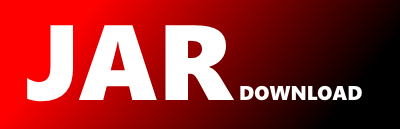
net.openesb.rest.api.resources.EndpointsResource Maven / Gradle / Ivy
The newest version!
package net.openesb.rest.api.resources;
import java.util.Map;
import java.util.Set;
import javax.inject.Inject;
import javax.ws.rs.Consumes;
import javax.ws.rs.FormParam;
import javax.ws.rs.GET;
import javax.ws.rs.POST;
import javax.ws.rs.Path;
import javax.ws.rs.Produces;
import javax.ws.rs.core.Context;
import javax.ws.rs.core.MediaType;
import net.openesb.model.api.Endpoint;
import net.openesb.management.api.EndpointService;
import net.openesb.management.api.ManagementException;
import net.openesb.management.api.StatisticsService;
import net.openesb.model.api.metric.Metric;
import net.openesb.rest.api.annotation.RequiresAuthentication;
/**
*
* @author David BRASSELY (brasseld at gmail.com)
* @author OpenESB Community
*/
@RequiresAuthentication
public class EndpointsResource extends AbstractResource {
@Inject
private EndpointService endpointService;
@Inject
private StatisticsService statisticsService;
private final String owner;
/**
* In the case of NMR.
*/
public EndpointsResource() {
this.owner = null;
}
/**
* In the case of component.
* @param ownerId Component name.
*/
public EndpointsResource(String ownerId) {
this.owner = ownerId;
}
@GET
@Produces(MediaType.APPLICATION_JSON)
public Set findAllEndpoints() throws ManagementException {
Set endpoints = endpointService.findEndpoints(owner, true);
endpoints.addAll(endpointService.findEndpoints(owner, false));
return endpoints;
}
@GET
@Path("out")
@Produces(MediaType.APPLICATION_JSON)
public Set findProvidingEndpoints() throws ManagementException {
return endpointService.findEndpoints(owner, false);
}
@GET
@Path("in")
@Produces(MediaType.APPLICATION_JSON)
public Set findConsumingEndpoints() throws ManagementException {
return endpointService.findEndpoints(owner, true);
}
@POST
@Produces(MediaType.APPLICATION_JSON)
@Consumes(MediaType.APPLICATION_FORM_URLENCODED)
public Map getStatistics(@FormParam("endpoint") String endpointName) throws ManagementException {
return statisticsService.getEndpointStatistics(endpointName);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy