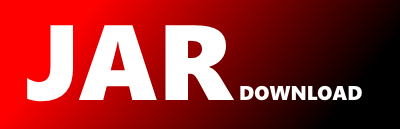
com.sun.jbi.ui.client.AbstractJMXClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jbi-admin-common Show documentation
Show all versions of jbi-admin-common Show documentation
The "jbi-admin-common" Common CLI code
The newest version!
/*
* BEGIN_HEADER - DO NOT EDIT
*
* The contents of this file are subject to the terms
* of the Common Development and Distribution License
* (the "License"). You may not use this file except
* in compliance with the License.
*
* You can obtain a copy of the license at
* https://open-esb.dev.java.net/public/CDDLv1.0.html.
* See the License for the specific language governing
* permissions and limitations under the License.
*
* When distributing Covered Code, include this CDDL
* HEADER in each file and include the License file at
* https://open-esb.dev.java.net/public/CDDLv1.0.html.
* If applicable add the following below this CDDL HEADER,
* with the fields enclosed by brackets "[]" replaced with
* your own identifying information: Portions Copyright
* [year] [name of copyright owner]
*/
/*
* @(#)AbstractJMXClient.java
* Copyright 2004-2007 Sun Microsystems, Inc. All Rights Reserved.
*
* END_HEADER - DO NOT EDIT
*/
package com.sun.jbi.ui.client;
import com.sun.jbi.ui.common.I18NBundle;
import com.sun.jbi.ui.common.JBIJMXObjectNames;
import com.sun.jbi.ui.common.JBIRemoteException;
import com.sun.jbi.ui.common.JMXConnectionException;
import javax.management.MalformedObjectNameException;
import javax.management.ObjectName;
/** This class is a abstract base class for jmx client implementations.
*
* @author Sun Microsystems, Inc.
*/
public abstract class AbstractJMXClient
{
/** i18n */
private static I18NBundle sI18NBundle = null;
/** JMX Client interface*/
protected JMXConnection mJmxConnection;
/**
* constructor
*/
protected AbstractJMXClient()
{
}
/** gives the I18N bundle
*@return I18NBundle object
*/
protected static I18NBundle getI18NBundle()
{
// lazzy initialize the JBI Client
if ( sI18NBundle == null )
{
sI18NBundle = new I18NBundle("com.sun.jbi.ui.client");
}
return sI18NBundle;
}
/**
* returns jmx connection object.
* @return jmx connection object.
*/
protected JMXConnection getJMXConnection()
{
return this.mJmxConnection;
}
/**
* sets the jmx connection object.
* @param jmxConn jmx connection object.
*/
protected void setJMXConnection(JMXConnection jmxConn)
{
this.mJmxConnection = jmxConn;
}
/**
* opens the jmx connection
* @throws JBIRemoteException on error
*/
protected void openJmxConnection() throws JBIRemoteException
{
try
{
this.mJmxConnection.openConnection();
}
catch (JMXConnectionException jmxEx )
{
// JMXConnectionException now carries jbi mgmt msg xml with serialized root casuse info
// so just pass the exception
throw new JBIRemoteException(jmxEx);
}
}
/**
* closes the jmx connection
* @throws JBIRemoteException on error
*/
protected void closeJmxConnection() throws JBIRemoteException
{
try
{
this.mJmxConnection.closeConnection();
}
catch (JMXConnectionException jmxEx )
{
throw new JBIRemoteException(jmxEx);
}
}
/**
* open connection, invokes the operation on mbean and closes connection.
* This should not be used if the connection is already opened or not to be close
* after the invoke operation.
* @return result object
* @param objectName object name
* @param operationName operation name
* @param params parameters
* @param signature signature of the parameters
* @throws JBIRemoteException on error
*/
public Object invokeMBeanOperation(ObjectName objectName,
String operationName, Object[] params, String[] signature)
throws JBIRemoteException
{
Object result = null;
openJmxConnection();
try
{
result = this.mJmxConnection.invokeMBeanOperation(objectName,
operationName, params, signature );
}
catch (JMXConnectionException jmxEx)
{
throw new JBIRemoteException(jmxEx);
}
catch (JBIRemoteException jbiREx)
{
throw jbiREx;
}
finally
{
try
{
closeJmxConnection();
}
catch (Exception ex)
{
// this is clean up. so ignore.
// log it.
}
}
return result;
}
/**
* single param opeartion invocation.
* This should not be used if the connection is already opened or not to be close
* after the invoke operation.
* @return result object
* @param objectName object name
* @param operationName operation name
* @param param String parameter
* @throws JBIRemoteException on error
*/
public Object invokeMBeanOperation(ObjectName objectName,
String operationName, String param)
throws JBIRemoteException
{
Object result = null;
Object[] params = new Object[1];
params[0] = param;
String[] signature = new String[1];
signature[0] = "java.lang.String";
return invokeMBeanOperation(objectName,operationName,params, signature);
}
/**
* single param opeartion invocation.
* This should not be used if the connection is already opened or not to be close
* after the invoke operation.
* @return result object
* @param objectName object name
* @param operationName operation name
* @param param1 String parameter
* @param param2 String parameter
* @throws JBIRemoteException on error
*/
public Object invokeMBeanOperation(ObjectName objectName,
String operationName, String param1, String param2)
throws JBIRemoteException
{
Object result = null;
Object[] params = new Object[2];
params[0] = param1;
params[1] = param2;
String[] signature = new String[2];
signature[0] = "java.lang.String";
signature[1] = "java.lang.String";
return invokeMBeanOperation(objectName,operationName,params, signature);
}
/**
* single param opeartion invocation.
* This should not be used if the connection is already opened or not to be close
* after the invoke operation.
* @return result object
* @param objectName object name
* @param operationName operation name
* @param param1 String parameter
* @param param2 String parameter
* @param param3 String parameter
* @throws JBIRemoteException on error
*/
public Object invokeMBeanOperation(ObjectName objectName,
String operationName, String param1, String param2, String param3)
throws JBIRemoteException
{
Object result = null;
Object[] params = new Object[3];
params[0] = param1;
params[1] = param2;
params[2] = param3;
String[] signature = new String[3];
signature[0] = "java.lang.String";
signature[1] = "java.lang.String";
signature[2] = "java.lang.String";
return invokeMBeanOperation(objectName,operationName,params, signature);
}
/**
* single param opeartion invocation.
* This should not be used if the connection is already opened or not to be close
* after the invoke operation.
* @return result object
* @param param4 String parameter.
* @param objectName object name
* @param operationName operation name
* @param param1 String parameter
* @param param2 String parameter
* @param param3 String parameter
* @throws JBIRemoteException on error
*/
public Object invokeMBeanOperation(ObjectName objectName,
String operationName, String param1, String param2, String param3, String param4)
throws JBIRemoteException
{
Object result = null;
Object[] params = new Object[4];
params[0] = param1;
params[1] = param2;
params[2] = param3;
params[3] = param4;
String[] signature = new String[4];
signature[0] = "java.lang.String";
signature[1] = "java.lang.String";
signature[2] = "java.lang.String";
signature[3] = "java.lang.String";
return invokeMBeanOperation(objectName,operationName,params, signature);
}
/**
* open connection, invokes the operation on mbean and closes connection.
* This should not be used if the connection is already opened or not to be close
* after the invoke operation.
* @return result object
* @param objectName object name
* @param attributeName attribute name
* @throws JBIRemoteException on error
*/
public Object getMBeanAttribute(ObjectName objectName,
String attributeName)
throws JBIRemoteException
{
Object result = null;
openJmxConnection();
try
{
result = this.mJmxConnection.getMBeanAttribute(objectName,
attributeName );
}
catch (JMXConnectionException jmxEx)
{
throw new JBIRemoteException(jmxEx);
}
catch (JBIRemoteException jbiREx)
{
throw jbiREx;
}
finally
{
try
{
closeJmxConnection();
}
catch (Exception ex)
{
// this is clean up. so ignore.
// log it.
}
}
return result;
}
/**
* returns admin service mbean object name
* @throws JBIRemoteException on error
* @return object name
*/
public ObjectName getAdminServiceMBeanObjectName() throws JBIRemoteException
{
try
{
ObjectName mbeanName =
JBIJMXObjectNames.getAdminServiceMBeanObjectName();
return mbeanName;
}
catch (MalformedObjectNameException objEx)
{
throw new JBIRemoteException(objEx.getMessage(), objEx);
}
}
/**
* returns ui mbean jmx object name.
* @throws JBIRemoteException on error
* @return object jmx object name
*/
public ObjectName getJbiAdminUiMBeanObjectName() throws JBIRemoteException
{
try
{
ObjectName mbeanName =
JBIJMXObjectNames.getJbiAdminUiMBeanObjectName();
return mbeanName;
}
catch (MalformedObjectNameException objEx)
{
throw new JBIRemoteException(objEx.getMessage(), objEx);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy