);
break;
default:
getLogger().log(Level.WARNING, "Unknown action {0} for component {1}",
new Object[]{action.getAction(), componentName});
break;
}
return Response.ok().build();
}
@Path("stats")
@GET
@Produces(MediaType.APPLICATION_JSON)
public String getStatistics() throws ManagementException, JsonProcessingException {
return mapper.writeValueAsString(statisticsService.getComponentStatistics(componentName));
}
@GET
@Path("schema")
@Produces(MediaType.APPLICATION_JSON)
public Configuration getConfigurationSchema() throws ManagementException {
return configurationService.getConfigurationSchema(componentName);
}
@Path("endpoints")
public EndpointsResource getEndpointsResource() {
return resourceContext.initResource(
new EndpointsResource(componentName));
}
@Path("loggers")
public ComponentLoggersResource getLoggersResource() {
return resourceContext.initResource(
new ComponentLoggersResource(componentName));
}
@Path("configuration")
public ComponentConfigurationResource getComponentConfigurationResource() {
return resourceContext.initResource(
new ComponentConfigurationResource(componentName));
}
@Path("application")
public ComponentApplicationResource getComponentApplicationResource() {
return resourceContext.initResource(
new ComponentApplicationResource(componentName));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy