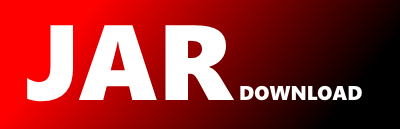
net.openesb.rest.api.resources.SharedLibrariesResource Maven / Gradle / Ivy
The newest version!
package net.openesb.rest.api.resources;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import java.util.Comparator;
import java.util.Set;
import java.util.TreeSet;
import java.util.logging.Level;
import javax.inject.Inject;
import javax.ws.rs.Consumes;
import javax.ws.rs.GET;
import javax.ws.rs.POST;
import javax.ws.rs.Path;
import javax.ws.rs.PathParam;
import javax.ws.rs.Produces;
import javax.ws.rs.QueryParam;
import javax.ws.rs.container.ResourceContext;
import javax.ws.rs.core.Context;
import javax.ws.rs.core.MediaType;
import javax.ws.rs.core.Response;
import net.openesb.model.api.SharedLibrary;
import net.openesb.management.api.ManagementException;
import net.openesb.management.api.SharedLibraryService;
import net.openesb.rest.api.annotation.RequiresAuthentication;
import org.glassfish.jersey.media.multipart.FormDataContentDisposition;
import org.glassfish.jersey.media.multipart.FormDataParam;
/**
*
* @author David BRASSELY (brasseld at gmail.com)
* @author OpenESB Community
*/
@Path("libraries")
@RequiresAuthentication
public class SharedLibrariesResource extends AbstractResource {
@Inject
private SharedLibraryService sharedLibraryService;
@Inject
private ResourceContext resourceContext;
@GET
@Produces(MediaType.APPLICATION_JSON)
public Set listSharedLibraries(
@QueryParam("component") String componentName)
throws ManagementException {
Set libraries = new TreeSet(new Comparator() {
@Override
public int compare(SharedLibrary lib1, SharedLibrary lib2) {
return lib1.getName().compareTo(lib2.getName());
}
});
libraries.addAll(sharedLibraryService.findSharedLibraries(componentName));
return libraries;
}
@POST
@Consumes(MediaType.MULTIPART_FORM_DATA)
public Response install(@FormDataParam("sharedLibrary") InputStream is,
@FormDataParam("sharedLibrary") FormDataContentDisposition fileDisposition) throws ManagementException {
File slArchive = null;
try {
slArchive = createTemporaryFile(is, fileDisposition.getFileName());
} catch (Exception e) {
getLogger().log(Level.SEVERE, "I/O errors while uploading the sharedlibraries archive.", e);
return Response.serverError().build();
} finally {
if (is != null) {
try {
is.close();
} catch (IOException ex) {
getLogger().log(Level.SEVERE, null, ex);
}
}
}
if (slArchive != null) {
String sharedLibraryName = sharedLibraryService.install(slArchive.toURI().toString());
return Response.ok().entity(sharedLibraryName).build();
}
return Response.serverError().build();
}
@Path("{sharedLibrary}")
public SharedLibraryResource getSharedLibraryResource(@PathParam("sharedLibrary") String sharedLibraryName) {
return resourceContext.initResource(
new SharedLibraryResource(sharedLibraryName));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy